Conventions and Grammar versions in Python
1. Conventions in Python
Like other languages, Python has some naming conventions such as naming rules of variable, function, class, module,...
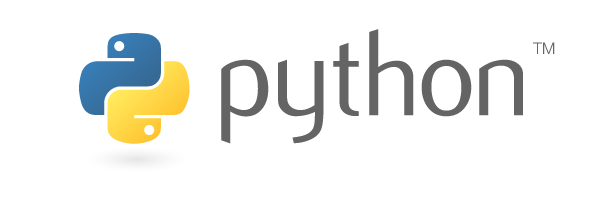
A name can begin with capital letters (A-Z), or lowercase ones (a-z), or undercore (_), followed by other letters or nothing.
Python does not accept the characters: @, $ and % in the name.
Python is a programming language that distinguishes between uppercase and lowercase, MyObject and myobject are two different ones.
Python does not accept the characters: @, $ and % in the name.
Python is a programming language that distinguishes between uppercase and lowercase, MyObject and myobject are two different ones.
Some naming rules in Python:
- Class name should begin with a capital letter, other names should begin with lowercase ones.
- A name begins with an underscore, it means that the name is private.
- A name begins with two underscores, it means that the name is very private.
- If a name bigins with two underscores and ends with two underscores, it means the name is special one that has already defined by Python.
2. The keywords in Python
There are a few the keywords in Python which can not be used to name and has no capital letters. Below is list of all the keywords in Python.
** keywords **
and assert break class
continue def del elif else
except exec finally for
from global if import in is
lambda not or pass
raise return try yield while
List of special words:
** special words **
None True False
self cls class_
The common functions:
** func **
__import__ abs all any apply
basestring bin bool buffer callable
chr classmethod cmp coerce
compile complex delattr dict dir
divmod enumerate eval execfile
file filter float format frozenset
getattr globals hasattr hash
help hex id input int intern
isinstance issubclass iter len
list locals long map max min next
object oct open ord pow print
property range raw_input reduce
reload repr reversed round set
setattr slice sorted staticmethod
str sum super tuple type type
unichr unicode vars xrange zip
3. Statement and Block
Unlike other programming languages, Python does not use the pairs of keywords such as “begin” and “end” or “{” và “}” to open or close a set of commands. Instead, Python makes rules that sequential commands having the same line indentation belongs in the same set of commands.
if True:
print ("Hello")
print ("True")
else:
print ("False")
You will get error report if you do it as below:
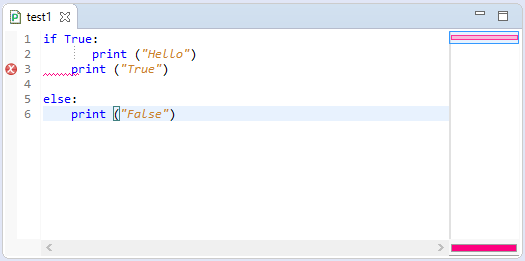
The rule of writing a statement on multiple lines:
Normally, a statement of Python will be written in 1 line, and a line break means the end of that statement. However, there are long statement that you want to write them on multiple lines, you need to inform Python of your intent. Let's use symbol \ to inform Python that the statement includes the next line. For example:
value = 1 + \
2 + \
3
The rules of writing multiple statements on a line
You can write multiple statements on a line, you need to use a semicolon (;) to separate them. For example:
a = 'One'; b = "Two"; c ="Three"
4. Rule writing a string
Python allows you to use single quotes ('), or double quotes (") to denote a string on a line:
str1 = 'Hello every body'
str2 = "Hello Python"
If a string is written on multiple lines, you need to use a pair of 3 quotes (And not use \):
multiLineStr = """This is a paragraph. It is
made up of multiple lines and sentences."""
5. Comment
The notation (#) not being in the string will begin with a comment line.
All the characters behind it until the end of line are considered a part of the comment and interpreter of Python and they will be ignored when running the programme.
All the characters behind it until the end of line are considered a part of the comment and interpreter of Python and they will be ignored when running the programme.
# First comment
print ("Hello, Python!") # second comment
# This is a comment.
# This is a comment, too.
# This is a comment, too.
print ("Finish")
6. Grammar Versions in Python
Currently, the latest version of Python is 3.x, Python 3.x has some stricter rules of grammar compared to Python 2.x, most the Python current documents on Internet are using Grammar 2.x and it can confuse you because you have carried out according to the guide but still getting error report.
Example:
In order to print out on the screen with the words "Hello World", for the version 2.x you need to use the "print" statement without round brackets ( ):
# Grammar Python 2.x
print "Hello World"
For the Python 3.x grammar, in order to print out the words "Hello World", you have to put it into round brackets ( ), or you will get error report.
# Grammar Python 3.x
print ("Hello World")
Therefore, when you create a project, you need to specify which the grammar version you use. The example below illustrates "Create a project" on Eclipse, and specifies "Python Grammar" version 3.6.
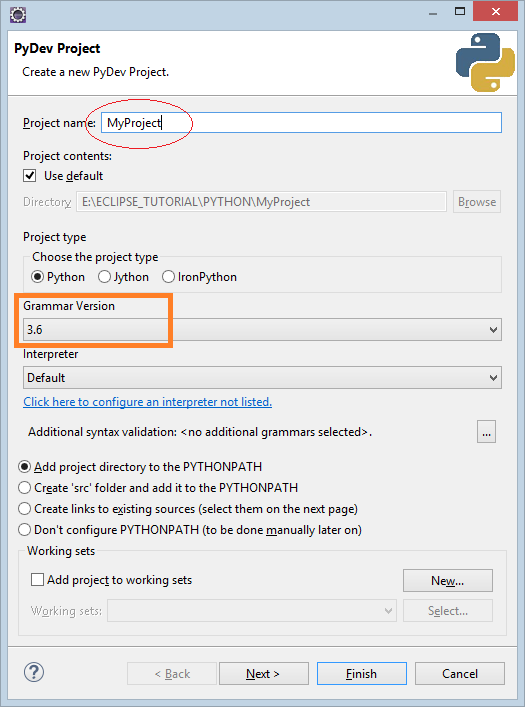
Python Programming Tutorials
- Lookup Python documentation
- Branching statements in Python
- Python Function Tutorial with Examples
- Class and Object in Python
- Inheritance and polymorphism in Python
- Python Dictionary Tutorial with Examples
- Python Lists Tutorial with Examples
- Python Tuples Tutorial with Examples
- Python Date Time Tutorial with Examples
- Connect to MySQL Database in Python using PyMySQL
- Python exception handling Tutorial with Examples
- Python String Tutorial with Examples
- Introduction to Python
- Install Python on Windows
- Install Python on Ubuntu
- Install PyDev for Eclipse
- Conventions and Grammar versions in Python
- Python Tutorial for Beginners
- Python Loops Tutorial with Examples
Show More