- What is Servlet?
- Servlet Lifecycle
- Install Tomcat Web Server
- Create Web Project to start with Servlet
- Configure Eclipse to run apps on tomcat
- Some classes participated in examples
- Create your first Servlet
- Init Parameter of Servlet
- Configuring Servlet with Annotations
- Servlet Url Pattern
- Get the basic information of the Servlet
- Forward
- Redirect
- Session
- Servlet-Filter Tutorial
- JSP Tutorial
Java Servlet Tutorial for Beginners
1. What is Servlet?
Java Servlets are programs that run on a Web or Application server and act as a middle layer between a request coming from a Web browser or other HTTP client and databases or applications on the HTTP server.
Using Servlets, you can collect input from users through web page forms, present records from a database or another source, and create web pages dynamically.
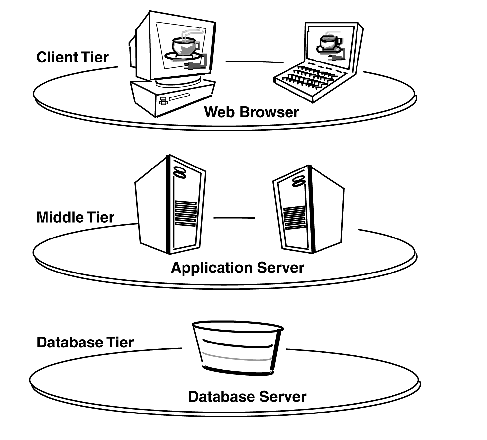
2. Servlet Lifecycle
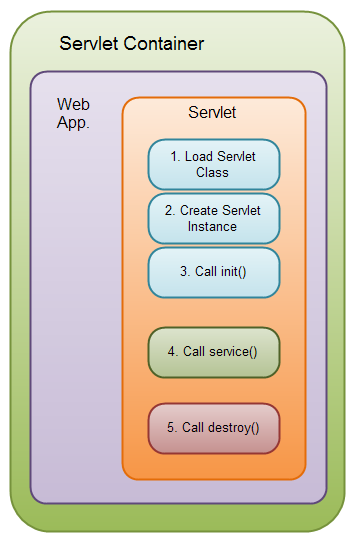
- Load Servlet Class.
- Create Instance of Servlet.
- Call the servlets init() method.
- Call the servlets service() method.
- Call the servlets destroy() method.
Step 1, 2 and 3 are executed only once, when the servlet is initially loaded. By default the servlet is not loaded until the first request is received for it. You can force the container to load the servlet when the container starts up though.
