Thymeleaf Variables Tutorial with Examples
1. Variables
You are not strange to the concept of variable in the Java language, but what about it in Thymeleaf? Definitely, Thymeleaf also has the concept of variables.
An attribute of org.springframework.ui.Model object, or an attribute of HttpServletRequest object is a variable of Thymeleaf. This variable can be used everywhere in Template.
(Java Spring)
@RequestMapping("/variable-example1")
public String variableExample1(Model model, HttpServletRequest request) {
// variable1
model.addAttribute("variable1", "Value of variable1!");
// variable2
request.setAttribute("variable2", "Value of variable2!");
return "variable-example1";
}
variable-example1.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>Variables</h1>
<h4>${variable1}</h4>
<span th:utext="${variable1}"></span>
<h4>${variable2}</h4>
<span th:utext="${variable2}"></span>
</body>
</html>
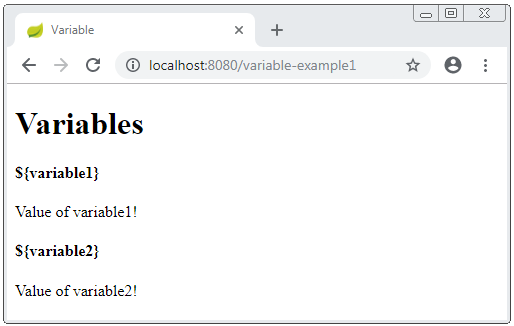
Local Variables
You can define variables in Template. They are local variables. They only exist and are available in a Template section.
In this example, two flower, iter variables only exist and are available within the loop that has declared them.
(Java Spring)
@RequestMapping("/variable-in-loop")
public String objectServletContext(Model model, HttpServletRequest request) {
String[] flowers = new String[] {"Rose","Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "variable-in-loop";
}
variable-in-loop.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>Variable: flower, iter</h1>
<table border="1">
<tr>
<th>No</th>
<th>Flower Name</th>
</tr>
<!--
Local Variable: flower
Local Variable: iter (Iterator).
-->
<tr th:each="flower, iter : ${flowers}">
<td th:utext="${iter.count}">No</td>
<td th:utext="${flower}">Flower Name</td>
</tr>
</table>
</body>
</html>
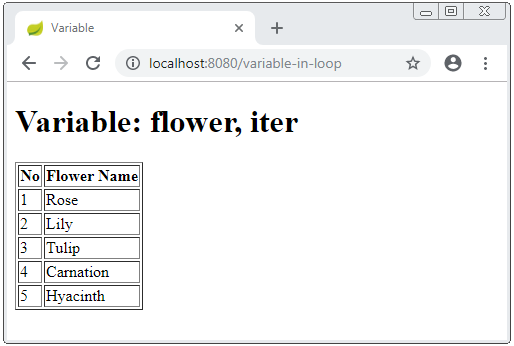
th:with
You can also create one or more local variables through the th:with attribute. Its syntax is like a regular "value assignment expression".
(Java Spring)
@RequestMapping("/variable-example3")
public String variableExample3(Model model) {
String[] flowers = new String[] {"Rose","Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "variable-example3";
}
variable-example3.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>th:with</h1>
<!-- Local variable: flower0 -->
<div th:with="flower0 = ${flowers[0]}">
<h4>${flower0}</h4>
<span th:utext="${flower0}"></span>
</div>
<!-- Local variable: flower1, flower2 -->
<div th:with="flower1 = ${flowers[1]}, flower2 = ${flowers[2]}">
<h4>${flower1}, ${flower2}</h4>
<span th:utext="${flower1}"></span>
<br/>
<span th:utext="${flower2}"></span>
</div>
<hr>
<!-- Local variable: firstName, lastName, fullName -->
<div th:with="firstName = 'James', lastName = 'Smith', fullName = ${firstName} +' ' + ${lastName}">
First Name: <span th:utext="${firstName}"></span>
<br>
Last Name: <span th:utext="${lastName}"></span>
<br>
Full Name: <span th:utext="${fullName}"></span>
</div>
</body>
</html>
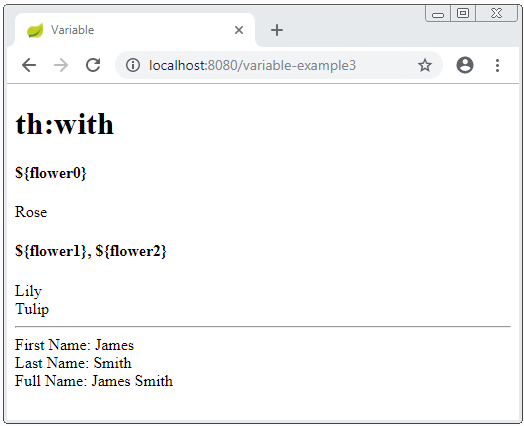
Example, create an array in the Thymeleaf:
variable-array-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>th:with (Array)</h1>
<!-- Create an Array: -->
<th:block th:with="flowers = ${ {'Rose', 'Lily', 'Tulip'} }">
<table border="1">
<tr>
<th>No</th>
<th>Flower</th>
</tr>
<tr th:each="flower, state : ${flowers}">
<td th:utext="${state.count}">No</td>
<td th:utext="${flower}">Flower</td>
</tr>
</table>
</th:block>
</body>
</html>
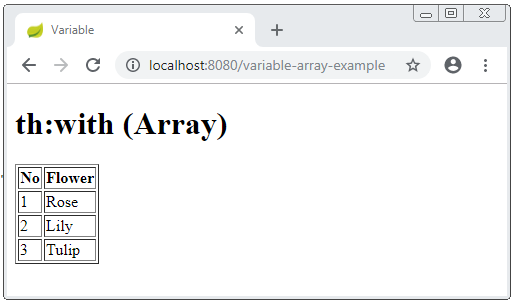
Thymeleaf Tutorials
- Thymeleaf Elvis Operator Tutorial with Examples
- Thymeleaf Loops Tutorial with Examples
- Thymeleaf Conditional statements: if, unless, switch
- Thymeleaf Predefined Objects Tutorial with Examples
- Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Introduction to Thymeleaf
- Thymeleaf Variables Tutorial with Examples
- Thymeleaf Fragments Tutorial with Examples
- Thymeleaf Page Layouts Tutorial with Examples
- Thymeleaf th:object and Asterisk Syntax *{ }
- Thymeleaf Form Select option Example
Show More