Java and MongoDB Tutorial with Examples
1. Some concepts of MongoDB
First of all, we need to understand some definitions in MongoDB:
- The definition of Collection in MongDB is equivalent to the definition of Table in relation database.
- The definition of Document in MongoDB is equivalent to the definition of Record in relation database.
MongoDB is very flexible. You can connect with a Database or Collection although they may not exist. They will be automatically created when you have some manipulation. This will be explained in related examples in this document.
2. The main objective
When you work with a database, the question is that how you can query, insert, update and delete data.
MongoDB has no definition of Join query between 2 Collections. Everything relating to query is executed on a Collection. Thus, you do not need to learn how to Join 2 Collections together. The remaining thing is to write the where condition to a Collection. Particularly:
MongoDB has no definition of Join query between 2 Collections. Everything relating to query is executed on a Collection. Thus, you do not need to learn how to Join 2 Collections together. The remaining thing is to write the where condition to a Collection. Particularly:
- Write the query conditions on one Collection.
- Write a condition to update data on one Collection.
- Write a condition to delete Documents on one Collection.
Java provides a DBObject class to describe a JSON data structure, and it describes a condition.
Let's look at some examples:
To find employees whose first_name= "John" and last_name = "Smith", JSON in this case would be:
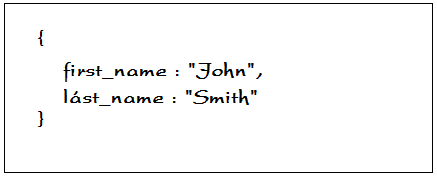
To find employees whose department ID is 10 and employee number are "E01" or "E02", the JSON structure you need to write is:
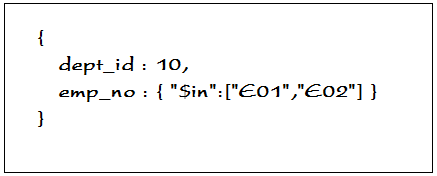
You need to learn how Java API creates DBObject that describes a conditional sentence to Query statement. Java provides you some classes:
- BasicDBObjectBuilder
- QueryBuilder
The above classes are utility classes to create DBObject object.
3. Create Project
Firstly you need to create a Project to practice examples.
- File/New/Other...
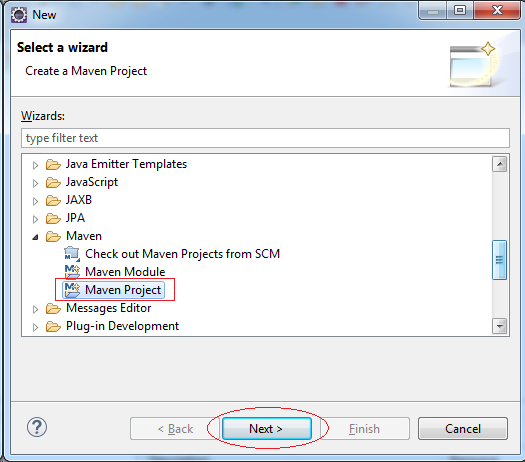
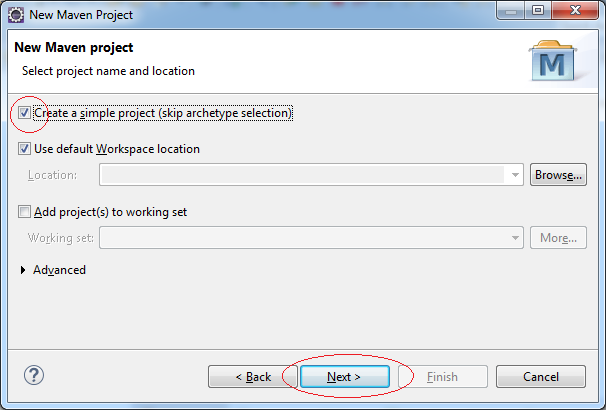
Enter:
- Group Id: org.o7planning
- Artifact Id: JavaMongoDBTutorial
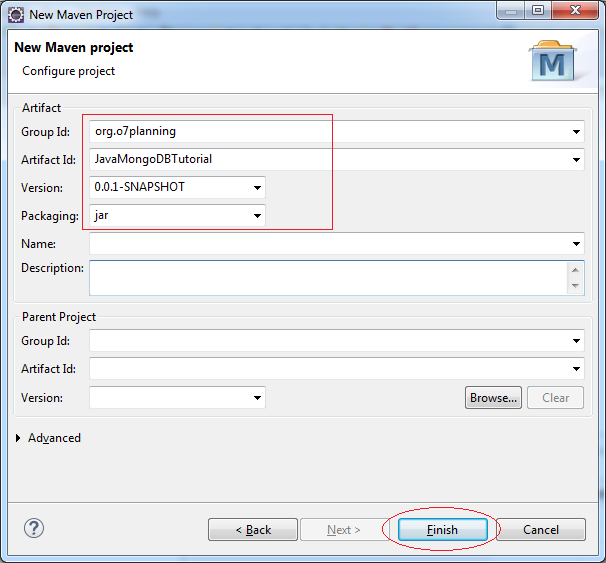
Project was created:
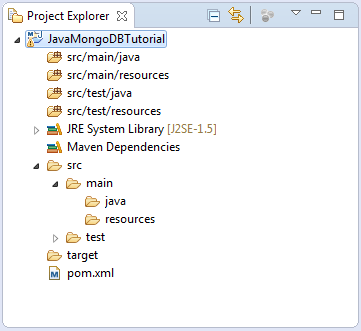
4. Declaring library (using Maven)
Configure pom.xml
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>JavaMongoDBTutorial</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<!-- http://mvnrepository.com/artifact/org.mongodb/mongo-java-driver -->
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongo-java-driver</artifactId>
<version>2.12.4</version>
</dependency>