Step 4 is executed multiple times - once for every HTTP request to the servlet.Step 5 is executed when the servlet container unloads the servlet.
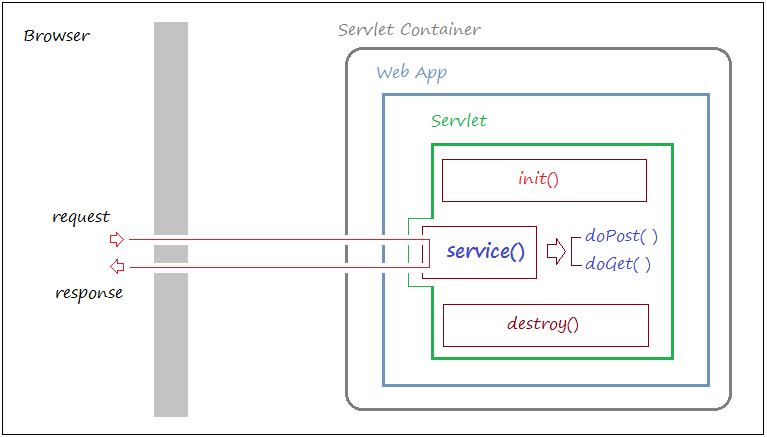
3. Install Tomcat Web Server
4. Create Web Project to start with Servlet
- File/New/Other
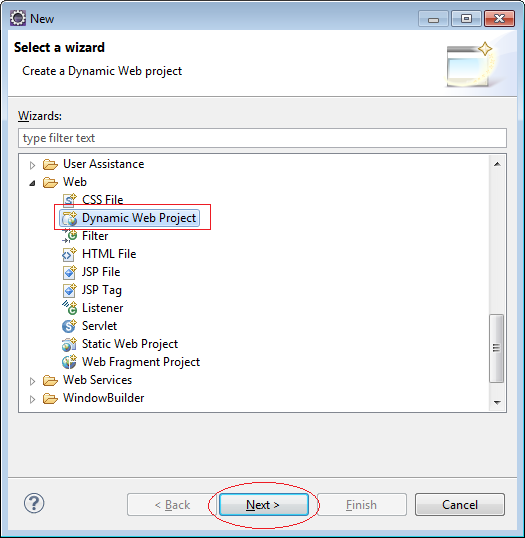
- Project Name: ServletTutorial
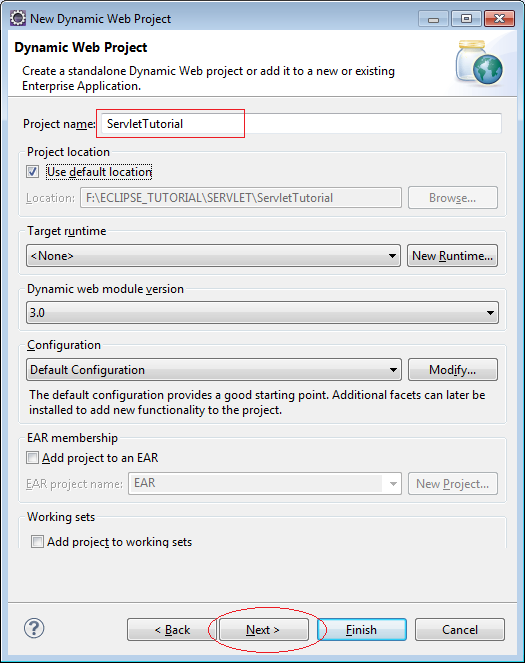
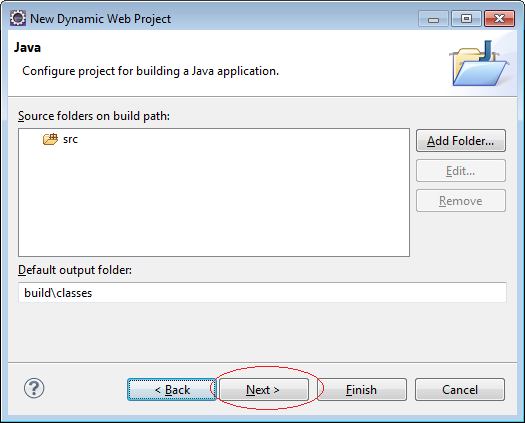
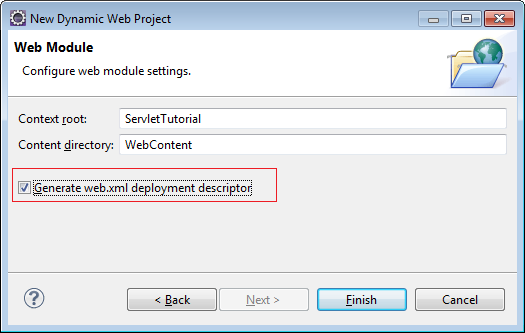
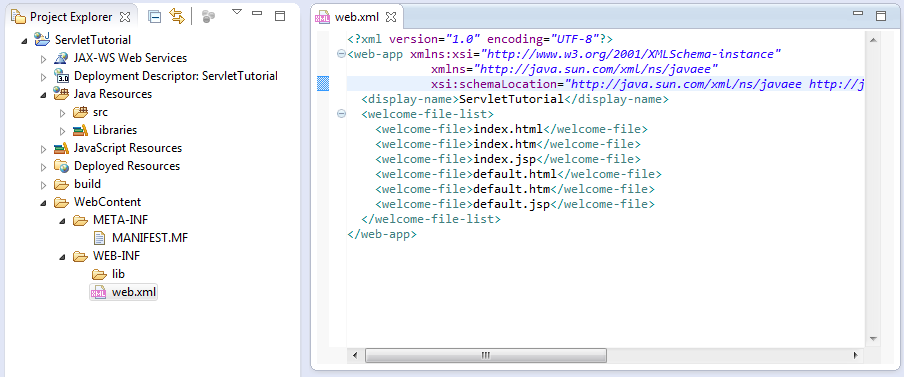
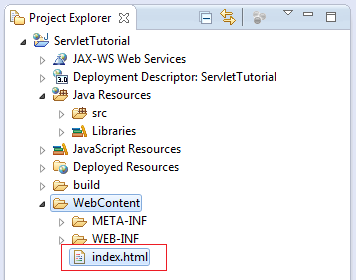
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1>Hello World</h1>
</body>
</html>
5. Configure Eclipse to run apps on tomcat
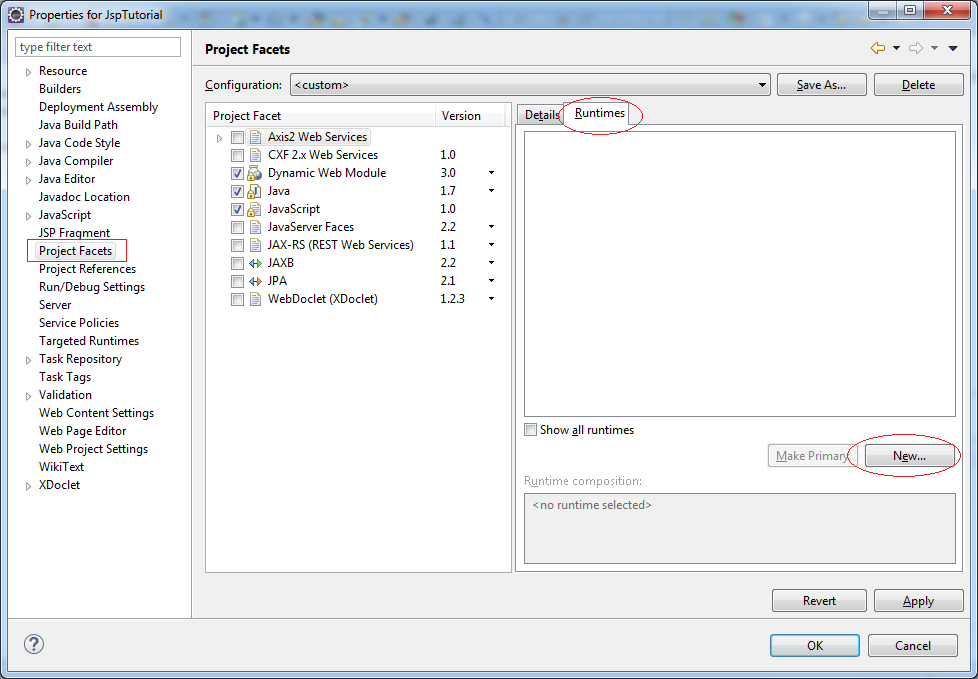
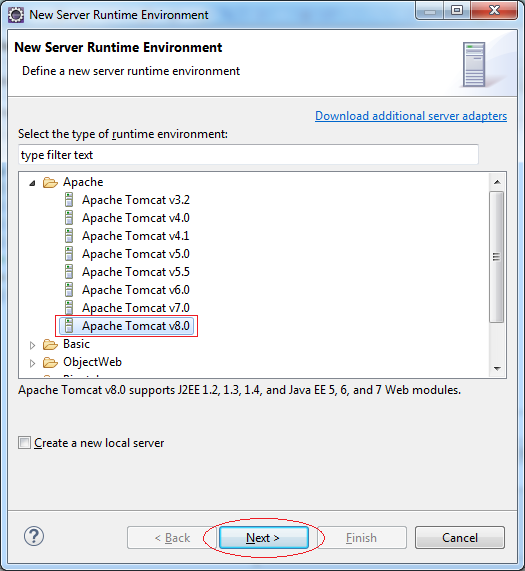
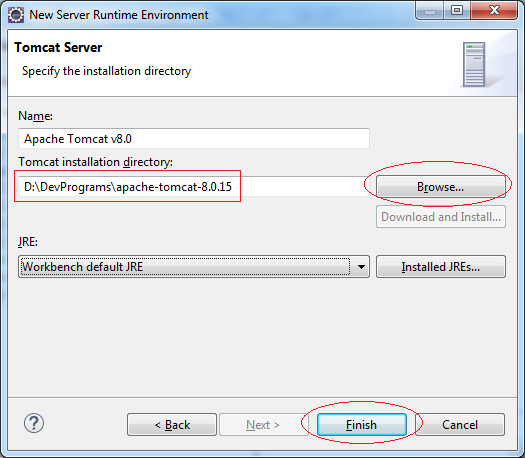
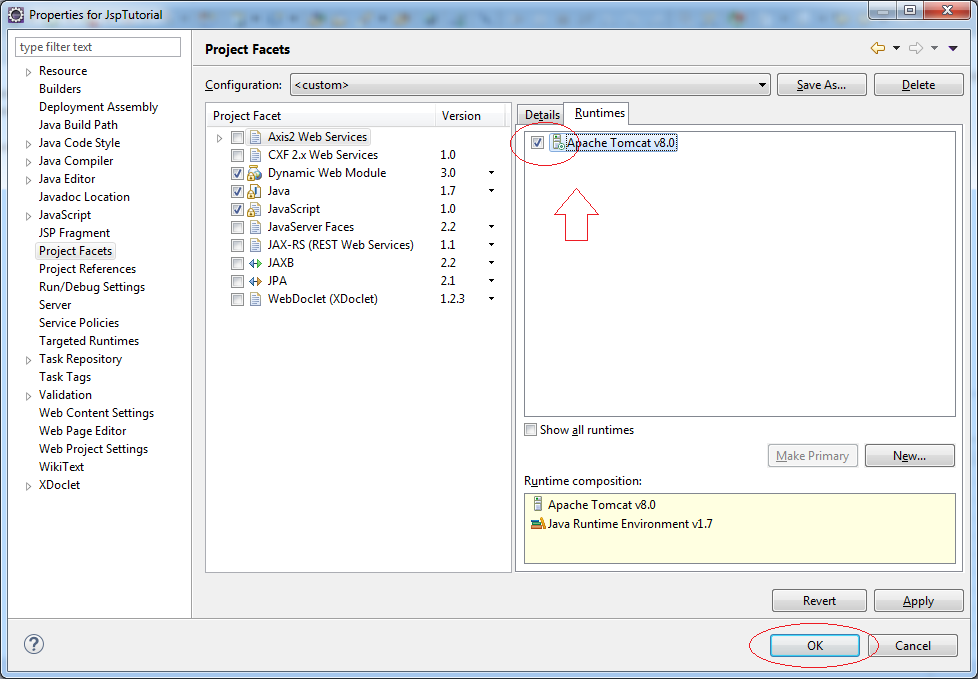

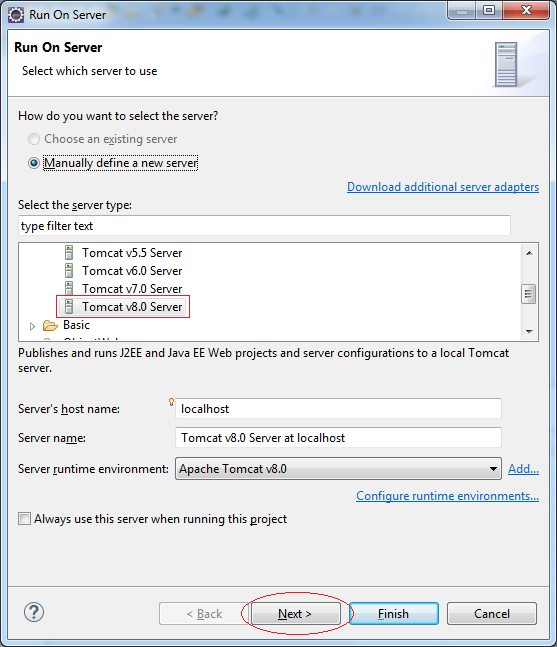
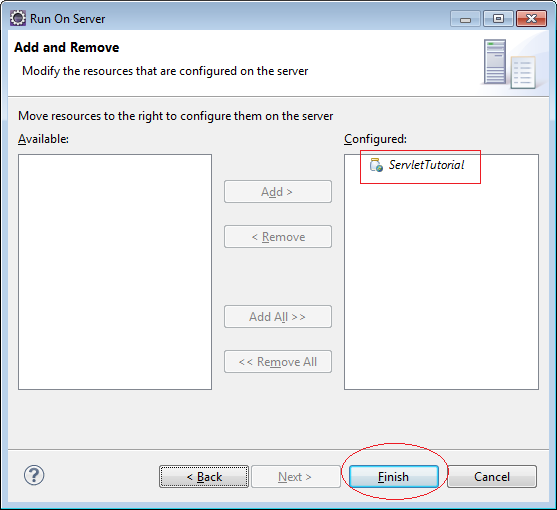
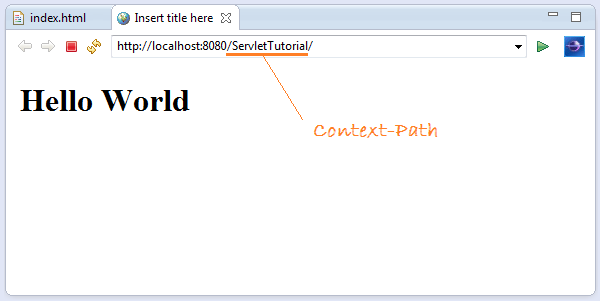
Principle of operation:
However, if you access URL:
Note: /ServletTutorial is called Context-Path. Each website has a Context-Path, and you can configure it with another value or let it empty. In the case of empty, you can access your web by:http://localhost:8080http://localhost:8080/index.htmlWhen it runs with Tomcat, Context-Path is named as Project name.
