Dart Variables Tutorial with Examples
1. What is variable?
In the Dart programming language, a variable is a name (an identifier) that points to an address where data is stored in memory.
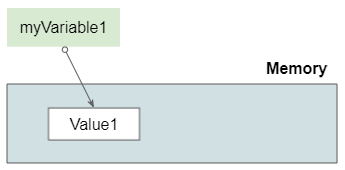
Example:
var myVariable1 = 100;
- var is a keyword to declare a variable.
- myVariable1 is the variable name.
- 100 is the value of this variable.
Here are the rules for naming variables:
- The keyword cannot be used to name a variable. For example "var" is a keyword, which cannot be used to name variables.
- The variable's name consists of letters of the Latin alphabet and numbers.
- The variable name cannot contain spaces or special characters except for the underscore ( _ ) or the dollar sign ( $ ).
- Variable names cannot begin with a digit.
2. Declare variable with var keyword
Variable declaration is mandatory before use. For each name you only need to declare it once.
The simplest way to declare a variable is to use the var keyword:
var myVariable1 = "My Text"; // Declare a variable and assign a value to it.
var myVariable2; // Declare a variable but have not assigned a value.
You can assign a new value to the variable, which means that the variable has pointed to a new data storage address in memory.
myVariable1 = "New My Text";
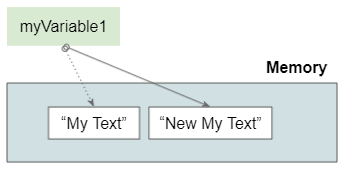
Example:
Variable_var_ex1.dart
void main() {
var myVariable1 = "My Text";
print(myVariable1);
myVariable1 = "New My Text";
print(myVariable1);
}
Output:
My Text
New My Text
Using the "var" keyword to declare a variable means you're telling Dart: "Detect the variable's data type yourself". The data type of the variable will be determined the first time it is assigned a value. If you declare a variable but never assign a value to it, its data type is Null.
Variable_var_ex2.dart
void main() {
var myVariable1 = "My Text"; // Data type: String
print("data type of myVariable1: " + myVariable1.runtimeType.toString());
var myVariable2 = 1000; // Data type: int
print("data type of myVariable2: " + myVariable2.runtimeType.toString());
var myVariable3; // Data type: Null
print("data type of myVariable3: " + myVariable3.runtimeType.toString());
myVariable3 = true; // Data type: bool
print("data type of myVariable3: " + myVariable3.runtimeType.toString());
}
Output:
data type of myVariable1: String
data type of myVariable2: int
data type of myVariable3: Null
data type of myVariable3: bool
- Dart Data Types
A variable is declared with the var keyword and the data type is detected as non-Null. You cannot assign it a value with a different data type.
Example: A variable has been determined by Dart that its data type is String. The compiler will report an error if you assign a numeric value to it.
void main() {
var myVariable1 = "My Text"; // Data type: String
print("data type of myVariable1: " + myVariable1.runtimeType.toString());
// Can not assign to it an integer value.
myVariable1 = 1000; // Error at compile time.
}
Compiler error message:
Error compiling to JavaScript:
Warning: Interpreting this as package URI, 'package:dartpad_sample/main.dart'.
lib/main.dart:6:17:
Error: A value of type 'int' can't be assigned to a variable of type 'String'.
myVariable1 = 1000; // Error at compile time.
^
Error: Compilation failed.
3. Declare variable with dynamic keyword
The dynamic keyword is also used to declare a variable, which is quite similar to the var keyword. The difference is that the data type of this variable can be changed.
dynamic v1 = 123; // v1 is of type int.
v1 = 456; // changing value of v1 from 123 to 456.
v1 = 'abc'; // changing type of v1 from int to String.
var v2 = 123; // v2 is of type int.
v2 = 456; // changing value of v2 from 123 to 456.
v2 = 'abc'; // ERROR: can't change type of v2 from int to String.
4. Declare variable with specific data type
You can declare a variable with a specific data type instead of asking Dart to automatically detect its data type.
String myVariable1 = "My Text";
int myVariable2 = 1000;
Specifying a specific data type for the variable at the time of declaration will help reduce errors in the coding process.
For example: You declare a variable of String type but assign it a numeric value, the compiler will give you an error.
String myVariable1 = 1000; // Error at compile time.
- Dart Data Types
5. Declare variable with final keyword
The final keyword is also used to declare a variable, and you can only assign a value to this variable once. The data type of this variable will be determined by Dart in the first assignment.
void main() {
final myVariable1; // Declare a variable.
myVariable1 = 2; // Assign a value to it.
myVariable1 = 3; // ERROR at compile time.
final myVariable2 = "Some Text"; // Declare and assign value to variable.
myVariable2 = "New Text"; // ERROR at compile time.
}
Using the final keyword helps you prevent accidentally assigning a new value to a variable when it already has a value. This ensures that the variable only points to a single address in memory during its lifetime.
A variable is declared with the final keyword. The data in the memory area to which this variable points can still change internally.
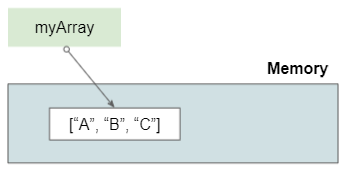
Example: The value of a variable is an array, the elements of the array can still change.
Variable_final_ex2.dart
void main() {
final myArray = ["A", "B", "C"];
print(myArray);
myArray[2] = "E";
print(myArray);
}
Output:
[A, B, C]
[A, B, E]
- Dart Switch
6. Declare variable with const keyword
The const keyword is used to declare a special variable, also known as a constant. When declaring a variable with the const keyword, you should assign a value to it, otherwise its value will be null. Once declared you cannot assign a value to this variable. The data area that the variable points to will become immutable, meaning it cannot change the data internally.
Variable_const_ex1.dart
void main() {
const myVariable1 = 100; // Declare and assign value to variable.
myVariable1 = 2; // ERROR: Can not assign new value.
const myVariable2; // Declare a variable (Its value is considered null).
myVariable2 = "Some Text"; // ERROR: Can not assign new value.
}
A variable is declared with the const keyword. The memory area it points to becomes immutable.
Example: The value of a variable is an array, this array will become immutable.
Variable_const_ex2.dart
void main() {
const myArray = ["A", "B", "C"];
print(myArray);
myArray[2] = "E"; // ERROR: myArray is immutable.
print(myArray);
}
Output:
[A, B, C]
: Unsupported operation: indexed setError: Unsupported operation: indexed set
- Dart Switch
Dart Programming Tutorials
- Dart Boolean Tutorial with Examples
- Dart Functions Tutorial with Examples
- Dart Closures Tutorial with Examples
- Dart methods Tutorial and Examples
- Dart Properties Tutorial and Examples
- Dart dot dot ( .. ) operator
- Dart programming with DartPad online tool
- Install Dart SDK on Windows
- Install Visual Studio Code on Windows
- Install Dart Code Extension for Visual Studio Code
- Install Dart Plugin for Android Studio
- Run your first Dart example in Visual Studio Code
- Run your first Dart example in Android Studio
- Parsing JSON with dart:convert
- Dart List Tutorial with Examples
- Dart Variables Tutorial with Examples
- Dart Map Tutorial with Examples
- Dart Loops Tutorial with Examples
- Dart dart_json_mapper Tutorial with Examples
- What is Transpiler?
Show More