Thymeleaf Predefined Objects Tutorial with Examples
1. Predefined Objects
In Thymeleaf, there are several predefined objects and you can use them everywhere in the Thymeleaf Template. Basically there are 2 such types of objects such as Basic Objects, and Utility Objects:
These predefined objects will be referenced according to OGNL standard, starting with the symbol (#).
2. Basic Objects
Object | Class/Interface | Description |
#ctx | org.thymeleaf.context.IContext org.thymeleaf.context.IWebContext | An object implements the IContext or IWebContext interface, depending on the environment (Standard or Web). |
#locale | java.util.Locale | An object that provides information related to Locale. |
#request | javax.servlet.http.HttpServletRequest | (Only in Web Contexts) the HttpServletRequest object. |
#response | javax.servlet.http.HttpServletResponse | (Only in Web Contexts) the HttpServletResponse object. |
#session | javax.servlet.http.HttpSession | (Only in Web Contexts) the HttpSession object. |
#servletContext | javax.servlet.http.ServletContext | (Only in Web Contexts) the ServletContext object. |
#ctx
#ctx is a Context object). It implements org.thymeleaf.context.IContext or org.thymeleaf.context.IWebContext interface, depending on environment (Standard or Web).
Two other objects such as #vars, #root are also similar to #ctx but #ctx is encouraged to use instead of 2 other objects.
Depending on the standard or web environment, the #ctx object can provide you with information:
<!--
* ===============================================
* See javadoc API for class org.thymeleaf.context.IContext
* ===============================================
-->
${#ctx.locale}
${#ctx.variableNames}
<!--
* ================================================
* See javadoc API for class org.thymeleaf.context.IWebContext
* ================================================
-->
${#ctx.request}
${#ctx.response}
${#ctx.session}
${#ctx.servletContext}
In the Spring environment, the #ctx object doesn't operate as expected, ${#ctx.locale}, ${#ctx.request}, ${#ctx.response}, ${#ctx.request}, ${#ctx.servletContext} always returns null. You should use the #locale, #request, #response, #servletContext objects for replacement.
#locale
The #locale (java.util.Locale) object gives you the information on the environment in which it is operating, for example, geographic area, language, culture, digital format, date and time format, ...
predefined-object-locale.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>Predefined Object #locale</h1>
<h3>#locale.locale</h3>
<span th:utext="${#locale.country}"></span>
<h3>#locale.language</h3>
<span th:utext="${#locale.language}"></span>
</body>
</html>
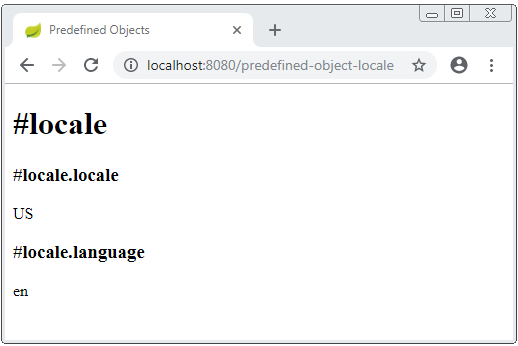
#request
predefined-object-request.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>Predefined Object #request</h1>
<h3>#request.contextPath</h3>
<span th:utext="${#request.contextPath}"></span>
<h3>#request.requestURI</h3>
<span th:utext="${#request.requestURI}"></span>
<h3>#request.requestURL</h3>
<span th:utext="${#request.requestURL}"></span>
