Manipulating files and folders on Google Drive using Java
1. Objective of lesson
See Also:
In this lesson, I am going to guide you for manipulating with Google Drive via Google Drive Java API. Topics to be discussed in this lesson include:
- Create a Java application and declare libraries to use the Google Drive API.
- Create a Credentials to be able to interact with the Google Drive.
- Manipulate with the files and folders on the Google Drive via the Google Drive API.
2. Create Credentials
Suppose that you have a Gmail account: abc@gmail.com, then the Google will provide you with 15GB of hard drive space free of charge on Google Drive. You can store your files on it. For your application to be able to manipulate with the files on Google Drive, it needs a credentials. The credentials is simply a file which will be placed on the computer where your application is being deployed like the following illustration:
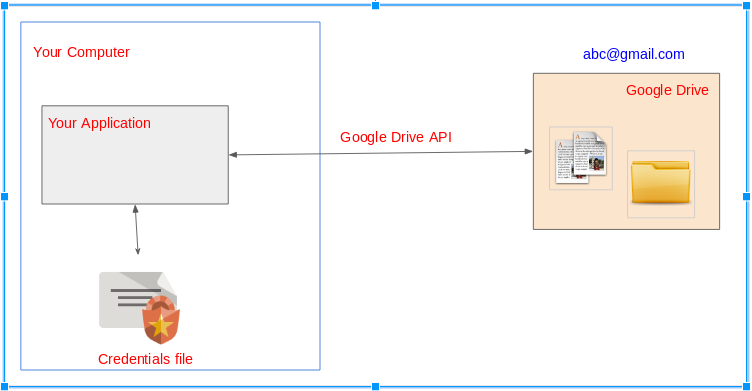
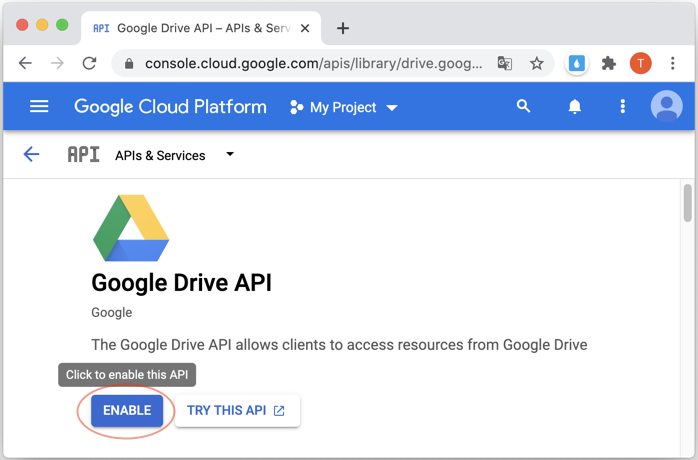
To obtain one foresaid Credentials, you need to create a Project on the Google Developer Console, and download it to the client_secret.json file.
3. Java Google Drive API
For the projects using Maven, you need to declare the following dependencies:
<!-- https://mvnrepository.com/artifact/com.google.apis/google-api-services-drive -->
<dependency>
<groupId>com.google.apis</groupId>
<artifactId>google-api-services-drive</artifactId>
<version>v3-rev105-1.23.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client -->
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client</artifactId>
<version>1.23.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.oauth-client/google-oauth-client-jetty -->
<dependency>
<groupId>com.google.oauth-client</groupId>
<artifactId>google-oauth-client-jetty</artifactId>
<version>1.23.0</version>
</dependency>
4. Get started quickly with an example
Create a Maven project and DriveQuickstart class to get started quickly with the Google Drive API.
