Understanding Spring Cloud Config Client with Example
1. The objective of the lesson
OK, we are in the serial of guiding for programming a distributed application using Spring Cloud. A Taxi Management Application. In the previous lesson, I showed you how to create a Config-Server application, which manages configuration information for other services.
See previous lesson:
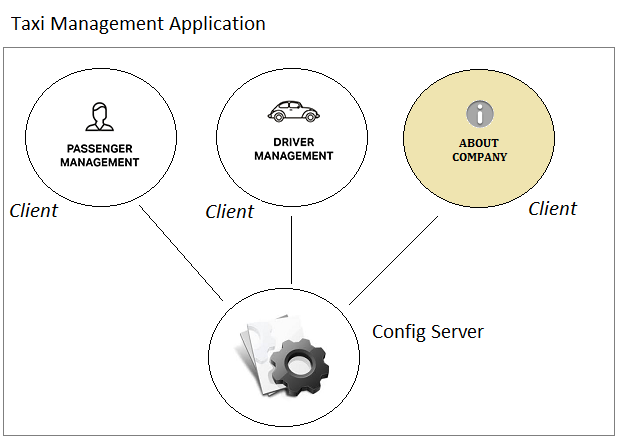
In this lesson, we are going to create an "About Company" application. It is independent of "Passenger Management" and "Diver Management" applications. For a big taxi company, for example, Uber, the number of users visiting their website is huge, it is a good idea to have an application to introduce the company separate from other functions.
The "About Company" application will use an independent database. Database configuration information will be managed on the Config-Server. In the Microservice architecture, this application is not simply a company introduction website; it can be a service that provides information for other applications, for example, provision of information on hotline, feedback email, etc. In brief, it is part of the Microservice system.
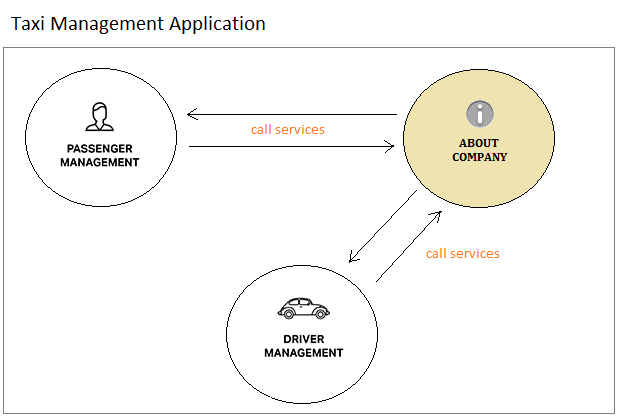
Note: The main objective of this lesson is to discuss and practise an example of "How an application can get its configuration information being managed on a Config-Server."
2. Create Spring Boot project
- Name: ConfigClientAboutCompany
- Group: org.o7planning
- Artifact: ConfigClientAboutCompany
- Description: Spring Cloud Config Client (About Company App)
- Package: org.o7planning.aboutcompany
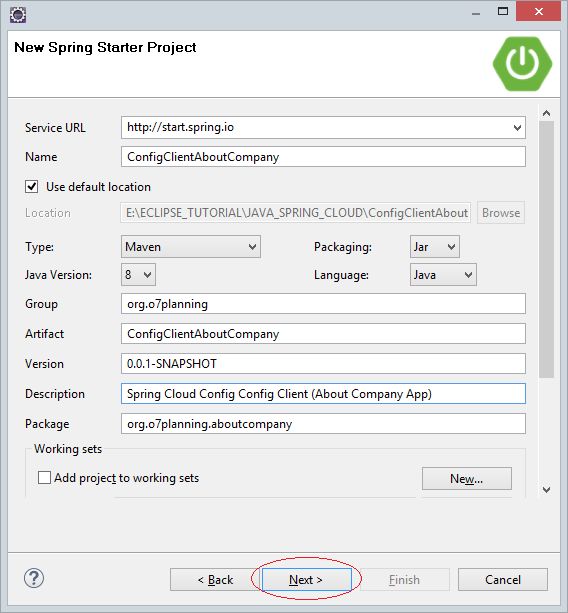
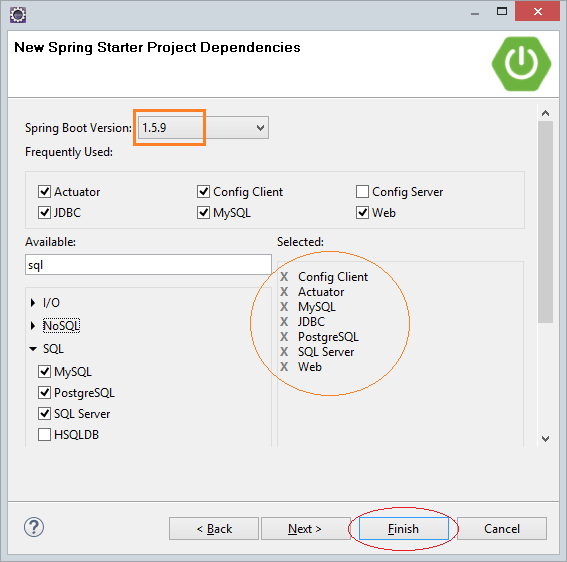
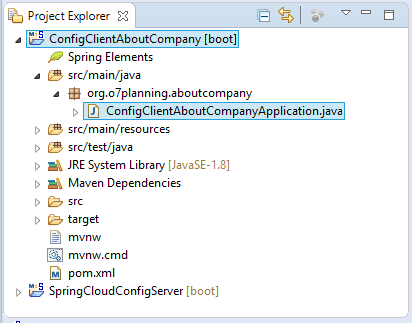
JDBC Driver (MySQL, PostGres,..)