</body>
</html>
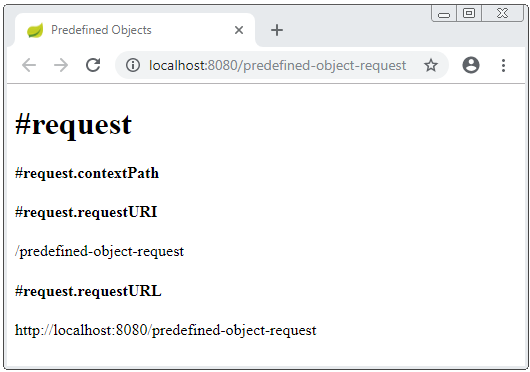
#response
predefined-object-response.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>Predefined Object #response</h1>
<h3>#response.headerNames (java.utils.Enumeration)</h3>
<ul>
<th:block th:each="headerName : ${#request.headerNames}">
<li th:utext="${headerName}">Header Name</li>
</th:block>
</ul>
</body>
</html>
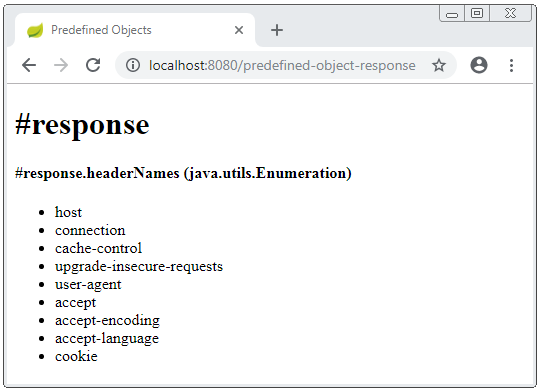
#servletContext
predefined-object-servletContext.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>Predefined Object #servletContext</h1>
<h3>#servletContext.attributeNames (java.utils.Enumeration)</h3>
<ul>
<th:block th:each="attrName : ${#servletContext.attributeNames}">
<li th:utext="${attrName}">Attribute Name</li>
<li th:utext="${#servletContext.getAttribute(attrName)}">Attribute Value</li>
</th:block>
</ul>
</body>
</html>
#session
Spring Controller
// ....
@RequestMapping("/predefined-object-session")
public String objectSession(HttpServletRequest request) {
HttpSession session = request.getSession();
session.setAttribute("mygreeting", "Hello Everyone!");
return "predefined-object-session";
}
predefined-object-session.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>Predefined Object #session</h1>
<h3>#session.getAttribute('mygreeting')</h3>
<span th:utext="${#session.getAttribute('mygreeting')}"></span>
</body>
</html>
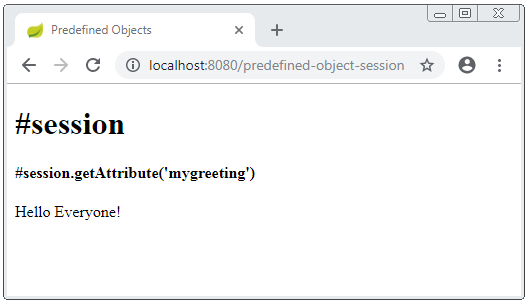
3. Utility Objects
Object | Class/Interface | Description |
#execInfo | org.thymeleaf.expression.ExecutionInfo | Information about the template being processed. |
#messages | org.thymeleaf.expression.Messages | Methods for working with messages. |
#uris | org.thymeleaf.expression.Uris | Methods for escaping parts of URLs/URIs |
#conversions | org.thymeleaf.expression.Conversions | Methods for executing the configured conversion service (if any). |
#dates | javax.servlet.http.HttpSession | Methods to format the java.util.Date object, or get related information such as date, month, year, .. |
#calendars | javax.servlet.http.ServletContext | Analogous to #dates, but for java.util.Calendar objects. |
#numbers | org.thymeleaf.expression.Numbers | Methods for formatting numeric objects. |
#strings | org.thymeleaf.expression.Strings | Methods for String objects. For example: contains, startsWith, etc. |
#objects | org.thymeleaf.expression.Objects | Methods for objects in general. |
#bools | org.thymeleaf.expression.Bools | Methods for boolean evaluation. |
#arrays | org.thymeleaf.expression.Arrays | Methods for arrays. |
#lists | org.thymeleaf.expression.Lists | Methods for lists. |
#sets | org.thymeleaf.expression.Sets | Methods for sets. |
#maps | org.thymeleaf.expression.Maps | Methods for maps. |
#aggregates | org.thymeleaf.expression.Aggregates | Methods for summing, averaging, .. on a set (collection) or array (array). |
#ids | org.thymeleaf.expression.Ids | Methods for dealing with id attributes that might be repeated (for example, as a result of an iteration). |
See also:
#execInfo
The object helps you get the information about the Template being processed.
