Java ChronoUnit Tutorial with Examples
1. ChronoUnit
ChronoUnit is an enum that implements the TemporalUnit interface, which provides the standard units used in the Java Date Time API. Basically these units are enough to use. However, if you want a custom unit, write a class that implements the TemporalUnit interface.
public enum ChronoUnit implements TemporalUnit
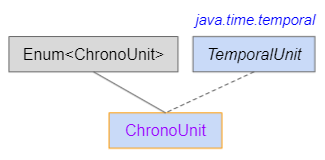
- TemporalField
- TemporalUnit
- ChronoField
These units are intended to be applicable in multiple calendar systems. For example, most "non-ISO" calendar systems define units of years, months and days, just with slightly different rules. The documentation of each unit explains how it operates.
2. ChronoUnit Values
Enum | Display Name | Estimated Duration |
NANOS | Nanos | Duration.ofNanos(1) |
MICROS | Micros | Duration.ofNanos(1000) |
MILLIS | Millis | Duration.ofNanos(1000_000) |
SECONDS | Seconds | Duration.ofSeconds(1) |
MINUTES | Minutes | Duration.ofSeconds(60) |
HOURS | Hours | Duration.ofSeconds(3600) |
HALF_DAYS | HalfDays | Duration.ofSeconds(43200) |
DAYS | Days | Duration.ofSeconds(86400) |
WEEKS | Weeks | Duration.ofSeconds(7 * 86400L) |
MONTHS | Months | Duration.ofSeconds(31556952L / 12) |
YEARS | Years | Duration.ofSeconds(31556952L) |
DECADES | Decades | Duration.ofSeconds(31556952L * 10L) |
CENTURIES | Centuries | Duration.ofSeconds(31556952L * 100L) |
MILLENNIA | Millennia | Duration.ofSeconds(31556952L * 1000L) |
ERAS | Eras | Duration.ofSeconds(31556952L * 1000_000_000L) |
FOREVER | Forever | Duration.ofSeconds(Long.MAX_VALUE, 999_999_999) |
3. ChronoUnit methods
public Duration getDuration()
public boolean isDurationEstimated()
public boolean isDateBased()
public boolean isTimeBased()
public boolean isSupportedBy(Temporal temporal)
public <R extends Temporal> R addTo(R temporal, long amount)
public long between(Temporal temporal1Inclusive, Temporal temporal2Exclusive)
4. CENTURIES
Unit that represents the concept of a century. For the ISO calendar system, it is equal to 100 years.
The above is also true for the Hijrah, Japanese, Minguo and ThaiBuddhist calendar systems.
- HijrahDate
- MinguoDate
- JapaneseDate
- ThaiBuddhistDate
When used with other calendar systems it must correspond to an integral number of days and is normally an integral number of years.
5. DAYS
Unit that represents the concept of a day. For the ISO calendar system, it is the standard day from midnight to midnight. The estimated duration of a day is 24 Hours.
The above is also true for the Hijrah, Japanese, Minguo and ThaiBuddhist calendar systems.
When used with other calendar systems it must correspond to the day defined by the rising and setting of the Sun on Earth. It is not required that days begin at midnight - when converting between calendar systems, they should be intersected at midday.
Example: Find the number of days between 2 periods of time.
ChronoUnit_DAYS_ex1.java
LocalDateTime localDateTime1 = LocalDateTime.of(2020, 3, 15, 0, 30, 45);
LocalDateTime localDateTime2 = LocalDateTime.of(2020, 3, 17, 13, 30, 45);
Duration duration = Duration.between(localDateTime1, localDateTime2);
System.out.println("duration: " + duration); // PT61H (61 Hours)
long days = ChronoUnit.DAYS.between(localDateTime1, localDateTime2);
System.out.println("days: " + days); // 2
Example: Add 2 days.
ChronoUnit_DAYS_ex2.java
ZonedDateTime now = ZonedDateTime.now();
// Plus 2 days
ZonedDateTime after2Days = now.plus(2, ChronoUnit.DAYS);
System.out.println("Now is: " + now);
System.out.println("After 2 days: " + after2Days);
Output:
Now is: 2021-07-17T00:50:13.048263+06:00[Asia/Bishkek]
After 2 days: 2021-07-19T00:50:13.048263+06:00[Asia/Bishkek]
6. DECADES
Unit that represents the concept of a decade. For the ISO calendar system, it is equal to 10 years.
The above is also true for the Hijrah, Japanese, Minguo and ThaiBuddhist calendar systems.
- HijrahDate
- MinguoDate
- JapaneseDate
- ThaiBuddhistDate
When used with other calendar systems it must correspond to an integral number of days and is normally an integral number of years.
7. ERAS
Unit that represents the concept of an era.