</dependencies>
</project>
5. Connect to MongoDB using Java
Ensure that your MongoDB is ready to run.
You can see also install and configure MongoDB at:
MyConstants.java
package org.o7planning.tutorial.mongodb;
public class MyConstants {
// This database may be not exist on your MongoDB.
/// But it will be automatically created.
// (You do not need to change this field).
public static final String DB_NAME ="MyStudyDB";
}
MongoUtils with utility method to connect to the MongoDB. In practice you can change the parameters HOST, PORT, .. to suit your circumstances.
MongoUtils.java
package org.o7planning.tutorial.mongodb;
import java.net.UnknownHostException;
import java.util.Arrays;
import java.util.List;
import com.mongodb.MongoClient;
import com.mongodb.MongoCredential;
import com.mongodb.ServerAddress;
public class MongoUtils {
private static final String HOST = "localhost";
private static final int PORT = 27017;
//
private static final String USERNAME = "mgdb";
private static final String PASSWORD = "1234";
// connect to MongoDB is not mandatory security.
private static MongoClient getMongoClient_1() throws UnknownHostException {
MongoClient mongoClient = new MongoClient(HOST, PORT);
return mongoClient;
}
// connect to the DB MongoDB with security.
private static MongoClient getMongoClient_2() throws UnknownHostException {
MongoCredential credential = MongoCredential.createMongoCRCredential(
USERNAME, MyConstants.DB_NAME, PASSWORD.toCharArray());
MongoClient mongoClient = new MongoClient(
new ServerAddress(HOST, PORT), Arrays.asList(credential));
return mongoClient;
}
public static MongoClient getMongoClient() throws UnknownHostException {
// Connect to MongoDB is not mandatory security.
return getMongoClient_1();
// You can replace by getMongoClient_2 ()
// in case of connection to MongoDB with security.
}
private static void ping() throws UnknownHostException {
MongoClient mongoClient = getMongoClient();
System.out.println("List all DB:");
// Get database names
List<String> dbNames = mongoClient.getDatabaseNames();
for (String dbName : dbNames) {
System.out.println("+ DB Name: " + dbName);
}
System.out.println("Done!");
}
// Test
public static void main(String[] args) throws UnknownHostException {
ping();
}
}
Running MongoUtils class to test the connection:
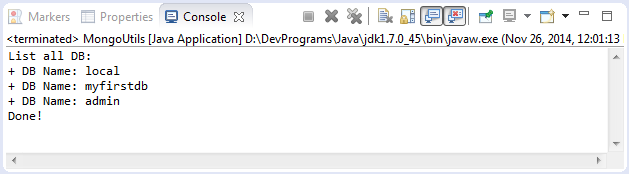
6. Insert Collection
MongoDB is very flexible, if you want to insert a Document into a Collection. If Collection this does not exist, it will be created automatically, then insert Document into
In this example, we will insert 4 documents into Department Collection. In MongoDB_id column is the primary key, in case you insert a document without specifying the primary key, columns _id will be created, with a value of a random string 36 characters.
