Java Period Tutorial with Examples
1. Period
The Period class uses year, month, and day units to represent a period of time. For example "3 years 2 months 15 days". If you want an amount of time based on hours, minutes and seconds, use the Duration class.
The year, month and day values in the Period are independent, which means if you add or subtract some days from the Period, it will not affect the year and month. The reason for this is the length of the month and year is not fixed.
public final class Period implements ChronoPeriod, Serializable
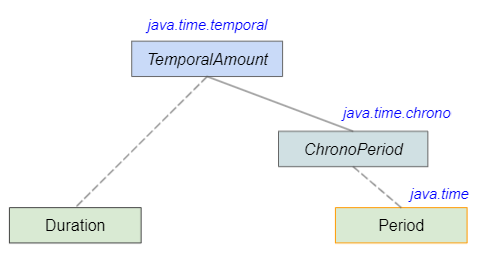
- ChronoPeriod
- Duration
- TemporalAmount
Example: Football player Cristiano Ronaldo was born on February 5th, 1985. On September 29th, 2002, Ronaldo made his debut in Primeira Liga, so he was 17 years old, 7 months 24 days at that time.
Period_ronaldo_ex1.java
// 1985-02-05 (Ronaldo's birthday).
LocalDate startInclusiveDate = LocalDate.of(1985, 2, 5);
// 2002-09-29 (First time playing in Primeira Liga ).
LocalDate endExclusiveDate = LocalDate.of(2002, 9, 29);
Period period = Period.between(startInclusiveDate, endExclusiveDate);
System.out.println(period); // P17Y7M24D
System.out.println("Years: " + period.getYears()); // 17
System.out.println("Months: " + period.getMonths()); // 7
System.out.println("Days: " + period.getDays()); // 24
2. Static Factory Methods
Static factory methods:
public static final Period ZERO = new Period(0, 0, 0);
public static Period ofYears(int years)
public static Period ofMonths(int months)
public static Period ofWeeks(int weeks)
public static Period ofDays(int days)
public static Period of(int years, int months, int days)
public static Period from(TemporalAmount amount)
public static Period parse(CharSequence text)
public static Period between(LocalDate startDateInclusive, LocalDate endDateExclusive)
3. Other methods
Other methods:
// -----------------------------------------------------------------
// getX()
// -----------------------------------------------------------------
// Inherited from ChronoPeriod interface
public long get(TemporalUnit unit)
// Inherited from ChronoPeriod interface
public List<TemporalUnit> getUnits()
// Inherited from ChronoPeriod interface
public IsoChronology getChronology()
public int getYears()
public int getMonths()
public int getDays()
// -----------------------------------------------------------------
// withX()
// -----------------------------------------------------------------
public Period withYears(int years)
public Period withMonths(int months)
public Period withDays(int days)
// -----------------------------------------------------------------
// plusX()
// -----------------------------------------------------------------
// Inherited from ChronoPeriod interface
public Period plus(TemporalAmount amountToAdd)
public Period plusYears(long yearsToAdd)
public Period plusMonths(long monthsToAdd)
public Period plusDays(long daysToAdd)
// -----------------------------------------------------------------
// minusX()
// -----------------------------------------------------------------
// Inherited from ChronoPeriod interface
public Period minus(TemporalAmount amountToSubtract)
public Period minusYears(long yearsToSubtract)
public Period minusMonths(long monthsToSubtract)
public Period minusDays(long daysToSubtract)
// -----------------------------------------------------------------
public long toTotalMonths()
// -----------------------------------------------------------------
// Other method inherited from ChronoPeriod interface
// -----------------------------------------------------------------
public boolean isZero()
public boolean isNegative()
public Period multipliedBy(int scalar)
public Period negated()
public Period normalized()
public Temporal addTo(Temporal temporal)
public Temporal subtractFrom(Temporal temporal)
4. get(TemporalUnit)
Return the value of the specified unit.
