Get Hardware information in Java application
1. Java API to retrieve computer hardware information?
Sometimes you need to use Java to get information of computer hardware, including Serial Number of the mainboard, serial of hard drive, CPU, ...
Unfortunately, Java has no such API, or may be there, but it was not released for free for programmers. However, on Windows you can obtain this information by executing the VB script.
Unfortunately, Java has no such API, or may be there, but it was not released for free for programmers. However, on Windows you can obtain this information by executing the VB script.
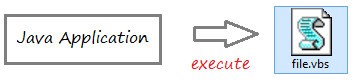
2. Get hardware info using vbscript file
OK, in order to be more simple, you can create a file named myscript.vbs:
myscript.vbs
Set objWMIService = GetObject("winmgmts:\\.\root\cimv2")
Set colItems = objWMIService.ExecQuery _
("Select Name,UUID,Vendor,Version from Win32_ComputerSystemProduct")
For Each objItem in colItems
Wscript.Echo objItem.Name
Wscript.Echo objItem.UUID
Wscript.Echo objItem.Vendor
Wscript.Echo objItem.Version
Next
On windows click file to run it:
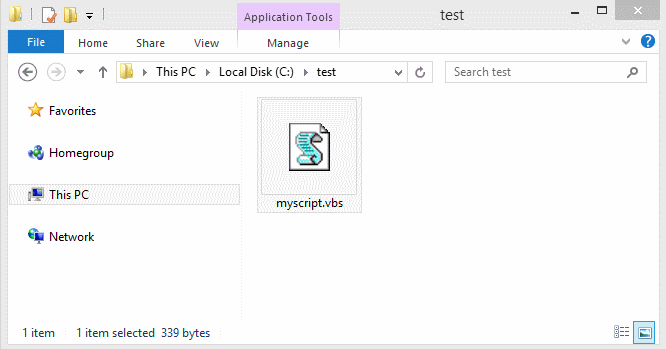
In which Win32_ComputerSystemProduct is a class of Visual Basic, the properties of this class:
Properties |
string Caption |
string Description |
string IdentifyingNumber |
string Name |
string SKUNumber |
string UUID |
string Vendor |
string Version |
You can run a full script file:
Win32_ComputerSystemProduct.vbs
Set objWMIService = GetObject("winmgmts:\\.\root\cimv2")
Set colItems = objWMIService.ExecQuery _
("Select * from Win32_ComputerSystemProduct")
For Each objItem in colItems
Wscript.Echo objItem.Caption
Wscript.Echo objItem.Description
Wscript.Echo objItem.IdentifyingNumber
Wscript.Echo objItem.Name
Wscript.Echo objItem.SKUNumber
Wscript.Echo objItem.UUID
Wscript.Echo objItem.Vendor
Wscript.Echo objItem.Version
Next
Results got (Correspond to my computer):
Property | Value (My Computer) |
Caption | Computer System Product |
Description | Computer System Product |
IdentifyingNumber | 3F027935U |
Name | Salellite S75B |
SKUNumber | null |
UUID | B09366C5-F0C7-E411-98E4-008CFA8C26DF |
Vendor | TOSHIBA |
Version | PSPPJU-07U051 |
See more Win32_ComputerSystemProduct:
Some other Visual Basic classes, you may be interested in:
3. Use Java to get computer hardware information
OK, above you know how to use vb script to retrieve the computer hardware information. Now you need to use the Java to execute vb script files and get the value returned.
MyUtility.java
package org.o7planning.hardwareinfo;
public class MyUtility {
public static String makeVbScript(String vbClassName, String[] propNames) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < propNames.length; i++) {
if (i < propNames.length - 1) {
sb.append(propNames[i]).append(",");
} else {
sb.append(propNames[i]);
}
}
String colNameString = sb.toString();
sb.setLength(0);
sb.append("Set objWMIService = GetObject(\"winmgmts:\\\\.\\root\\cimv2\")").append("\n");
sb.append("Set colItems = objWMIService.ExecQuery _ ").append("\n");
sb.append("(\"Select ").append(colNameString).append(" from ").append(vbClassName).append("\") ").append("\n");
sb.append("For Each objItem in colItems ").append("\n");
for (String propName : propNames) {
sb.append(" Wscript.Echo objItem.").append(propName).append("\n");
}
sb.append("Next ").append("\n");
return sb.toString();
}
}
GetHardwareInfo.java
package org.o7planning.hardwareinfo;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileWriter;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
public class GetHardwareInfo {
public static void printComputerSystemProductInfo() {
String vbClassName = "Win32_ComputerSystemProduct";
String[] propNames = new String[] { "Name", "UUID", "Vendor", "Version" };
String vbScript = MyUtility.makeVbScript(vbClassName, propNames);
System.out.println("----------------------------------------");
System.out.println(vbScript);
System.out.println("----------------------------------------");
try {
// Create temporary file.
File file = File.createTempFile("vbsfile", ".vbs");
System.out.println("Create File: " + file.getAbsolutePath());
System.out.println("------");
// Write script content to file.
FileWriter fw = new FileWriter(file);
fw.write(vbScript);
fw.close();
// Execute the file.
Process p = Runtime.getRuntime().exec("cscript //NoLogo " + file.getPath());
// Create Input stream to read data returned after execute vb script file.
BufferedReader input = new BufferedReader(new InputStreamReader(p.getInputStream()));
Map<String, String> map = new HashMap<String, String>();
String line;
int i = 0;
while ((line = input.readLine()) != null) {
if (i >= propNames.length) {
break;
}
String key = propNames[i];
map.put(key, line);
i++;
}
input.close();
//
for (String propName : propNames) {
System.out.println(propName + " : " + map.get(propName));
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
printComputerSystemProductInfo();
}
}
Run the example:
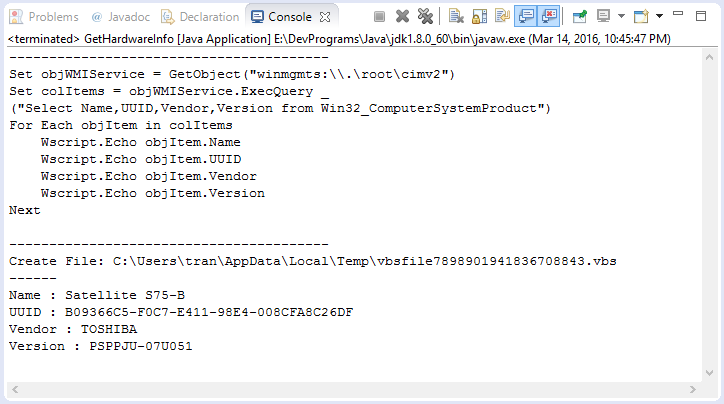
Java Open Source Libraries
- Java JSON Processing API Tutorial (JSONP)
- Using Scribe OAuth Java API with Google OAuth2
- Get Hardware information in Java application
- Restfb Java API for Facebook
- Create Credentials for Google Drive API
- Java JDOM2 Tutorial with Examples
- Java XStream Tutorial with Examples
- Jsoup Java Html Parser Tutorial with Examples
- Retrieve Geographic information based on IP Address using GeoIP2 Java API
- Read and Write Excel file in Java using Apache POI
- Explore the Facebook Graph API
- Java Sejda WebP ImageIO convert Images to WEBP
- Java JAVE Convert audio and video to mp3
- Manipulating files and folders on Google Drive using Java
Show More