{
"_id" : 10 ,
"dept_no" : "D10" ,
"dept_name" : "ACCOUNTING" ,
"location" : "NEW YORK"
}
{
"_id" : 20 ,
"dept_no" : "D20" ,
"dept_name" : "RESEARCH" ,
"location" : "DALLAS" ,
"description" : "First department"
}
{
"_id" : 30 ,
"dept_no" : "D30" ,
"dept_name" : "SALES" ,
"location" : "CHICAGO"
}
{
"_id" : 40 ,
"dept_no" : "D40" ,
"dept_name" : "OPERATIONS" ,
"location" : "BOSTON"
}
InsertDemo.java
package org.o7planning.tutorial.mongodb.insert;
import java.net.UnknownHostException;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBObject;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.MongoClient;
public class InsertDemo {
public static void main(String args[]) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
// No need to be an existing DB
// it will be automatically created if not exists
DB db = mongoClient.getDB(MyConstants.DB_NAME);
// Get the Collection with name Department
// Not necessarily this 'Collection' must exist in the DB.
DBCollection dept = db.getCollection("Department");
// Insert Document 1
BasicDBObject doc1 = new BasicDBObject();
doc1.append("_id", 10);
doc1.append("dept_no", "D10");
doc1.append("dept_name", "ACCOUNTING");
doc1.append("location", "NEW YORK");
dept.insert(doc1);
// Insert Document 2
BasicDBObject doc2 = new BasicDBObject();
doc2.append("_id", 20);
doc2.append("dept_no", "D20");
doc2.append("dept_name", "RESEARCH");
doc2.append("location", "DALLAS");
doc2.append("description", "First department");
dept.insert(doc2);
// Insert Document 3
BasicDBObject doc3 = new BasicDBObject();
doc3.append("_id", 30);
doc3.append("dept_no", "D30");
doc3.append("dept_name", "SALES");
doc3.append("location", "CHICAGO");
dept.insert(doc3);
// Insert Document 4
BasicDBObject doc4 = new BasicDBObject();
doc4.append("_id", 40);
doc4.append("dept_no", "D40");
doc4.append("dept_name", "OPERATIONS");
doc4.append("location", "BOSTON");
dept.insert(doc4);
System.out.println("Done!");
}
}
Running InsertDemo class and see the results on RoboMongo (visual management tool for MongoDB).
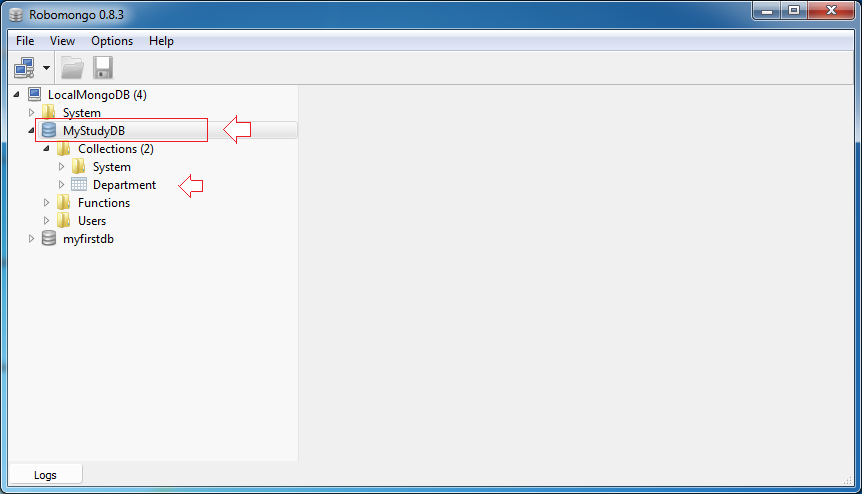
If the database does not exist, it will be created. If Collection does not exist it is created automatically. That is a difference of MongoDB with other types of relational database (Oracle, MySQL, SQLServer ...)