@RequestMapping("/predefined-u-object-execInfo")
public String execInfo_object() {
return "predefined-u-object-execInfo";
}
predefined-u-object-execInfo.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#execInfo</h1>
<h3>#execInfo.templateMode</h3>
<span th:utext="${#execInfo.templateMode}"></span>
<h3>#execInfo.templateName</h3>
<span th:utext="${#execInfo.templateName}"></span>
<h3>#execInfo.now (java.util.Calendar)</h3>
<span th:utext="${#execInfo.now}"></span>
</body>
</html>
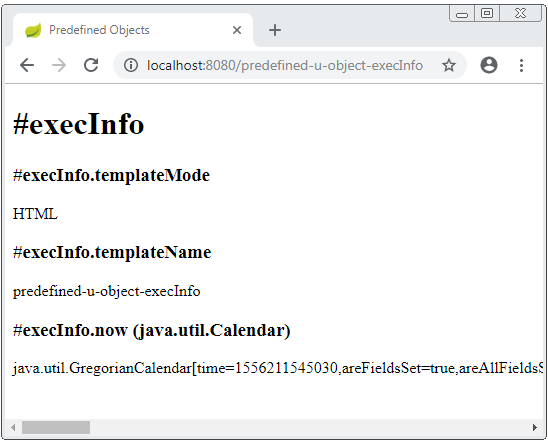
#uris
Provide methods for escaping parts of URLs/URIs
predefined-u-object-uris.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#uris</h1>
<h4>#uris.escapePath('https://example.com?user=Tom&gender=Male')</h4>
<span th:utext="${#uris.escapePath('https://example.com?user=Tom&gender=Male')}"></span>
<h4>#uris.unescapePath('https://example.com%3Fuser=Tom&gender=Male')</h4>
<span th:utext="${#uris.unescapePath('https://example.com%3Fuser=Tom&gender=Male')}"></span>
</body>
</html>
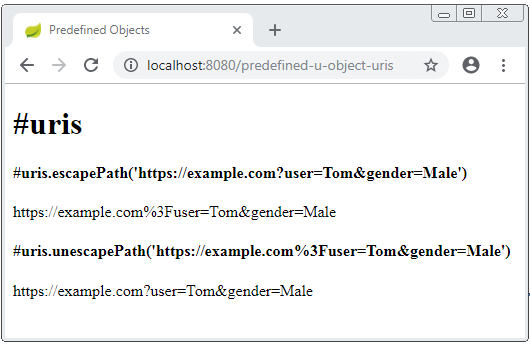
#dates
Provide methods to format the java.util.Date object, or get the relevant information such as date, month, year, ..
predefined-u-object-dates.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#dates</h1>
<!-- Create a variable 'now' (java.util.Date), it exists in block -->
<th:block th:with="now = ${#dates.createNow()}">
<span th:utext="${now}"></span>
<h4>#dates.format(now, 'yyyy-MM-dd HH:mm:ss')</h4>
<span th:utext="${#dates.format( now , 'yyyy-MM-dd HH:mm:ss')}">Date String</span>
<h4>#dates.year(now)</h4>
<span th:utext="${#dates.year(now)}">Year</span>
<h4>#dates.month(now)</h4>
<span th:utext="${#dates.month(now)}">Month</span>
</th:block>
</body>
</html>
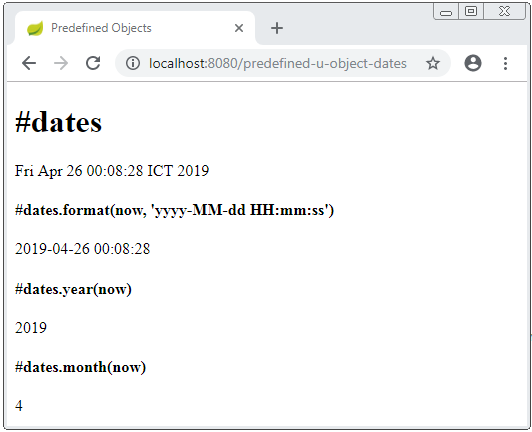
#calendars
Provide methods to format the java.util.Calendar object, or get related information such as date, month, year, ..
