Swift Closures Tutorial with Examples
1. What is Closure?
Closure: Closure is a special block, it may have 0 or more parameters, and can have return type. It's almost like a block in C or Object-C.
To simpler, you can see the following declaration, can you guest its meaning?
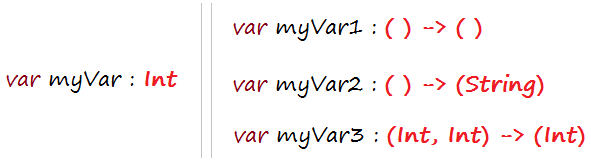
Above declaration can be explained in this illustration below:
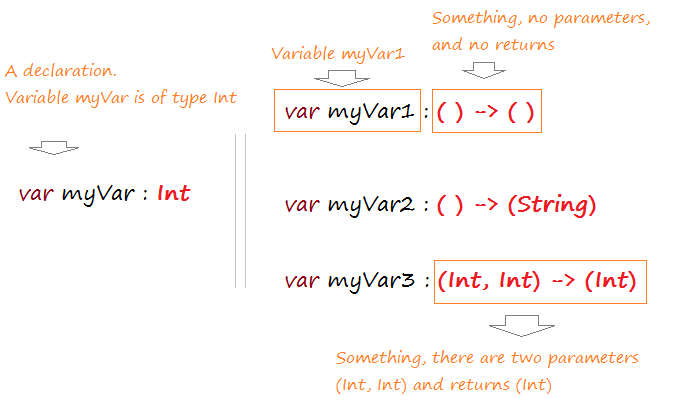
This is a syntax to declare variables with the data type, and assign values to variables that you are familiar:
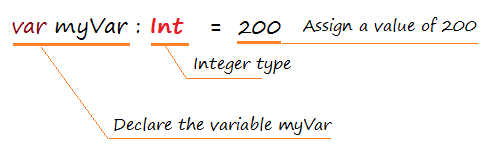
MyFirstClosure.swift
import Foundation
// Declare the variable myVar1, with data type, and assign value.
var myVar1 : () -> () = {
print("Hello from Closure 1");
}
// Declare the variable myVar2, with data types, and assign value.
var myVar2 : () -> (String) = { () -> (String) in
return "Hello from Closure 2"
}
// Declare the variable myVar3, with data types, and assign value.
var myVar3 : (Int, Int) -> (Int) = { (a : Int, b: Int) -> (Int) in
var c : Int = a + b
return c
}
func test_closure() {
// Execute Closure.
myVar1()
// Execute Closure, and get returns value.
var str2 = myVar2()
print(str2)
// Execute closure, pass parameters
// and get returns value.
var c: Int = myVar3(11, 22)
print(c)
}
Closure is the block, there may be parameters, and can have the return type:
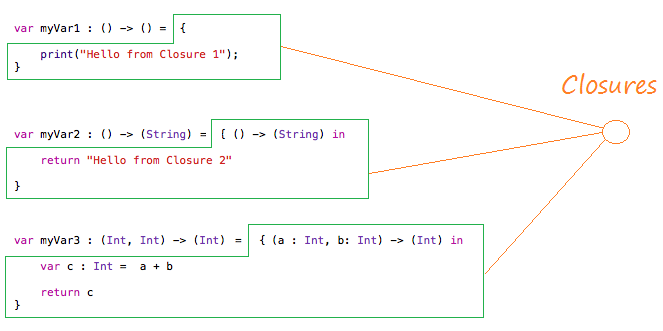
Syntax of Closure:
{ (parameters) -> returntype in
// statements
}
2. Function vs Closure
function is a special case of Closure.function is Closure named or can be said Closure is a anonymous function.
Definition
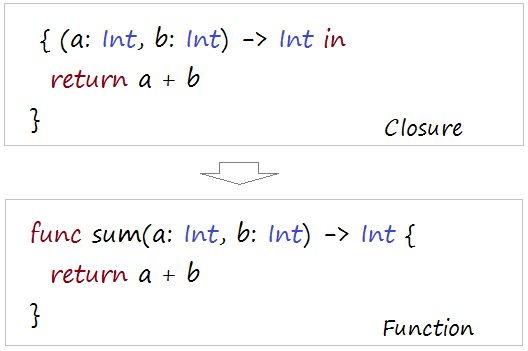
Using:
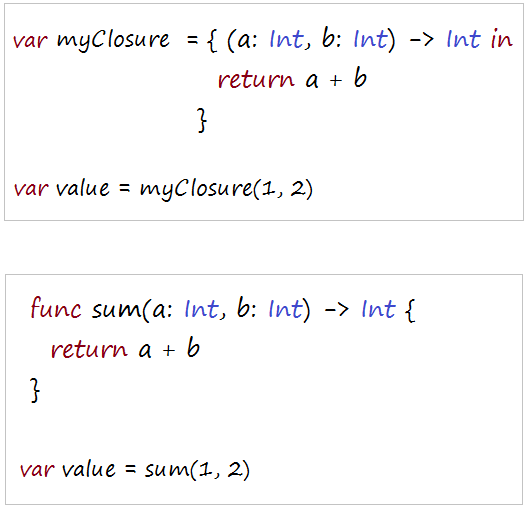
3. Anonymous Closure
When declaring Closure, you may not need to write the name of the parameters, the parameters can be referenced through $0, $1, ...
AnonymousClosure.swift
import Foundation
// Declare a Closure in the usual way.
var myClosure : (String, String) -> String
= { (firstName: String, lastName: String) -> String in
return firstName + " " + lastName
}
// Declare a Closure in the anonymous way.
// (Ignore parameter names).
var anonymousClosure : (String, String) -> String
= {
// Using
// $0: For first parameter
// $1: For second parameter.
return $0 + " " + $1
}
Note: $0, $1, ... are the anonymous parameters, they are only used in the anonymous Closure, if used in the common Closure you will get an error message:Anonymous closure arguments cannot be used inside a closure that has explicit arguments
For example, the anonymous Closure (2)
AnonymosClosure2.swift
import Foundation
func test_anonymousClosure() {
// Declare a variable type of Closure.
var mySum : ( Int, Int ) -> (Int)
// Assign a anonymous closure.
// $0: For first parameter.
// $1: For second parameter.
mySum = {
return $0 + $1
}
var value = mySum(1, 2)
print(value)
}
4. Implicit Return Values
If the content of Calosure have an single expression, you can omit the return keyword.
ImplicitReturnValues.swift
import Foundation
// This is a closure whose content only has a single expression
var closureWithReturn = { (a: Int, b: Int) -> Int in
// A single expression.
return a + b
}
// Can omit the 'return' keyword.
var closureWithoutReturn = { (a: Int, b: Int) -> Int in
// If only a single expression.
// Omit 'return' keyword.
a + b
}
Swift Programming Tutorials
- Install Mac OS X 10.11 El Capitan in VMWare
- Install XCode
- Swift Tutorial for Beginners
- Swift Functions Tutorial with Examples
- Swift Closures Tutorial with Examples
- Class and Object in Swift
- Swift Enums Tutorial with Examples
- Swift Structs Tutorial with Examples
- Programming for Team using XCode and SVN
Show More