Connect to MySQL database in C#
1. Download MySQL Connector for Dotnet
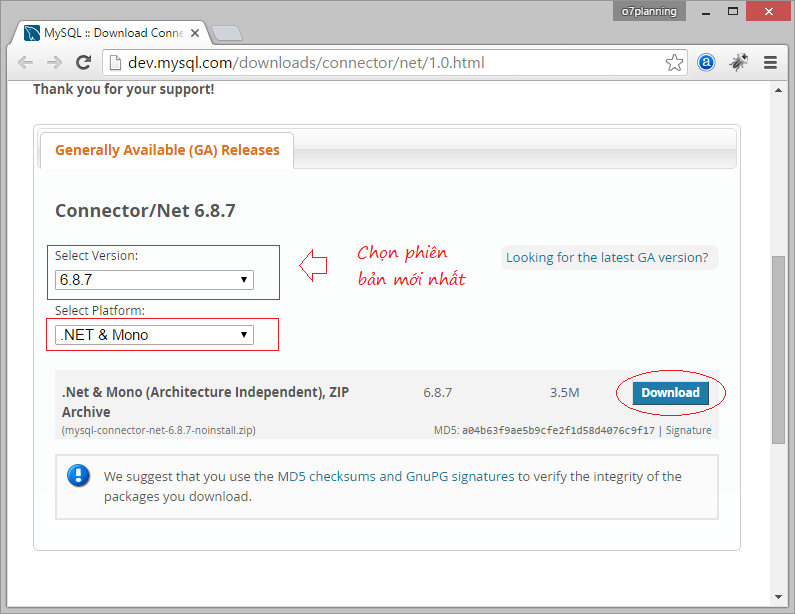
You need to login to download. You can register freely an account. This is the result downloaded:
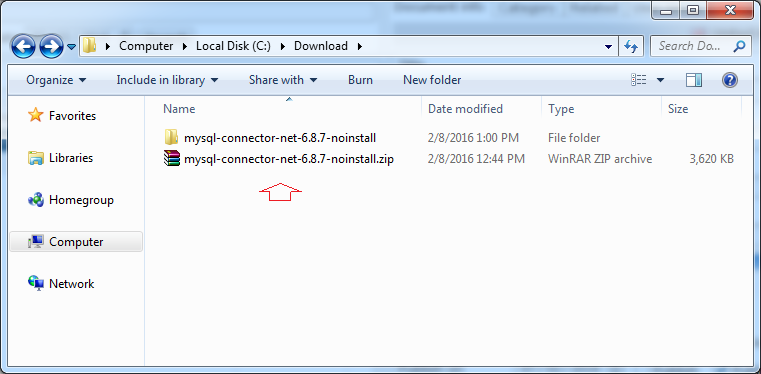
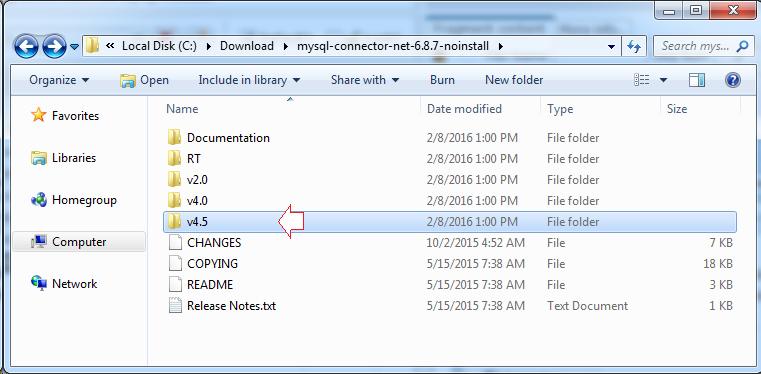
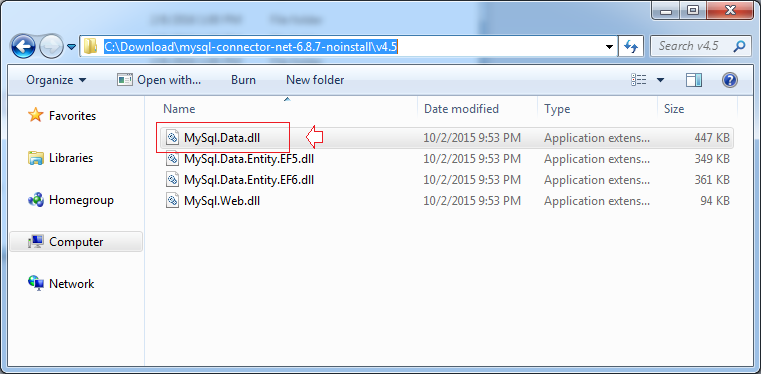
2. Connect to MySQL using C#
Create a Project named ConnectMySQL:
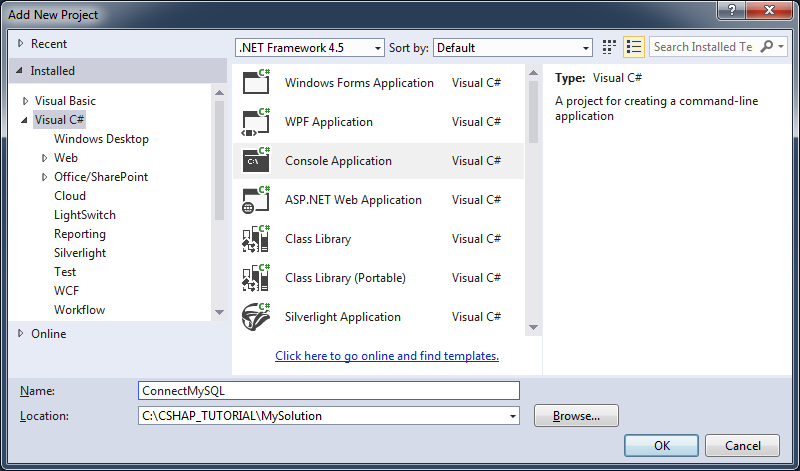
Project is created, you need to declare Reference with MySql.Data.dll.
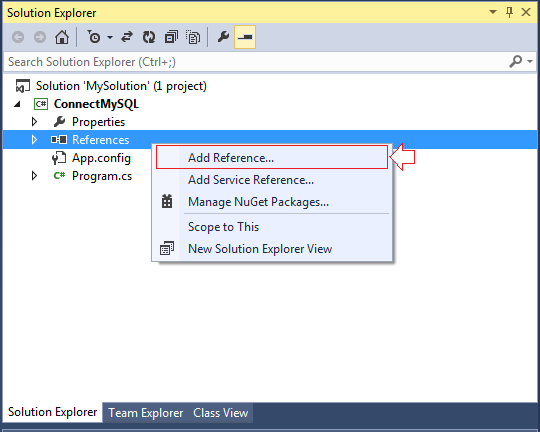
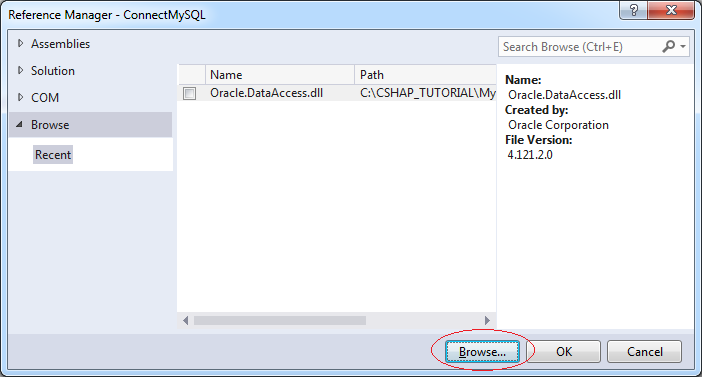
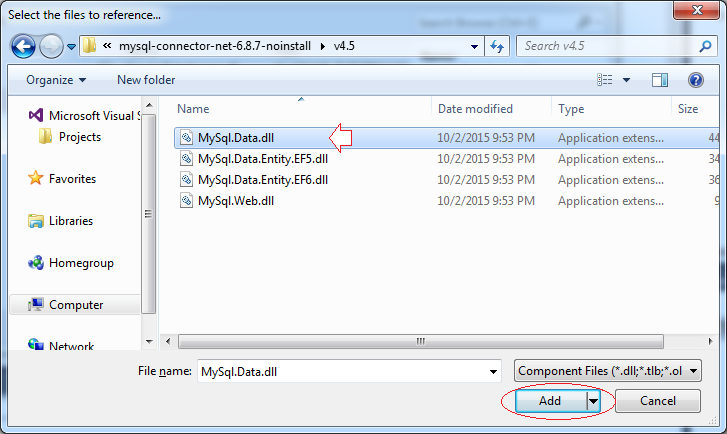
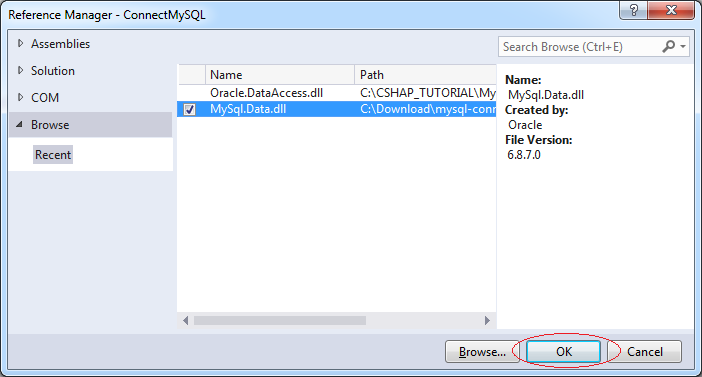
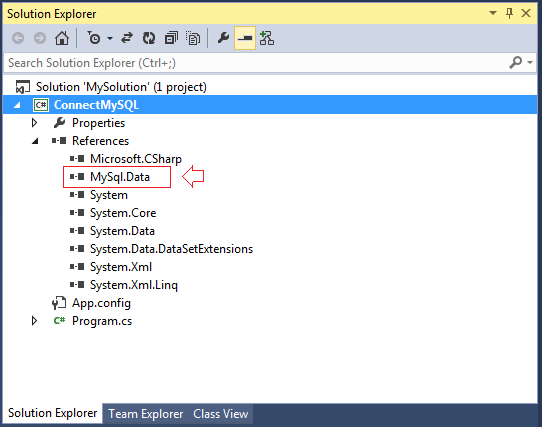
Create some utility class to help connect to the MySQL database:
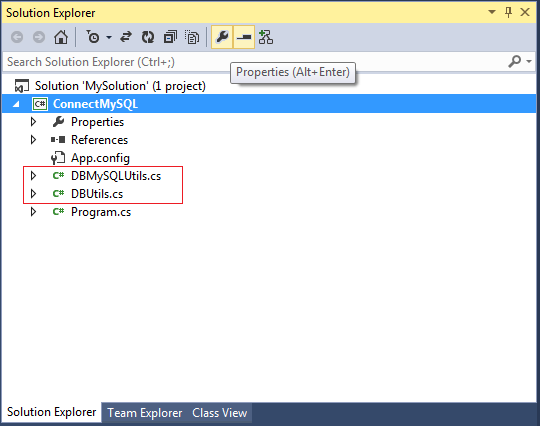