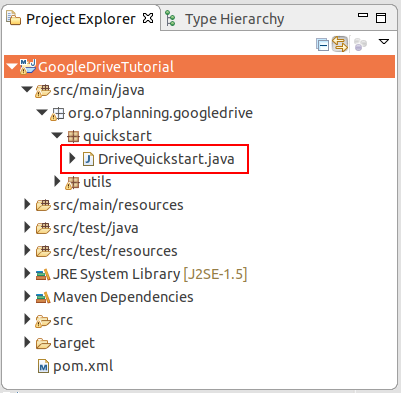
DriveQuickstart.java
package org.o7planning.googledrive.quickstart;
import com.google.api.client.auth.oauth2.Credential;
import com.google.api.client.extensions.java6.auth.oauth2.AuthorizationCodeInstalledApp;
import com.google.api.client.extensions.jetty.auth.oauth2.LocalServerReceiver;
import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow;
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport;
import com.google.api.client.http.javanet.NetHttpTransport;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.json.jackson2.JacksonFactory;
import com.google.api.client.util.store.FileDataStoreFactory;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.DriveScopes;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.security.GeneralSecurityException;
import java.util.Collections;
import java.util.List;
public class DriveQuickstart {
private static final String APPLICATION_NAME = "Google Drive API Java Quickstart";
private static final JsonFactory JSON_FACTORY = JacksonFactory.getDefaultInstance();
// Directory to store user credentials for this application.
private static final java.io.File CREDENTIALS_FOLDER //
= new java.io.File(System.getProperty("user.home"), "credentials");
private static final String CLIENT_SECRET_FILE_NAME = "client_secret.json";
//
// Global instance of the scopes required by this quickstart. If modifying these
// scopes, delete your previously saved credentials/ folder.
//
private static final List<String> SCOPES = Collections.singletonList(DriveScopes.DRIVE);
private static Credential getCredentials(final NetHttpTransport HTTP_TRANSPORT) throws IOException {
java.io.File clientSecretFilePath = new java.io.File(CREDENTIALS_FOLDER, CLIENT_SECRET_FILE_NAME);
if (!clientSecretFilePath.exists()) {
throw new FileNotFoundException("Please copy " + CLIENT_SECRET_FILE_NAME //
+ " to folder: " + CREDENTIALS_FOLDER.getAbsolutePath());
}
// Load client secrets.
InputStream in = new FileInputStream(clientSecretFilePath);
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(in));
// Build flow and trigger user authorization request.
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(HTTP_TRANSPORT, JSON_FACTORY,
clientSecrets, SCOPES).setDataStoreFactory(new FileDataStoreFactory(CREDENTIALS_FOLDER))
.setAccessType("offline").build();
return new AuthorizationCodeInstalledApp(flow, new LocalServerReceiver()).authorize("user");
}
public static void main(String... args) throws IOException, GeneralSecurityException {
System.out.println("CREDENTIALS_FOLDER: " + CREDENTIALS_FOLDER.getAbsolutePath());
// 1: Create CREDENTIALS_FOLDER
if (!CREDENTIALS_FOLDER.exists()) {
CREDENTIALS_FOLDER.mkdirs();
System.out.println("Created Folder: " + CREDENTIALS_FOLDER.getAbsolutePath());
System.out.println("Copy file " + CLIENT_SECRET_FILE_NAME + " into folder above.. and rerun this class!!");
return;
}
// 2: Build a new authorized API client service.
final NetHttpTransport HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport();
// 3: Read client_secret.json file & create Credential object.
Credential credential = getCredentials(HTTP_TRANSPORT);
// 5: Create Google Drive Service.
Drive service = new Drive.Builder(HTTP_TRANSPORT, JSON_FACTORY, credential) //
.setApplicationName(APPLICATION_NAME).build();
// Print the names and IDs for up to 10 files.
FileList result = service.files().list().setPageSize(10).setFields("nextPageToken, files(id, name)").execute();
List<File> files = result.getFiles();
if (files == null || files.isEmpty()) {
System.out.println("No files found.");
} else {
System.out.println("Files:");
for (File file : files) {
System.out.printf("%s (%s)\n", file.getName(), file.getId());
}
}
}
}
For the first time, run the DriveQuickstart class, a {user_home}/credentials folder will be created if it doesn't exist.
