Restfb Java API for Facebook
1. What is Restfb?
Firstly Facebook considers the relationship between the entity as a "social graph" . And they built an API, called "Facebook Graph API", this API allows to retrieve information, modify the information of the entity, such as uploading photos, writing comments, get a list of friends, ... this API is based on HTTP so it allows any languages has HTTP library to work with it, including Java.
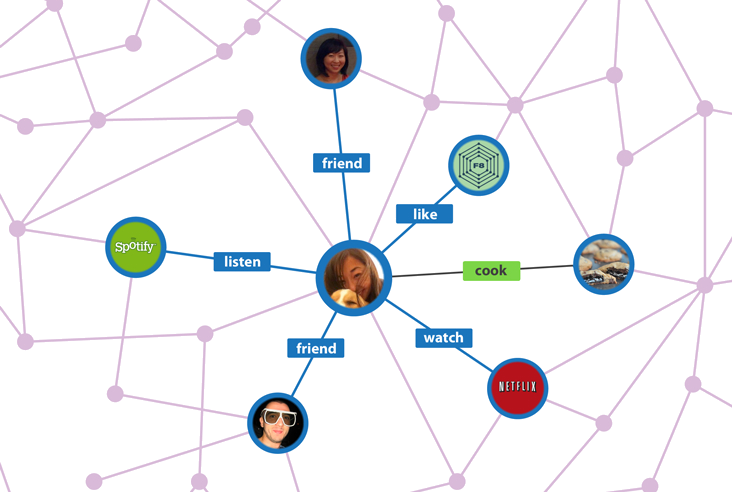
Restfb is a Java API that allows you to interact with the Facebook Graph API.
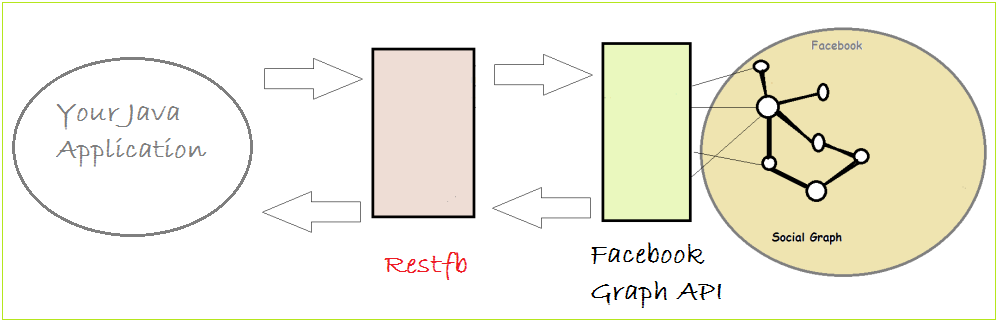
You need to know about "the Facebook Graph API" before starting to read this document. You can refer to the instruction on "Facebook Graph API" at:
2. Restfb download
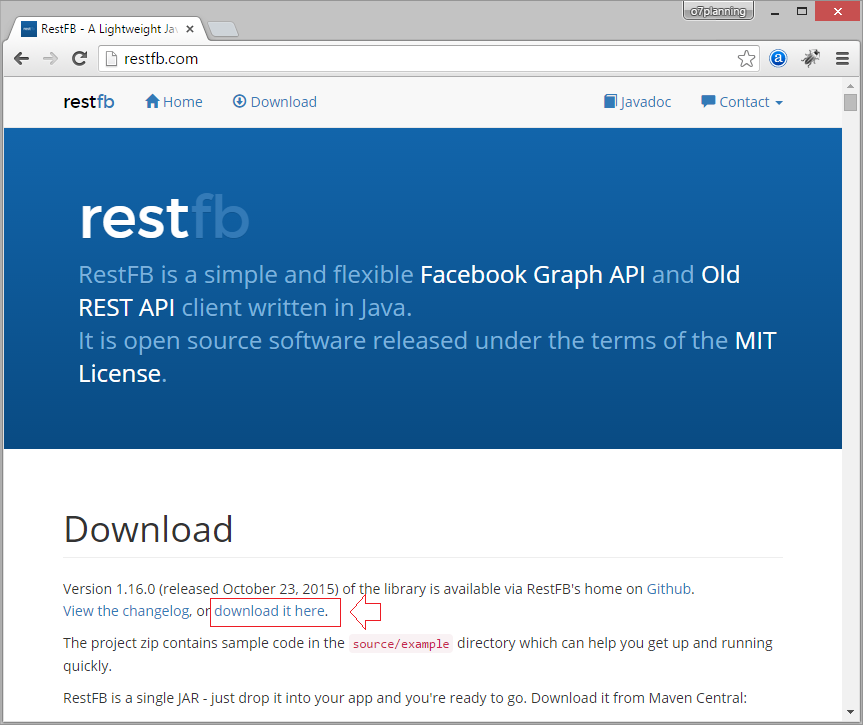
If you use Maven:
<!-- http://mvnrepository.com/artifact/com.restfb/restfb -->
<dependency>
<groupId>com.restfb</groupId>
<artifactId>restfb</artifactId>
<version>1.16.0</version>
</dependency>
3. Create Project
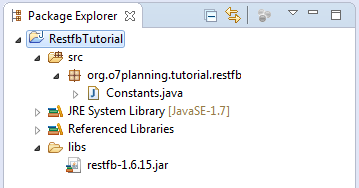
