Get Started with the Google Search Console API
The Search Console API provides programmatic access to much of the functionality of Google Search Console. Use the API to view, add, or remove properties and sitemaps, run advanced queries for Google Search results data for the properties that you manage in Search Console, and test individual pages.
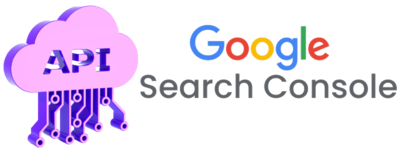
This API is exposed as HTTP Rest Service, you can call its functions through REST libraries.
In this article I will show you how to set up a Java project using the Google Search Console API.
1. Requirements
First of all, you need to register and create a project on Google Cloud Console.
Next, enable the "Google Search Console" service for your account.
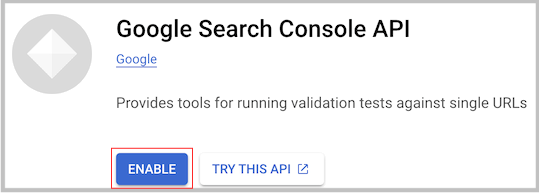
Create a "Google Service Account" and download Credentials as a JSON file.
After the above step, you are provided with an email in the format "xxx@yyy.iam.gserviceaccount.com".
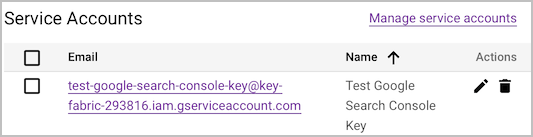
On Google Search Console, add the Google Service Account email you got in the step above as an owner for your website.
Choose a property (website), for example:
- https://mydomain.com/
Settings > Users and permissions:
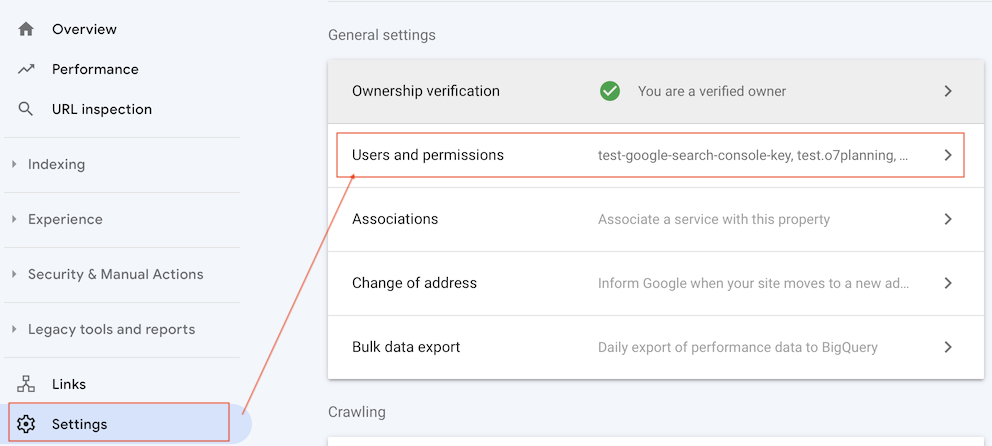
Add the Google Service Account email you got in the step above as an owner for your property.
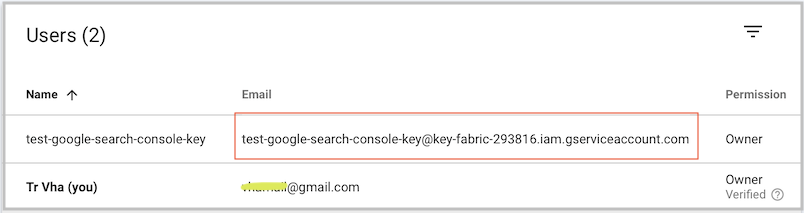
2. Libraries
Add Java libraries to your project:
<!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client -->
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client</artifactId>
<version>2.2.0</version>
</dependency>
<!-- Github -->
<!-- https://github.com/googleapis/google-api-java-client-services/tree/main/clients/google-api-services-searchconsole/v1 -->
<!-- SEE ALL VERSION -->
<!-- https://libraries.io/maven/com.google.apis:google-api-services-searchconsole/versions -->
<dependency>
<groupId>com.google.apis</groupId>
<artifactId>google-api-services-searchconsole</artifactId>
<version>v1-rev20230920-2.0.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.auth/google-auth-library-oauth2-http -->
<dependency>
<groupId>com.google.auth</groupId>
<artifactId>google-auth-library-oauth2-http</artifactId>
<version>1.20.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client-gson -->
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client-gson</artifactId>
<version>2.2.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.15.1</version>
</dependency>
3. Utility classes
After downloading the key of "Google Service Account" in the above step, we have a JSON file. Next, we write a utility class to create an HttpRequestInitializer object, which is needed to initialize requests before they are sent to Google.
MyUtils.java
package org.o7planning.googleapis.utils;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import org.apache.commons.io.FileUtils;
import com.google.api.client.http.HttpRequest;
import com.google.api.client.http.HttpRequestInitializer;
import com.google.api.client.json.JsonObjectParser;
import com.google.api.client.json.gson.GsonFactory;
import com.google.api.client.util.ObjectParser;
import com.google.auth.http.HttpCredentialsAdapter;
import com.google.auth.oauth2.GoogleCredentials;
import com.google.auth.oauth2.ServiceAccountCredentials;
public class MyUtils {
public static final String SERVICE_ACCOUNT_FILE_PATH = "/Volumes/New/_gsc/test-google-search-console-key.json";
private static byte[] serviceAccountBytes;
public static HttpRequestInitializer createHttpRequestInitializer(String... scopes) throws IOException {
InputStream serviceAccountInputStream = getServiceAccountInputStream();
GoogleCredentials credentials = ServiceAccountCredentials //
.fromStream(serviceAccountInputStream) //
.createScoped(scopes);
HttpRequestInitializer requestInitializer = new HttpRequestInitializer() {
@Override
public void initialize(HttpRequest request) throws IOException {
HttpCredentialsAdapter adapter = new HttpCredentialsAdapter(credentials);
adapter.initialize(request);
//
if (request.getParser() == null) {
ObjectParser parser = new JsonObjectParser(GsonFactory.getDefaultInstance());
request.setParser(parser);
}
//
request.setConnectTimeout(60000); // 1 minute connect timeout
request.setReadTimeout(60000); // 1 minute read timeout
}
};
return requestInitializer;
}
public static synchronized InputStream getServiceAccountInputStream() throws IOException {
if (serviceAccountBytes == null) {
serviceAccountBytes = FileUtils.readFileToByteArray(new File(SERVICE_ACCOUNT_FILE_PATH));
}
return new ByteArrayInputStream(serviceAccountBytes);
}
}
- Creating a Google API Console project and OAuth2 Client ID
- Register Google Map API Key
- Use Google reCAPTCHA in Java Web Application
- Get Started with the Google Search Console API
- Index pages with the Java Google Indexing API
- Manipulating files and folders on Google Drive using Java
- Create a Google Service Account
- List, add and delete Sites with Google Search Java API
- List, submit and delete Sitemaps with Google Search Java API
Show More