Windows | C:\Users\{user}\credentials |
Linux | /home/{user}/credentials |
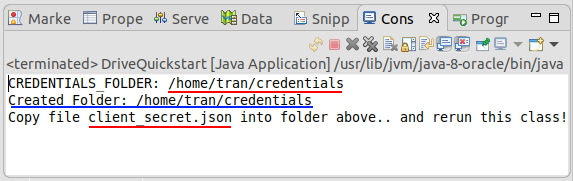
Ensure that you have obtained the client_secret.json file. If not, let's see the following instructions:
Copy the client_secret.json file to the above folder:
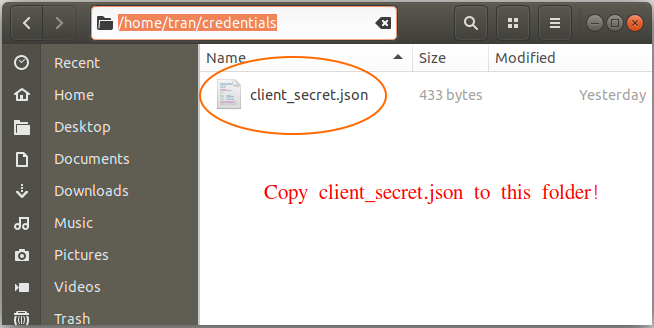
Run the DriveQuickstart class once again. On the Console window, copy the Link and access it on the browser.
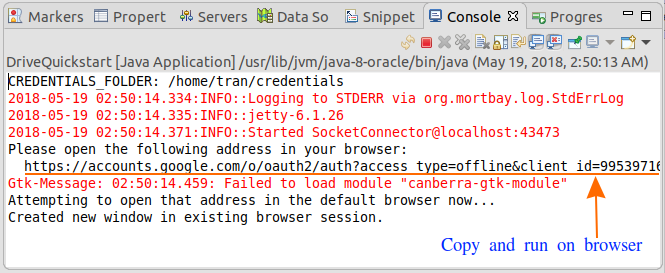
When you access the link on the browser, it will ask you to log in with a Gmail account. At this moment, you need to log in with the Gmail account with which you have created the client_secret.json file.
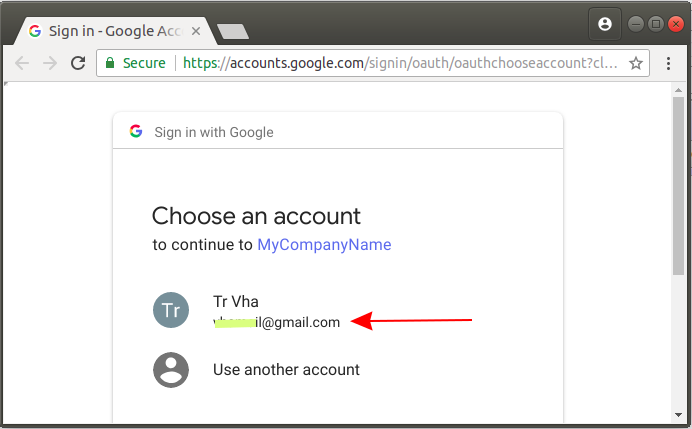
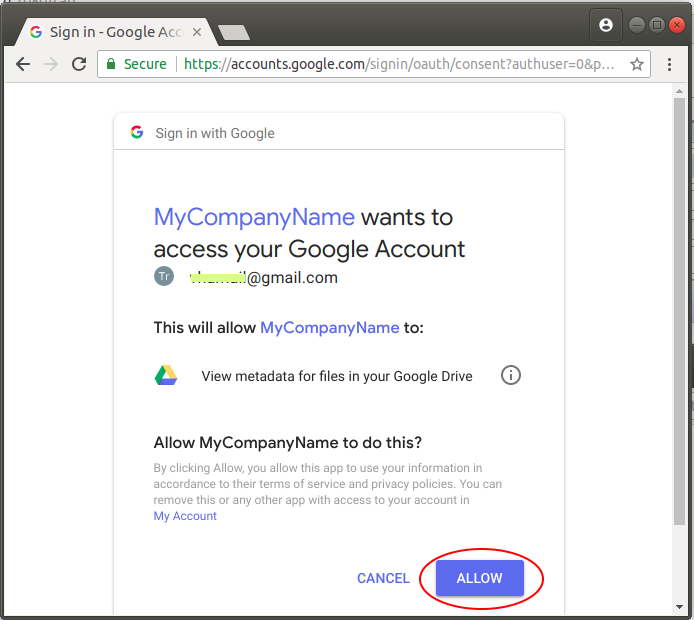
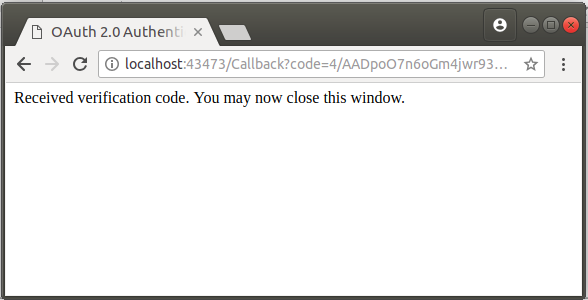
At this moment, a file named StoredCredential will be created on the {user_home}/credential folder.
