Java Awssdk Common Credentials Providers
To interact with Amazon Web Services (AWS), you need to create an AwsCredentialsProvider object to provide credentials to AWS each time a request is sent to AWS.
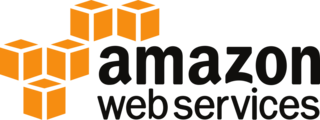
Essentially, credentials are an "accessKeyId/secretAccessKey" value pair, which is also called an "Access Key", and is included in each request sent to AWS. This value pair can be hardcoded in Java code via StaticCredentialsProvider. Additionally "Access Key" can also be stored in a file and read by ProfileCredentialsProvider, or stored in environment variables and read by EnvironmentVariableCredentialsProvider, ...
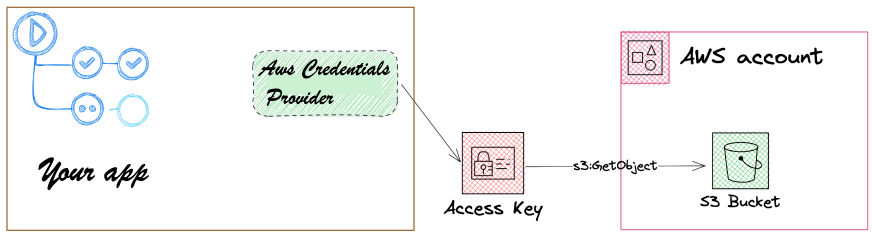
So, first you need to ensure that you have created an "Access Key" on AWS. The article below guides you to complete this step.
1. Library
<!-- https://mvnrepository.com/artifact/software.amazon.awssdk/s3 -->
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>s3</artifactId>
<version>2.21.10</version>
</dependency>
<!-- Log Library -->
<!-- https://mvnrepository.com/artifact/org.slf4j/slf4j-api -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>2.0.9</version>
</dependency>
<!-- Log Library -->
<!-- https://mvnrepository.com/artifact/org.slf4j/slf4j-simple -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>2.0.9</version>
</dependency>
2. StaticCredentialsProvider
A simple and fast way to create an AwsCredentialsProvider object is to provide the accessKeyId and secretAccessKey directly in the Java code.
Create a Java class to store the "accessKeyId/secretAccessKey" obtained in the step above:
MyAccessKey.java
package org.o7planning.java_14219_awssdk_s3;
public class MyAccessKey {
public static final String ACCESS_KEY_ID = "Your-Access-Key-ID";
public static final String SECRET_ACCESS_KEY = "Your-Secret-Access-Key";
}
A utility class that creates an StaticCredentialsProvider object with "accessKeyId/secretAccessKey" is provided directly.
StaticCredentialsProviderExample1.java
package org.o7planning.java_14219_awssdk_s3;
import software.amazon.awssdk.auth.credentials.AwsBasicCredentials;
import software.amazon.awssdk.auth.credentials.AwsCredentials;
import software.amazon.awssdk.auth.credentials.StaticCredentialsProvider;
import software.amazon.awssdk.regions.Region;
public class StaticCredentialsProviderExample1 {
public static StaticCredentialsProvider createCredentialsProvider(Region region) {
AwsCredentials credentials = AwsBasicCredentials.create( //
MyAccessKey.ACCESS_KEY_ID, // accessKeyId
MyAccessKey.SECRET_ACCESS_KEY // secretAccessKey
);
StaticCredentialsProvider credentialsProvider = StaticCredentialsProvider.create(credentials);
return credentialsProvider;
}
}
See the topical article on StaticCredentialsProvider with detailed and advanced explanations:
- Java Awssdk StaticCredentialsProvider
3. SystemPropertyCredentialsProvider
The "accessKeyId/secretAccessKey" value pair can be provided to the AWSSDK via System Properties. They are passed to the Java virtual machine at the time the virtual machine is started.
java -jar myweb.war -Daws.accessKeyId=my-accessKeyId -Daws.secretAccessKey=my-secretAccessKey
The values of the two properties below will be read by SystemPropertyCredentialsProvider:
- aws.accessKeyId
- aws.secretAccessKey
Example, create an SystemPropertyCredentialsProvider object with "accessKeyId/secretAccessKey" provided through System Properties.
