Java Date Time Tutorial with Examples
1. Date, time, calendar classes in Java
Java provides several classes related to the time and calendar (Calendar), below is a list of this class:
Class | Description |
java.util.Date | A class that represents a date and time. Most methods in this class is deprecated. |
java.util.concurrent.TimeUnit | TIMEUnit is an Enum describing the units of date and time. |
java.sql.Date | A class that represents a date. All time information cut off. This date class is used with JDBC. |
java.sql.Time | Time: A class describes the time (hours minutes seconds, milliseconds), and does not contain day month year information. This class is often used in JDBC. |
java.sql.Timestamp | A class that represents a date and time. This date and time class is used with JDBC. |
java.util.Calendar | A base class for calendar classes. Has methods to do date and time arithmethics like adding a day or month to another date. |
java.util.GregorianCalendar | A concrete class subclassing java.util.Calendar, representing the Gregorian calendar which is used in most of the western world today. Has all the methods from java.util.Calendar to do date and time arithmethics. |
java.util.TimeZone | The Java TimeZone class is a class that represents time zones, and is helpful when doing calendar arithmetics across time zones. |
java.text.SimpleDateFormat | A class that can help you parse String's into Date's, and format Date's as String's. |
2. System.currentTimeMillis()
currentTimeMillis() is a static method of System class . It returns the amount of time in milliseconds from the date of 1-1-1970 to the present time.
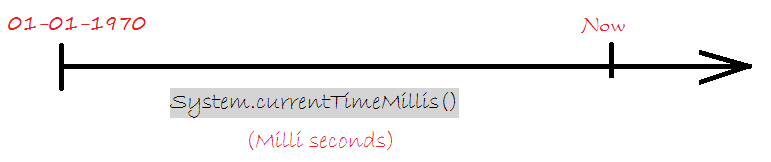
System.currentTimeMillis() is often used to measure the amount of time to do something by calling this method before starting work, and after finishing work.
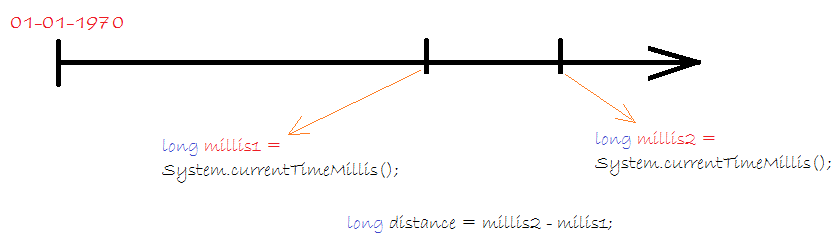
JobTimeDemo.java
package org.o7planning.tutorial.dt;
public class JobTimeDemo {
// This is the method to sum the numbers from 1 to 100.
private static int sum() {
int sum = 0;
for (int i = 0; i <= 100; i++) {
sum += i;
}
return sum;
}
private static void doJob(int count) {
// Invoke 'sum' method with the number of times
// given by the parameter.
for (int i = 0; i < count; i++) {
sum();
}
}
public static void main(String[] args) {
long millis1 = System.currentTimeMillis();
doJob(10000);
long millis2 = System.currentTimeMillis();
long distance = millis2 - millis1;
System.out.println("Distance time in milli second: " + distance);
}
}
Running example:
Distance time in milli second: 3
3. TimeUnit
TimeUnit is an Enum, it was introduced from Java5. It has some method to convert between units of time, and really useful in some situations.
// Number of minutes.
int minute = 5;
// Convert to milliseconds.
// This is the traditional way.
int millisecond = minute * 60 * 1000;
// Using TimeUnit:
long millisecond = TimeUnit.MINUTES.toMillis(minute);
Some methods TimeUnit
// Convert to nanoseconds.
public long toNanos(long d);
// Convert to microseconds.
public long toMicros(long d);
// Convert to milliseconds.
public long toMillis(long d);
// Convert to seconds.
public long toSeconds(long d);
// Convert to minutes.
public long toMinutes(long d);
// Convert to hours.
public long toHours(long d);
// Convert to days.
public long toDays(long d) ;
// Convert to another time unit, given by the parameter 'u'.