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
<!-- SQL Server - Mssql-Jdbc driver -->
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId>
<scope>runtime</scope>
</dependency>
<!-- Oracle Driver -->
<dependency>
<groupId>com.oracle</groupId>
<artifactId>ojdbc6</artifactId>
<version>11.2.0.3</version>
</dependency>
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>ConfigClientAboutCompany</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>ConfigClientAboutCompany</name>
<description>Spring Cloud Config Config Client (About Company App)</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath/>
<!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
<spring-cloud.version>Edgware.RELEASE</spring-cloud.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
<!-- SQL Server - Mssql-Jdbc driver -->
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId>
<scope>runtime</scope>
</dependency>
<!-- Oracle Driver -->
<dependency>
<groupId>com.oracle</groupId>
<artifactId>ojdbc6</artifactId>
<version>11.2.0.3</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<repositories>
<!-- Repository for ORACLE JDBC Driver -->
<repository>
<id>codelds</id>
<url>https://code.lds.org/nexus/content/groups/main-repo</url>
</repository>
</repositories>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
ConfigClientAboutCompanyApplication.java
package org.o7planning.aboutcompany;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ConfigClientAboutCompanyApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigClientAboutCompanyApplication.class, args);
}
}
3. Configure Spring Cloud Config Client
This application needs to read its configurations stored on the Config-Server; therefore,you need to tell it the information on the Config-Server. This information is configured on the bootstrap.properties (or bootstrap.yml) file . This file is used very early by the application during the application startup.
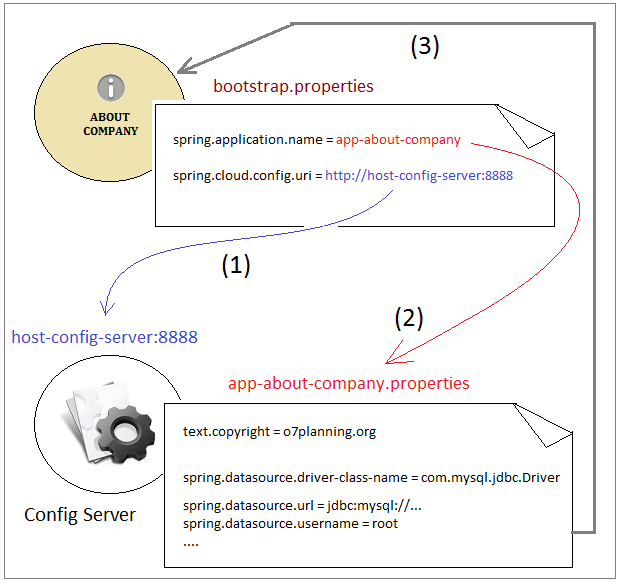
There is a slight difference in the format of the * .properties file and the * .yml file, and you can learn more in the following:
- properties vs yml
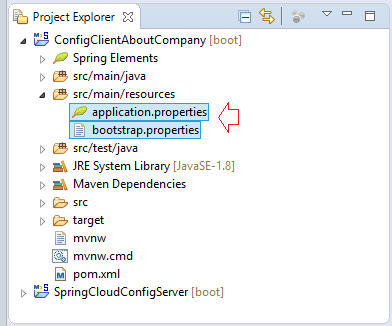
bootstrap.properties
# Read file on Config-Server:
# app-about-company.properties or app-about-company.yml
spring.application.name=app-about-company
