TypeScript Arrays Tutorial with Examples
1. What is array?
In TypeScript, array is a special data type used to store many values of many different data types. Unlike Java, array in TypeScript can automatically expand its length if necessary.
A Type[] array in TypeScript has the same way of storing data as Map<Integer,Type> in Java. We will clarify this in the article.
Similar to JavaScript, TypeScript supports two syntaxes for creating an array:
Type[] Syntax:
The Type[] syntax is the common syntax for declaring an array, which is used in many different languages and of course includes TypeScript:
Example: Declare an array just to store string elements. The TypeScript compiler will report an error if you accidentally add an element of another type to the array.
let fruits: string[];
fruits = ['Apple', 'Orange', 'Banana'];
// Or:
let fruits: string[] = ['Apple', 'Orange', 'Banana'];
Example: Declare an array with elements of different data types:
let arr = [1, 3, 'Apple', 'Orange', 'Banana', true, false];
Example: Declare an array with union data type - number and string:
let values: (string | number)[] = ['Apple', 'Orange', 1, 2, 'Banana', 3];
Array<Type> Syntax:
Declaring an array based on the Array<Type> syntax is also equivalent to the Type[] syntax. There is no difference.
let fruits: Array<string>;
fruits = ['Apple', 'Orange', 'Banana'];
// Or:
let fruits: Array<string> = ['Apple', 'Orange', 'Banana'];
In TypeScript, the Type[] syntax is just a shorthand of the Array<Type> syntax. Arrays in TypeScript are objects of the Array<T> interface, so they comply with the standards defined in this interface (see below).
Example: Declare an array with union data type - number and string:
let values: Array<string | number> = ['Apple', 'Orange', 1, 2, 'Banana', 3];
2. Accessing array elements
The array elements can be accessed using the index of an element e.g. myArray[index]. The array index starts from zero, so the index of the first element is zero, the index of the second element is one and so on.
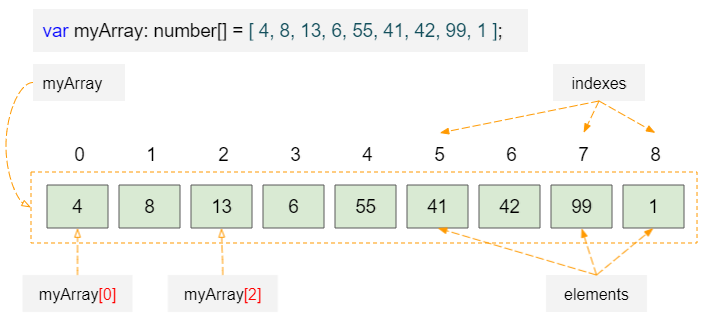
Example:
array_ex1.ts
function array_ex1_test() {
var myArray: number[] = [4, 8, 13, 6, 55, 41, 42, 99, 1];
console.log("Length of myArray: " + myArray.length); // 9 Elements
console.log("Element at index 0: " + myArray[0]); // 4
console.log("Element at index 1: " + myArray[1]); // 8
console.log("Element at index 4: " + myArray[4]); // 55
}
array_ex1_test(); // Call the function
Output:
Length of myArray: 9
Element at index 0: 4
Element at index 1: 8
Element at index 4: 55
You can also assign new values to the elements of an array through their index:
array_ex2.ts
function array_ex2_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`fruits[0] = ${fruits[0]}`);
console.log(`fruits[1] = ${fruits[1]}`);
console.log("--- Assign new values to elements ... --- ")
fruits[0] = "Breadfruit";
fruits[1] = "Carambola";
console.log(`fruits[0] = ${fruits[0]}`);
console.log(`fruits[1] = ${fruits[1]}`);
}
array_ex2_test(); // Call the function.
Output:
fruits[0] = Acerola
fruits[1] = Apple
--- Assign new values to elements ... ---
fruits[0] = Breadfruit
fruits[1] = Carambola
Unlike Java, arrays in TypeScript can automatically increase in length if necessary. In the example below, we have an array with initial length 3. We assign values to the elements at index 6 and 7. Now, the length of the array will be 8.
array_ex3.ts
function array_ex3_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3
console.log(" --- Set the value for the elements at index 6 and 7. ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[7] = "Carambola";
console.log(`Now Array length is ${fruits.length}`); // 8 indexes: [0,1,2,3,4,5,6,7]
console.log(`Element at index 4: ${fruits[4]}`); // undefined
console.log(`Element at index 5: ${fruits[5]}`); // undefined
}
array_ex3_test(); // Call the function.