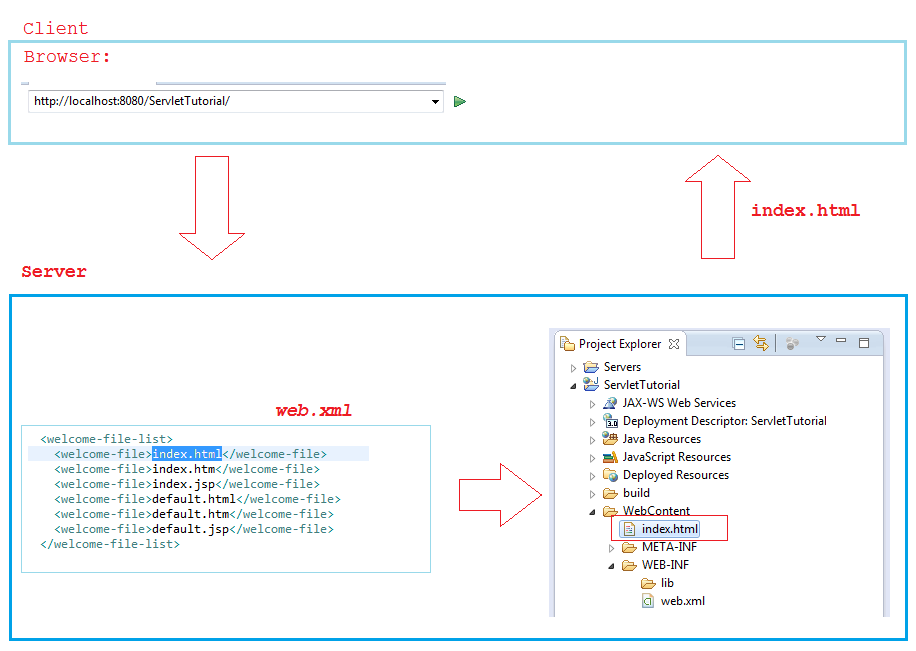
6. Some classes participated in examples
package org.o7planning.tutorial.beans;
public class Constants {
public static final String ATTRIBUTE_USER_NAME_KEY ="ATTRIBUTE_USER_NAME_KEY";
public static final String SESSION_USER_KEY ="SESSION_USER_KEY";
public static final String CALLBACK_URL_KEY ="CALLBACK_URL_KEY";
}
package org.o7planning.tutorial.beans;
public class UserInfo {
public String userName;
private int post;
private String country;
public UserInfo(String userName, String country, int post) {
this.userName= userName;
this.country= country;
this.post= post;
}
public int getPost() {
return post;
}
public void setPost(int post) {
this.post = post;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public void setUserName(String userName) {
this.userName = userName;
}
public UserInfo(String userName) {
this.userName = userName;
}
public String getUserName() {
return this.userName;
}
}
7. Create your first Servlet
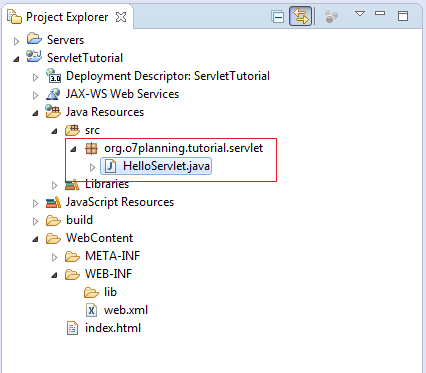
package org.o7planning.tutorial.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class HelloServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public HelloServlet() {
}
@Override
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
out.println("<html>");
out.println("<head><title>Hello Servlet</title></head>");