The ISO calendar system has no concept of era, therefore it does not appear in strings with date and time formats. The artificially estimated time of the era in this system is 1 billion years.
Calendar system | Date Time String |
ISO | 1989-02-08 |
Japanese Imperial Calendar | Japanese Heisei 1-02-08 |
Era is associated with the period of reign of a king or the duration of a dynasty. The concept of an era is most evident in the Japanese imperial calendar system.
- Era
- JapaneseEra
- JapaneseDate
- IsoEra
When used with other calendar systems, there is no restriction on its value.
ChronoUnit_ERAS_ex1.java
LocalDate isoDate = LocalDate.of(2020, 3, 15);
System.out.printf("isoDate: %s%n%n", isoDate); // 2020-03-15
JapaneseDate japaneseDate = JapaneseDate.from(isoDate);
JapaneseEra era = japaneseDate.getEra();
System.out.printf("japaneseDate: %s%n", japaneseDate); // Japanese Reiwa 2-03-15
System.out.printf(" > era: %s", era); // Reiwa
Output:
isoDate: 2020-03-15
japaneseDate: Japanese Reiwa 2-03-15
> era: Reiwa
8. FOREVER
Artificial unit that represents the concept of forever. This is primarily used with TemporalField to represent unbounded fields such as the year or era. The estimated duration of this unit is artificially defined as the largest duration supported by Duration.
Duration maxDuration = ChronoUnit.FOREVER.getDuration();
Example:
ChronoUnit_FOREVER_ex1.java
for (final ChronoUnit unit : ChronoUnit.values()) {
final Duration duration = unit.getDuration();
System.out.println(unit + ": " + duration + " (" + duration.getSeconds() + " seconds)");
}
Output:
Nanos: PT0.000000001S (0 seconds)
Micros: PT0.000001S (0 seconds)
Millis: PT0.001S (0 seconds)
Seconds: PT1S (1 seconds)
Minutes: PT1M (60 seconds)
Hours: PT1H (3600 seconds)
HalfDays: PT12H (43200 seconds)
Days: PT24H (86400 seconds)
Weeks: PT168H (604800 seconds)
Months: PT730H29M6S (2629746 seconds)
Years: PT8765H49M12S (31556952 seconds)
Decades: PT87658H12M (315569520 seconds)
Centuries: PT876582H (3155695200 seconds)
Millennia: PT8765820H (31556952000 seconds)
Eras: PT8765820000000H (31556952000000000 seconds)
Forever: PT2562047788015215H30M7.999999999S (9223372036854775807 seconds)
- Duration
- TemporalField
9. HALF_DAYS
Unit that represents the concept of half a day, as used in AM/PM. For the ISO calendar system, it is equal to 12 hours.
Example: Truncate the remainder when divided by half a day from a LocalDateTime.
ChronoUnit_HALF_DAYS_ex1.java
LocalDateTime localDateTime1 = LocalDateTime.of(2020, 3, 15, 9, 30, 45);
LocalDateTime truncatedLDT1 = localDateTime1.truncatedTo(ChronoUnit.HALF_DAYS);
System.out.printf("localDateTime1: %s%n", localDateTime1); // 2020-03-15T09:30:45
System.out.printf(" > truncated: %s%n%n", truncatedLDT1); // 2020-03-15T00:00
LocalDateTime localDateTime2 = LocalDateTime.of(2020, 3, 15, 13, 30, 45);
LocalDateTime truncatedLDT2 = localDateTime2.truncatedTo(ChronoUnit.HALF_DAYS);
System.out.printf("localDateTime2: %s%n", localDateTime2); // 2020-03-15T13:30:45
System.out.printf(" > truncated: %s", truncatedLDT2); // 2020-03-15T12:00
Output:
localDateTime1: 2020-03-15T09:30:45
> truncated: 2020-03-15T00:00
localDateTime2: 2020-03-15T13:30:45
> truncated: 2020-03-15T12:00
10. HOURS
Unit that represents the concept of an hour. For the ISO calendar system, it is equal to 60 minutes.
Example:
ChronoUnit_HOURS_ex1.java
ZonedDateTime parisNow = ZonedDateTime.now(ZoneId.of("Europe/Paris"));
System.out.println("Now in Paris: " + parisNow);
ZonedDateTime parisNowHour = parisNow.truncatedTo(ChronoUnit.HOURS);
System.out.println("Truncated to Hour: " + parisNowHour);
Output:
Now in Paris: 2021-07-17T05:40:15.939515+02:00[Europe/Paris]
Truncated to Hour: 2021-07-17T05:00+02:00[Europe/Paris]
11. MICROS
Unit that represents the concept of a microsecond. For the ISO calendar system, 1 second equals 1 million microseconds.
12. MILLENNIA
Unit that represents the concept of a millennium. For the ISO calendar system, it is equal to 1000 years.