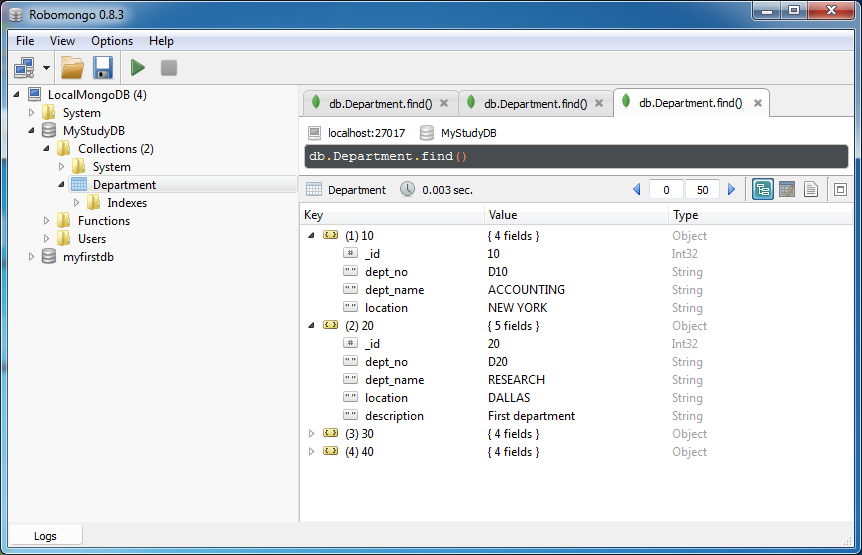
7. Collection
Collection in MongoDB corresponding Table concept in the Relational database. We see an example which retrieves a list of Collection in the database:
GettingCollectionDemo.java
package org.o7planning.tutorial.mongodb.collection;
import java.net.UnknownHostException;
import java.util.Set;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.MongoClient;
public class GettingCollectionDemo {
public static void main(String[] args) throws UnknownHostException {
MongoClient mongoClient = MongoUtils.getMongoClient();
DB db = mongoClient.getDB(MyConstants.DB_NAME);
// Collection in MongoDB corresponding to one Table
// in the relational database.
DBCollection dept = db.getCollection("Department");
System.out.println("Collection: "+ dept);
// Document count.
// Note: Document in MongoDB corresponding to one record
// in the relational database.
long rowCount = dept.count();
System.out.println(" Document count: "+ rowCount);
System.out.println(" ------------ ");
// List of Collections
Set<String> collections = db.getCollectionNames();
for(String coll: collections) {
System.out.println("Collection: "+ coll);
}
}
}
Results of running the example:
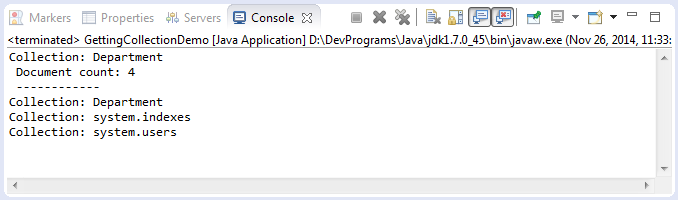
8. Query Collection
Conditions queries in MongoDB
Let's see some cases that set where condition (This is purely knowledge of MongoDB)
Java API for querying data
// Some methods of DBCollection, for query:
public DBCursor find(DBObject ref)
public DBCursor find(DBObject ref, DBObject keys)
A simple query example
Query Department Collection:
SimpleQueryDemo.java
package org.o7planning.tutorial.mongodb.query;
import java.net.UnknownHostException;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBCursor;
import com.mongodb.MongoClient;
public class SimpleQueryDemo {
public static void main(String args[]) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
DBCollection dept = db.getCollection("Department");
// Query
DBCursor cursor = dept.find();
int i = 1;
while (cursor.hasNext()) {
System.out.println("Document: " + i);
System.out.println(cursor.next());
i++;
}
}
}
Results of running the example:
Document: 1
{ "_id" : 10 , "dept_no" : "D10" , "dept_name" : "ACCOUNTING" , "location" : "NEW YORK"}
Document: 2
{ "_id" : 20 , "dept_no" : "D20" , "dept_name" : "RESEARCH" , "location" : "DALLAS" , "description" : "First department"}
Document: 3
{ "_id" : 30 , "dept_no" : "D30" , "dept_name" : "SALES" , "location" : "CHICAGO"}
Document: 4
{ "_id" : 40 , "dept_no" : "D40" , "dept_name" : "OPERATIONS" , "location" : "BOSTON"}
Query Example with conditions (1)
To create the DBObject object, you can use BasicDBObjectBuilder, for example:
BasicDBObjectBuilder whereBuilder = BasicDBObjectBuilder.start();
whereBuilder.append("dept_name", "ACCOUNTING");
//
DBObject where = whereBuilder.get();
// Query
DBCursor cursor = dept.find(where);
See the example query Department, find departments has dept_name = 'ACCOUNTING'.