out.println("<body>");
out.println("<h3>Hello World</h3>");
out.println("This is my first Servlet");
out.println("</body>");
out.println("<html>");
}
@Override
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
this.doGet(request, response);
}
}
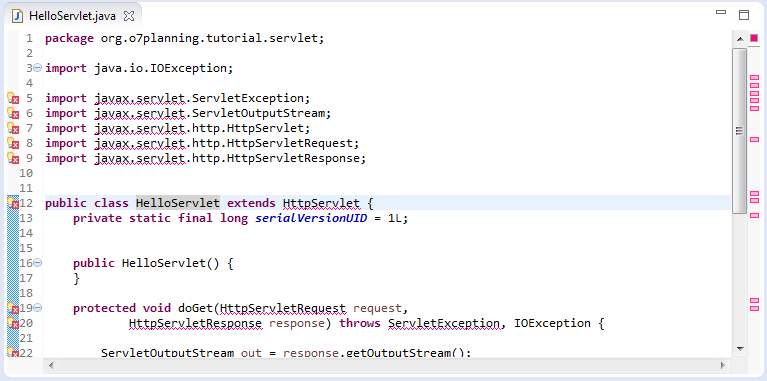
Click right mouse on Project, select Properties:
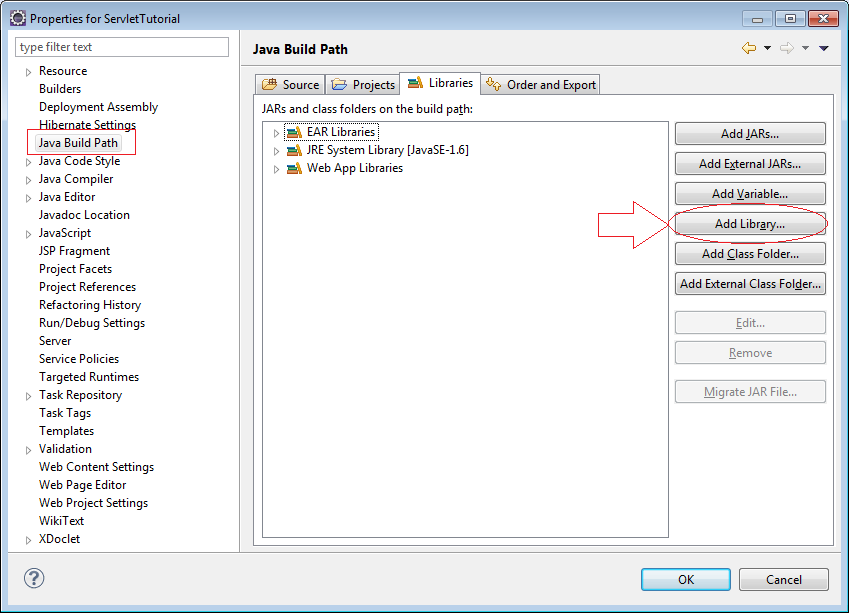
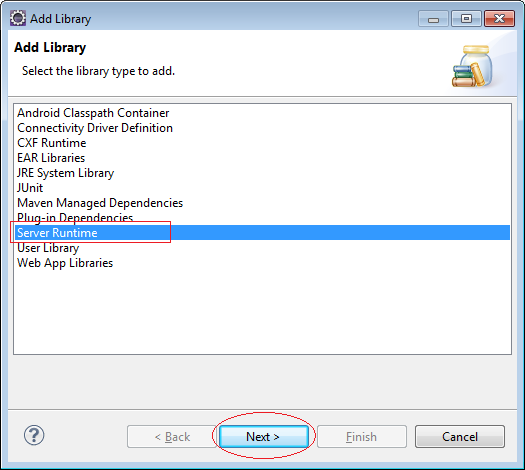
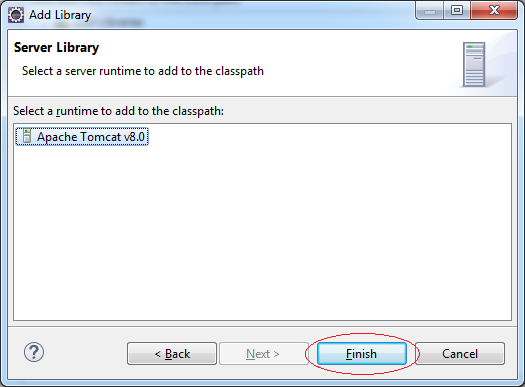
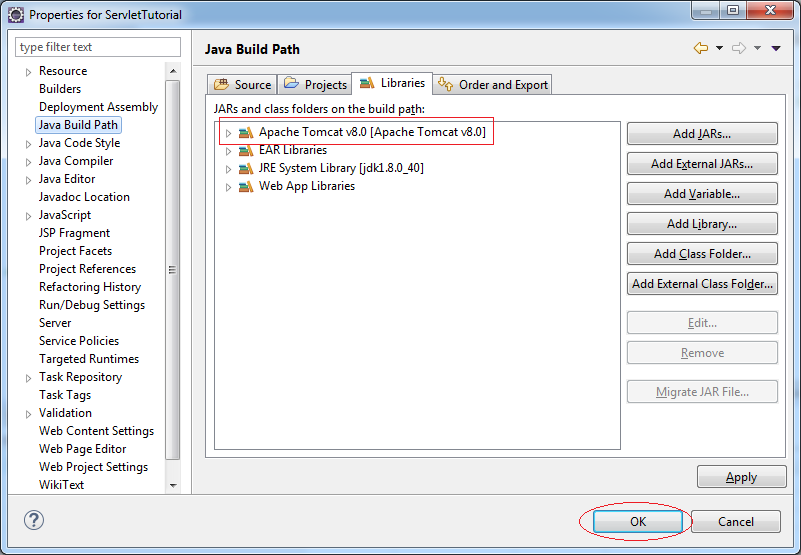
<!-- Define servlet, named helloServlet -->
<servlet>
<servlet-name>helloServlet</servlet-name>
<servlet-class>org.o7planning.tutorial.servlet.HelloServlet</servlet-class>
</servlet>
<!-- Defines the path to access this Servlet -->
<servlet-mapping>
<servlet-name>helloServlet</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>ServletTutorial</display-name>
<servlet>
<servlet-name>helloServlet</servlet-name>
<servlet-class>org.o7planning.tutorial.servlet.HelloServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>helloServlet</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
</web-app>
- Run As/Run on Server
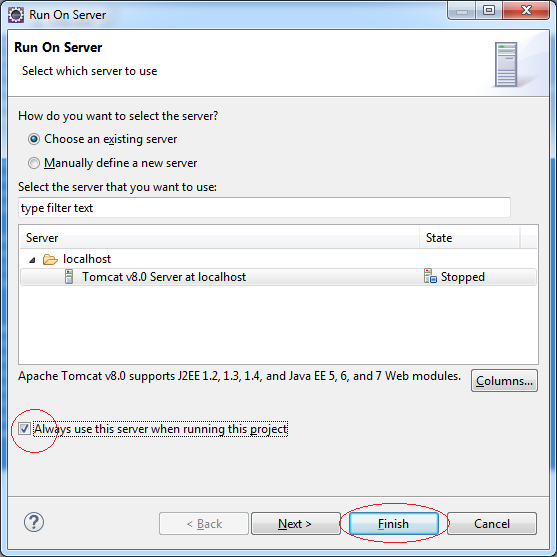
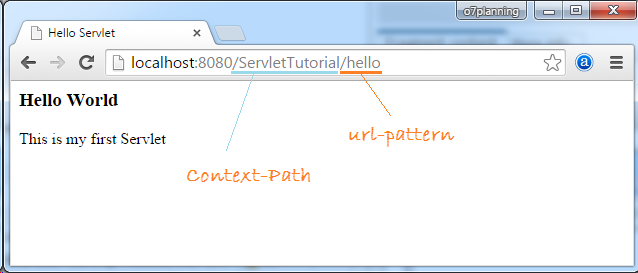
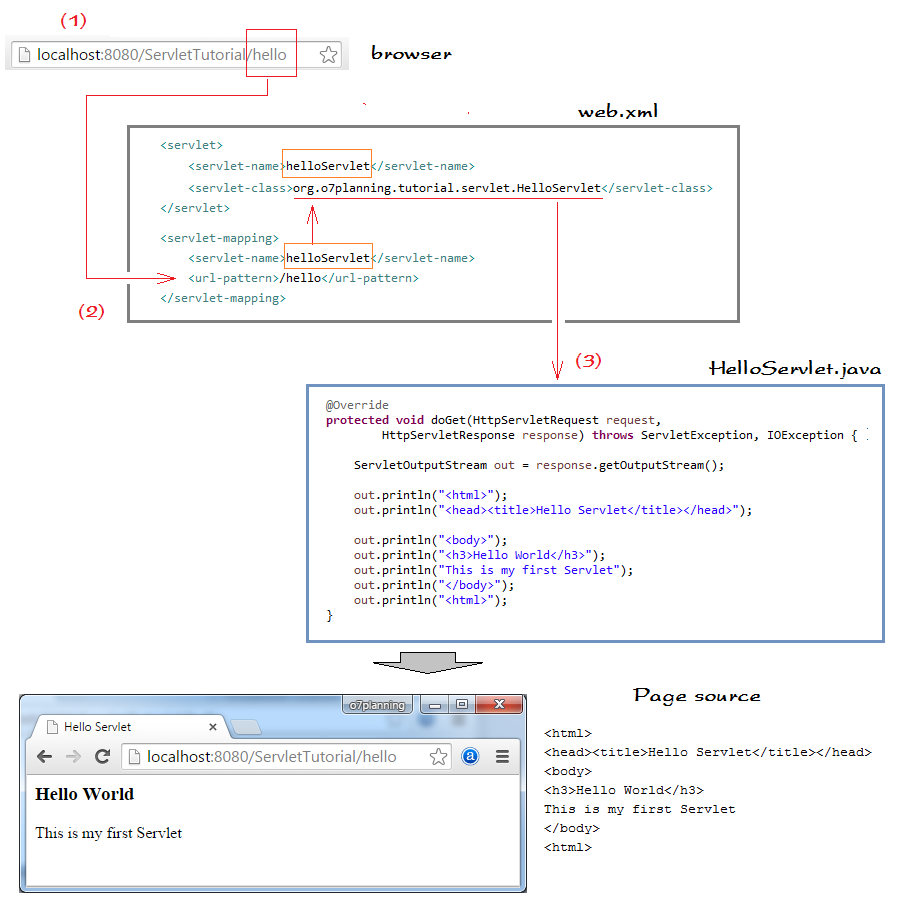
- Specifically, when called doGet(..) and when called doPost(..) we will discuss later.