DBMySQLUtils.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using MySql.Data.MySqlClient;
namespace Tutorial.SqlConn
{
class DBMySQLUtils
{
public static MySqlConnection
GetDBConnection(string host, int port, string database, string username, string password)
{
// Connection String.
String connString = "Server=" + host + ";Database=" + database
+ ";port=" + port + ";User Id=" + username + ";password=" + password;
MySqlConnection conn = new MySqlConnection(connString);
return conn;
}
}
}
DBUtils.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using MySql.Data.MySqlClient;
namespace Tutorial.SqlConn
{
class DBUtils
{
public static MySqlConnection GetDBConnection( )
{
string host = "192.168.205.130";
int port = 3306;
string database = "simplehr";
string username = "root";
string password = "1234";
return DBMySQLUtils.GetDBConnection(host, port, database, username, password);
}
}
}
Test Connection:
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Tutorial.SqlConn;
using MySql.Data.MySqlClient;
namespace ConnectMySQL
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Getting Connection ...");
MySqlConnection conn = DBUtils.GetDBConnection();
try
{
Console.WriteLine("Openning Connection ...");
conn.Open();
Console.WriteLine("Connection successful!");
}
catch(Exception e)
{
Console.WriteLine("Error: " + e.Message);
}
Console.Read();
}
}
}
Running Program class to test the connection:
Getting Connection ...
Openning Connection ...
Connection successful!
3. Working with MySQL Database using C#
Next tutorial - Working with MySQL Database using C#:
Content included:
- Insert
- Update
- Delete
- Call Function and Procedure.