SystemPropertyS3ClientUtils.java
package org.o7planning.java_14219_awssdk_s3;
import software.amazon.awssdk.auth.credentials.SystemPropertyCredentialsProvider;
import software.amazon.awssdk.regions.Region;
public class SystemPropertyCredentialsProviderExample1 {
public static SystemPropertyCredentialsProvider createCredentialsProvider(Region region) {
//
// SystemPropertyCredentialsProvider will read "System Properties":
// aws.accessKeyId
// aws.secretAccessKey
//
return SystemPropertyCredentialsProvider.create();
}
}
See the topical article about SystemPropertyCredentialsProvider with detailed and advanced explanations:
- Java System Properties
4. ProfileCredentialsProvider
ProfileCredentialsProvider allows reading "accessKeyId/secretAccessKey" in a file located somewhere in the host. This file is called "Profile-File", its location can be specified in the Java code or set through an environment variable, or be a default path convention.
There are two types of "Profile Files":
- ProfileFile.Type.CREDENTIALS
- ProfileFile.Type.CONFIGURATION
Basically, you can specify the path of the "Profile File" directly in Java code, or through an environment variable or using the default path convention.
- {UserHome}/.aws/credentials
- {UserHome}/.aws/config
ProfileCredentialsProviderExample1.java
package org.o7planning.java_14219_awssdk_s3;
import java.nio.file.Paths;
import software.amazon.awssdk.auth.credentials.ProfileCredentialsProvider;
import software.amazon.awssdk.profiles.ProfileFile;
import software.amazon.awssdk.regions.Region;
//
// ProfileFile.Type.CREDENTIALS Example.
//
public class ProfileCredentialsProviderExample1 {
private static final String MY_PROFILE_FILE_PATH = "/Volumes/New/my-profile-credentials.txt";
public static ProfileCredentialsProvider createCredentialsProvider(Region region) {
System.out.println("User Home: " + System.getProperty("user.home"));
// Profile File:
ProfileFile profileFile = ProfileFile.builder() //
.type(ProfileFile.Type.CREDENTIALS) // CREDENTIALS or CONFIGURATION
.content(Paths.get(MY_PROFILE_FILE_PATH)) //
.build();
// Credentials Provider
return ProfileCredentialsProvider.builder() //
.profileFile(profileFile) //
// .profileName("my-custom-profile-1") // Optional
.build();
}
}
my-profile-credentials.txt
# This is a Comment Line 1
# This is a Comment Line 2
#
# ProfileType.Type.CREDENTIALS Sample:
#
# Profile Definition values: [default] or [your-custom-name]
[default] # Default profile
aws_access_key_id = Your-Access-Key-Id-1
aws_secret_access_key = Your-Secret-Access-Key-1
[my-custom-profile-1] # Custom Profile.
aws_access_key_id = Your-Access-Key-Id-2
aws_secret_access_key = Your-Secret-Access-Key-2
See the topical article about ProfileCredentialsProvider with detailed and advanced explanations:
5. EnvironmentVariableCredentialsProvider
The "accessKeyId/secretAccessKey" values can be set via environment variables and read by EnvironmentVariableCredentialsProvider.
Specifically, EnvironmentVariableCredentialsProvider will read the 3 environment variables below, of which the third environment variable is an option.
- AWS_ACCESS_KEY_ID
- AWS_SECRET_ACCESS_KEY
- AWS_SESSION_TOKEN (Optional)
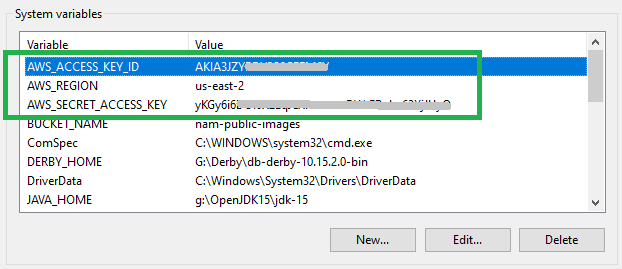
Image illustrating environment variables on Windows operating system.
After setting the environment variables, creating an EnvironmentVariableCredentialsProvider object is really simple.