// The output stream to send data to user's browser
ServletOutputStream out = response.getOutputStream();
8. Init Parameter of Servlet
package org.o7planning.tutorial.servlet;
import java.io.IOException;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class InitParamServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private String emailSupport1;
public InitParamServlet() {
}
// This method is always called once after the Servlet object is created.
@Override
public void init(ServletConfig config) throws ServletException {
super.init(config);
// Get the value of the initialization parameter of the Servlet.
// (According to the Configuration of this Servlet in web.xml).
this.emailSupport1 = config.getInitParameter("emailSupport1");
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Get the initialization parameter's value in a different way.
String emailSupport2 = this.getServletConfig().getInitParameter("emailSupport2");
ServletOutputStream out = response.getOutputStream();
out.println("<html>");
out.println("<head><title>Init Param</title></head>");
out.println("<body>");
out.println("<h3>Init Param</h3>");
out.println("<p>emailSupport1 = " + this.emailSupport1 + "</p>");
out.println("<p>emailSupport2 = " + emailSupport2 + "</p>");
out.println("</body>");
out.println("<html>");
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
<servlet>
<servlet-name>initParamServlet</servlet-name>
<servlet-class>org.o7planning.tutorial.servlet.InitParamServlet</servlet-class>
<init-param>
<param-name>emailSupport1</param-name>
<param-value>abc@example.com</param-value>
</init-param>
<init-param>
<param-name>emailSupport2</param-name>
<param-value>tom@example.com</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>initParamServlet</servlet-name>
<url-pattern>/initParam</url-pattern>
</servlet-mapping>
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>ServletTutorial</display-name>
<servlet>
<servlet-name>helloServlet</servlet-name>
<servlet-class>org.o7planning.tutorial.servlet.HelloServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>helloServlet</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
<servlet>
<servlet-name>initParamServlet</servlet-name>
<servlet-class>org.o7planning.tutorial.servlet.InitParamServlet</servlet-class>
<init-param>
<param-name>emailSupport1</param-name>
<param-value>abc@example.com</param-value>
</init-param>
<init-param>
<param-name>emailSupport2</param-name>
<param-value>tom@example.com</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>initParamServlet</servlet-name>
<url-pattern>/initParam</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
</web-app>
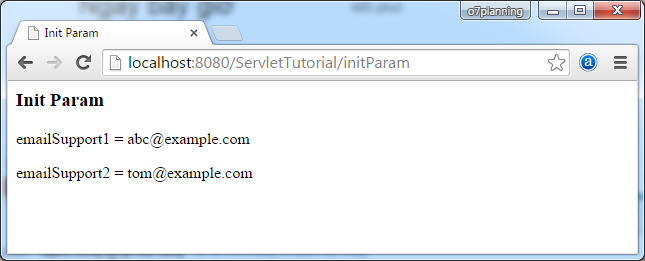
9. Configuring Servlet with Annotations
package org.o7planning.tutorial.servlet;
import java.io.IOException;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebInitParam;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
// You can configure one or multiple 'URL Patterns' can access this Servlet.
@WebServlet(urlPatterns = { "/annotationExample", "/annExample" }, initParams = {
@WebInitParam(name = "emailSupport1", value = "abc@example.com"),
@WebInitParam(name = "emailSupport2", value = "tom@example.com") })
public class AnnotationExampleServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private String emailSupport1;
public AnnotationExampleServlet() {
}
// In any case, init() is guaranteed to be called
// before the Servlet handles its first request.
@Override
public void init(ServletConfig config) throws ServletException {
super.init(config);
this.emailSupport1 = config.getInitParameter("emailSupport1");
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String emailSupport2 = this.getServletConfig().getInitParameter("emailSupport2");
ServletOutputStream out = response.getOutputStream();
out.println("<html>");
out.println("<head><title>Init Param</title></head>");
out.println("<body>");
out.println("<h3>Servlet with Annotation configuration</h3>");
out.println("<p>emailSupport1 = " + this.emailSupport1 + "</p>");
out.println("<p>emailSupport2 = " + emailSupport2 + "</p>");
out.println("</body>");
out.println("<html>");
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
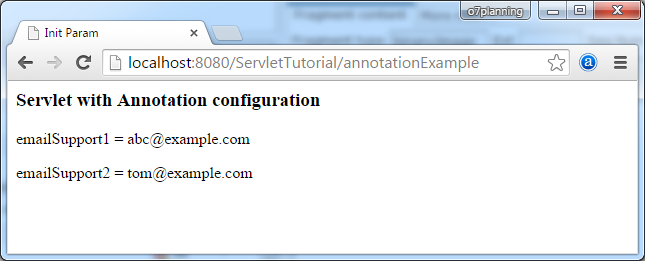
10. Servlet Url Pattern
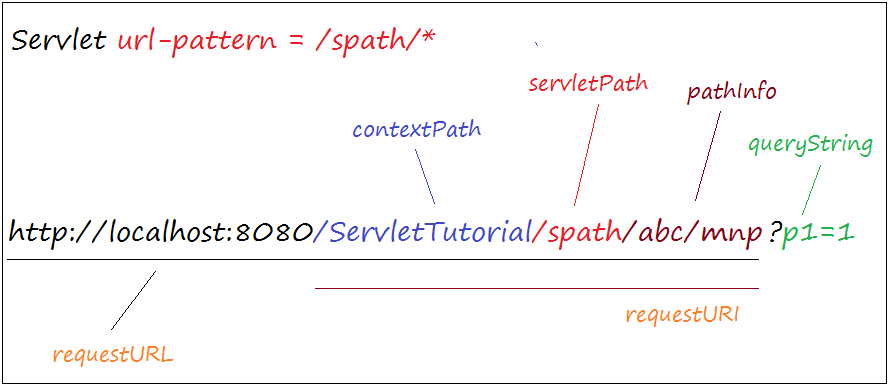
URL Pattern | Examples |
/* | http://example.com/contextPath |
/* | http://example.com/contextPath/status/abc |
/status/abc/* | http://example.com/contextPath/status/abc |
/status/abc/* | http://example.com/contextPath/status/abc/mnp |
/status/abc/* | http://example.com/contextPath/status/abc/mnp?date=today |
/status/abc/* | |
*.map | http://example.com/contextPath/status/abc.map |
*.map | http://example.com/contextPath/status.map?date=today |
*.map | |
/ | Đây là Servlet mặc định. |
Servlet will service this request from users.