The above is also true for the Hijrah, Japanese, Minguo and ThaiBuddhist calendar systems.
When used with other calendar systems it must correspond to an integral number of days and is normally an integral number of years.
- ThaiBuddhistDate
- JapaneseDate
- MinguoDate
- HijrahDate
13. MILLIS
Unit that represents the concept of a millisecond. For the ISO calendar system, 1 second equals 1000 milliseconds.
14. MINUTES
Unit that represents the concept of a minute. For the ISO calendar system, it is equal to 60 seconds.
15. MONTHS
Unit that represents the concept of a month. For the ISO calendar system, the length of the month varies by month-of-year. The estimated duration of a month is one twelfth of 365.2425 Days.
When used with other calendar systems it must correspond to an integral number of days.
16. NANOS
Unit that represents the concept of a nanosecond, the smallest supported unit of time. For the ISO calendar system, 1 second equals 1 billion nanoseconds.
17. SECONDS
Unit that represents the concept of a second. For the ISO calendar system, it is equal to the second in the SI system of units, except around a leap-second.
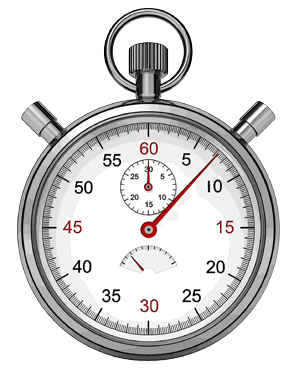
18. WEEKS
Unit that represents the concept of a week. For the ISO calendar system, it is equal to 7 days.
The above is also true for the Hijrah, Japanese, Minguo and ThaiBuddhist calendar systems.
- HijrahDate
- MinguoDate
- JapaneseDate
- ThaiBuddhistDate
When used with other calendar systems it must correspond to an integral number of days.
19. YEARS
Unit that represents the concept of a year. For the ISO calendar system, it is equal to 12 months. The estimated duration of a year is 365.2425 Days.
A year in the Hijrah, Japanese, Minguo and ThaiBuddhist calendar systems is also equal to 12 months.
- HijrahDate
- MinguoDate
- JapaneseDate
- ThaiBuddhistDate
When used with other calendar systems it must correspond to an integral number of days or months roughly equal to a year defined by the passage of the Earth around the Sun.
20. getDuration()
Returns the estimated duration of this unit in the ISO calendar system.
public Duration getDuration()
All of the units in this class have an estimated duration. Days vary due to "Daylight Saving Time" (DST), while months have different lengths.
Example: A month is estimated to be 30 days, but in hours it is estimated to be 730 (30.41 days).
ChronoUnit_getDuration_ex1.java
Duration monthDuration = ChronoUnit.MONTHS.getDuration();
// to days: 30 days
System.out.println("monthDuration.toDays(): " + monthDuration.toDays());
// to hours: 730 hours ~ 30.41 days.
System.out.println("monthDuration.toHours(): " + monthDuration.toHours());
Output:
monthDuration.toDays(): 30
monthDuration.toHours(): 730
Example: Estimate the number of hours in "1 year 2 months 15 days".
ChronoUnit_getDuration_ex2.java
Period period = Period.of(1, 2, 15); // 1 year 2 months 15 days.
int years = period.getYears(); // 1
int months = period.getMonths(); // 2
int days = period.getDays(); // 15
System.out.println("years: " + years); // 1
System.out.println("months: " + months);// 2
System.out.println("days: " + days); // 15
Duration yearsDuration = ChronoUnit.YEARS.getDuration().multipliedBy(years);
Duration monthsDuration = ChronoUnit.MONTHS.getDuration().multipliedBy(months);
Duration daysDuration = ChronoUnit.DAYS.getDuration().multipliedBy(days);
Duration totalDuration = yearsDuration.plus(monthsDuration).plus(daysDuration);
long estimatedDays = totalDuration.toDays();
long estimatedHours = totalDuration.toHours();
System.out.println("Estimated days: " + estimatedDays); // 441 days
System.out.println("Estimated hours: " + estimatedHours); // 10586 hours ~ 441.08 days.
Output:
years: 1
months: 2
days: 15
Estimated days: 441
Estimated hours: 10586
21. isDurationEstimated()
Checks if the duration of the unit is an estimate.
public boolean isDurationEstimated()
All time units in this class are considered to be accurate, while all date units in this class are considered to be estimated. See more isTimeBased() and isDateBased() methods.
This definition ignores leap seconds, but considers that Days vary due to daylight saving time and months have different lengths.
Example: Print a list of accuracy units of this class.