public long convert(long d, TimeUnit u);
Example:
TimeUnitConvertDemo.java
package org.o7planning.tutorial.timeunit;
import java.util.concurrent.TimeUnit;
public class TimeUnitConvertDemo {
public static void main(String[] args) {
// Second.
long second = 125553;
// Convert the number of seconds above to minutes.
long minute = TimeUnit.MINUTES.convert(second, TimeUnit.SECONDS);
System.out.println("Minute " + minute);
// Convert the number of seconds above to hours.
long hour = TimeUnit.HOURS.convert(second, TimeUnit.SECONDS);
System.out.println("Hour " + hour);
System.out.println("------");
// Convert 3 days to minutes
minute = TimeUnit.DAYS.toMinutes(3);
System.out.println("Minute " + minute);
// Convert 3 days to hours
hour = TimeUnit.DAYS.toHours(3);
System.out.println("Hour " + hour);
}
}
Running example
Minute 2092
Hour 34
------
Minute 4320
Hour 72
4. java.util.Date
Java's java.util.Date class was one of Java's first date classes. Today most of the methods in the class are deprecated in favor of the java.util.Calendar class. You can still use the java.util.Date class to represent a date though.
There are only 2 constructors can be used:
// Create a Date object describing the current time.
Date date1 = new Date();
// Create a Date object,
// millis - the milliseconds since January 1, 1970, 00:00:00 GMT.
long millis = .....;
Date date2 = new Date(millis);
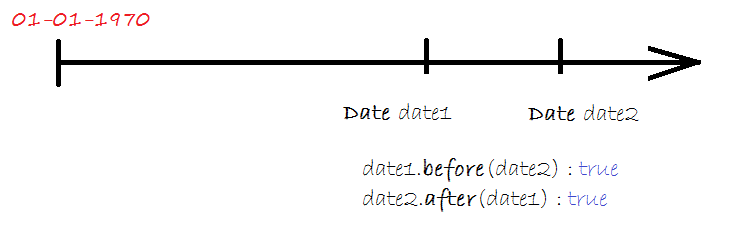
DateDemo.java
package org.o7planning.tutorial.date;
import java.util.Date;
import java.util.concurrent.TimeUnit;
public class DateDemo {
public static void main(String[] args) throws InterruptedException {
// Create a Date object describing the current time.
Date date1 = new Date();
// Stop 3 seconds.
Thread.sleep(TimeUnit.SECONDS.toMillis(3));
// Returns the current time in milliseconds.
// (From 01-01-1970 to now).
long millis = System.currentTimeMillis();
Date date2 = new Date(millis);
// Compare two objects date1 and date2.
// i < 0 means date1 < date2
// i = 0 means date1 = date2
// i > 0 means date1 > date2
int i = date1.compareTo(date2);
System.out.println("date1 compareTo date2 = " + i);
// Tests if this date is before the specified date.
boolean before = date1.before(date2);
System.out.println("date1 before date2 ? " + before);
// Tests if this date is after the specified date.
boolean after = date1.after(date2);
System.out.println("date1 after date2 ? " + after);
}
}
Running example:
date1 compareTo date2 = -1
date1 before date2 ? true
date1 after date2 ? false
5. Date, Time, Timestamp (java.sql)
java.sql has 3 classes related to date and time:
- java.sql.Date
- java.sql.Time
- java.sql.Timestamp
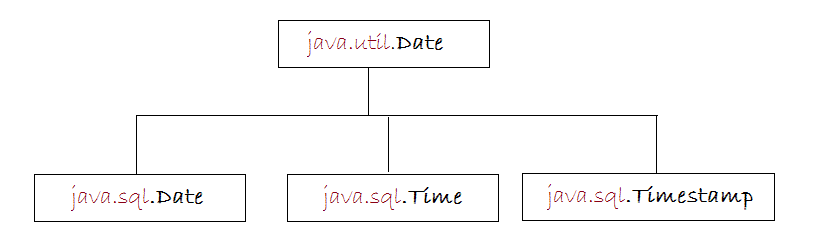
Specifically:
- java.sql.Date corresponds to SQL DATE which means it stores years, months and days while hour, minute, second and millisecond are ignored. Additionally sql.Date isn't tied to timezones.