QueryWithParamsDemo1.java
package org.o7planning.tutorial.mongodb.query;
import java.net.UnknownHostException;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBObjectBuilder;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBCursor;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
public class QueryWithParamsDemo1 {
// Building JSON:
// { "dept_name" : "ACCOUNTING"}
private static DBObject getWhereClause_1() {
BasicDBObjectBuilder whereBuilder = BasicDBObjectBuilder.start();
// Use the append method (similar to the use of add method)
whereBuilder.append("dept_name", "ACCOUNTING");
//
DBObject where = whereBuilder.get();
System.out.println("Where: " + where.toString());
return where;
}
public static void main(String args[]) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
DBCollection dept = db.getCollection("Department");
DBObject where = getWhereClause_1();
// Query
DBCursor cursor = dept.find(where);
int i = 1;
while (cursor.hasNext()) {
System.out.println("Document: " + i);
System.out.println(cursor.next());
i++;
}
System.out.println("Done!");
}
}
Results of running the example:
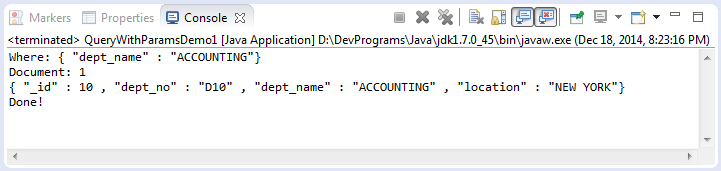
Query Example with conditions (2)
The next example uses BasicDBObjectBuilder. You can easily know how to use it according to the illustration below:
Note: The add method similar to the append method.
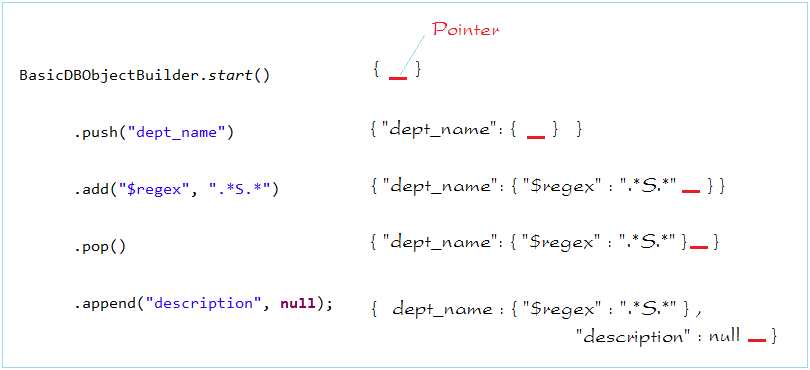
QueryWithParamsDemo2.java
package org.o7planning.tutorial.mongodb.query;
import java.net.UnknownHostException;
import java.util.regex.Pattern;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBObjectBuilder;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBCursor;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
//
// Find Department like SQL:
// where dept_name like '%S%' and description is null
//
public class QueryWithParamsDemo2 {
// dept_name like '%S%' and description is null
// Building JSON:
// { "dept_name" : { "$regex" : ".*S.*"} , "description" : null }
public static DBObject getWhereClause_1() {
BasicDBObjectBuilder whereBuilder = BasicDBObjectBuilder.start();
// Using append (same as 'add')
whereBuilder.push("dept_name").add("$regex", ".*S.*") ;
whereBuilder.pop();
whereBuilder.append("description", null);
//
DBObject where = whereBuilder.get();
System.out.println("Where " + where.toString());
return where;
}
// dept_name like '%S%' and description is null
// Building JSON:
// { "dept_name" : { "$regex" : ".*S.*"} , "description" : null }
public static DBObject getWhereClause_2() {
BasicDBObjectBuilder whereBuilder = BasicDBObjectBuilder.start();
// Regular expression describes a string
// started by any characters appear 0 or more times
// next by 'S'
// and any character appear 0 or more times
String regex = ".*S.*";
Pattern pattern = Pattern.compile(regex);
whereBuilder.append("dept_name", pattern);
whereBuilder.append("description", null);
//
DBObject where = whereBuilder.get();
System.out.println("Where: " + where.toString());