Output:
Array length is 3
--- Set the value for the elements at index 6 and 7. ---
Now Array length is 8
Element at index 4: undefined
Element at index 5: undefined
3. Non-integer index
Unlike Java and C#. TypeScript arrays accept non-integer indices, which also accepts negative indices. However, such elements do not count towards the length of the array.
array_idx_ex1.ts
function array_idx_ex1_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3
console.log(" --- Set the value for the elements at indexes 6, 10.5 and -100 ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[10.5] = "Carambola"; // !!!!!!!!!!
fruits[-100] = "Grapefruit"; // !!!!!!!!!!
console.log(`Now Array length is ${fruits.length}`); // 7 indexes: [0,1,2,3,4,5,6]
console.log(`Element at index 4: ${fruits[4]}`); // undefined
console.log(`Element at index 5: ${fruits[5]}`); // undefined
console.log(`Element at index 10.5: ${fruits[10.5]}`); // Carambola
console.log(`Element at index -100: ${fruits[-100]}`); // Grapefruit
}
array_idx_ex1_test(); // Call the function.
Output:
Array length is 3
--- Set the value for the elements at indexes 6, 10.5 and -100 ---
Now Array length is 7
Element at index 4: undefined
Element at index 5: undefined
Element at index 10.5: Carambola
Element at index -100: Grapefruit
4. Loop over array
Basically, there are two for loop syntaxes for accessing the elements of an array.
Syntax 1:
This syntax only allows access to elements with a non-negative integer index.
for(let idx =0; idx < fruits.length; idx++) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
Example:
array_for_loop_ex1.ts
function array_for_loop_ex1_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3 indexes [0,1,2]
console.log(" --- Set the value for the elements at indexes 6 and 10.5 ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[10.5] = "Carambola"; // !!!!!!!!!!
for(let idx =0; idx < fruits.length; idx++) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
}
array_for_loop_ex1_test(); // Call the function.
Output:
Element at index 0 is Acerola
Element at index 1 is Apple
Element at index 2 is Banana
Element at index 3 is undefined
Element at index 4 is undefined
Element at index 5 is undefined
Element at index 6 is Breadfruit
Syntax 2:
The for loop syntax below only accesses indices that actually exist in the array, including indices that are not integers or negative numbers.
for(let idx in fruits) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
Example:
array_for_loop_ex2.ts
function array_for_loop_ex2_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3 indexes [0,1,2]
console.log(" --- Set the value for the elements at indexes 6 and 10.5 ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[10.5] = "Carambola"; // !!!!!!!!!!
for(let idx in fruits) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
}
array_for_loop_ex2_test(); // Call the function.
Output:
Element at index 0 is Acerola
Element at index 1 is Apple
Element at index 2 is Banana
Element at index 6 is Breadfruit
Element at index 10.5 is Carambola
Other Examples:
Example: An array containing elements of string type, converts all its elements to uppercase.
array_for_loop_ex3.ts
function array_for_loop_ex3_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
for(let idx in fruits) {
let v = fruits[idx];
if(v) {
fruits[idx] = v.toUpperCase();
}
}
// Print elements:
for(let idx in fruits) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
}
array_for_loop_ex3_test(); // Call the function.
Output:
Element at index 0 is ACEROLA
Element at index 1 is APPLE
Element at index 2 is BANANA
5. Read-only Array
Use the readonly keyword in an array declaration to create a read-only array. This means that you cannot add, remove, or update array elements.
- Note: The readonly keyword is only allowed in the Type[] syntax, not in the Array<Type> syntax.
let okArray1 : readonly string[] = ["Acerola", "Apple", "Banana" ]; // OK
let okArray2 : readonly string[];
okArray2 = ["Acerola", "Apple", "Banana" ]; // OK
let errorArray1 : readonly Array<String>; // Compile Error!!!
Without using the readonly keyword, you can also declare a read-only array with the ReadonlyArray<T> interface:
let okArray3 : ReadonlyArray<String>; // OK
Example:
let fruits: readonly string[] = ["Acerola", "Apple", "Banana" ]; // OK
fruits[1] = "Breadfruit"; // Compile Error!!!!!!
let years: ReadonlyArray<number> = [2001, 2010, 2020 ]; // OK
years[1] = 2021; // Compile Error!!!!!!