- java.sql.Time corresponds to SQL TIME and as should be obvious, only contains information about hour, minutes, seconds and milliseconds.
- java.sql.Timestamp corresponds to SQL TIMESTAMP which is exact date to the nanosecond (note that util.Date only supports milliseconds!) with customizable precision.
The classes above is used in the JDBC API, for example the method of PreparedStatement.setDate(), PreparedStatement.setTime(), PreparedStatement.setTimestamp(). Or maybe retrieved from ResultSet.
6. java.util.Calendar
Sơ lược về các bộ lịch:
Gregorian Calendar:The Gregorian calendar, also called the Western calendar and the Christian calendar, is internationally the most widely used civil calendar.It is named for Pope Gregory XIII, who introduced it in 1582.
Buddhist Calendar: This is a Buddhist calendar, commonly used in some former Southeast Asian countries such as Thailand, Laos, Cambodia, as well as Sri Lanka. The calendar is now used in Buddhist festivals. And no other country using the calendar officially, these countries have switched to Gregorian Calendar. You can find more information about this calendar at:
Japanese Imperial Calendar: This is the traditional calendar of Japan, nowadays Japan has switched to Gregorian Calendar, but traditional calendar is still used unofficially.
Calendar class:
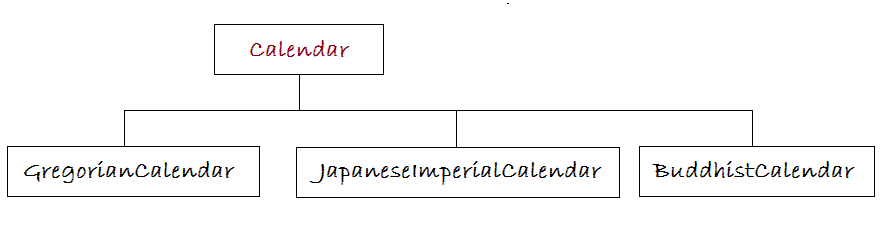
Subclasses of Calendar:
- GregorianCalendar
- JapaneseImperialCalendar
- BuddhistCalendar
Calendar is an abstract class. It means that you can not create it from constructor. However there are two static methods to create Calendar object.
public static Calendar getInstance();
public static Calendar getInstance(TimeZone zone);
public static Calendar getInstance(Locale aLocale);
public static Calendar getInstance(TimeZone zone,Locale aLocale);
Example:
// Create Calendar object describes the present time.
// With default Locale, and default TimeZone.
// (Of running computer).
Calendar c = Calendar.getInstance();
When you use Calendar.getInstance (TimeZone, Locale) will get returns as one of the aforementioned classes. But mostly returned GregorianCalendar.
CallCalendar.getInstance() returns the object of Calendar with the TimeZone parameter according to your computer and the default Locale.
CallCalendar.getInstance() returns the object of Calendar with the TimeZone parameter according to your computer and the default Locale.
Take a look at the code of the Calendar class (JDK 7):
Calendar (JDK7)
/**
* Gets a calendar using the default time zone and locale. The
* <code>Calendar</code> returned is based on the current time
* in the default time zone with the default locale.
*
* @return a Calendar.
*/
public static Calendar getInstance()
{
Calendar cal = createCalendar(TimeZone.getDefaultRef(),
Locale.getDefault(Locale.Category.FORMAT));
cal.sharedZone = true;
return cal;
}
/**
* Gets a calendar using the specified time zone and default locale.
* The <code>Calendar</code> returned is based on the current time
* in the given time zone with the default locale.
*
* @param zone the time zone to use
* @return a Calendar.
*/
public static Calendar getInstance(TimeZone zone)
{
return createCalendar(zone, Locale.getDefault(Locale.Category.FORMAT));
}
/**
* Gets a calendar using the default time zone and specified locale.
* The <code>Calendar</code> returned is based on the current time
* in the default time zone with the given locale.
*
* @param aLocale the locale for the week data
* @return a Calendar.
*/
public static Calendar getInstance(Locale aLocale)
{
Calendar cal = createCalendar(TimeZone.getDefaultRef(), aLocale);
cal.sharedZone = true;
return cal;
}
/**
* Gets a calendar with the specified time zone and locale.