predefined-u-object-calendars.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#calendars</h1>
<!-- Create a variable 'now' (java.util.Calendar), it exists in block -->
<th:block th:with="now = ${#calendars.createNow()}">
<span th:utext="${now}"></span>
<h4>#calendars.format(now, 'yyyy-MM-dd HH:mm:ss')</h4>
<span th:utext="${#dates.format( now , 'yyyy-MM-dd HH:mm:ss')}">Date String</span>
<h4>#dates.year(now)</h4>
<span th:utext="${#dates.year(now)}">Year</span>
<h4>#dates.month(now)</h4>
<span th:utext="${#dates.month(now)}">Month</span>
</th:block>
</body>
</html>
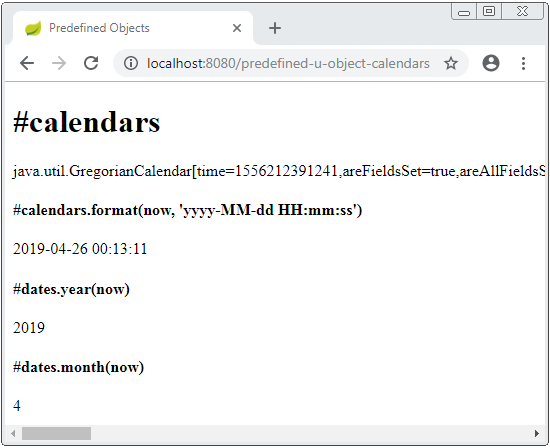
#numbers
Provide methods for formatting numeric objects.
predefined-u-object-numbers.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#numbers</h1>
<!-- Create a Number, it exists in block -->
<th:block th:with="num = 12345.987654">
<h4>num</h4>
<span th:utext="${num}">Number</span>
<h4>${#numbers.formatInteger(num,3)}</h4>
<span th:utext="${#numbers.formatInteger(num,3)}">Number</span>
<h4>${#numbers.formatInteger(num,3,'POINT')}</h4>
<span th:utext="${#numbers.formatInteger(num,3,'POINT')}">Number</span>
<h4>${#numbers.formatDecimal(num,3,'POINT',2,'COMMA')}</h4>
<span th:utext="${#numbers.formatDecimal(num,3,'POINT',2,'COMMA')}">Number</span>
</th:block>
</body>
</html>
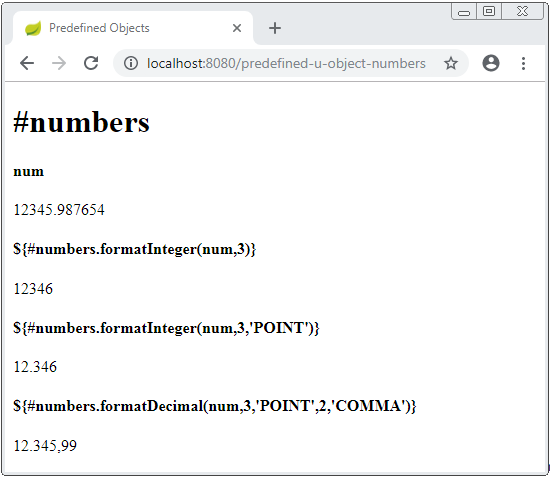
#strings
#objects
Provide methods for objects in general.
@RequestMapping("/predefined-u-object-objects")
public String objects_object(Model model) {
// An array store null values.
String[] colors = new String[] {"red", "blue", null, "green", null, "red"};
model.addAttribute("colors", colors);
return "predefined-u-object-objects";
}
predefined-u-object-objects.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#objects</h1>
<h4>#objects.arrayNullSafe(colors,'white')</h4>
<ul>
<li th:each="color : ${#objects.arrayNullSafe(colors,'white')}"
th:utext="${color}"></li>
</ul>
<h4>And other methods..</h4>
</body>
</html>
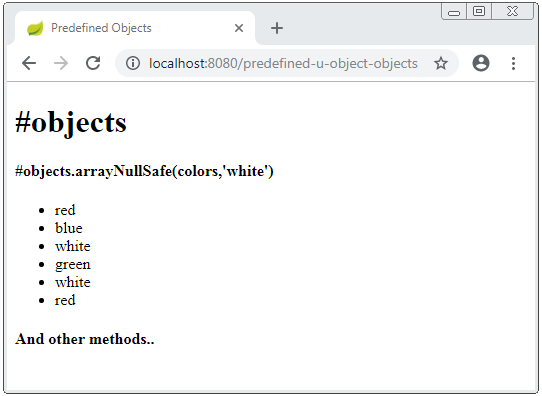
#bools
Provide methods for boolean evaluation.