6. Array methods
In TypeScript, an array is an object of the Array<T> interface, so it has methods defined in this interface.
interface Array<T> {
length: number;
toString(): string;
toLocaleString(): string;
pop(): T | undefined;
push(...items: T[]): number;
concat(...items: ConcatArray<T>[]): T[];
concat(...items: (T | ConcatArray<T>)[]): T[];
join(separator?: string): string;
reverse(): T[];
shift(): T | undefined;
slice(start?: number, end?: number): T[];
sort(compareFn?: (a: T, b: T) => number): this;
splice(start: number, deleteCount?: number): T[];
splice(start: number, deleteCount: number, ...items: T[]): T[];
unshift(...items: T[]): number;
indexOf(searchElement: T, fromIndex?: number): number;
lastIndexOf(searchElement: T, fromIndex?: number): number;
every<S extends T>(predicate: (value: T, index: number, array: T[]) => value is S, thisArg?: any): this is S[];
every(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): boolean;
some(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): boolean;
forEach(callbackfn: (value: T, index: number, array: T[]) => void, thisArg?: any): void;
map<U>(callbackfn: (value: T, index: number, array: T[]) => U, thisArg?: any): U[];
filter<S extends T>(predicate: (value: T, index: number, array: T[]) => value is S, thisArg?: any): S[];
filter(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): T[];
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T): T;
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue: T): T;
reduce<U>(callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: T[]) => U, initialValue: U): U;
reduceRight(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T): T;
reduceRight(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue: T): T;
reduceRight<U>(callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: T[]) => U, initialValue: U): U;
[n: number]: T;
}
7. forEach(..)
forEach(callbackfn: (value: T, index: number, array: T[]) => void, thisArg?: any): void;
Performs the specified action for each element in an array.
Example:
array_forEach_ex1.ts
function array_forEach_ex1_test() {
let fruits: string[] = ["Acerola", "Apple", "Banana" ]; // OK
// A callback function.
var callback = function(value:string, index:number, thisArray:string[]) {
if(index % 2 == 0) {
console.log(value);
} else {
console.log(value.toUpperCase());
}
}
fruits.forEach(callback);
}
array_forEach_ex1_test(); // Call the function.
Output:
Acerola
APPLE
Banana
8. every(..)
every(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): boolean;
Checks if all elements of an array pass the test of a specified function.
predicate: A function to check each element of the array.
Example: An array consists of years. Check if all years are greater than 1990.
array_every_ex1.ts
function array_every_ex1_test() {
let years: number[] = [2001, 1995, 2007, 2010, 2020];
// Arrow function.
var predicate = (value: number, index: number, thisArray: number[]) => {
return value > 1990;
}
var ok: boolean = years.every(predicate);
console.log(`All years > 1990? ${ok}`); // true
}
array_every_ex1_test(); // Call the function.
9. sort(..)
sort(compareFn?: (a: T, b: T) => number): this;
Sorts this array based on the specified function and returns this array. If a function is not provided, the elements of the array will be sorted according to natural rules.
Example: Sorting a string array:
array_sort_ex1.ts
function array_sort_ex1_test() {
let fruits: string[] = ["Banana", "Acerola", "Apple", "Carambola", "Breadfruit"];
// Compare Function:
var compareFn = (a: string, b: string) => {
// v > 0 --> a > b
// v = 0 --> a == b
// v < 0 --> a < b
let v: number = a.localeCompare(b);
return v;
}
fruits.sort(compareFn);
console.log("--- after sorting --- ");
console.log(fruits);
}
array_sort_ex1_test(); // Call the function.
Output:
--- after sorting ---
[ 'Acerola', 'Apple', 'Banana', 'Breadfruit', 'Carambola' ]
10. filter(..)
filter(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): T[];
filter<S extends T>(predicate: (value: T, index: number, array: T[]) => value is S, thisArg?: any): S[];
Returns a new array of elements that pass the test of a specified function.
Example: An array consists of years, filter out a new array consisting of only even years.
array_filter_ex1.ts
function array_filter_ex1_test() {
let years: number[] = [2001, 1995, 2007, 2010, 2020];
// Filter even value.
var predicate = (value: number, index: number, thisArray: number[]) => {
return value % 2 == 0;
}
var evenYears: number[] = years.filter(predicate);
console.log(" --- Even Years: --- ");
console.log(evenYears);
}
array_filter_ex1_test(); // Call the function.
Output:
--- Even Years: ---
[ 2010, 2020 ]
TypeScript Tutorials
- Run your first TypeScript example in Visual Studio Code
- TypeScript Namespaces Tutorial with Examples
- TypeScript Modules tutorial with Examples
- TypeScript typeof operator
- TypeScript Loops Tutorial with Examples
- Install TypeScript on Windows
- TypeScript Functions Tutorial with Examples
- TypeScript Tuples Tutorial with Examples
- TypeScript Interfaces Tutorial with Examples
- TypeScript Arrays Tutorial with Examples
- TypeScript instanceof operator
- TypeScript Methods Tutorial with Examples
- TypeScript Closures Tutorial with Examples
- TypeScript Constructors Tutorial with Examples
- TypeScript Properties Tutorial with Examples
- Parsing JSON in TypeScript
- Parsing JSON in TypeScript with the json2typescript library
- What is Transpiler?
Show More