EnvironmentVariableCredentialsProviderExample1.java
package org.o7planning.java_14219_awssdk_s3;
import software.amazon.awssdk.auth.credentials.EnvironmentVariableCredentialsProvider;
import software.amazon.awssdk.regions.Region;
public class EnvironmentVariableCredentialsProviderExample1 {
public static EnvironmentVariableCredentialsProvider createCredentialsProvider(Region region) {
//
// EnvironmentVariableCredentialsProvider will read Environment Variables:
// AWS_ACCESS_KEY_ID
// AWS_SECRET_ACCESS_KEY
// AWS_SESSION_TOKEN (Optional)
//
return EnvironmentVariableCredentialsProvider.create();
}
}
See the topical article about EnvironmentVariableCredentialsProvider with detailed and advanced explanations:
6. InstanceProfileCredentialsProvider
InstanceProfileCredentialsProvider is useful if you use Amazon AWS's EC2 service, it provides credentials that help your EC2 connect to other AWS resources.
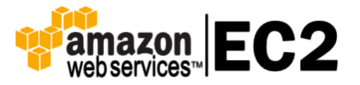
- Giới thiệu về Amazon EC2
InstanceProfileCredentialsProviderExample1.java
package org.o7planning.java_14219_awssdk_s3;
import software.amazon.awssdk.auth.credentials.InstanceProfileCredentialsProvider;
import software.amazon.awssdk.regions.Region;
public class InstanceProfileCredentialsProviderExample1 {
public static InstanceProfileCredentialsProvider createCredentialsProvider(Region region) {
return InstanceProfileCredentialsProvider.create();
}
}
See the topical article about InstanceProfileCredentialsProvider with detailed and advanced explanations:
- Java Awssdk InstanceProfileCredentialsProvider
7. ContainerCredentialsProvider
Wait for update...
ContainerS3ClientUtils.java
package org.o7planning.java_awssdk_s3.s3client;
import software.amazon.awssdk.auth.credentials.AwsCredentialsProvider;
import software.amazon.awssdk.auth.credentials.ContainerCredentialsProvider;
import software.amazon.awssdk.regions.Region;
import software.amazon.awssdk.services.s3.S3Client;
public class ContainerS3ClientUtils {
public static S3Client createS3Client(Region region) {
//
// AWS_CONTAINER_CREDENTIALS_RELATIVE_URI
// AWS_CONTAINER_CREDENTIALS_FULL_URI
//
AwsCredentialsProvider provider = ContainerCredentialsProvider.builder().build();
S3Client client = S3Client.builder() //
.credentialsProvider(provider) //
.region(region) //
.build();
return client;
}
}
See the topical article about ContainerCredentialsProvider with detailed and advanced explanations:
- Java Awssdk ContainerCredentialsProvider
Amazon Web Services Tutorials
- Introduction to Amazon Web Services (AWS)
- Introduction to Amazon S3
- Introduction to Amazon Cloudfront and its architecture
- How to reduce Amazon Cloudfront Costs?
- Amazon CloudFront Invalidation
- Introduction to DigitalOcean Spaces
- Create DigitalOcean Spaces Bucket
- Introduction to Amazon ACM
- Java Awssdk S3 S3Client Upload object
- Create AWS accessKeyId/secretAccessKey
- Java Awssdk S3 List objects
- Host a static website on Amazon S3
- Java Awssdk CloudFront Invalidation
- DigitalOcean Spaces Create Access Key
- Java Awssdk Common Credentials Providers
- Java Awssdk ProfileCredentialsProvider
- Java Awssdk Creating and using EnvironmentVariableCredentialsProvider
- Java Awssdk Creating and using SystemPropertyCredentialsProvider
- Java Awssdk S3 Upload object with S3TransferManager
- Java Awssdk S3 S3TransferManager download object
- Java Manipulate DigitalOcean Spaces using S3TransferManager
- Java Create, list and delete S3 Bucket
- Aws Console create IAM User
- Create Amazon S3 Bucket
- Configure custom domain for Amazon S3 static website
- Create a CloudFront distribution for S3 Bucket
- Configure Amazon CloudFront Error Pages
- Amazon S3 Bucket policies
- Amazon AWS Policy Generator - policygen
- Migrate DNS service to Amazon Route 53
- Transfer domain registration to Amazon Route 53
- Request an SSL certificate from Amazon ACM
Show More