// Inherited from ChronoPeriod interface
public long get(TemporalUnit unit) {
Only the following three standard units are supported, the others will throw an UnsupportedTemporalTypeException.
- ChronoUnit.YEARS
- ChronoUnit.MONTHS
- ChronoUnit.DAYS
Example:
Period_get_unit_ex1.java
// 20 years 5 months 115 days.
Period period = Period.of(20, 5, 115);
long years = period.get(ChronoUnit.YEARS); // 20
long months = period.get(ChronoUnit.MONTHS); // 5
long days = period.get(ChronoUnit.DAYS); // 115
System.out.printf("%d years, %d months %d days", years, months, days);
5. getX() *
The getDays() method returns the number of days represented in this Period. The day, month, and year values in Period are independent of each other, they cannot be converted to each other because the length of month and year is not fixed.
The getMonths(), getYears() methods are also understood as their names.
public int getYears()
public int getMonths()
public int getDays()
Example: July 20th, 1969, the Apollo 11 spacecraft landed on the moon. We can calculate how long ago this event took place compared to the present:
Period_getX_ex1.java
// 1969-07-20
LocalDate apollo11Date = LocalDate.of(1969, 7, 20);
// Now
LocalDate now = LocalDate.now();
System.out.println("Now is " + now);
Period period = Period.between(apollo11Date, now);
System.out.println(period);
System.out.println("Years: " + period.getYears());
System.out.println("Months: " + period.getMonths());
System.out.println("Days: " + period.getDays());
Output:
Now is 2021-06-25
P51Y11M5D
Years: 51
Months: 11
Days: 5
Actually, to calculate the number of years, months, weeks or days between 2 different times, you can also use the ChronoUnit class. See for example:
ChronoUnit_between_ex1.java
// 1969-07-20
LocalDate apollo11Date = LocalDate.of(1969, 7, 20);
// Now
LocalDate now = LocalDate.now();
System.out.println("Now is " + now);
long years = ChronoUnit.YEARS.between(apollo11Date, now);
long months = ChronoUnit.MONTHS.between(apollo11Date, now);
long weeks = ChronoUnit.WEEKS.between(apollo11Date, now);
long days = ChronoUnit.DAYS.between(apollo11Date, now);
System.out.println("Years: " + years);
System.out.println("Months: " + months);
System.out.println("Weeks: " + weeks);
System.out.println("Days:" + days);
Output:
Now is 2021-06-25
Years: 51
Months: 623
Weeks: 2709
Days:18968
6. getUnits()
Return the TemporalUnit(s) supported by this Period. Only the following 3 standard TemporalUnit(s) are supported:
- ChronoUnit.YEARS
- ChronoUnit.MONTHS
- ChronoUnit.DAYS
// Inherited from ChronoPeriod interface
public List<TemporalUnit> getUnits()
Example:
Period_getUnits_ex1.java
Period period = Period.of(2, 8, 0);
// ChronoUnit.YEARS, ChronoUnit.MONTHS, ChronoUnit.DAYS
List<TemporalUnit> units = period.getUnits();
for(TemporalUnit unit: units) {
System.out.println("Unit: " + unit.getClass().getName() + " - " + unit);
}
Output:
Unit: java.time.temporal.ChronoUnit - Years
Unit: java.time.temporal.ChronoUnit - Months
Unit: java.time.temporal.ChronoUnit - Days
7. getChronology()
Return the chronology of this Period, which is the ISO calendar system (IsoChronology.INSTANCE).
// Inherited from ChronoPeriod interface
public IsoChronology getChronology()
Example:
Period_getChronology_ex1.java
Period period = Period.of(20, 5, 10);
IsoChronology isoChronology = period.getChronology();
System.out.println("IsoChronology: " + isoChronology.getId()); // ISO.
- Java IsoChronology
8. withX(..) *
The withDays(days) method returns a copy of this Period with the number of days replaced by the specified number of days. The year and month are not affected by this method.