@RequestMapping("/predefined-u-object-bools")
public String bools_object(Model model) {
// An array store null values.
String[] colors = new String[] {"red", null , "blue"};
model.addAttribute("colors", colors);
return "predefined-u-object-bools";
}
predefined-u-object-bools.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#bools</h1>
<h4>Array: colors</h4>
<ul>
<li th:each="color : ${colors}"
th:utext="${color}"></li>
</ul>
<h4>#bools.arrayIsTrue(colors)</h4>
<ul>
<li th:each="color : ${#bools.arrayIsTrue(colors)}"
th:utext="${color}"></li>
</ul>
<h4>#bools.arrayIsFalse(colors)</h4>
<ul>
<li th:each="color : ${#bools.arrayIsFalse(colors)}"
th:utext="${color}"></li>
</ul>
<h4>And other methods..</h4>
</body>
</html>
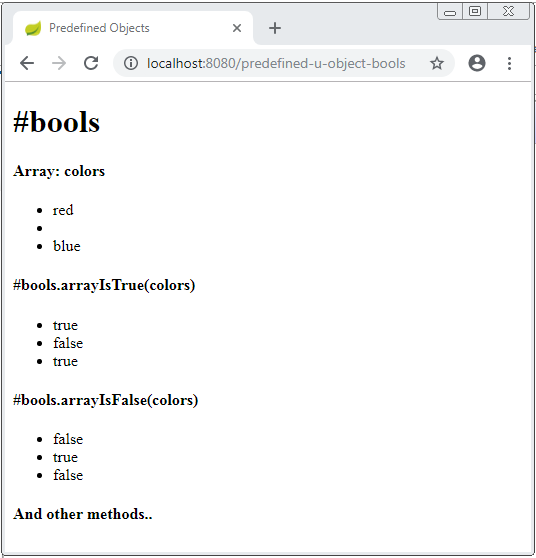
#arrays
Provide methods for arrays.
@RequestMapping("/predefined-u-object-arrays")
public String arrays_object(Model model) {
String[] colors = new String[] {"red", null , "blue"};
model.addAttribute("colors", colors);
return "predefined-u-object-arrays";
}
predefined-u-object-arrays.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#arrays</h1>
<h4>Array: colors</h4>
<ul>
<li th:each="color : ${colors}"
th:utext="${color}"></li>
</ul>
<h4>#arrays.isEmpty(colors)</h4>
<span th:utext="${#arrays.isEmpty(colors)}"></span>
<h4>#arrays.length(colors)</h4>
<span th:utext="${#arrays.length(colors)}"></span>
<h4>#arrays.contains(colors,'red')</h4>
<span th:utext="${#arrays.contains(colors,'red')}"></span>
<h4>And other methods..</h4>
</body>
</html>
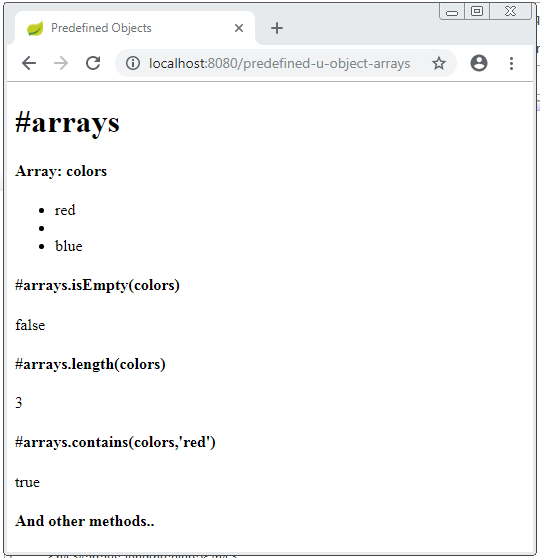
#lists
Provide methods for lists.