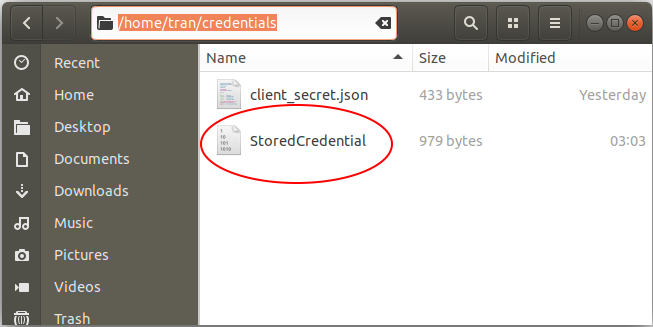
The Credential has been stored on your computer hard drive. From now, when working with Google Drive, you don't have to go through the above steps:
Run the DriveQuickstart class once again and view the results on the Console window. The program will print out a list of files and folders on your Google Drive.
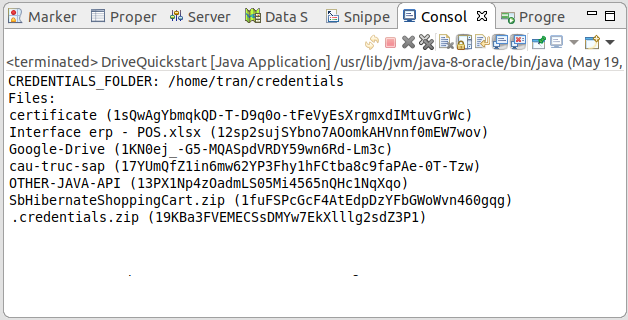
5. File and Directory Structure of Google Drive
Concept of Directory and File in Google Drive is a little different from the Directory & File concept in operating systems. Following are basic characteristics:
- In Google Drive, one File/Directory can have one or more parent Directories.
- In the same directory, the files can have the same name but different ID.
- The com.google.api.services.drive.model.File class represents for both, File and Directory.
The com.google.api.services.drive.model.File class has a lot of fields as in the following illustration:
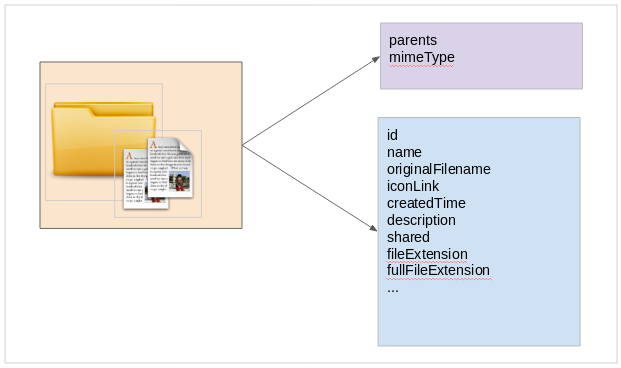
There are 2 important fields such as mineType & parents:
parents
The ID list of parent directories of present file (directory). Root directories or root file have parent directory with ID = "root".