The figure below illustrates how WebContainer decided to use Servlet to service request from the client.
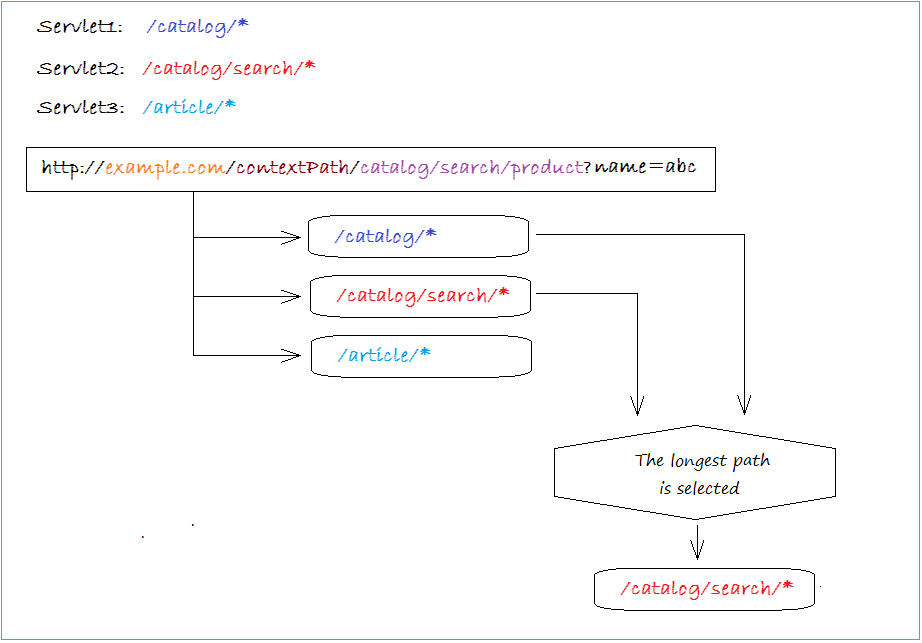
- url-pattern = "/any/*";
package org.o7planning.tutorial.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet(urlPatterns = { "/any/*" })
public class AsteriskServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public AsteriskServlet() {
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
out.println("<html>");
out.println("<head><title>Asterisk</title></head>");
out.println("<body>");
out.println("<h3>Hi, your URL match /any/*</h3>");
out.println("</body>");
out.println("<html>");
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
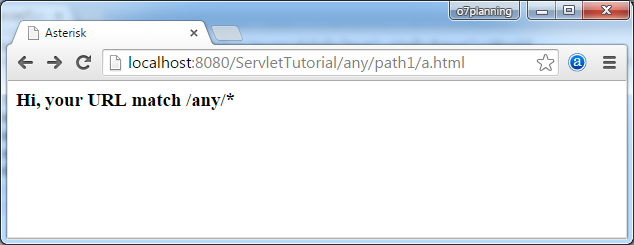
As a default servlet, servlet will be used for processing the requests that the path does not match any url-pattern of the Servlet is declared in your application.
package org.o7planning.tutorial.servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet(urlPatterns = { "/" })
public class MyDefaultServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public MyDefaultServlet() {
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
out.println("<html>");
out.println("<head><title>Page not found</title></head>");
out.println("<body>");
out.println("<h3>Sorry! Page not found</h3>");
out.println("<h1>404</h1>");
out.println("Message from servlet: " + this.getClass().getName());
out.println("</body>");
out.println("<html>");
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
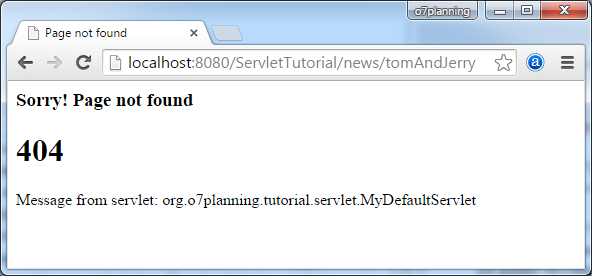
11. Get the basic information of the Servlet
- Information requests from the client.
- Server Information
- Client Information
- Header information sent on request
- ....
package org.o7planning.tutorial.servlet;
import java.io.IOException;
import java.util.Enumeration;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/other/exampleInfo")
public class ExampleInfoServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public ExampleInfoServlet() {
super();
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
out.println("<style> span {color:blue;} </style>");
String requestURL = request.getRequestURL().toString();
out.println("<br><span>requestURL:</span>");
out.println(requestURL);
String requestURI = request.getRequestURI();
out.println("<br><span>requestURI:</span>");
out.println(requestURI);
String contextPath = request.getContextPath();
out.println("<br><span>contextPath:</span>");
out.println(contextPath);
out.println("<br><span>servletPath:</span>");
String servletPath = request.getServletPath();
out.println(servletPath);
String queryString = request.getQueryString();
out.println("<br><span>queryString:</span>");
out.println(queryString);
String param1 = request.getParameter("text1");
out.println("<br><span>getParameter text1:</span>");
out.println(param1);
String param2 = request.getParameter("text2");
out.println("<br><span>getParameter text2:</span>");
out.println(param2);
// Server Infos
out.println("<br><br><b>Server info:</b>");
out.println("<br><span>serverName:</span>");
String serverName = request.getServerName();
out.println(serverName);
out.println("<br><span>serverPort:</span>");
int serverPort = request.getServerPort();
out.println(serverPort + "");
// Client Infos
out.println("<br><br><b>Client info:</b>");