The withYears(years), withMonths(months) methods are also understood as their names.
public Period withYears(int years)
public Period withMonths(int months)
public Period withDays(int days)
Example:
Period_withX_ex1.java
Period period = Period.of(20, 3, 15);
period = period.withDays(100);
System.out.println(period);
System.out.println("Years: " + period.getYears()); // 20
System.out.println("Months: " + period.getMonths()); // 3
System.out.println("Days: " + period.getDays()); // 100
9. plus(TemporalAmount)
Return a copy of this Period with an amount of time added.
public Period plus(TemporalAmount amountToAdd)
The day, month, and year values are independent of each other, no normalization will be performed. Such as:
- "2 years 10 months 110 days" + "3 years 5 months 10 days" --> "5 years 15 months 120 days".
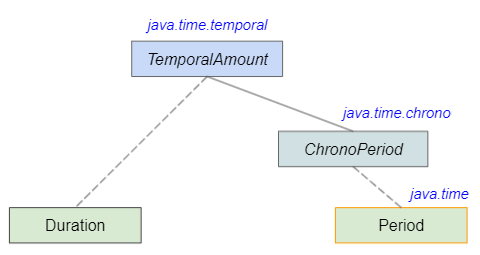
Example:
Period_plus_amount_ex1.java
// 20 years 3 months 15 days.
Period period = Period.of(20, 3, 15);
System.out.println("period: " + period); // P20Y3M15D
TemporalAmount amount = Period.ofMonths(15); // 15 months
Period period2 = period.plus(amount);
System.out.println("period2: " + period2); // P20Y18M15D (20 years, 18 months, 15 days)
10. plusX(..) *
The plusDays(daysToAdd) method returns a copy of this Period with the specified number of days added, which does not affect the year and month values.
The plusYears(yearsToAdd) and plusMonths(monthsToAdd) methods are also understood as their names.
public Period plusYears(long yearsToAdd)
public Period plusMonths(long monthsToAdd)
public Period plusDays(long daysToAdd)
Example:
Period_plusX_ex1.java
Period period = Period.of(20, 3, 15);
// period1
Period period1 = period.plusDays(100);
System.out.println("period1.getYears(): " + period1.getYears()); // 20
System.out.println("period1.getMonths(): " + period1.getMonths()); // 3
System.out.println("period1.getDays(): " + period1.getDays()); // 115
System.out.println(" ------- \n");
// period2
Period period2 = period.plusDays(-100);
System.out.println("period2.getYears(): " + period2.getYears()); // 20
System.out.println("period2.getMonths(): " + period2.getMonths()); // 3
System.out.println("period2.getDays(): " + period2.getDays()); // -85
System.out.println(" ------- \n");
// period3
Period period3 = period.plusYears(-30);
System.out.println("period3.getYears(): " + period3.getYears()); // -10
System.out.println("period3.getMonths(): " + period3.getMonths()); // 3
System.out.println("period3.getDays(): " + period3.getDays()); // 15
Output:
period1.getYears(): 20
period1.getMonths(): 3
period1.getDays(): 115
-------
period2.getYears(): 20
period2.getMonths(): 3
period2.getDays(): -85
-------
period3.getYears(): -10
period3.getMonths(): 3
period3.getDays(): 15
11. minus(TemporalAmount)
Return a copy of this Period with the specified amount of time subtracted.
public Period minus(TemporalAmount amountToSubtract)
The day, month, and year values are independent of each other, no normalization will be performed. Such as:
- "2 years 10 months 110 days" - "3 years 5 months 10 days" --> "-1 years 10 months 90 days".
Example:
Period_minus_amount_ex1.java
// 20 years 3 months 15 days.
Period period = Period.of(20, 3, 15);
System.out.println("period: " + period); // P20Y3M15D
TemporalAmount amount = Period.ofMonths(5); // 5 months
Period period2 = period.minus(amount);
System.out.println("period2: " + period2); // P20Y-2M15D (20 years, -2 months, 15 days)
- Java TemporalAmount
12. minusX(..) *
The minusDays(daysToSubtract) method returns a copy of this Period with the specified number of days subtracted, which does not affect the other two fields, year and month.