Copy ACCESS_TOKEN that you see on "Facebook Graph Explorer" into the MY_ACCESS_TOKEN field of Constants class below.
Note: ACCESS_TOKEN on "Facebook Graph Explorer" is not last long, it will expire in a certain time period, then you need to copy again. In the app, you can get value Access_Token dynamically at runtime. This issue will also be instructed in this document.
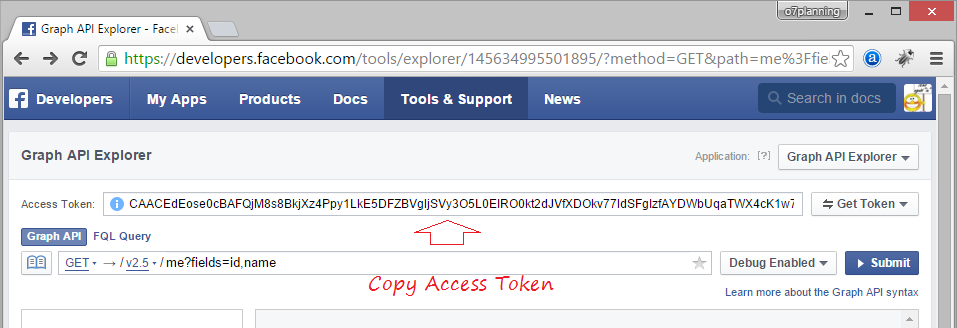
Constants.java
package org.o7planning.tutorial.restfb;
public class Constants {
public static final String REDIRECT_URI
= "http://localhost:8080/facebookfriends/FriendsListServlet";
public static final String MY_ACCESS_TOKEN = "<Your Access Token>";
// Facebook App
public static final String MY_APP_ID = "<your app id>";
public static final String MY_APP_SECRET = "<your app secret>";
}
If you are using Access Token with multiple data access rights. On the Facebook Graph Explorer, clicking on "Get Token/Get User Access Token". And choose the rights, then clicking "Get Access Token" to get a new Access Token with more powers.
