Dart Properties Tutorial and Examples
1. What is Property?
Before giving the definition "What is property?" we need to clarify "What is a field?".
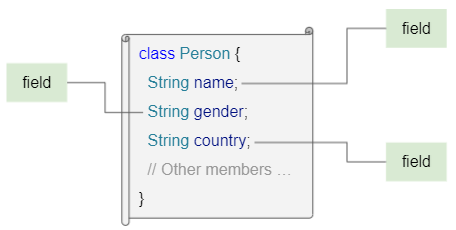
A field is a variable declared directly in a class.
See the constructor article to understand how Dart assigns values to fields:
- Dart Constructors
In the Dart language, all the fields of the class can be accessed from outside the class. You can get their values and set new values for them. For example:
field_ex1.dart
class Person {
String name;
String gender;
String? country; // Allow null value.
Person(this.name, this.gender, this.country); // Constructor
Person.nameAndGender(this.name, this.gender); // Constructor
// Method:
void selfIntroduce() {
if (country != null) {
print('Hi, My name is $name, from $country');
} else {
print('Hi, My name is $name');
}
}
}
void main() {
Person emma = new Person('Emma', 'Female', 'USA'); // Create an object
emma.selfIntroduce(); // Call method.
var name = emma.name; // get the value of a field
print('emma.name: $name');
print('emma.gender: ${emma.gender}');
print('emma.country: ${emma.country}');
print(' --- Set new value to country field ---: ');
emma.country = 'Canada'; // set new value to a field
print('emma.country: ${emma.country}');
}
Output:
Hi, My name is Emma, from USA
emma.name: Emma
emma.gender: Female
emma.country: USA
--- Set new value to country field ---:
emma.country: Canada
Private Field?
Unlike Java, Dart does not provide private, protected and public keywords. If you want a private field, you should name the field starting with an underscore ( _ ). For example _fieldName.
Fields with names starting with an underscore ( _ ) are implicitly private, they are used internally by the library. It's merely an implicit convention, programmers using the library should not access these fields from the outside as it is against the idea of library design. Intentionally accessing these fields from the outside can cause unexpected mistakes.
Property?
Basically, once you can reach a field somewhere, you can retrieve its value and assign a new value to it.
Property is a similar concept to a field, however it has more special features. Property is divided into 3 types:
- Read-only Property: Allow access to its value, but does not allow to set new values for it.
- Write-only Property: Allow to set a new value for it, but does not allow access to its value.
- Read/Write Property: Allows access to the value, and allows setting a new value for it.
According to Dart's design idea, you should name all the fields in your class starting with an underscore ( _ ), this means they are only used internally by the library and using properties to replace the role of fields in communicating with the outside world.
2. Getter
The Getter syntax allows you to define a property outside the class that can access its value, but cannot set a new value for it unless you also define a Setter for this property.
data_type get property_name {
// code ...
return a_value;
}
For example, the Person class below allows access to the value of the property name but does not allow its value to be changed from the outside.
property_getter_ex1.dart
class Person {
String _name; // private field
String gender;
String? _country; // private field
Person(this._name, this.gender, this._country); // Constructor
Person.nameAndGender(this._name, this.gender); // Constructor
// Getter
String get name {
return _name;
}
// Getter
String get country {
return _country ?? '[Not Provided]';
}
// Method:
void selfIntroduce() {
if (_country != null) {
print('Hi, My name is $name, from $country');
} else {
print('Hi, My name is $name');
}
}
}
void main() {
var emma = Person.nameAndGender('Emma', 'Female'); // Create an object
emma.selfIntroduce(); // Call method.
var name = emma.name; // get the value of a property
var country = emma.country; // get the value of a property
print('emma.name: $name');
print('emma.country: $country');
// Can not set new value to property - name
// emma.name = 'New Name'; // ERROR!!
}
Output:
Hi, My name is Emma
emma.name: Emma
emma.country: [Not Provided]
3. Setter
The Setter syntax allows you to define a property that allows to set a new value for it, but does not allow access to its value unless you also define a Getter for this property.
set property_name(data_type newValue) {
// code
}
Example:
property_setter_ex1.dart
class Person {
String name;
String gender;
String _country; // private field
Person(this.name, this.gender, this._country); // Constructor
// Setter
set country(String newCountry) {
_country = newCountry;
}
// Method:
void selfIntroduce() {
print('Hi, My name is $name, from $_country');
}
}
void main() {
var emma = Person('Emma', 'Female', 'USA'); // Create an object
emma.selfIntroduce(); // Call method.
// Set new value to country property.
emma.country = 'Canada';
emma.selfIntroduce();
// Can not get the value of country property
// var country = emma.country; // ERROR!!
}
Output:
Hi, My name is Emma, from USA
Hi, My name is Emma, from Canada
4. Examples
Example: A property with both Getter and Setter:
property_gettersetter_ex1.dart
class Person {
String name;
String gender;
String? _country; // private field
Person(this.name, this.gender, this._country); // Constructor
Person.nameAndGender(this.name, this.gender); // Constructor
// Getter
String get country {
return _country ?? '[Not Provided]';
}
// Setter
set country(String newCountry) {
_country = newCountry;
}
// Method:
void selfIntroduce() {
if(_country != null) {
print('Hi, My name is $name, from $_country');
} else {
print('Hi, My name is $name');
}
}
}
void main() {
var emma = Person.nameAndGender('Emma', 'Female'); // Create an object
emma.selfIntroduce(); // Call method.
var country = emma.country;
print('Country: $country');
print(' --- set new value to country property ---');
emma.country = 'Canada'; // Set new value to country property.
emma.selfIntroduce();
}
Output:
Hi, My name is Emma
emma.country: [Not Provided]
--- set new value to country property ---
Hi, My name is Emma, from Canada
Dart Programming Tutorials
- Dart Boolean Tutorial with Examples
- Dart Functions Tutorial with Examples
- Dart Closures Tutorial with Examples
- Dart methods Tutorial and Examples
- Dart Properties Tutorial and Examples
- Dart dot dot ( .. ) operator
- Dart programming with DartPad online tool
- Install Dart SDK on Windows
- Install Visual Studio Code on Windows
- Install Dart Code Extension for Visual Studio Code
- Install Dart Plugin for Android Studio
- Run your first Dart example in Visual Studio Code
- Run your first Dart example in Android Studio
- Parsing JSON with dart:convert
- Dart List Tutorial with Examples
- Dart Variables Tutorial with Examples
- Dart Map Tutorial with Examples
- Dart Loops Tutorial with Examples
- Dart dart_json_mapper Tutorial with Examples
- What is Transpiler?
Show More