Python String Tutorial with Examples
1. Python String
String is the most common type in Python, and you often have to work with them. Note that there is no character type in Python, a simple character is called as a string of length 1.
There are two ways to declare a string written on a line by using single quotes or double quotes.
str1 = "Hello Python"
str2 = 'Hello Python'
str3 = "I'm from Vietnam"
str4 = 'This is a "Cat"! '
If you want to write string on multiple lines using a pair of 3 single quotes.
str = """Hello World
Hello Python"""
2. Access values in string
Python does not support character type, the character is considered as a string of length 1. The characters in string are indexed by starting index 0. You can access substrings via index.
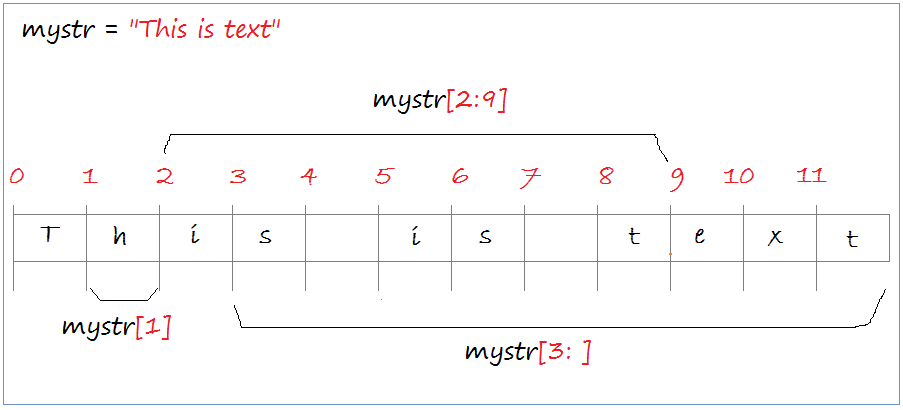
stringExample.py
mystr = "This is text"
# --> h
print ("mystr[1] = ", mystr[1])
# --> is is t
print ("mystr[2,9] = ", mystr[2:9])
# --> s is text
print ("mystr[3:] = ", mystr[3:])
3. String is immutable
String is a special data type in Python, and it is immutable. Each string has an address stored on memory. All operations on strings create another object. For example, if you want to concatenate a string with another string, this action creates another string on memory.
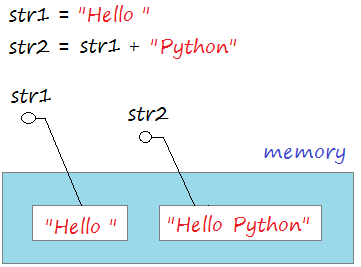
== and is operator
Python uses the == operator to compare the values of two objects. And use the "is" operator to compare locations on memory.
compareObject.py
class Person(object):
def __init__(self, name, age):
self.name = name
self.age = age
# Override __eq__ method
def __eq__(self, other):
return self.name == other.name and self.age == other.age
jack1 = Person('Jack', 23)
jack2 = Person('Jack', 23)
# Call to __eq__ method
print ("jack1 == jack2 ?", jack1 == jack2) # True
print ("jack1 is jack2 ?", jack1 is jack2) # False
See more:
- Comparing and Sorting in Python
String is a special data type and is frequently used in Python applications. And so it has some features below:
- If you declare two string type variable with the same value, they all will point to the actual string in memory.
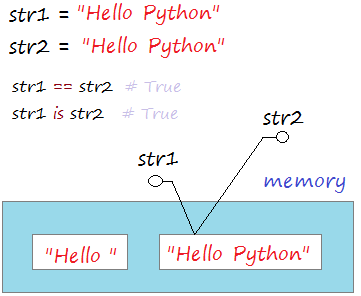
Operators with strings will create new strings in memory.
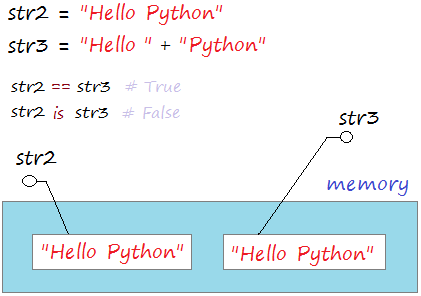
compareString.py
str1 = "Hello Python"
str2 = "Hello Python"
str3 = "Hello " + "Python"
print ("str1 == str2? ", str1 == str2) # --> True
print ("str1 is str2? ", str1 is str2) # --> True
print ("str1 == str3? ", str1 == str3) # --> True
print ("str1 is str3? ", str1 is str3) # --> False
4. Escape Characters
Escape characters are special characters in Python. It is non-printable characters. However if you want it to appear in your string, you need a notation to notify to Python. For example, "\n" is a newline character.
escapeCharacterExample.py
# Two "tab" characters between "Hello World" and "Hello Python".
mystr = "Hello World\t\tHello Python"
print (mystr)
# Two "newline" characters between "Hello World" and "Hello Python".
mystr = "Hello World\n\nHello Python"
print (mystr)
Output:
Hello World Hello Python
Hello World
Hello Python
Backslash
notation | Hexadecimal
character | Description |
\a | 0x07 | Bell or alert |
\b | 0x08 | Backspace |
\cx | Control-x | |
\C-x | Control-x | |
\e | 0x1b | Escape |
\f | 0x0c | Formfeed |
\M-\C-x | Meta-Control-x | |
\n | 0x0a | Newline |
\nnn | Octal notation, where n is in the range 0.7 | |
\r | 0x0d | Carriage return |
\s | 0x20 | Space |
\t | 0x09 | Tab |
\v | 0x0b | Vertical tab |
\x | Character x | |
\xnn | Hexadecimal notation, where n is in the range 0.9, a.f, or A.F |
5. String operators
In Python, there are some special operators below:
Operator | Description | Example |
+ | Concatenation - Adds values on either side of the operator | "Hello" +"Python" ==> "Hello Python" |
* | Repetition - Creates new strings, concatenating multiple copies of the same string | "Hello"*2 ==> "HelloHello" |
[] | Slice - Gives the character from the given index | a = "Hello"
a[1] ==> "e" |
[ : ] | Range Slice - Gives the characters from the given range | a = "Hello"
a[1:4] ==> "ell" a[1: ] ==> "ello" |
in | Membership - Returns true if a character exists in the given string | a = "Hello"
'H' in a ==> True |
not in | Membership - Returns true if a character does not exist in the given string | a = "Hello"
'M' not in a ==> True |
r/R | Raw String - Suppresses actual meaning of Escape characters. The syntax for raw strings is exactly the same as for normal strings with the exception of the "raw string operator", the letter "r," which precedes the quotation marks. The "r" can be lowercase (r) or uppercase (R) and must be placed immediately preceding the first quote mark. | print (r'\n\t') ==> \n\t
print (R'\n\t') ==> \n\t |
% | Format - Performs String formatting | See at next section |
Python Programming Tutorials
- Lookup Python documentation
- Branching statements in Python
- Python Function Tutorial with Examples
- Class and Object in Python
- Inheritance and polymorphism in Python
- Python Dictionary Tutorial with Examples
- Python Lists Tutorial with Examples
- Python Tuples Tutorial with Examples
- Python Date Time Tutorial with Examples
- Connect to MySQL Database in Python using PyMySQL
- Python exception handling Tutorial with Examples
- Python String Tutorial with Examples
- Introduction to Python
- Install Python on Windows
- Install Python on Ubuntu
- Install PyDev for Eclipse
- Conventions and Grammar versions in Python
- Python Tutorial for Beginners
- Python Loops Tutorial with Examples
Show More