@RequestMapping("/predefined-u-object-lists")
public String lists_object(Model model) {
String[] array = new String[] {"red", "blue", "green"};
List<String> colors = Arrays.asList(array);
model.addAttribute("colors", colors);
return "predefined-u-object-lists";
}
predefined-u-object-lists.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#lists</h1>
<h4>List: colors</h4>
<ul>
<li th:each="color : ${colors}"
th:utext="${color}"></li>
</ul>
<h4>#lists.sort(colors)</h4>
<ul>
<li th:each="color : ${#lists.sort(colors)}"
th:utext="${color}"></li>
</ul>
<h4>#lists.isEmpty(colors)</h4>
<span th:utext="${#lists.isEmpty(colors)}"></span>
<h4>#lists.size(colors)</h4>
<span th:utext="${#lists.size(colors)}"></span>
<h4>#lists.contains(colors,'red')</h4>
<span th:utext="${#lists.contains(colors,'red')}"></span>
<h4>And other methods..</h4>
</body>
</html>
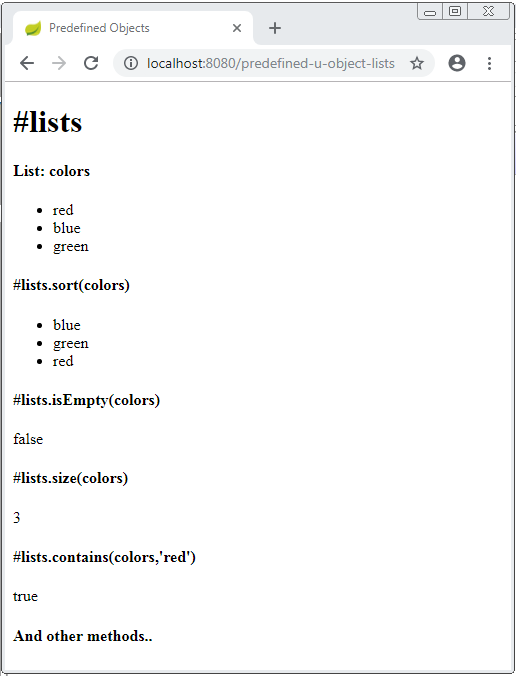
#sets
Provide methods for sets.
@RequestMapping("/predefined-u-object-sets")
public String sets_object(Model model) {
Set<String> colors = new HashSet<String>();
colors.add("red");
colors.add("blue");
colors.add("green");
model.addAttribute("colors", colors);
return "predefined-u-object-sets";
}
predefined-u-object-sets.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#sets</h1>
<h4>List: colors</h4>
<ul>
<li th:each="color : ${colors}"
th:utext="${color}"></li>
</ul>
<h4>#sets.isEmpty(colors)</h4>
<span th:utext="${#sets.isEmpty(colors)}"></span>
<h4>#sets.size(colors)</h4>
<span th:utext="${#sets.size(colors)}"></span>
<h4>#sets.contains(colors,'red')</h4>
<span th:utext="${#sets.contains(colors,'red')}"></span>
<h4>And other methods..</h4>
</body>
</html>
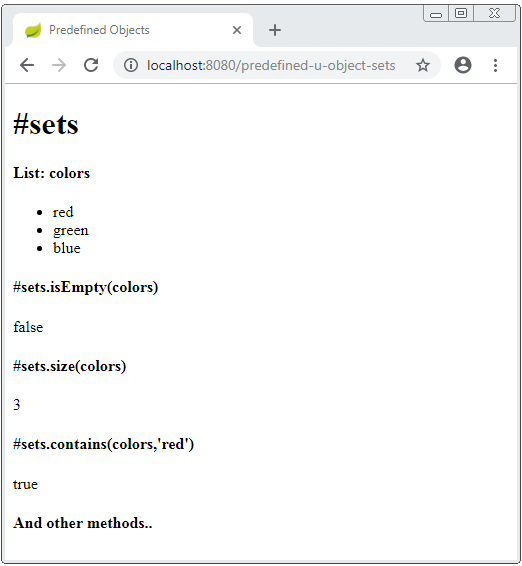
#maps
Provide methods for maps.