mineType
application/vnd.google-apps.audio | |
application/vnd.google-apps.document | Google Docs |
application/vnd.google-apps.drawing | Google Drawing |
application/vnd.google-apps.file | Google Drive file |
application/vnd.google-apps.folder | Google Drive folder |
application/vnd.google-apps.form | Google Forms |
application/vnd.google-apps.fusiontable | Google Fusion Tables |
application/vnd.google-apps.map | Google My Maps |
application/vnd.google-apps.photo | |
application/vnd.google-apps.presentation | Google Slides |
application/vnd.google-apps.script | Google Apps Scripts |
application/vnd.google-apps.site | Google Sites |
application/vnd.google-apps.spreadsheet | Google Sheets |
application/vnd.google-apps.unknown | |
application/vnd.google-apps.video | |
application/vnd.google-apps.drive-sdk | 3rd party shortcut |
..... |
Operators used for fields:
name | string | contains, =, != | Name of the file. |
fullText | string | contains | Full text of the file including name, description, content, and indexable text. |
mimeType | string | contains, =, != | MIME type of the file. |
modifiedTime | date | <=, <, =, !=, >, >= | Date of the last modification of the file. |
viewedByMeTime | date | <=, <, =, !=, >, >= | Date that the user last viewed a file. |
trashed | boolean | =, != | Whether the file is in the trash or not. |
starred | boolean | =, != | Whether the file is starred or not. |
parents | collection | in | Whether the parents collection contains the specified ID. |
owners | collection | in | Users who own the file. |
writers | collection | in | Users or groups who have permission to modify the file. |
readers | collection | in | Users or groups who have permission to read the file. |
sharedWithMe | boolean | =, != | Files that are in the user's "Shared with me" collection. |
properties | collection | has | Public custom file properties. |
appProperties | collection | has | Private custom file properties. |
visibility | string | =, '!=' | The visibility level of the file. Valid values are anyoneCanFind, anyoneWithLink, domainCanFind, domainWithLink, and limited. |
6. GoogleDriveUtils Class
GoogleDriveUtils.java
package org.o7planning.googledrive.utils;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.Collections;
import java.util.List;
import com.google.api.client.auth.oauth2.Credential;
import com.google.api.client.extensions.java6.auth.oauth2.AuthorizationCodeInstalledApp;
import com.google.api.client.extensions.jetty.auth.oauth2.LocalServerReceiver;
import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow;
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport;
import com.google.api.client.http.HttpTransport;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.json.jackson2.JacksonFactory;
import com.google.api.client.util.store.FileDataStoreFactory;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.DriveScopes;
public class GoogleDriveUtils {
private static final String APPLICATION_NAME = "Google Drive API Java Quickstart";
private static final JsonFactory JSON_FACTORY = JacksonFactory.getDefaultInstance();
// Directory to store user credentials for this application.
private static final java.io.File CREDENTIALS_FOLDER //
= new java.io.File(System.getProperty("user.home"), "credentials");
private static final String CLIENT_SECRET_FILE_NAME = "client_secret.json";
private static final List<String> SCOPES = Collections.singletonList(DriveScopes.DRIVE);
// Global instance of the {@link FileDataStoreFactory}.
private static FileDataStoreFactory DATA_STORE_FACTORY;
// Global instance of the HTTP transport.
private static HttpTransport HTTP_TRANSPORT;
private static Drive _driveService;
static {
try {
HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport();
DATA_STORE_FACTORY = new FileDataStoreFactory(CREDENTIALS_FOLDER);
} catch (Throwable t) {
t.printStackTrace();
System.exit(1);
}
}
public static Credential getCredentials() throws IOException {
java.io.File clientSecretFilePath = new java.io.File(CREDENTIALS_FOLDER, CLIENT_SECRET_FILE_NAME);
if (!clientSecretFilePath.exists()) {
throw new FileNotFoundException("Please copy " + CLIENT_SECRET_FILE_NAME //
+ " to folder: " + CREDENTIALS_FOLDER.getAbsolutePath());
}
InputStream in = new FileInputStream(clientSecretFilePath);
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(in));
// Build flow and trigger user authorization request.
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(HTTP_TRANSPORT, JSON_FACTORY,
clientSecrets, SCOPES).setDataStoreFactory(DATA_STORE_FACTORY).setAccessType("offline").build();
Credential credential = new AuthorizationCodeInstalledApp(flow, new LocalServerReceiver()).authorize("user");
return credential;
}
public static Drive getDriveService() throws IOException {
if (_driveService != null) {
return _driveService;
}
Credential credential = getCredentials();
//
_driveService = new Drive.Builder(HTTP_TRANSPORT, JSON_FACTORY, credential) //
.setApplicationName(APPLICATION_NAME).build();
return _driveService;
}
}
7. SubFolder & RootFolder
If you know ID of a directory, you can take the list of subdirectories of this directory. Please note that in Google Drive, a file (or a directory) can have one or more ParentDirectories.