4. Appendix: Connection errors and how to fix it
In case you connect to MySQL Database in other computer, you may receive an error as illustrated below, which caused by MySQL is disabling connections from another computer, you need to configure MySQL to allow this. You can see the instructions at:
Error: Host '192.168.205.134' is not allowed to connect to this MySQL server
If your MySQL is installed in another compter (with Windows OS), you need to open firewall for port 3306 (On computer running MySQL)
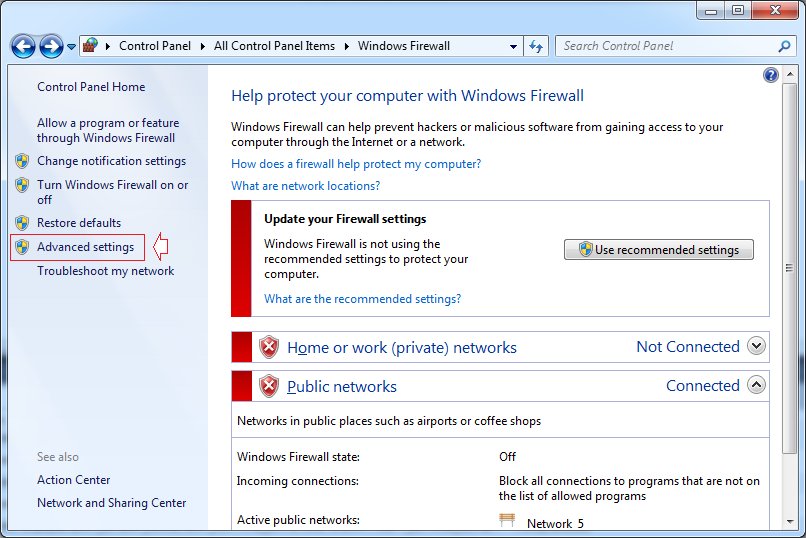
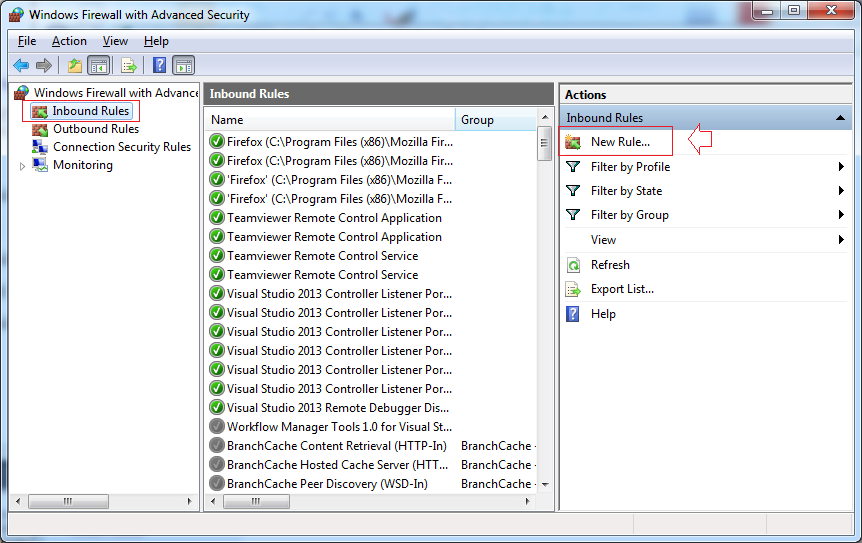
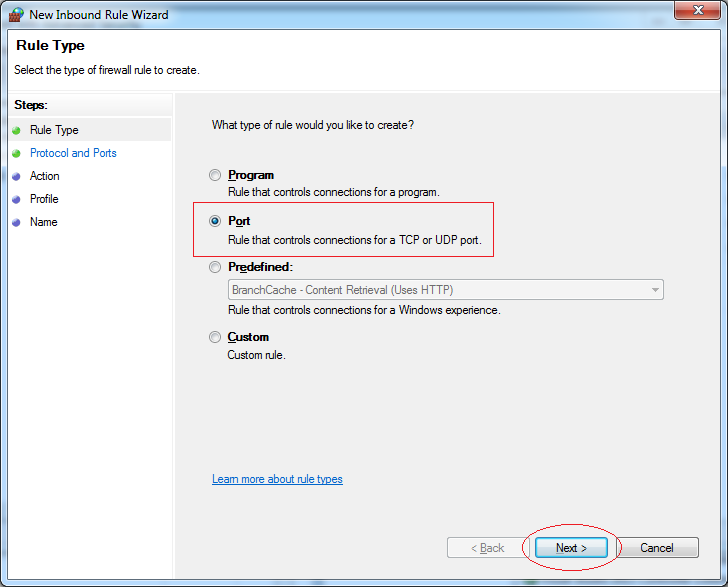
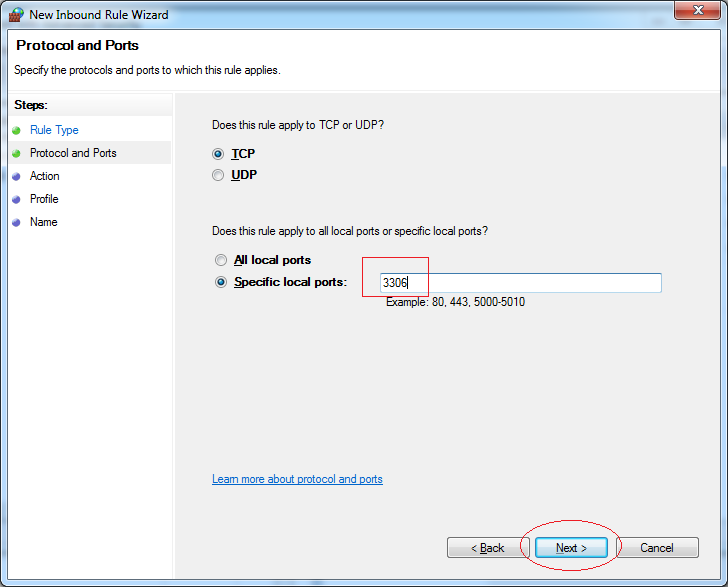