* The <code>Calendar</code> returned is based on the current time
* in the given time zone with the given locale.
*
* @param zone the time zone to use
* @param aLocale the locale for the week data
* @return a Calendar.
*/
public static Calendar getInstance(TimeZone zone,
Locale aLocale)
{
return createCalendar(zone, aLocale);
}
private static Calendar createCalendar(TimeZone zone,
Locale aLocale)
{
Calendar cal = null;
String caltype = aLocale.getUnicodeLocaleType("ca");
if (caltype == null) {
// Calendar type is not specified.
// If the specified locale is a Thai locale,
// returns a BuddhistCalendar instance.
if ("th".equals(aLocale.getLanguage())
&& ("TH".equals(aLocale.getCountry()))) {
cal = new BuddhistCalendar(zone, aLocale);
} else {
cal = new GregorianCalendar(zone, aLocale);
}
} else if (caltype.equals("japanese")) {
cal = new JapaneseImperialCalendar(zone, aLocale);
} else if (caltype.equals("buddhist")) {
cal = new BuddhistCalendar(zone, aLocale);
} else {
// Unsupported calendar type.
// Use Gregorian calendar as a fallback.
cal = new GregorianCalendar(zone, aLocale);
}
return cal;
}
The important methods:
Method get(int) | Return |
get(Calendar.DAY_OF_WEEK) | 1 (Calendar.SUNDAY) to 7 (Calendar.SATURDAY). |
get(Calendar.YEAR) | year |
get(Calendar.MONTH) | 0 (Calendar.JANUARY) to 11 (Calendar.DECEMBER). |
get(Calendar.DAY_OF_MONTH) | 1 to 31 |
get(Calendar.DATE) | 1 to 31 |
get(Calendar.HOUR_OF_DAY) | 0 to 23 |
get(Calendar.MINUTE) | 0 to 59 |
get(Calendar.SECOND) | 0 to 59 |
get(Calendar.MILLISECOND) | 0 to 999 |
get(Calendar.HOUR) | 0 to 11, to be used together with Calendar.AM_PM. |
get(Calendar.AM_PM) | 0 (Calendar.AM) or 1 (Calendar.PM). |
get(Calendar.DAY_OF_WEEK_IN_MONTH) | DAY_OF_MONTH 1 through 7 always correspond to DAY_OF_WEEK_IN_MONTH 1;
8 through 14 correspond to DAY_OF_WEEK_IN_MONTH 2, and so on. |
get(Calendar.DAY_OF_YEAR) | 1 to 366 |
get(Calendar.ZONE_OFFSET) | GMT offset value of the time zone. |
get(Calendar.ERA) | Indicate AD (GregorianCalendar.AD), BC (GregorianCalendar.BC). |
CalendarFieldsDemo.java
package org.o7planning.tutorial.calendar;
import java.util.Calendar;
public class CalendarFieldsDemo {
public static void main(String[] args) {
// Create a calendar using the default time zone and locale.
Calendar c = Calendar.getInstance();
int year = c.get(Calendar.YEAR);
// Returns value from 0 - 11
int month = c.get(Calendar.MONTH);
int day = c.get(Calendar.DAY_OF_MONTH);
int hour = c.get(Calendar.HOUR_OF_DAY);
int minute = c.get(Calendar.MINUTE);
int second = c.get(Calendar.SECOND);
int millis = c.get(Calendar.MILLISECOND);
System.out.println("Year: " + year);
System.out.println("Month: " + (month + 1));
System.out.println("Day: " + day);
System.out.println("Hour: " + hour);
System.out.println("Minute: " + minute);
System.out.println("Second: " + second);
System.out.println("Minute: " + minute);
System.out.println("Milli Second: " + millis);
}
}
Running example:
Year: 2021
Month: 5
Day: 15
Hour: 20
Minute: 34
Second: 52
Minute: 34
Milli Second: 382
Some other methods of Calendar:
void set(int calendarField, int value)
void set(int year, int month, int date)
void set(int year, int month, int date, int hour, int minute, int second)
// Adds or subtracts the specified amount of time to the given calendar field.
// Based on the calendar's rules.
void add(int field, int amount)
// Roll (up/down) a field of Calendar.
