Introduction to Thymeleaf
1. What is Thymeleaf?
Thymeleaf is a modern Java Template Engine, operating at the server side for two Web and standard environments. It can process HTML, XML, Javascript, CSS, evenplain text.
A Thymeleaf Template
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Person List</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}"/>
</head>
<body>
<h1>Person List</h1>
<a href="addPerson">Add Person</a>
<br/><br/>
<div>
<table border="1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr th:each ="person : ${persons}">
<td th:utext="${person.firstName}">...</td>
<td th:utext="${person.lastName}">...</td>
</tr>
</table>
</div>
</body>
</html>
Thymeleaf Engine will parseThymeleaf Template. It uses Java data to replace the positions marked on the Thymeleaf Template to create a new text.
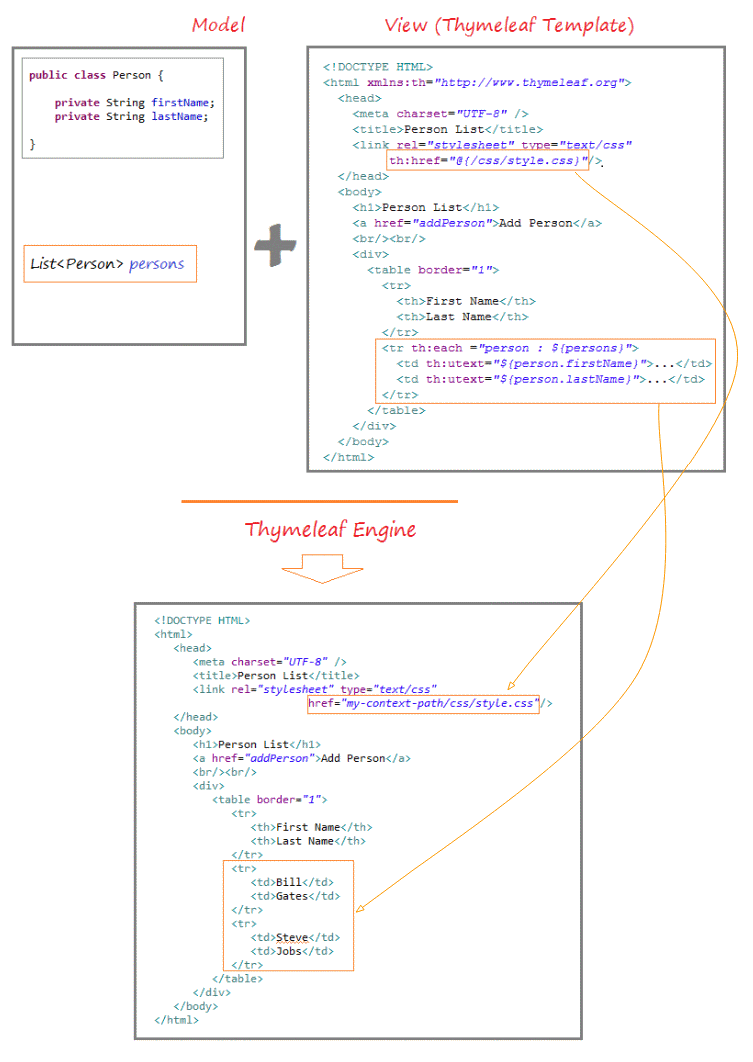
2. Types of Template in Thymeleaf
Thymeleaf consists of 6 types of Template. Each type of Template is also referred to as a Template Mode.
- HTML
- XML
- TEXT
- JAVASCRIPT
- CSS
- RAW
HTML Template Mode
The HTML Template mode allows any HTML input, including HTML5, HTML4, XHTML. When processing these materials, Thymeleaf Engine will not check the well-formed of material and it also doesn't validate this material.
In the HTML Template mode, Thymeleaf doesn't create new tags (excluding the <th:block> tag). It adds its markups to the available tags of HTML. You can imagine the interface of page when you open HTML Thymeleaf Template directly on the browser. Therefore, the Thymeleaf helps to remarkably narrow the distance between design team and application development team.