# This is the default:
spring.cloud.config.uri=http://localhost:8888
management.security.enabled=false
Configure so that this application runs on port 7777:
application.properties
server.port=7777
Configuration file for this application (see on GitHub):
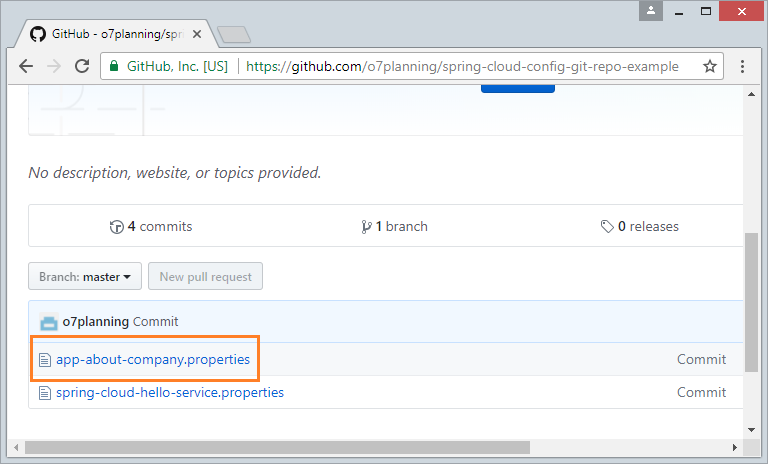
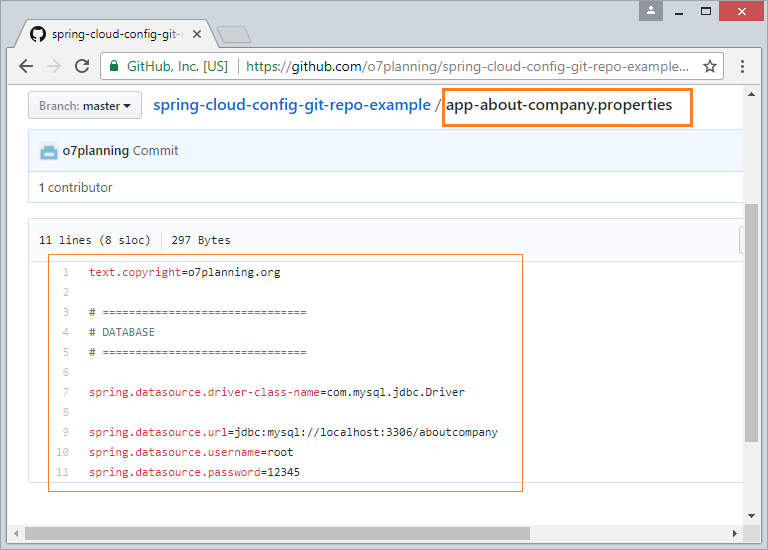
4. Controller
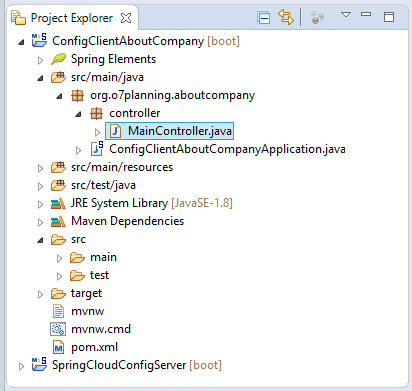
MainController.java
package org.o7planning.aboutcompany.controller;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
@RefreshScope
@RestController
public class MainController {
// https://github.com/o7planning/spring-cloud-config-git-repo-example
// See: app-about-company.properties
@Value("${text.copyright: Default Copyright}")
private String copyright;
@Value("${spring.datasource.driver-class-name}")
private String driverClassName;
@Value("${spring.datasource.url}")
private String url;
@Value("${spring.datasource.username}")
private String userName;
@Value("${spring.datasource.password}")
private String password;
@Autowired
private DataSource dataSource;
@RequestMapping("/showConfig")
@ResponseBody
public String showConfig() {
String configInfo = "Copy Right: " + copyright //
+ "<br/>spring.datasource.driver-class-name=" + driverClassName //
+ "<br/>spring.datasource.url=" + url //
+ "<br/>spring.datasource.username=" + userName //
+ "<br/>spring.datasource.password=" + password;
return configInfo;
}
@RequestMapping("/pingDataSource")
@ResponseBody
public String pingDataSource() {
try {
return this.dataSource.toString();
} catch (Exception e) {
e.printStackTrace();
return "Error: " + e.getMessage();
}
}
}
@RefreshScope:
Any Spring Bean annotated by @RefreshScope will be refreshed at runtime. And any components that are using them will get a new instance on the next method call, fully initialized and injected with all dependencies.
Spring Cloud Tutorials
- What is Cloud Computing?
- Introduction to Netflix and its cloud computing technology
- Introduction to Spring Cloud
- Understanding Spring Cloud Config Server with Example
- Understanding Spring Cloud Config Client with Example
- Understanding Spring Cloud Eureka Server with Example
- Understanding Spring Cloud Discovery Eureka Client with Example
- Undertanding load balancing in Spring Cloud with Ribbon and example
Show More