// roll(): Does not affect other fields of Calendar.
void roll(int calendarField, boolean up)
// Roll up a field of Calendar.
// roll(): Does not affect other fields of Calendar.
void roll(int calendarField, int amount):
// Returns a Date object based on values of Calendar.
Date getTime()
void setTime(Date date)
// Return number of milliseconds of this Calendar.
long getTimeInMills():
void setTimeInMillis(long millis)
void setTimeZone(TimeZone value)
CalendarDemo.java
package org.o7planning.tutorial.calendar;
import java.util.Calendar;
public class CalendarDemo {
public static void showCalendar(Calendar c) {
int year = c.get(Calendar.YEAR);
// Return value from 0 - 11
int month = c.get(Calendar.MONTH);
int day = c.get(Calendar.DAY_OF_MONTH);
int hour = c.get(Calendar.HOUR_OF_DAY);
int minute = c.get(Calendar.MINUTE);
int second = c.get(Calendar.SECOND);
int millis = c.get(Calendar.MILLISECOND);
System.out.println(" " + year + "-" + (month + 1) + "-" + day //
+ " " + hour + ":" + minute + ":" + second + " " + millis);
}
public static void main(String[] args) {
// Create a calendar using the default time zone and locale
Calendar c = Calendar.getInstance();
System.out.println("First calendar info");
showCalendar(c);
// roll(..): Roll a field of Calendar.
// roll(..): Does not change other fields.
// Example: Roll up one hour (boolean up = true)
c.roll(Calendar.HOUR_OF_DAY, true);
System.out.println("After roll 1 hour");
showCalendar(c);
// roll(..): Does not change other fields.
// Roll down one hour (boolean up = false)
c.roll(Calendar.HOUR_OF_DAY, false);
System.out.println("After roll -1 hour");
showCalendar(c);
// add(..): Can change other fields of Calendar.
// Adding one hour (boolean up = true)
c.add(Calendar.HOUR_OF_DAY, 1);
System.out.println("After add 1 hour");
showCalendar(c);
// roll(..): Does not change other fields of Calendar.
// Roll down 30 day (boolean up = false)
c.roll(Calendar.DAY_OF_MONTH, -30);
System.out.println("After roll -30 day");
showCalendar(c);
// add(..): Can change other fields of Calendar.
// Adding 30 days (boolean up = true)
c.add(Calendar.DAY_OF_MONTH, 30);
System.out.println("After add 30 day");
showCalendar(c);
}
}
Running example:
First calendar info
2021-5-15 20:35:27 395
After roll 1 hour
2021-5-15 21:35:27 395
After roll -1 hour
2021-5-15 20:35:27 395
After add 1 hour
2021-5-15 21:35:27 395
After roll -30 day
2021-5-16 21:35:27 395
After add 30 day
2021-6-15 21:35:27 395
7. Convert between Date and Calendar
- Date ==> Calendar
Date now = new Date();
Calendar c = Calendar.getInstance();
c.setTime(now);
- Calendar ==> Date
Calendar c = Calendar.getInstance();
Date date = c.getTime();
CalendarDateConversionDemo.java
package org.o7planning.tutorial.calendar;
import java.util.Calendar;
import java.util.Date;
import java.util.concurrent.TimeUnit;
public class CalendarDateConversionDemo {
public static void showCalendar(Calendar c) {
int year = c.get(Calendar.YEAR);
// Returns the value from 0-11
int month = c.get(Calendar.MONTH);
int day = c.get(Calendar.DAY_OF_MONTH);
int hour = c.get(Calendar.HOUR_OF_DAY);
int minute = c.get(Calendar.MINUTE);
int second = c.get(Calendar.SECOND);
int millis = c.get(Calendar.MILLISECOND);
System.out.println(year + "-" + (month + 1) + "-" + day //
+ " " + hour + ":" + minute + ":" + second + " " + millis);
}
public static void main(String[] args) {
Calendar c = Calendar.getInstance();
// year, month, day
c.set(2000, 11, 24);
Date date = c.getTime();
System.out.println("Date " + date);