Now, let's take a look at the example of getting a list of subdirectories of a directory, and retrieving the list of root directories.
GetSubFolders.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
public class GetSubFolders {
// com.google.api.services.drive.model.File
public static final List<File> getGoogleSubFolders(String googleFolderIdParent) throws IOException {
Drive driveService = GoogleDriveUtils.getDriveService();
String pageToken = null;
List<File> list = new ArrayList<File>();
String query = null;
if (googleFolderIdParent == null) {
query = " mimeType = 'application/vnd.google-apps.folder' " //
+ " and 'root' in parents";
} else {
query = " mimeType = 'application/vnd.google-apps.folder' " //
+ " and '" + googleFolderIdParent + "' in parents";
}
do {
FileList result = driveService.files().list().setQ(query).setSpaces("drive") //
// Fields will be assigned values: id, name, createdTime
.setFields("nextPageToken, files(id, name, createdTime)")//
.setPageToken(pageToken).execute();
for (File file : result.getFiles()) {
list.add(file);
}
pageToken = result.getNextPageToken();
} while (pageToken != null);
//
return list;
}
// com.google.api.services.drive.model.File
public static final List<File> getGoogleRootFolders() throws IOException {
return getGoogleSubFolders(null);
}
public static void main(String[] args) throws IOException {
List<File> googleRootFolders = getGoogleRootFolders();
for (File folder : googleRootFolders) {
System.out.println("Folder ID: " + folder.getId() + " --- Name: " + folder.getName());
}
}
}
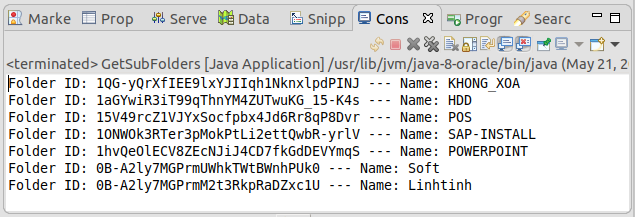
Note: You have a com.google.api.services.drive.model.File object, but not all of its fields are assigned values. Only cases in which you are interested are assigned values. On the contrary, it has null value.FileList result = driveService.files().list().setQ(query).setSpaces("drive") // // Fields will be assigned values: id, name, createdTime .setFields("nextPageToken, files(id, name, createdTime)")// .setPageToken(pageToken).execute();
A next example: search directories by name which are subdirectories of a directory.
GetSubFoldersByName.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
public class GetSubFoldersByName {
// com.google.api.services.drive.model.File
public static final List<File> getGoogleSubFolderByName(String googleFolderIdParent, String subFolderName)
throws IOException {
Drive driveService = GoogleDriveUtils.getDriveService();
String pageToken = null;
List<File> list = new ArrayList<File>();
String query = null;
if (googleFolderIdParent == null) {
query = " name = '" + subFolderName + "' " //
+ " and mimeType = 'application/vnd.google-apps.folder' " //
+ " and 'root' in parents";
} else {
query = " name = '" + subFolderName + "' " //
+ " and mimeType = 'application/vnd.google-apps.folder' " //
+ " and '" + googleFolderIdParent + "' in parents";
}
do {
FileList result = driveService.files().list().setQ(query).setSpaces("drive") //
.setFields("nextPageToken, files(id, name, createdTime)")//
.setPageToken(pageToken).execute();
for (File file : result.getFiles()) {
list.add(file);
}
pageToken = result.getNextPageToken();
} while (pageToken != null);
//
return list;
}
// com.google.api.services.drive.model.File
public static final List<File> getGoogleRootFoldersByName(String subFolderName) throws IOException {