Below is a HTML Thymeleaf Template sample:
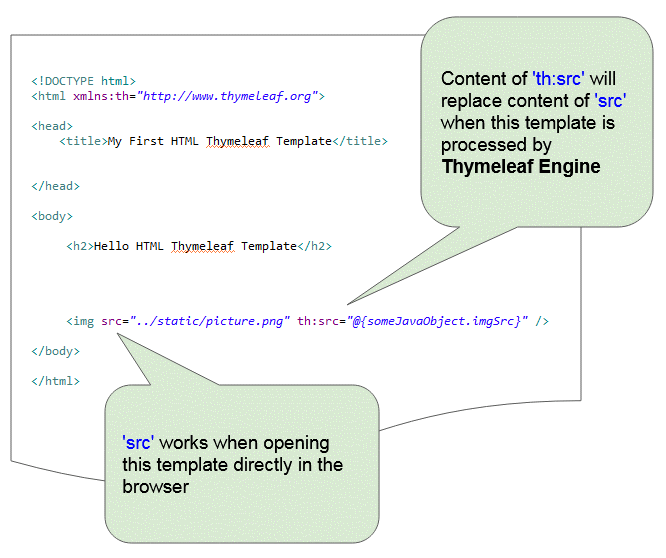
When opening HTML Template directly on browser, you can see the interface of page.
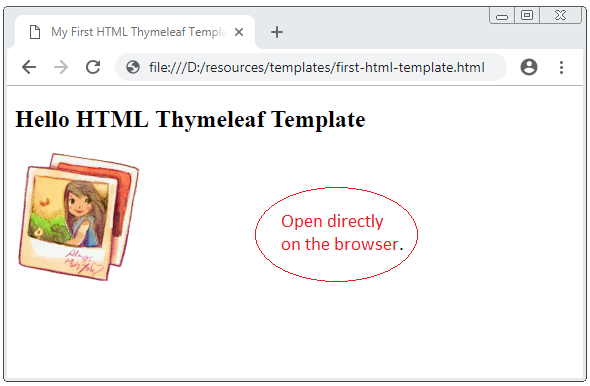
first-html-template.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>My First HTML Thymeleaf Template</title>
</head>
<body>
<h2>Hello HTML Thymeleaf Template</h2>
<!-- 'src' works when opening this template directly in the browser -->
<!-- 'th:src' will replate 'src' when this template is processed by Thymeleaf Engine -->
<img src="../static/picture.png" th:src="@{someJavaObject.imgSrc}" />
</body>
</html>
XML Template Mode
The XML Template mode allows that input is XML. Themeleaf Engine will check to ensure that this XML material is well-formed , ei. it is imperative to ensure that each open tag must have one close tag, and value of an attribute must be put in single quotation marks ' ' or double quotation marks " ",... in case of violating an exception will be thrown out. Note: Themeleaft Engine doesn't validate that this XML material is suitable or not for a DTD or a XML Schema.
XML Template example
<?xml version="1.0" encoding="UTF-8"?>
<persons>
<person>
<fname th:text="${personInfo.lastName}"></fname>
<lname th:text="${personInfo.firstName}"></lname>
<country th:text="${personInfocountry}"></country>
</person>
</persons>
TEXT Template Mode
TEXT Template mode uses a special syntax for Templates. The inputs are normal texts such as Text Email,... It is noted that HTML Template or XML Template can also be considered as a plain text .Thymeleaf Engine will not care about tags. They are only considered as texts.
TEXT Template example
Dear [(${customer.name})],
This is the list of our products:
[# th:each="p : ${products}"]
- [(${p.name})]. Price: [(${#numbers.formatdecimal(p.price,1,2)})] EUR/kg
[/]
Thanks,
The Thymeleaf Shop
JAVASCRIPT template Mode
Javascript Template mode allows to process Javascript files in the Thymeleaf application. This means that it is possible to use the data model in Javascript files like the way under which it can make in HTML files. However, the Javascript file is only considered as a plain text, so it uses the syntax similar to that of the TEXT Template mode.
