The concept of Callback in NodeJS
1. What is Callback?
To explain what the Callback is, let's see a situation as follows:
You come to a shop to buy a something you like. The shop staff tells you that the item is out of stock now. You leave the phone number and ask them to call back immediately after the goods is available. Then you can go out or do a job and do not need to care about the shop anymore, until you get a phone call notifying of the item asked by you from the shop.
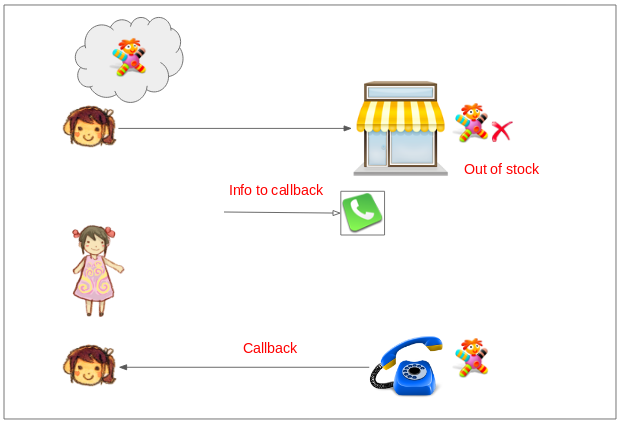
NodeJS server can receive many requests from many users. Therefore to improve serviceability, all APIs of the NodeJS are designed to support Callback. The "callback" is a function that will be called when the NodeJs completes a specific task.
2. Example of NodeJS Callback
In the NodeJS, APIs is designed to support the Callback. Assume that you are writing a program to read 2 files. To do this you use the fs module, which gives you two functions such as readFile and readFileSync to read the file. We will learn about the differences between these two functions.
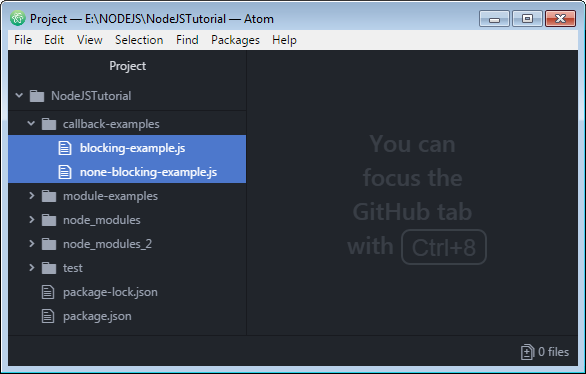
Blocking
The readFileSync is a function reading files synchronously,. Therefore, while this function is executing, it will block the program from executing next code lines.
blocking-example.js
var fs = require("fs");
// -----> Read file 1:
console.log("\n");
console.log("Read File 1");
var data1 = fs.readFileSync('C:\\test\\file1.txt');
console.log("- Data of file 1: ");
console.log(data1.toString());
// -----> Read file 2:
console.log("\n");
console.log("Read File 2");
var data2 = fs.readFileSync('C:\\test\\file2.txt');
console.log("- Data of file 2: ");
console.log(data2.toString());
console.log("\n");
console.log("Program Ended");
Open CMD window and execute the blocking-example.js file
node callback-examples/blocking-example.js
And this is the results received by you:
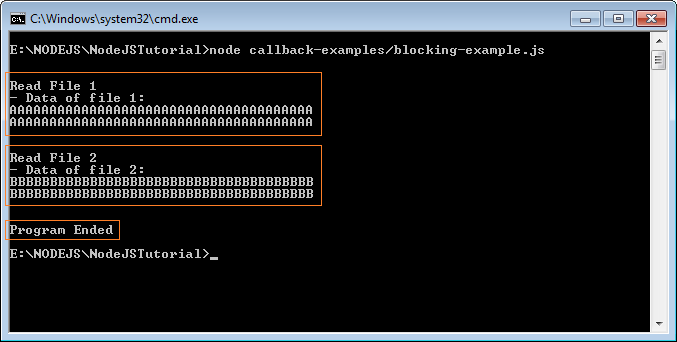
Non Blocking
You should use readFile function to achieve better performance for the program. This function reads files asynchronously. It doesn't block the program executing the next code lines. In other words, the program does not wait for the function to complete. But when this function completes its task, it calls the Callback function.
// Read a file Asynchronous.
fs.readFile('C:\\test\\file1.txt', aCallbackFunction );
See the full example:
non-blocking-example.js
var fs = require("fs");
// A Callback function!
function readFinishedFile1(err, data) {
if (err) console.log(err);
console.log("- Data of file 1: ");
console.log(data.toString());
}
// A Callback function!
function readFinishedFile2(err, data) {
if (err) console.log(err);
console.log("- Data of file 2: ");
console.log(data.toString());
}
// -----> Read file 1:
console.log("\n");
console.log("Read File 1");
fs.readFile('C:\\test\\file1.txt', readFinishedFile1);
// -----> Read file 2:
console.log("\n");
console.log("Read File 2");
fs.readFile('C:\\test\\file2.txt', readFinishedFile2);
console.log("\n");
console.log("Program Ended \n");
Open CMD window and run the non-blocking-example.js file:
node callback-examples/non-blocking-example.js
And this is the result that you receives:
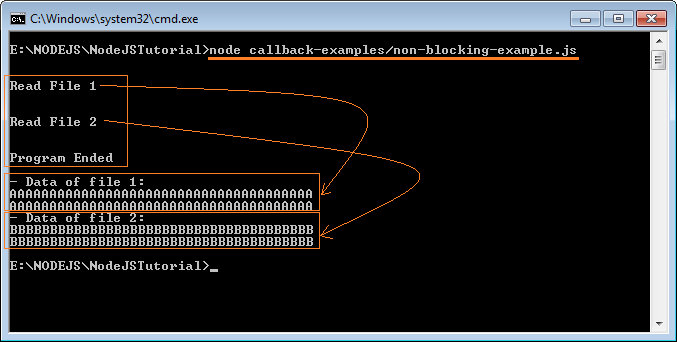
NodeJS Tutorials
- Introduction to NodeJs
- What is NPM?
- NodeJS Tutorial for Beginners
- Install Atom Editor
- Install NodeJS on Windows
- NodeJS Modules Tutorial with Examples
- The concept of Callback in NodeJS
- Create a Simple HTTP Server with NodeJS
- Understanding Event Loop in NodeJS
- NodeJS EventEmitter Tutorial with Examples
- Connect to MySQL database in NodeJS
Show More