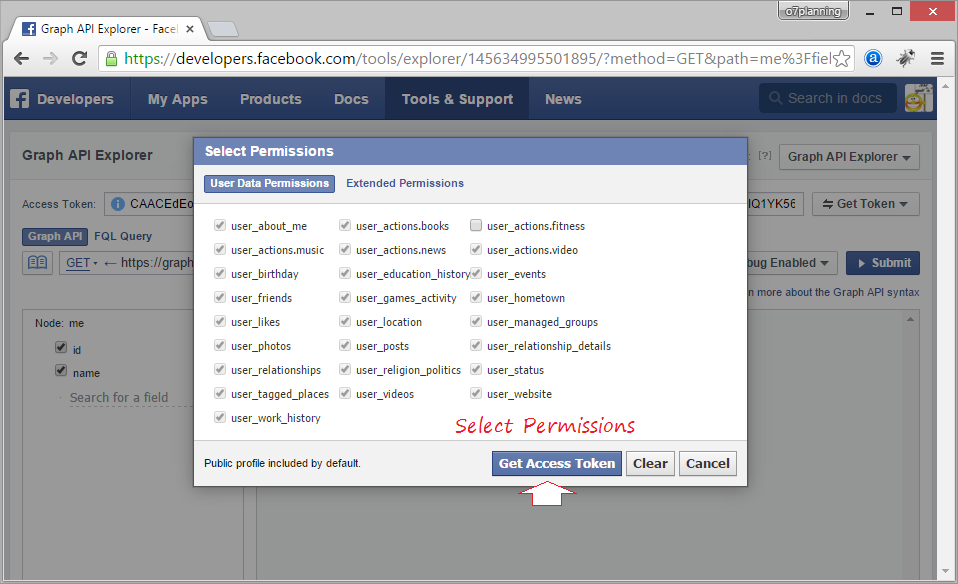
4. Examples
You need to have FacebookClient object to accessing Facebook data . Here are instructions for creating this object.
// DefaultFacebookClient is the FacebookClient implementation
// that ships with RestFB. You can customize it by passing in
// custom JsonMapper and WebRequestor implementations, or simply
// write your own FacebookClient instead for maximum control.
FacebookClient facebookClient = new DefaultFacebookClient(MY_ACCESS_TOKEN);
// It's also possible to create a client that can only access
// publicly-visible data - no access token required.
// Note that many of the examples below will not work unless you supply an access token!
FacebookClient publicOnlyFacebookClient = new DefaultFacebookClient();
// Get added security by using your app secret:
FacebookClient facebookClient = new DefaultFacebookClient(MY_APP_ACCESS_TOKEN, MY_APP_SECRET);
Take your information example
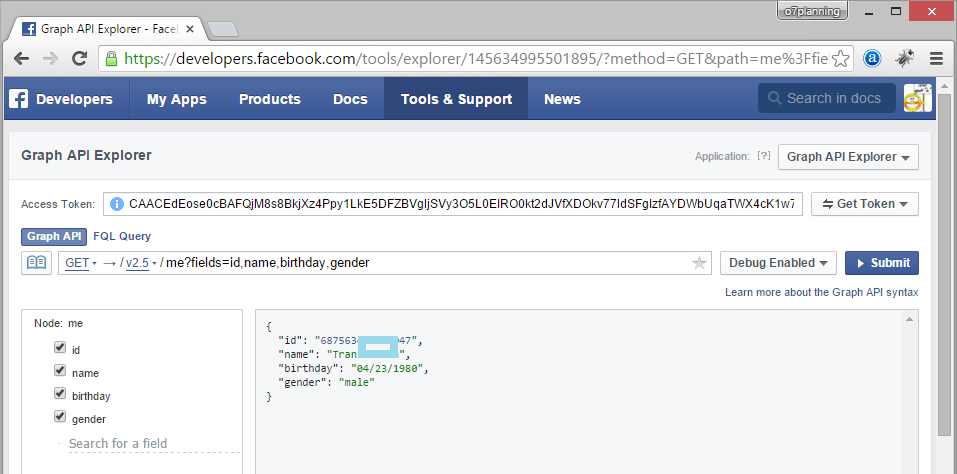
SimpleMeExample.java
package org.o7planning.tutorial.restfb.me;
import org.o7planning.tutorial.restfb.Constants;
import com.restfb.DefaultFacebookClient;
import com.restfb.FacebookClient;
import com.restfb.types.User;
public class SimpleMeExample {
public static void main(String[] args) {
// Tạo đối tượng FacebookClient
FacebookClient facebookClient= new DefaultFacebookClient(Constants.MY_ACCESS_TOKEN);
// User là một class có sẵn của Restfb mô tả các thông tin của User
// Trong tình huống này chúng ta biết trước dữ liệu trả về là User.