The methods minusYears(yearsToSubtract) and minusMonths(monthsToSubtract) are also understood as their names.
public Period minusYears(long yearsToSubtract)
public Period minusMonths(long monthsToSubtract)
public Period minusDays(long daysToSubtract)
Example:
Period_minusX_ex1.java
Period period = Period.of(20, 3, 15);
// period1
Period period1 = period.minusDays(100);
System.out.println("period1.getYears(): " + period1.getYears()); // 20
System.out.println("period1.getMonths(): " + period1.getMonths()); // 3
System.out.println("period1.getDays(): " + period1.getDays()); // -85
System.out.println(" ------- \n");
// period2
Period period2 = period.minusDays(-100);
System.out.println("period2.getYears(): " + period2.getYears()); // 20
System.out.println("period2.getMonths(): " + period2.getMonths()); // 3
System.out.println("period2.getDays(): " + period2.getDays()); // 115
System.out.println(" ------- \n");
// period3
Period period3 = period.minusYears(30);
System.out.println("period3.getYears(): " + period3.getYears()); // -10
System.out.println("period3.getMonths(): " + period3.getMonths()); // 3
System.out.println("period3.getDays(): " + period3.getDays()); // 15
Output:
period1.getYears(): 20
period1.getMonths(): 3
period1.getDays(): -85
-------
period2.getYears(): 20
period2.getMonths(): 3
period2.getDays(): 115
-------
period3.getYears(): -10
period3.getMonths(): 3
period3.getDays(): 15
13. isZero()
Return true if all 3 values of day, month and year in this Period are 0, otherwise return false.
// Inherited from ChronoPeriod interface
public boolean isZero()
Example:
Period_isZero_ex1.java
Period period1 = Period.of(0, -1, 30);
System.out.println("period1.isZero()? " + period1.isZero()); // false
Period period2 = Period.of(-1, 12, 0);
System.out.println("period2.isZero()? " + period2.isZero()); // false
Period period3 = Period.of(0, 0, 0);
System.out.println("period3.isZero()? " + period3.isZero()); // true
14. isNegative()
Return true if any day, month or year of this Period is negative, otherwise return false.
// Inherited from ChronoPeriod interface
public boolean isNegative()
Example:
Period_isNegative_ex1.java
Period period1 = Period.of(10, -1, 30);
System.out.println("period1.isNegative()? " + period1.isNegative()); // true
Period period2 = Period.of(1, -12, -15);
System.out.println("period2.isNegative()? " + period2.isNegative()); // true
Period period3 = Period.of(0, 0, 0);
System.out.println("period3.isNegative()? " + period3.isNegative()); // false
Period period4 = Period.of(5, 10, 15);
System.out.println("period4.isNegative()? " + period4.isNegative()); // false
15. multipliedBy(int)
Multiply this Period by a specified value and return a new Period.
// Inherited from ChronoPeriod interface
public Period multipliedBy(int scalar)
The day, month, and year values are independent of each other, no normalization will be performed.
Example:
Period_multipliedBy_ex1.java
// 20 years 10 months 35 days.
Period period = Period.of(20, 10, 35);
Period result = period.multipliedBy(2);
long years = result.get(ChronoUnit.YEARS); // 40
long months = result.get(ChronoUnit.MONTHS); // 20
long days = result.get(ChronoUnit.DAYS); // 70
System.out.printf("%d years, %d months %d days", years, months, days);
16. negated()
// Inherited from ChronoPeriod interface
public Period negated()
Example:
Period_negated_ex1.java
// 20 years 3 months 15 days.
Period period = Period.of(20, 3, 15);
System.out.println("period: " + period); // P20Y3M15D
// period2 = 0 - period1
Period period2 = period.negated();
System.out.println("period2: " + period2); // P-20Y-3M-15D (-20 Years, -3 months, -15 days)
17. normalized()
Return a copy of this Period with the year and month normalized. A year is considered equal to 12 months, after normalizing the value of the month will be in the range from -11 to 11.