Example withthe Javascript Template file:
Javascript Template example
// Javascript Template:
function showCode() {
var code = /*[[${code}]]*/ '12345';
document.getElementById('code').innerHTML = code;
}
// ==> Output:
function showCode() {
var code = 11223';
document.getElementById('code').innerHTML = code;
}
Example with Javascript Template embedded in HTML:
Javascript Template (Inline example)
<!-- Javascript Template -->
<script th:inline="javascript">
/*<![CDATA[*/
var user = /*[[${someObject.userName}]]*/ 'User Name';
/*]]>*/
</script>
<!-- OUTPUT: -->
<script th:inline="javascript">
/*<![CDATA[*/
var user = 'Tom';
/*]]>*/
</script>
CSS template Mode
CSS Template mode allows processing CSS files in the Thymeleaf application. It uses a syntax similar to that of the Javascript Template mode.
Example with CSS Template embedded in HTML:
Css Template (Inline example)
<style th:inline="text">
.some-div {
background-image: url([[@{(${someObject.imagePath})}]]);
}
</style>
RAW Template Mode
RAW Template mode will not process Templates. It is used to insert unprocessed resources (File, URL Response, ..) to Template, for example, external resources, or not controlled by HTML format.
3. Dialect
Thymeleaf is a tool allowing customization, specifically, it allows you define how your Template will be processed. The core library of Thymeleaf provides a dialect called Standard Dialect, which is enough for use for everyone.
Spring Framework creates a private dialect called SpringStandard Dialect, like the standard dialect of Thymeleaf, but with minor adjustments for better use of some features in Spring Framework, for example: It uses Spring Expression Language (SpringEL) instead of using OGNL. Therefore, if you are a Spring Framework user, you won't waste your time, because almost everything you learn here will be used in your Spring applications.
SpringStandard Dialect example
<table border="1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr th:each ="person : ${persons}">
<td th:utext="${person.firstName}">...</td>
<td th:utext="${person.lastName}">...</td>
</tr>
</table>
Standard Dialect example
<table border="1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr th:each ="person : ${persons}">
<td th:utext="${person['firstName']}">...</td>
<td th:utext="${person['lastName']}">...</td>
</tr>
</table>
Thymeleaf is created for Java language, and therefore, you can use it in any platform of Java, for example, Servlet, Struts, Spring,... However, Spring Framework has really supported very much therefore, using the Thymeleaf in Spring becomes easier.
In January 2017, a lot of evaluations of demands for using Thymeleaf for programmers occured at the Thymeleaf forum and it is evaluated which framework is used the most with Thymeleaf, and below are the results.
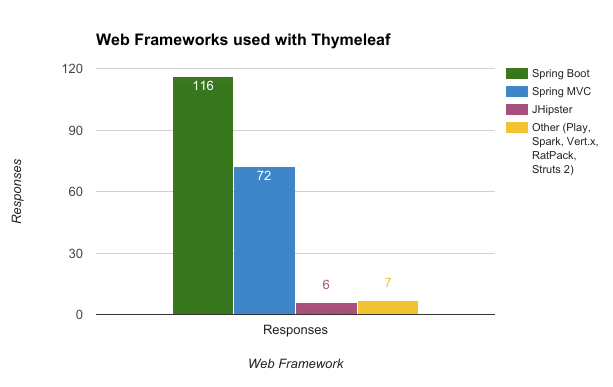
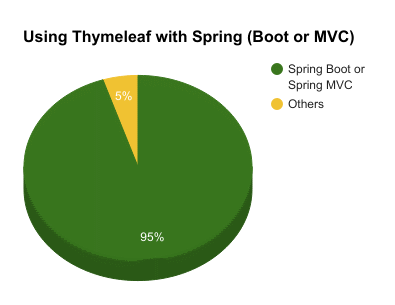
Thymeleaf Tutorials
- Thymeleaf Elvis Operator Tutorial with Examples
- Thymeleaf Loops Tutorial with Examples
- Thymeleaf Conditional statements: if, unless, switch
- Thymeleaf Predefined Objects Tutorial with Examples
- Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Introduction to Thymeleaf
- Thymeleaf Variables Tutorial with Examples
- Thymeleaf Fragments Tutorial with Examples
- Thymeleaf Page Layouts Tutorial with Examples
- Thymeleaf th:object and Asterisk Syntax *{ }
- Thymeleaf Form Select option Example
Show More