Uploading and downloading files stored to hard drive with Java Servlet
1. Update files and stored on the hard drive
In this lesson, I will guide you to upload file and store files on your hard drive. This example use Servlet API >= 3.0.
Note: You can see a similar guide uploading and downloading file from Database using Servlet at:
2. Upload file example
Uploading and storing files on the hard drive:
UploadFileServlet.java
package org.o7planning.servletexamples;
import java.io.File;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
@WebServlet("/uploadFile")
@MultipartConfig(fileSizeThreshold = 1024 * 1024 * 2, // 2MB
maxFileSize = 1024 * 1024 * 10, // 10MB
maxRequestSize = 1024 * 1024 * 50) // 50MB
public class UploadFileServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public static final String SAVE_DIRECTORY = "uploadDir";
public UploadFileServlet() {
super();
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
RequestDispatcher dispatcher = request.getServletContext().getRequestDispatcher("/WEB-INF/jsps/uploadFile.jsp");
dispatcher.forward(request, response);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
try {
String description = request.getParameter("description");
System.out.println("Description: " + description);
// Gets absolute path to root directory of web app.
String appPath = request.getServletContext().getRealPath("");
appPath = appPath.replace('\\', '/');
// The directory to save uploaded file
String fullSavePath = null;
if (appPath.endsWith("/")) {
fullSavePath = appPath + SAVE_DIRECTORY;
} else {
fullSavePath = appPath + "/" + SAVE_DIRECTORY;
}
// Creates the save directory if it does not exists
File fileSaveDir = new File(fullSavePath);
if (!fileSaveDir.exists()) {
fileSaveDir.mkdir();
}
// Part list (multi files).
for (Part part : request.getParts()) {
String fileName = extractFileName(part);
if (fileName != null && fileName.length() > 0) {
String filePath = fullSavePath + File.separator + fileName;
System.out.println("Write attachment to file: " + filePath);
// Write to file
part.write(filePath);
}
}
// Upload successfully!.
response.sendRedirect(request.getContextPath() + "/uploadFileResults");
} catch (Exception e) {
e.printStackTrace();
request.setAttribute("errorMessage", "Error: " + e.getMessage());
RequestDispatcher dispatcher = getServletContext().getRequestDispatcher("/WEB-INF/jsps/uploadFile.jsp");
dispatcher.forward(request, response);
}
}
private String extractFileName(Part part) {
// form-data; name="file"; filename="C:\file1.zip"
// form-data; name="file"; filename="C:\Note\file2.zip"
String contentDisp = part.getHeader("content-disposition");
String[] items = contentDisp.split(";");
for (String s : items) {
if (s.trim().startsWith("filename")) {
// C:\file1.zip
// C:\Note\file2.zip
String clientFileName = s.substring(s.indexOf("=") + 2, s.length() - 1);
clientFileName = clientFileName.replace("\\", "/");
int i = clientFileName.lastIndexOf('/');
// file1.zip
// file2.zip
return clientFileName.substring(i + 1);
}
}
return null;
}
}
UploadFileResultsServlet.java
package org.o7planning.servletexamples;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/uploadFileResults")
public class UploadFileResultsServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public UploadFileResultsServlet() {
super();
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
RequestDispatcher dispatcher
= request.getServletContext().getRequestDispatcher("/WEB-INF/jsps/uploadFileResults.jsp");
dispatcher.forward(request, response);
}
}
/WEB-INF/jsps/uploadFile.jsp
<!DOCTYPE >
<html>
<head>
<title>Upload files</title>
</head>
<body>
<div style="padding:5px; color:red;font-style:italic;">
${errorMessage}
</div>
<h2>Upload Files</h2>
<form method="post" action="${pageContext.request.contextPath}/uploadFile"
enctype="multipart/form-data">
Select file to upload:
<br />
<input type="file" name="file" />
<br />
<input type="file" name="file" />
<br />
Description:
<br />
<input type="text" name="description" size="100" />
<br />
<br />
<input type="submit" value="Upload" />
</form>
</body>
</html>
/WEB-INF/jsps/uploadFileResults.jsp
<!DOCTYPE >
<html>
<head>
<title>Upload files</title>
</head>
<body>
<h3>Upload has been done successfully!</h3>
<a href="${pageContext.request.contextPath}/uploadFile">Continue Upload</a>
</body>
</html>
Running the apps:
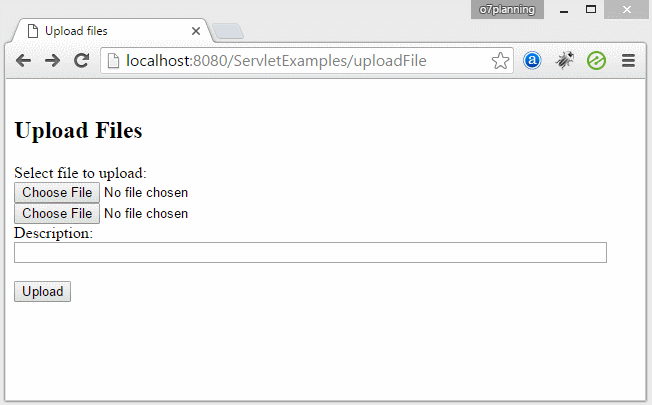
Java Servlet/Jsp Tutorials
- Install Tomcat Server for Eclipse
- Install Glassfish Web Server on Windows
- Run Maven Java Web Application in Tomcat Maven Plugin
- Run Maven Java Web Application in Jetty Maven Plugin
- Run background task in Java Servlet Application
- Java Servlet Tutorial for Beginners
- Java Servlet Filter Tutorial with Examples
- Java JSP Tutorial for Beginners
- Java JSP Standard Tag Library (JSTL) Tutorial with Examples
- Install Web Tools Platform for Eclipse
- Create a simple Login application and secure pages with Java Servlet Filter
- Create a Simple Java Web Application Using Servlet, JSP and JDBC
- Uploading and downloading files stored to hard drive with Java Servlet
- Upload and download files from Database using Java Servlet
- Displaying Image from Database with Java Servlet
- Redirect 301 Permanent redirect in Java Servlet
- How to automatically redirect http to https in a Java Web application?
- Use Google reCAPTCHA in Java Web Application
Show More