// Inherited from ChronoPeriod interface
public Period normalized()
Example:
- "1 years -25 months 50 days" --> "-1 year -1 months 50 days".
Period_normalized_ex1.java
// 1 years -25 months 50 days.
Period period = Period.of(1, -25, 50);
Period result = period.normalized();
long years = result.get(ChronoUnit.YEARS); // -1
long months = result.get(ChronoUnit.MONTHS); // -1
long days = result.get(ChronoUnit.DAYS); // 50
System.out.printf("%d years, %d months %d days", years, months, days);
18. toTotalMonths()
Return the total months in this Period by multiplying the number of years by 12 and adding the number of months. The number of days will be ignored.
public long toTotalMonths()
Example:
Period_toTotalMonths_ex1.java
// 1 years 3 months 145 days.
Period period = Period.of(1, 3, 145);
System.out.println("period: " + period); // P1Y3M145D
long months = period.toTotalMonths();
System.out.println("period.toTotalMonths(): " + months); // 15
19. addTo(Temporal)
Add this Period to a specified Temporal object and return a new Temporal object.
// Inherited from ChronoPeriod interface
public Temporal addTo(Temporal temporal)
This method is equivalent to using the Temporal.plus(TemporalAmount) method.
// Defined in Temporal interface.
public Temporal plus(TemporalAmount amount)
Example:
Period_addTo_ex1.java
// Create Temporal object.
LocalDateTime localDateTime = LocalDateTime.of(2000, 1, 10, 23, 30, 0);
System.out.println("localDateTime: " + localDateTime); // 2000-01-10T23:30
// 1 Year 1 month 25 days.
Period period = Period.of(1, 1, 25);
LocalDateTime newLocalDateTime = (LocalDateTime) period.addTo(localDateTime);
System.out.println("newLocalDateTime: " + newLocalDateTime); // 2001-03-07T23:30
20. subtractFrom(Temporal)
Subtract this Period from a specified Temporal object and return a new Temporal object.
// Inherited from ChronoPeriod interface
public Temporal subtractFrom(Temporal temporal)
This method is equivalent to using the Temporal.minus(TemporalAmount) method.
// Defined in Temporal interface.
public Temporal minus(TemporalAmount amount)
Example:
Period_subtractFrom_ex1.java
// Create Temporal object.
LocalDateTime localDateTime = LocalDateTime.of(2000, 1, 10, 23, 30, 0);
System.out.println("localDateTime: " + localDateTime); // 2000-01-10T23:30
// 1 Year 1 month 25 days.
Period period = Period.of(1, 1, 25);
LocalDateTime newLocalDateTime = (LocalDateTime) period.subtractFrom(localDateTime);
System.out.println("newLocalDateTime: " + newLocalDateTime); // 1998-11-15T23:30
Java Date Time Tutorials
- Java ZoneId Tutorial with Examples
- Java Temporal Tutorial with Examples
- Java Period Tutorial with Examples
- Java TemporalAdjusters Tutorial with Examples
- Java MinguoDate Tutorial with Examples
- Java TemporalAccessor Tutorial with Examples
- Java JapaneseEra Tutorial with Examples
- Java HijrahDate Tutorial with Examples
- Java Date Time Tutorial with Examples
- What is Daylight Saving Time (DST)?
- Java LocalDate Tutorial with Examples
- Java LocalTime Tutorial with Examples
- Java LocalDateTime Tutorial with Examples
- Java ZonedDateTime Tutorial with Examples
- Java JapaneseDate Tutorial with Examples
- Java Duration Tutorial with Examples
- Java TemporalQuery Tutorial with Examples
- Java TemporalAdjuster Tutorial with Examples
- Java ChronoUnit Tutorial with Examples
- Java TemporalQueries Tutorial with Examples
Show More