ChronoUnit_isDurationEstimated_ex1.java
for (ChronoUnit unit : ChronoUnit.values()) {
if (unit.isDurationEstimated()) {
System.out.println(unit.name());
}
}
Output:
DAYS
WEEKS
MONTHS
YEARS
DECADES
CENTURIES
MILLENNIA
ERAS
FOREVER
22. isDateBased()
Check if this unit is date based?
public boolean isDateBased()
All units from DAYS to ERAS return true. Time-based units and FOREVER return false.
ChronoUnit_isDateBased_ex1.java
for (ChronoUnit unit : ChronoUnit.values()) {
if (unit.isDateBased()) {
System.out.println(unit.name());
}
}
Output:
DAYS
WEEKS
MONTHS
YEARS
DECADES
CENTURIES
MILLENNIA
ERAS
23. isTimeBased()
Check if this unit is time based?
public boolean isTimeBased()
All units from NANOS to HALF_DAYS return true. Date-based units and FOREVER return false.
ChronoUnit_isTimeBased_ex1.java
for (ChronoUnit unit : ChronoUnit.values()) {
if (unit.isTimeBased()) {
System.out.println(unit.name());
}
}
Output:
NANOS
MICROS
MILLIS
SECONDS
MINUTES
HOURS
HALF_DAYS
24. isSupportedBy(Temporal)
Checks if this unit is supported by the specified Temporal object.
public boolean isSupportedBy(Temporal temporal)
Example: Find units supported by LocalDate:
ChronoUnit_isSupportedBy_ex1.java
Temporal localDate = LocalDate.now();
System.out.println("Does LocalDate support: ");
for(ChronoUnit unit: ChronoUnit.values()) {
System.out.println(unit.name() + "? " + unit.isSupportedBy(localDate));
}
Output:
Does LocalDate support:
NANOS? false
MICROS? false
MILLIS? false
SECONDS? false
MINUTES? false
HOURS? false
HALF_DAYS? false
DAYS? true
WEEKS? true
MONTHS? true
YEARS? true
DECADES? true
CENTURIES? true
MILLENNIA? true
ERAS? true
FOREVER? false
25. addTo(R temporal, long amount)
Returns a copy of the specified Temporal object with the specified period added.
public <R extends Temporal> R addTo(R temporal, long amount)
This method is equivalent to the Temporal.plus(long,TemporalUnit) method. This approach is recommended.
// Defined in Temporal interface
public Temporal plus(long amountToAdd, TemporalUnit unit)
Example:
ChronoUnit_addTo_ex1.java
Temporal ym = YearMonth.of(2020, 3); // 2020-03
// Add 10 monthds to ym.
YearMonth ym2 = (YearMonth) ChronoUnit.MONTHS.addTo(ym, 10);
System.out.println("ym2: " + ym2); // 2021-01
- Temporal
- YearMonth
26. between(Temporal, Temporal)
Returns the amount of time between two Temporal objects. This unit needs to be supported by both Temporal objects, otherwise an exception will be thrown.
public long between(Temporal temporal1Inclusive, Temporal temporal2Exclusive)
This method is equivalent to using Temporal.until(Temporal,TemporalUnit) method.
// Defined in Temporal interface
public long until(Temporal endExclusive, TemporalUnit unit)
Example:
ChronoUnit_between_ex1.java
LocalDate from = LocalDate.of(2020, 3, 15);
LocalDate to = LocalDate.of(2020, 3, 17);
long days = ChronoUnit.DAYS.between(from, to);
System.out.println("days: " + days); // 2
Example:
ChronoUnit_between_ex2.java
YearMonth from = YearMonth.of(2020, 7);
LocalDateTime to = LocalDateTime.of(2021, 3, 17, 13, 45, 30);
long months = ChronoUnit.MONTHS.between(from, to);
System.out.println("months: " + months); // 8
- Temporal
- LocalDateTime
- YearMonth
Java Date Time Tutorials
- Java ZoneId Tutorial with Examples
- Java Temporal Tutorial with Examples
- Java Period Tutorial with Examples
- Java TemporalAdjusters Tutorial with Examples
- Java MinguoDate Tutorial with Examples
- Java TemporalAccessor Tutorial with Examples
- Java JapaneseEra Tutorial with Examples
- Java HijrahDate Tutorial with Examples
- Java Date Time Tutorial with Examples
- What is Daylight Saving Time (DST)?
- Java LocalDate Tutorial with Examples
- Java LocalTime Tutorial with Examples
- Java LocalDateTime Tutorial with Examples
- Java ZonedDateTime Tutorial with Examples
- Java JapaneseDate Tutorial with Examples
- Java Duration Tutorial with Examples
- Java TemporalQuery Tutorial with Examples
- Java TemporalAdjuster Tutorial with Examples
- Java ChronoUnit Tutorial with Examples
- Java TemporalQueries Tutorial with Examples
Show More