return getGoogleSubFolderByName(null,subFolderName);
}
public static void main(String[] args) throws IOException {
List<File> rootGoogleFolders = getGoogleRootFoldersByName("TEST");
for (File folder : rootGoogleFolders) {
System.out.println("Folder ID: " + folder.getId() + " --- Name: " + folder.getName());
}
}
}
8. Search Files
To search files on Google Drive, you should use the following query condition:
- mimeType != 'application/vnd.google-apps.folder'
FindFilesByName.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
public class FindFilesByName {
// com.google.api.services.drive.model.File
public static final List<File> getGoogleFilesByName(String fileNameLike) throws IOException {
Drive driveService = GoogleDriveUtils.getDriveService();
String pageToken = null;
List<File> list = new ArrayList<File>();
String query = " name contains '" + fileNameLike + "' " //
+ " and mimeType != 'application/vnd.google-apps.folder' ";
do {
FileList result = driveService.files().list().setQ(query).setSpaces("drive") //
// Fields will be assigned values: id, name, createdTime, mimeType
.setFields("nextPageToken, files(id, name, createdTime, mimeType)")//
.setPageToken(pageToken).execute();
for (File file : result.getFiles()) {
list.add(file);
}
pageToken = result.getNextPageToken();
} while (pageToken != null);
//
return list;
}
public static void main(String[] args) throws IOException {
List<File> rootGoogleFolders = getGoogleFilesByName("u");
for (File folder : rootGoogleFolders) {
System.out.println("Mime Type: " + folder.getMimeType() + " --- Name: " + folder.getName());
}
System.out.println("Done!");
}
}
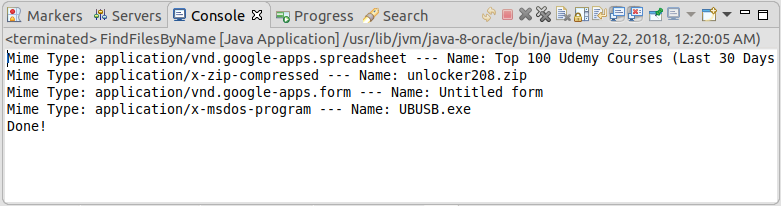
9. Create a directory on Google Drive
CreateFolder.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
public class CreateFolder {
public static final File createGoogleFolder(String folderIdParent, String folderName) throws IOException {
File fileMetadata = new File();
fileMetadata.setName(folderName);
fileMetadata.setMimeType("application/vnd.google-apps.folder");
if (folderIdParent != null) {
List<String> parents = Arrays.asList(folderIdParent);
fileMetadata.setParents(parents);
}
Drive driveService = GoogleDriveUtils.getDriveService();
// Create a Folder.
// Returns File object with id & name fields will be assigned values
File file = driveService.files().create(fileMetadata).setFields("id, name").execute();
return file;
}
public static void main(String[] args) throws IOException {
// Create a Root Folder
File folder = createGoogleFolder(null, "TEST-FOLDER");
System.out.println("Created folder with id= "+ folder.getId());
System.out.println(" name= "+ folder.getName());
System.out.println("Done!");
}
}
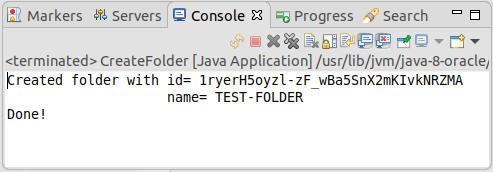
10. Create a file on Google Drive
There are 3 common ways for you to create a file on Google Driver:
- Upload File
- byte[]
- InputStream
CreateGoogleFile.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import java.io.InputStream;
import java.util.Arrays;
import java.util.List;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.client.http.AbstractInputStreamContent;
import com.google.api.client.http.ByteArrayContent;
import com.google.api.client.http.FileContent;
import com.google.api.client.http.InputStreamContent;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.File;
public class CreateGoogleFile {
// PRIVATE!