long timeInMillis = System.currentTimeMillis();
// Subtract 24 hours
timeInMillis -= TimeUnit.HOURS.toMillis(24);
Date date2 = new Date(timeInMillis);
Calendar c2 = Calendar.getInstance();
c2.setTime(date2);
showCalendar(c2);
}
}
Running example:
Date Sun Dec 24 20:36:10 KGT 2000
2021-5-14 20:36:10 608
8. DateFormat & SimpleDateFormat
- Date ==> String
Date date = new Date();
DateFormat df = new SimpleDateFormat("dd-MM-yyyy HH:mm:ss");
String dateString = df.format(date);
- String ==> Date
String dateString = "23/04/2005 23:11:59";
DateFormat df = new SimpleDateFormat("dd/MM/yyyy HH:mm:ss");
Date date = df.parse(dateString);
DateFormatDemo.java
package org.o7planning.tutorial.dateformat;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateFormatDemo {
public static void main(String[] args) throws ParseException {
final DateFormat df1 = new SimpleDateFormat("dd/MM/yyyy HH:mm:ss");
String dateString1 = "23/04/2005 23:11:59";
System.out.println("dateString1 = " + dateString1);
// String ==> Date
Date date1 = df1.parse(dateString1);
System.out.println("date1 = " + date1);
final DateFormat df2 = new SimpleDateFormat("dd-MM-yyyy HH:mm:ss");
// Date ==> String.
String dateString2 = df2.format(date1);
System.out.println("dateString2 = " + dateString2);
}
}
Running example:
dateString1 = 23/04/2005 23:11:59
date1 = Sat Apr 23 23:11:59 KGST 2005
dateString2 = 23-04-2005 23:11:59
Customize formats for date, time
Customizing Date format . Let's see some examples of format and returned results.
Pattern | Output |
dd.MM.yy | 30.06.09 |
yyyy.MM.dd G 'at' hh:mm:ss z | 2009.06.30 AD at 08:29:36 PDT |
EEE, MMM d, ''yy | Tue, Jun 30, '09 |
h:mm a | 8:29 PM |
H:mm | 8:29 |
H:mm:ss:SSS | 8:28:36:249 |
K:mm a,z | 8:29 AM,PDT |
yyyy.MMMMM.dd GGG hh:mm aaa | 2009.June.30 AD 08:29 AM |
Date Format pattern Syntax
Symbol | Meaning | Presentation | Example |
G | era designator | Text | AD |
y | year | Number | 2009 |
M | month in year | Text & Number | July & 07 |
d | day in month | Number | 10 |
h | hour in am/pm (1-12) | Number | 12 |
H | hour in day (0-23) | Number | 0 |
m | minute in hour | Number | 30 |
s | second in minute | Number | 55 |
S | millisecond | Number | 978 |
E | day in week | Text | Tuesday |
D | day in year | Number | 189 |
F | day of week in month | Number | 2 (2nd Wed in July) |
w | week in year | Number | 27 |
W | week in month | Number | 2 |
a | am/pm marker | Text | PM |
k | hour in day (1-24) | Number | 24 |
K | hour in am/pm (0-11) | Number | 0 |
z | time zone | Text | Pacific Standard Time |
' | escape for text | Delimiter | (none) |
' | single quote | Literal | ' |
- Java Date Time Format Pattern
Java Date Time Tutorials
- Java ZoneId Tutorial with Examples
- Java Temporal Tutorial with Examples
- Java Period Tutorial with Examples
- Java TemporalAdjusters Tutorial with Examples
- Java MinguoDate Tutorial with Examples
- Java TemporalAccessor Tutorial with Examples
- Java JapaneseEra Tutorial with Examples
- Java HijrahDate Tutorial with Examples
- Java Date Time Tutorial with Examples
- What is Daylight Saving Time (DST)?
- Java LocalDate Tutorial with Examples
- Java LocalTime Tutorial with Examples
- Java LocalDateTime Tutorial with Examples
- Java ZonedDateTime Tutorial with Examples
- Java JapaneseDate Tutorial with Examples
- Java Duration Tutorial with Examples
- Java TemporalQuery Tutorial with Examples
- Java TemporalAdjuster Tutorial with Examples
- Java ChronoUnit Tutorial with Examples
- Java TemporalQueries Tutorial with Examples
Show More