Run your first TypeScript example in Visual Studio Code
1. Necessary installations
To be able to create and run TypeScript applications on Visual Studio Code, make sure that the following software is installed on your computer:
2. Create a project
On your computer create a new empty folder to store the project. Such as:
- C:/TypeScript/myfirstproject
Next, on Visual Studio Code open the folder you just created in the previous step.
- File > Open Folder
Click the "New File" icon to create a new TypeScript file.
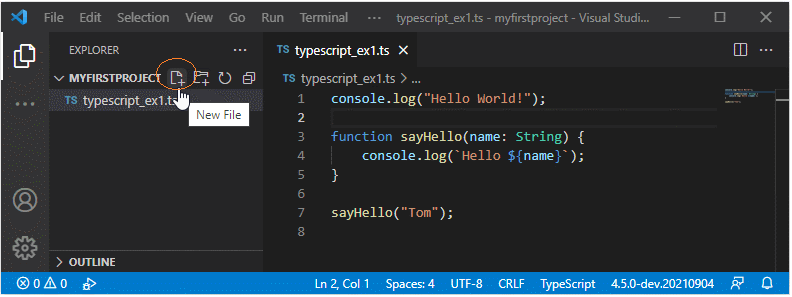
typescript_ex1.ts
console.log("Hello World!");
function sayHello(name: String) {
console.log(`Hello ${name}`);
}
sayHello("Tom");
Next, we need to configure to run the above example.
3. Project Configuration
On Visual Studio Code open a Terminal window:
- View > Terminal
On a Terminal window, execute the following command to create a configuration file for your project:
tsc --init
After executing the above command, the tsconfig.json file is created:
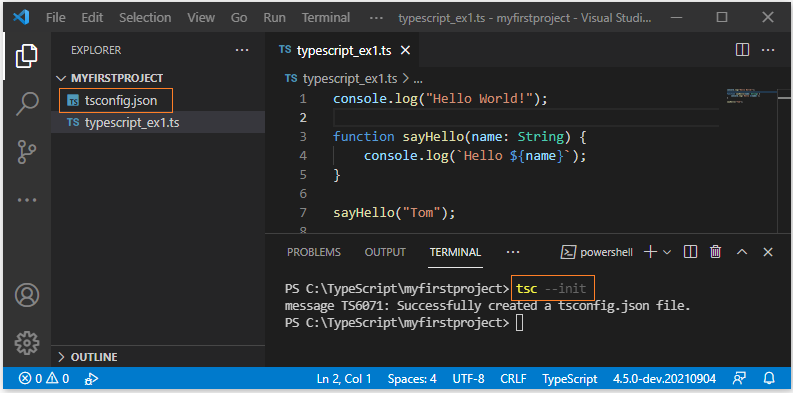
If you receive an error message like the one below, consider one of the solutions from Stackoverflow:tsc : File C:\Users\Windows10\AppData\Roaming\npm\tsc.ps1 cannot be loaded because running scripts is disabled on this system. For more information, see about_Execution_Policies at https:/go.microsoft.com/fwlink/?LinkID=135170. At line:1 char:1 + tsc typescript_ex1.ts + ~~~ + CategoryInfo : SecurityError: (:) [], PSSecurityException + FullyQualifiedErrorId : UnauthorizedAccess
Open the tsconfig.json file to change the values of some parameters.
Find line starting with.. | Change to |
"target": "es5" | "target": "es6" |
// "outDir": "./", | "outDir": "./dist", |
tsconfig.json
{
"compilerOptions": {
"target": "es6",
"outDir": "./dist",
// Other codes...
}
}
Next, on the Terminal window of Visual Studio Code, execute the command to compile all your typescript files into javascript files.
tsc
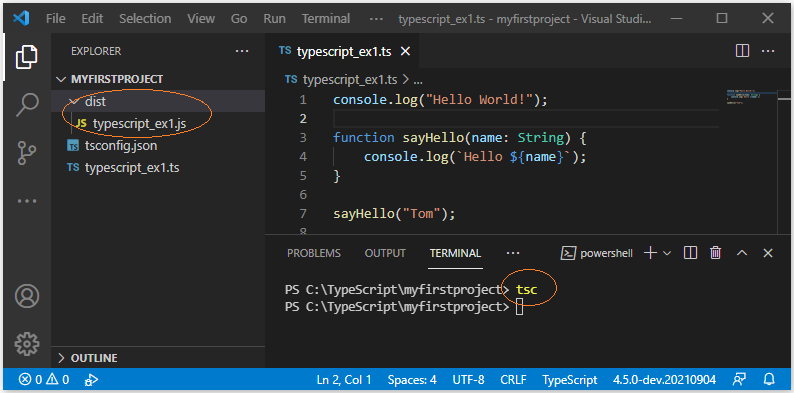
Finally, run the example.
node ./dist/typescript_ex1.js
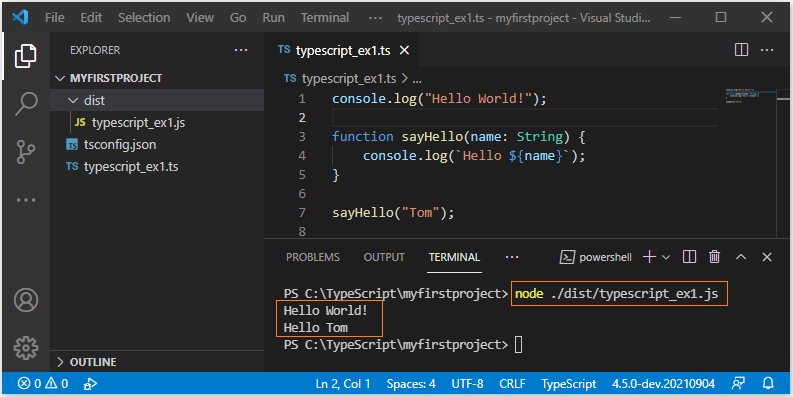
TypeScript Tutorials
- Run your first TypeScript example in Visual Studio Code
- TypeScript Namespaces Tutorial with Examples
- TypeScript Modules tutorial with Examples
- TypeScript typeof operator
- TypeScript Loops Tutorial with Examples
- Install TypeScript on Windows
- TypeScript Functions Tutorial with Examples
- TypeScript Tuples Tutorial with Examples
- TypeScript Interfaces Tutorial with Examples
- TypeScript Arrays Tutorial with Examples
- TypeScript instanceof operator
- TypeScript Methods Tutorial with Examples
- TypeScript Closures Tutorial with Examples
- TypeScript Constructors Tutorial with Examples
- TypeScript Properties Tutorial with Examples
- Parsing JSON in TypeScript
- Parsing JSON in TypeScript with the json2typescript library
- What is Transpiler?
Show More