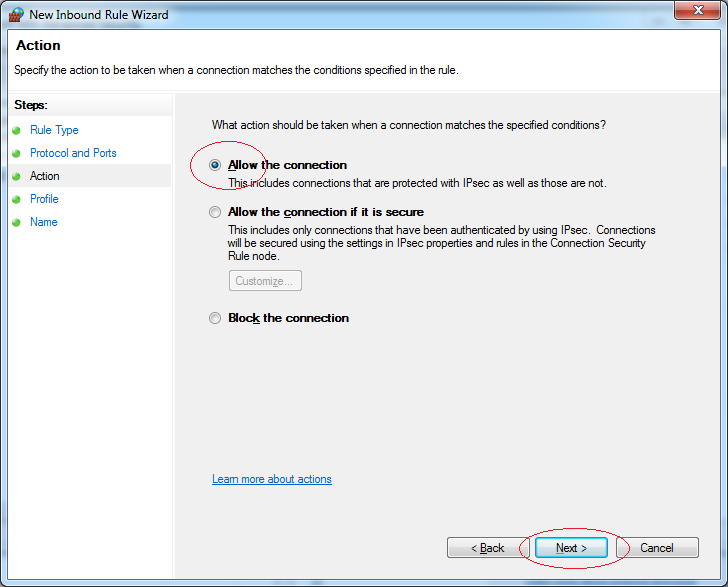
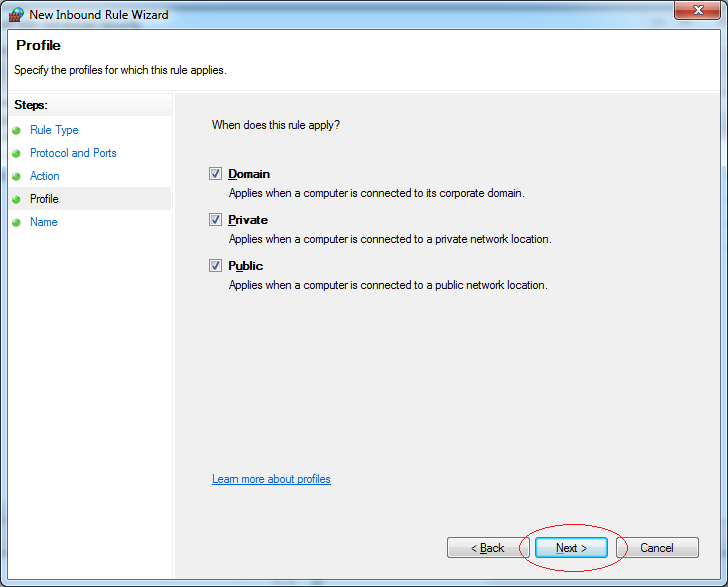
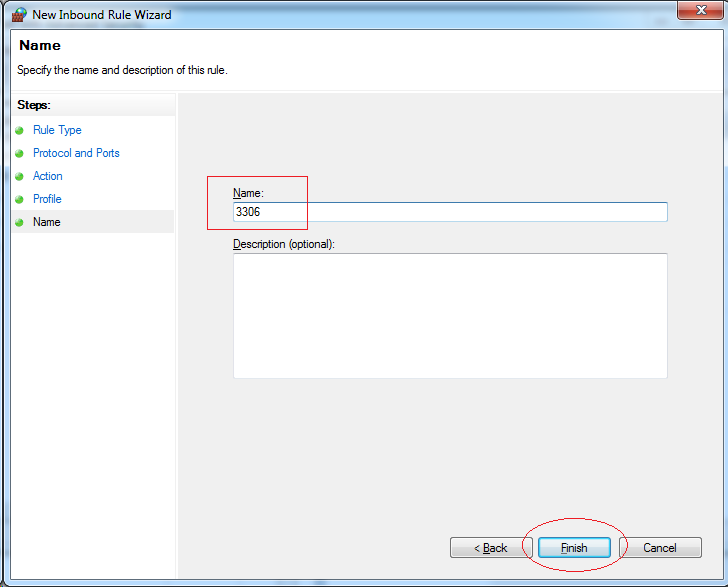
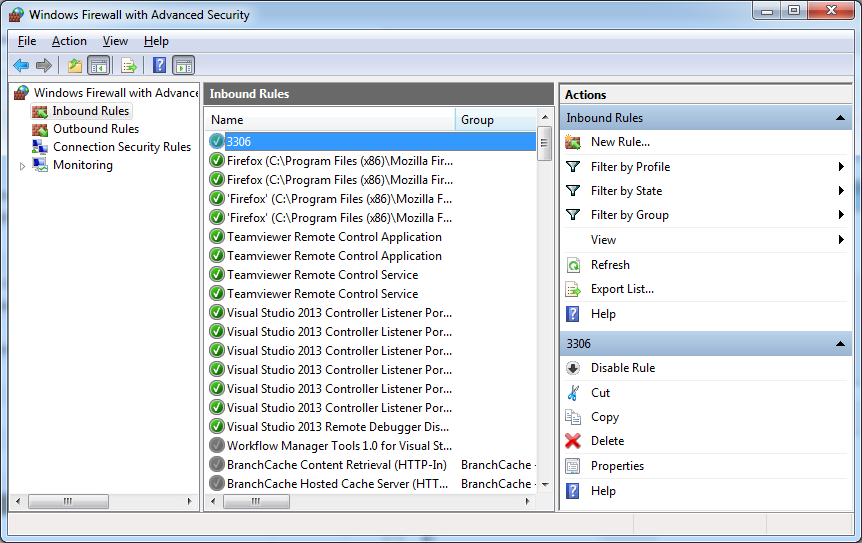
C# Programming Tutorials
- Inheritance and polymorphism in C#
- What is needed to get started with C#?
- Quick learning C# for Beginners
- Install Visual Studio 2013 on Windows
- Abstract class and Interface in C#
- Install Visual Studio 2015 on Windows
- Compression and decompression in C#
- C# Multithreading Programming Tutorial with Examples
- C# Delegates and Events Tutorial with Examples
- Install AnkhSVN on Windows
- C# Programming for Team using Visual Studio and SVN
- Install .Net Framework
- Access Modifier in C#
- C# String and StringBuilder Tutorial with Examples
- C# Properties Tutorial with Examples
- C# Enums Tutorial with Examples
- C# Structures Tutorial with Examples
- C# Generics Tutorial with Examples
- C# Exception Handling Tutorial with Examples
- C# Date Time Tutorial with Examples
- Manipulating files and directories in C#
- C# Streams tutorial - binary streams in C#
- C# Regular Expressions Tutorial with Examples
- Connect to SQL Server Database in C#
- Work with SQL Server database in C#
- Connect to MySQL database in C#
- Work with MySQL database in C#
- Connect to Oracle Database in C# without Oracle Client
- Work with Oracle database in C#
Show More