private static File _createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, AbstractInputStreamContent uploadStreamContent) throws IOException {
File fileMetadata = new File();
fileMetadata.setName(customFileName);
List<String> parents = Arrays.asList(googleFolderIdParent);
fileMetadata.setParents(parents);
//
Drive driveService = GoogleDriveUtils.getDriveService();
File file = driveService.files().create(fileMetadata, uploadStreamContent)
.setFields("id, webContentLink, webViewLink, parents").execute();
return file;
}
// Create Google File from byte[]
public static File createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, byte[] uploadData) throws IOException {
//
AbstractInputStreamContent uploadStreamContent = new ByteArrayContent(contentType, uploadData);
//
return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent);
}
// Create Google File from java.io.File
public static File createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, java.io.File uploadFile) throws IOException {
//
AbstractInputStreamContent uploadStreamContent = new FileContent(contentType, uploadFile);
//
return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent);
}
// Create Google File from InputStream
public static File createGoogleFile(String googleFolderIdParent, String contentType, //
String customFileName, InputStream inputStream) throws IOException {
//
AbstractInputStreamContent uploadStreamContent = new InputStreamContent(contentType, inputStream);
//
return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent);
}
public static void main(String[] args) throws IOException {
java.io.File uploadFile = new java.io.File("/home/tran/Downloads/test.txt");
// Create Google File:
File googleFile = createGoogleFile(null, "text/plain", "newfile.txt", uploadFile);
System.out.println("Created Google file!");
System.out.println("WebContentLink: " + googleFile.getWebContentLink() );
System.out.println("WebViewLink: " + googleFile.getWebViewLink() );
System.out.println("Done!");
}
}
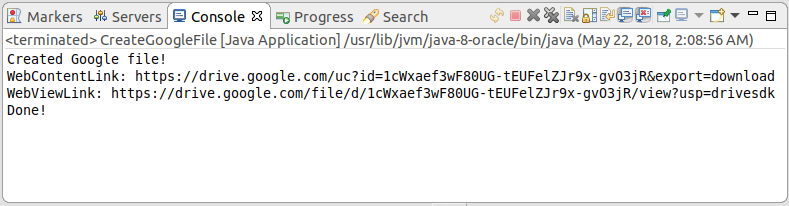
11. Share Google File/Folder
ShareGoogleFile.java
package org.o7planning.googledrive.example;
import java.io.IOException;
import org.o7planning.googledrive.utils.GoogleDriveUtils;
import com.google.api.services.drive.Drive;
import com.google.api.services.drive.model.Permission;
public class ShareGoogleFile {
// Public a Google File/Folder.
public static Permission createPublicPermission(String googleFileId) throws IOException {
// All values: user - group - domain - anyone
String permissionType = "anyone";
// All values: organizer - owner - writer - commenter - reader
String permissionRole = "reader";
Permission newPermission = new Permission();
newPermission.setType(permissionType);
newPermission.setRole(permissionRole);
Drive driveService = GoogleDriveUtils.getDriveService();
return driveService.permissions().create(googleFileId, newPermission).execute();
}
public static Permission createPermissionForEmail(String googleFileId, String googleEmail) throws IOException {
// All values: user - group - domain - anyone
String permissionType = "user"; // Valid: user, group
// organizer - owner - writer - commenter - reader
String permissionRole = "reader";
Permission newPermission = new Permission();
newPermission.setType(permissionType);
newPermission.setRole(permissionRole);
newPermission.setEmailAddress(googleEmail);
Drive driveService = GoogleDriveUtils.getDriveService();
return driveService.permissions().create(googleFileId, newPermission).execute();
}
public static void main(String[] args) throws IOException {
String googleFileId1 = "some-google-file-id-1";
String googleEmail = "test.o7planning@gmail.com";
// Share for a User
createPermissionForEmail(googleFileId1, googleEmail);
String googleFileId2 = "some-google-file-id-2";
// Share for everyone
createPublicPermission(googleFileId2);
System.out.println("Done!");
}
}
- Creating a Google API Console project and OAuth2 Client ID
- Register Google Map API Key
- Use Google reCAPTCHA in Java Web Application
- Get Started with the Google Search Console API
- Index pages with the Java Google Indexing API
- Manipulating files and folders on Google Drive using Java
- Create a Google Service Account
- List, add and delete Sites with Google Search Java API
- List, submit and delete Sitemaps with Google Search Java API
Show More
Java Open Source Libraries
- Java JSON Processing API Tutorial (JSONP)
- Using Scribe OAuth Java API with Google OAuth2
- Get Hardware information in Java application
- Restfb Java API for Facebook
- Create Credentials for Google Drive API
- Java JDOM2 Tutorial with Examples
- Java XStream Tutorial with Examples
- Jsoup Java Html Parser Tutorial with Examples
- Retrieve Geographic information based on IP Address using GeoIP2 Java API
- Read and Write Excel file in Java using Apache POI
- Explore the Facebook Graph API
- Java Sejda WebP ImageIO convert Images to WEBP
- Java JAVE Convert audio and video to mp3
- Manipulating files and folders on Google Drive using Java
Show More