User user = facebookClient.fetchObject("me", User.class);
System.out.println("User="+ user);
System.out.println("UserName= "+ user.getUsername());
System.out.println("Birthday= "+ user.getBirthday());
}
}
Running example:
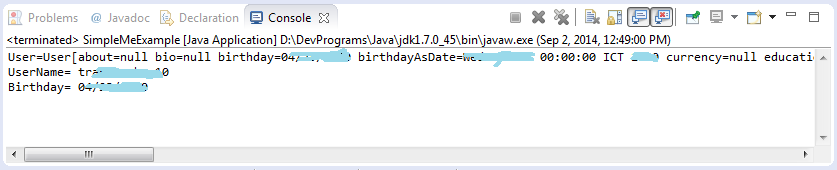
Customize your information
CustomUser.java
package org.o7planning.tutorial.restfb.me;
import com.restfb.Facebook;
public class CustomUser {
@Facebook("first_name")
private String firstName;
@Facebook("last_name")
private String lastName;
@Facebook("name")
private String fullName;
@Facebook
private String email;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
CustomDataMeExample.java
package org.o7planning.tutorial.restfb.me;
import org.o7planning.tutorial.restfb.Constants;
import com.restfb.DefaultFacebookClient;
import com.restfb.FacebookClient;
import com.restfb.Parameter;
public class CustomDataMeExample {
public static void main(String[] args) {
FacebookClient facebookClient = new DefaultFacebookClient(
Constants.MY_ACCESS_TOKEN);
CustomUser user = facebookClient.fetchObject("me", CustomUser.class,
Parameter.with("fields",
"id, name, email, first_name, last_name"));
System.out.println("First Name= " + user.getFirstName());
System.out.println("Last Name= " + user.getLastName());
System.out.println("Full Name= " + user.getFullName());
System.out.println("Email= " + user.getEmail());
}
}
Running example

JsonObject Class
Restfb build some class description for the data, such User, Album, .. in fact you need more than that. In the general case, it is a JSONObject. For example, a simple JSON data below:
{
"id": "687563464611117",
"email": "abc@yahoo.com",
"birthday": "04/11/2000",
"albums": {
"data": [
{
"name": "Test Album",
"type": "normal",
"id": "726713597396700",
"created_time": "2014-09-01T08:11:52+0000"
},
{
"name": "Untitled Album",
"type": "normal",
"id": "726695580731835",
"created_time": "2014-09-01T06:55:23+0000"
}
]
}
}
JsonUserDataExample.java
package org.o7planning.tutorial.restfb.me;
import org.o7planning.tutorial.restfb.Constants;
import com.restfb.DefaultFacebookClient;
import com.restfb.FacebookClient;
import com.restfb.Parameter;
import com.restfb.json.JsonObject;
public class JsonUserDataExample {
public static void main(String[] args) {
FacebookClient facebookClient = new DefaultFacebookClient(
Constants.MY_ACCESS_TOKEN);
//
// Trong tình huống tổng quát, sử dụng JsonObject.
//
JsonObject userData = facebookClient.fetchObject("me",
JsonObject.class, Parameter.with("fields", "name, first_name"));
System.out.println("userData=" + userData);
System.out.println("FirstName=" + userData.getString("first_name"));
System.out.println("Name= " + userData.getString("name"));
}
}
Running example:

Connection
Connection is the link between the "things" in facebook, such as the relationship between a photo and its Comment, the relationship between the user and their friend. Sometimes, it also called Edge.