@RequestMapping("/predefined-u-object-maps")
public String maps_object(Model model) {
Map<String,String> contacts = new HashMap<String,String>();
contacts.put("111 222","Tom");
contacts.put("111 333","Jerry");
contacts.put("111 444","Donald");
model.addAttribute("contacts", contacts);
return "predefined-u-object-maps";
}
predefined-u-object-maps.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#maps</h1>
<h4>Map: contacts</h4>
<h4>#maps.isEmpty(contacts)</h4>
<span th:utext="${#maps.isEmpty(contacts)}"></span>
<h4>#maps.size(contacts)</h4>
<span th:utext="${#maps.size(contacts)}"></span>
<h4>And other methods..</h4>
</body>
</html>
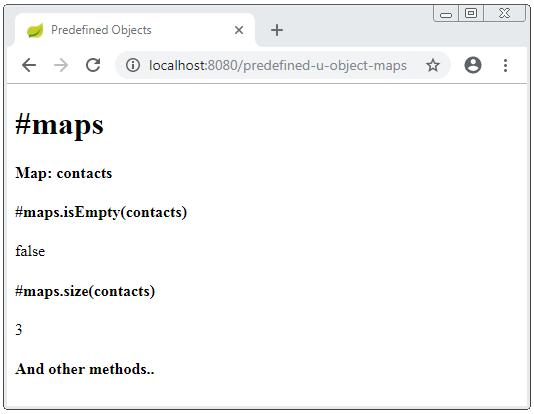
#aggregates
Cung cấp các phương thức để tính tổng, giá trị trung bình,.. trên một tập hợp (collection) hoặc một mảng (array).
#aggregates
@RequestMapping("/predefined-u-object-aggregates")
public String aggregates_object(Model model) {
double[] salaries = new double[] {100, 200, 500};
model.addAttribute("salaries", salaries);
return "predefined-u-object-aggregates";
}
predefined-u-object-aggregates.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Predefined Objects</title>
</head>
<body>
<h1>#aggregates</h1>
<h4>Array: salaries</h4>
<ul>
<li th:each="salary : ${salaries}"
th:utext="${salary}"></li>
</ul>
<h4>#aggregates.avg(salaries)</h4>
<span th:utext="${#aggregates.avg(salaries)}"></span>
<h4>#aggregates.sum(salaries)</h4>
<span th:utext="${#aggregates.sum(salaries)}"></span>
</body>
</html>
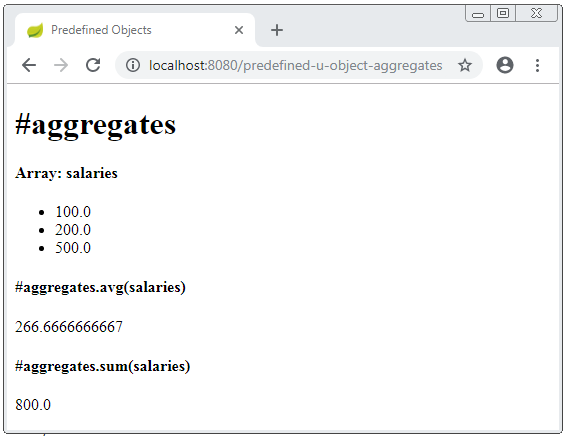
#ids
Thymeleaf Tutorials
- Thymeleaf Elvis Operator Tutorial with Examples
- Thymeleaf Loops Tutorial with Examples
- Thymeleaf Conditional statements: if, unless, switch
- Thymeleaf Predefined Objects Tutorial with Examples
- Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Introduction to Thymeleaf
- Thymeleaf Variables Tutorial with Examples
- Thymeleaf Fragments Tutorial with Examples
- Thymeleaf Page Layouts Tutorial with Examples
- Thymeleaf th:object and Asterisk Syntax *{ }
- Thymeleaf Form Select option Example
Show More