out.println("<br><span>remoteAddr:</span>");
String remoteAddr = request.getRemoteAddr();
out.println(remoteAddr);
out.println("<br><span>remoteHost:</span>");
String remoteHost = request.getRemoteHost();
out.println(remoteHost);
out.println("<br><span>remoteHost:</span>");
int remotePort = request.getRemotePort();
out.println(remotePort + "");
out.println("<br><span>remoteUser:</span>");
String remoteUser = request.getRemoteUser();
out.println(remoteUser);
// Header Infos
out.println("<br><br><b>headers:</b>");
Enumeration<String> headers = request.getHeaderNames();
while (headers.hasMoreElements()) {
String header = headers.nextElement();
out.println("<br><span>" + header + "</span>: " + request.getHeader(header));
}
// Servlet Context info:
out.println("<br><br><b>Servlet Context info:</b>");
ServletContext servletContext = request.getServletContext();
// Location of web application in hard disk
out.println("<br><span>realPath:</span>");
String realPath = servletContext.getRealPath("");
out.println(realPath);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
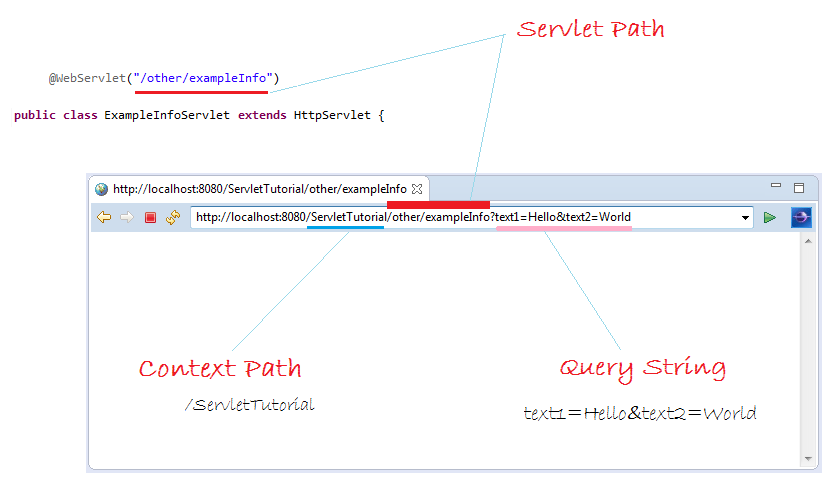
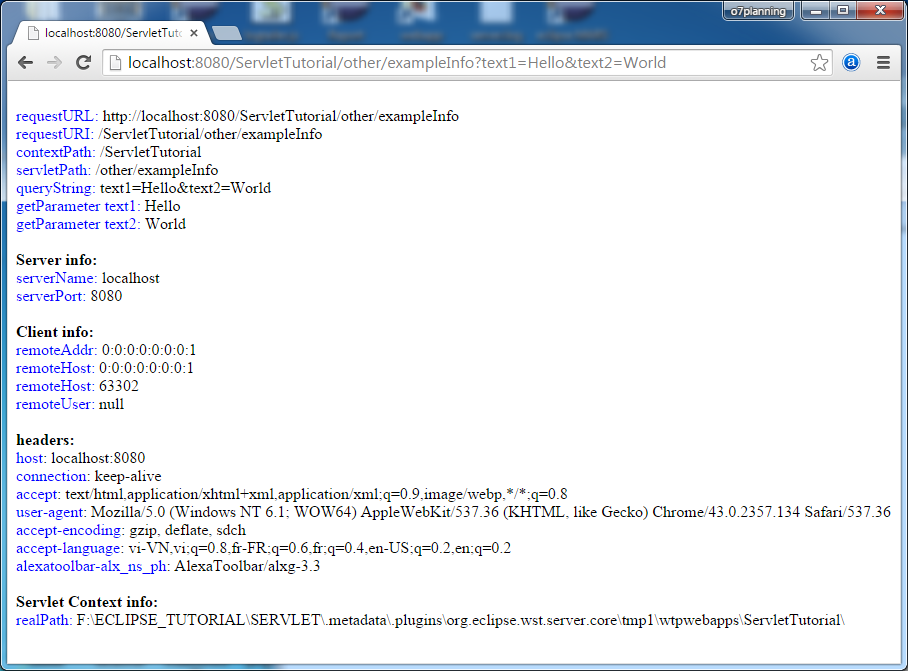
12. Forward
The page was forwarded to, compulsory is a page (or servlet) located on your web application.
With Forward you can use request.setAttribute() to transfer data from page 1 to page 2.
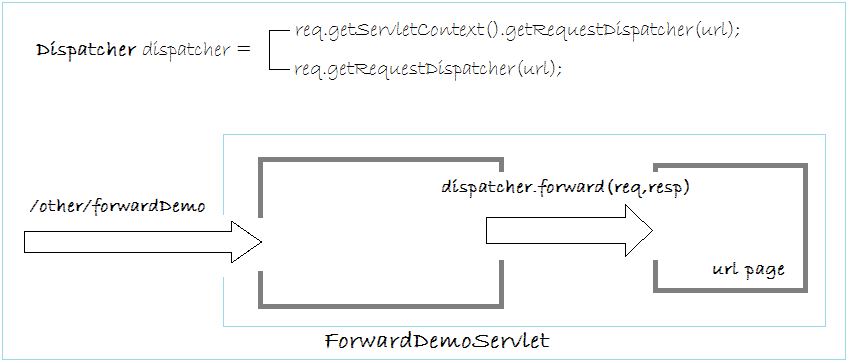
package org.o7planning.tutorial.servlet.other;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.o7planning.tutorial.beans.Constants;
@WebServlet("/other/forwardDemo")
public class ForwardDemoServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
// Request:
// http://localhost:8080/ServletTutorial/other/forwardDemo?forward=true
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Get value of parameter on URL.
String forward = request.getParameter("forward");
if ("true".equals(forward)) {
System.out.println("Forward to ShowMeServlet");
// Set data to attribute of the request.
request.setAttribute(Constants.ATTRIBUTE_USER_NAME_KEY, //
"Hi, I'm Tom come from Walt Disney !");
RequestDispatcher dispatcher //
= request.getServletContext().getRequestDispatcher("/showMe");
dispatcher.forward(request, response);
return;
}
ServletOutputStream out = response.getOutputStream();
out.println("<h3>Text of ForwardDemoServlet</h3>");
out.println("- servletPath=" + request.getServletPath());
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
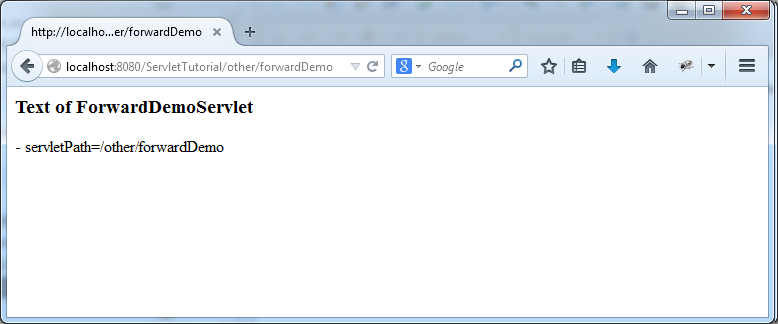
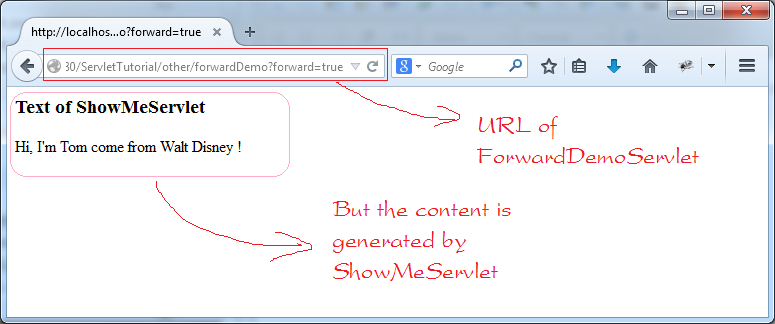

- http://localhost:8080/contextPath
- http://localhost:8080/ServletTutorial
- http://localhost:8080/ServletTutorial/other/forwardDemo
Note:
- Redirect allows you to navigate to pages, including ones outside Website.
- Forward only allows to move to pages within Website, and can transfer data between two pages through request.setAttribute.
13. Redirect
Unlike Forward. With Redirect you can not use request.setAttribute(..) to transfer data from page A to page B.
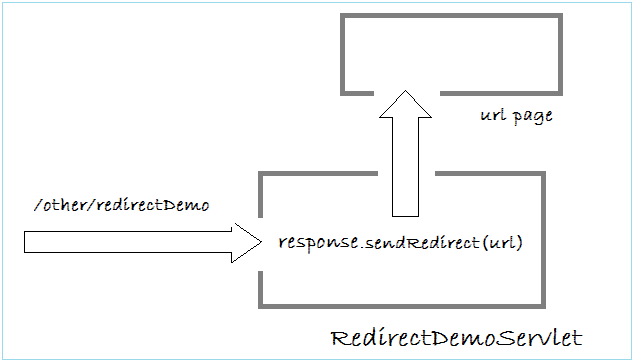
package org.o7planning.tutorial.servlet.other;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.o7planning.tutorial.beans.Constants;
@WebServlet("/showMe")
public class ShowMeServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Get value of an attribute of the request.