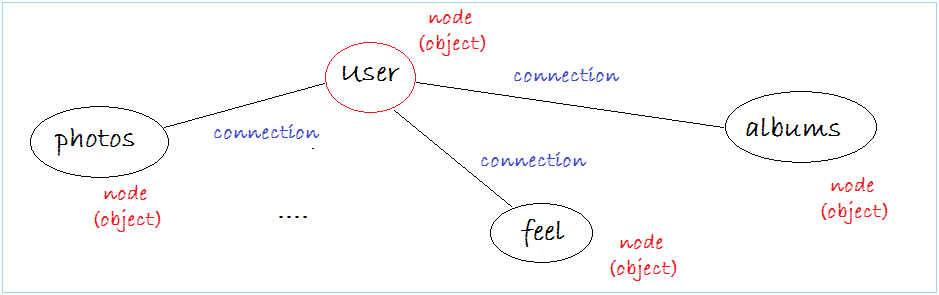
See in "Facebook Graph Explorer":
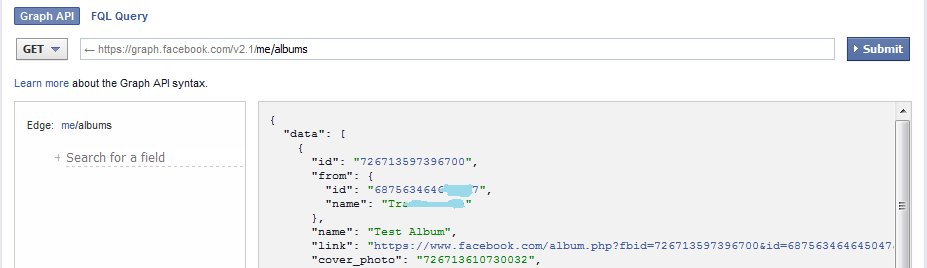
Albums example
AlbumsExample.java
package org.o7planning.tutorial.restfb.connection;
import java.util.List;
import org.o7planning.tutorial.restfb.Constants;
import com.restfb.Connection;
import com.restfb.DefaultFacebookClient;
import com.restfb.FacebookClient;
import com.restfb.types.Album;
public class GetAlbumsExample {
public static void main(String[] args) {
FacebookClient facebookClient = new DefaultFacebookClient(
Constants.MY_ACCESS_TOKEN);
Connection<Album> albumConnection = facebookClient.fetchConnection(
"me/albums", Album.class);
List<Album> albums = albumConnection.getData();
for (Album album : albums) {
System.out.println("Album name:" + album.getName());
}
}
}
Running the example:

Album and params example
CustomGetAlbumsExample.java
package org.o7planning.tutorial.restfb.connection;
import java.util.Date;
import java.util.List;
import org.o7planning.tutorial.restfb.Constants;
import com.restfb.Connection;
import com.restfb.DefaultFacebookClient;
import com.restfb.FacebookClient;
import com.restfb.Parameter;
import com.restfb.types.Album;
public class CustomGetAlbumsExample {
public static void main(String[] args) {
FacebookClient facebookClient = new DefaultFacebookClient(
Constants.MY_ACCESS_TOKEN);
// 1 tuần trước.
Date oneWeekAgo = new Date(System.currentTimeMillis() - 1000L * 60L
* 60L * 24L * 7L);
// Lấy ra kết nối tới các Album tạo trong khoảng 1 tuần trước.
// Và tối đa 3 Album.
Connection<Album> albumConnection = facebookClient.fetchConnection(
"me/albums", Album.class, Parameter.with("limit", 3),
Parameter.with("since", oneWeekAgo));
List<Album> albums = albumConnection.getData();
for (Album album : albums) {
System.out.println("Album name:" + album.getName());
}
}
}
Running the example

Java Open Source Libraries
- Java JSON Processing API Tutorial (JSONP)
- Using Scribe OAuth Java API with Google OAuth2
- Get Hardware information in Java application
- Restfb Java API for Facebook
- Create Credentials for Google Drive API
- Java JDOM2 Tutorial with Examples
- Java XStream Tutorial with Examples
- Jsoup Java Html Parser Tutorial with Examples
- Retrieve Geographic information based on IP Address using GeoIP2 Java API
- Read and Write Excel file in Java using Apache POI
- Explore the Facebook Graph API
- Java Sejda WebP ImageIO convert Images to WEBP
- Java JAVE Convert audio and video to mp3
- Manipulating files and folders on Google Drive using Java
Show More