String value = (String) request.getAttribute(Constants.ATTRIBUTE_USER_NAME_KEY);
ServletOutputStream out = response.getOutputStream();
out.println("<h1>ShowMeServlet</h1>");
out.println(value);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
package org.o7planning.tutorial.servlet.other;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/other/redirectDemo")
public class RedirectDemoServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
// Request:
// http://localhost:8080/ServletTutorial/other/redirectDemo?redirect=true
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Get the value of parameter on the URL.
String redirect = request.getParameter("redirect");
if ("true".equals(redirect)) {
System.out.println("Redirect to ShowMeServlet");
// contextPath: Is an empty string "" or non-empty.
// If it is non-empty, it always starts with /
// and does not ends with /
String contextPath = request.getContextPath();
// ==> /ServletTutorial/showMe
response.sendRedirect(contextPath + "/showMe");
return;
}
ServletOutputStream out = response.getOutputStream();
out.println("<h3>Text of RedirectDemoServlet</h3>");
out.println("- servletPath=" + request.getServletPath());
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
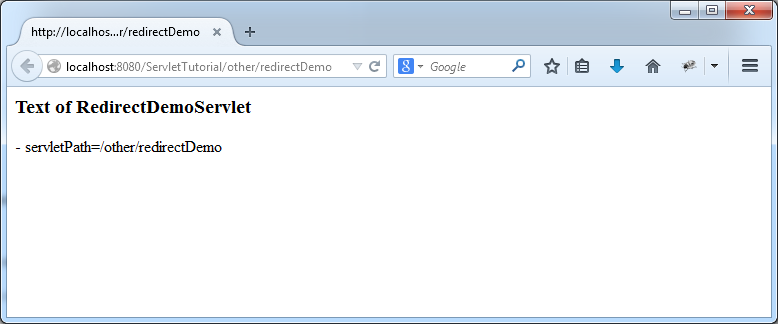
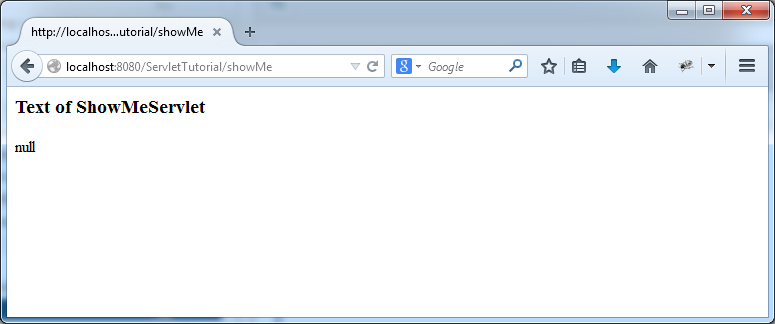
14. Session
The HttpSession object represents a user session. A user session contains information about the user across multiple HTTP requests.
When a user enters your site for the first time, the user is given a unique ID to identify his session by. This ID is typically stored in a cookie or in a request parameter.
protected void doGet(HttpServletRequest request,
HttpServletResponse response)
throws ServletException, IOException {
HttpSession session = request.getSession();
}
// Get the HttpSession object.
HttpSession session = request.getSession();
// Suppose user has successfully logged.
UserInfo loginedInfo = new UserInfo("Tom", "USA", 5);
// Store user information into a Session attribute.
// You can retrieve this information using the getAttribute method.
session.setAttribute(Constants.SESSION_USER_KEY, loginedInfo);
// Get the HttpSession object.
HttpSession session = request.getSession();
// Get the UserInfo object stored to the session
// after the user login successfully.
UserInfo loginedInfo
= (UserInfo) session.getAttribute(Constants.SESSION_USER_KEY);
package org.o7planning.tutorial.servlet.session;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.o7planning.tutorial.beans.Constants;
import org.o7planning.tutorial.beans.UserInfo;
@WebServlet(urlPatterns = { "/login" })
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public LoginServlet() {
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
// Get HttpSession object
HttpSession session = request.getSession();
// Suppose a user has successfully logged.
UserInfo loginedInfo = new UserInfo("Tom", "USA", 5);
// Storing user information in an attribute of Session.
session.setAttribute(Constants.SESSION_USER_KEY, loginedInfo);
out.println("<html>");
out.println("<head><title>Session example</title></head>");
out.println("<body>");
out.println("<h3>You are logined!, info stored in session</h3>");
out.println("<a href='userInfo'>View User Info</a>");
out.println("</body>");
out.println("<html>");
}
}
package org.o7planning.tutorial.servlet.session;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.o7planning.tutorial.beans.Constants;
import org.o7planning.tutorial.beans.UserInfo;
@WebServlet(urlPatterns = { "/userInfo" })
public class UserInfoServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public UserInfoServlet() {
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
// Get HttpSession object.
HttpSession session = request.getSession();
// Get UserInfo object stored in session after user login successful.
UserInfo loginedInfo = (UserInfo) session.getAttribute(Constants.SESSION_USER_KEY);
// If not logined, redirect to login page (LoginServlet).
if (loginedInfo == null) {
// ==> /ServletTutorial/login
response.sendRedirect(this.getServletContext().getContextPath() + "/login");
return;
}
out.println("<html>");
out.println("<head><title>Session example</title></head>");
out.println("<body>");
out.println("<h3>User Info:</h3>");
out.println("<p>User Name:" + loginedInfo.getUserName() + "</p>");
out.println("<p>Country:" + loginedInfo.getCountry() + "</p>");
out.println("<p>Post:" + loginedInfo.getPost() + "</p>");
out.println("</body>");
out.println("<html>");
}
}
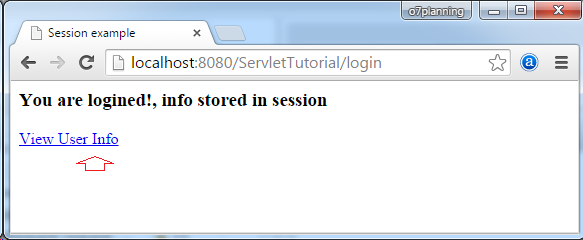
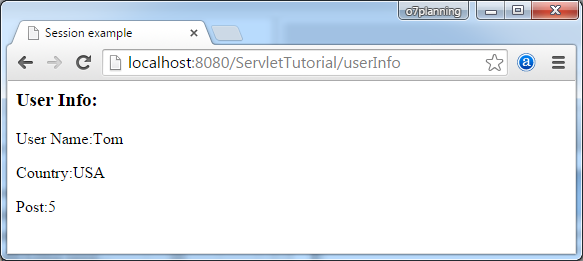
Java Servlet/Jsp Tutorials
- Install Tomcat Server for Eclipse
- Install Glassfish Web Server on Windows
- Run Maven Java Web Application in Tomcat Maven Plugin
- Run Maven Java Web Application in Jetty Maven Plugin
- Run background task in Java Servlet Application
- Java Servlet Tutorial for Beginners
- Java Servlet Filter Tutorial with Examples
- Java JSP Tutorial for Beginners
- Java JSP Standard Tag Library (JSTL) Tutorial with Examples
- Install Web Tools Platform for Eclipse
- Create a simple Login application and secure pages with Java Servlet Filter
- Create a Simple Java Web Application Using Servlet, JSP and JDBC
- Uploading and downloading files stored to hard drive with Java Servlet
- Upload and download files from Database using Java Servlet
- Displaying Image from Database with Java Servlet
- Redirect 301 Permanent redirect in Java Servlet
- How to automatically redirect http to https in a Java Web application?
- Use Google reCAPTCHA in Java Web Application