return where;
}
public static void main(String args[]) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
DBCollection dept = db.getCollection("Department");
DBObject where = getWhereClause_1();
// Query
DBCursor cursor = dept.find(where);
int i = 1;
while (cursor.hasNext()) {
System.out.println("Document: " + i);
System.out.println(cursor.next());
i++;
}
System.out.println("Done!");
}
}
Results of running the example:
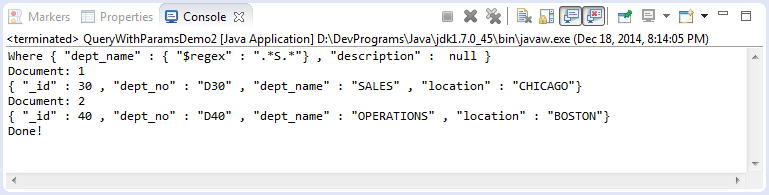
Query Example with conditions (3)
QueryWithParamsDemo3.java
package org.o7planning.tutorial.mongodb.query;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBList;
import com.mongodb.BasicDBObjectBuilder;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBCursor;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
import com.mongodb.QueryBuilder;
//
// Fine Department like SQL:
// where dept_name in ('ACCOUNTING', 'RESEARCH') or location = 'BOSTON'.
//
public class QueryWithParamsDemo3 {
// Option 1:
//
// dept_name in ('ACCOUNTING', 'RESEARCH') or location = 'BOSTON'.
// { "$or" : [ { "dept_name" : { "$in" : [ "ACCOUNTING" , "RESEARCH"]}} , {"location" : "BOSTON"}]}
protected static DBObject getWhereClase_1() {
List<String> list = new ArrayList<String>();
list.add("ACCOUNTING");
list.add("RESEARCH");
//
BasicDBObjectBuilder ob1 = BasicDBObjectBuilder.start();
ob1.push("dept_name").add("$in", list);
DBObject clause1 = ob1.get();
//
BasicDBObjectBuilder ob2 = BasicDBObjectBuilder.start();
ob2.append("location", "BOSTON");
DBObject clause2 = ob2.get();
//
BasicDBList or = new BasicDBList();
or.add(clause1);
or.add(clause2);
//
BasicDBObjectBuilder builder = BasicDBObjectBuilder.start();
builder.add("$or", or);
DBObject query = builder.get();
System.out.println("Query = " + query);
return query;
}
// Option 2:
// Using QueryBuilder:
// dept_name in ('ACCOUNTING', 'RESEARCH') or location = 'BOSTON'.
// { "$or" : [ { "dept_name" : { "$in" : [ "ACCOUNTING" , "RESEARCH"]}} , {"location" : "BOSTON"}]}
protected static DBObject getWhereClause_2() {
List<String> list = new ArrayList<String>();
list.add("ACCOUNTING");
list.add("RESEARCH");
//
QueryBuilder qb1 = new QueryBuilder();
qb1.put("dept_name").in(list);
DBObject q1 = qb1.get();
//
QueryBuilder qb2 = new QueryBuilder();
qb2.put("location").is("BOSTON");
DBObject q2 = qb2.get();
//
QueryBuilder queryBuilder = QueryBuilder.start();
queryBuilder.or(q1, q2);
DBObject query = queryBuilder.get();
return query;
}
// Option 3 (brief for option 2):
// Using QueryBuilder.
// dept_name in ('ACCOUNTING', 'RESEARCH') or location = 'BOSTON'.
// { "$or" : [ { "dept_name" : { "$in" : [ "ACCOUNTING" , "RESEARCH"]}} , {"location" : "BOSTON"}]}
protected static DBObject getWhereClause_3() {
List<String> list = new ArrayList<String>();
list.add("ACCOUNTING");
list.add("RESEARCH");
//
QueryBuilder queryBuilder = QueryBuilder.start();
queryBuilder.or(QueryBuilder.start().put("dept_name").in(list).get(),
QueryBuilder.start().put("location").is("BOSTON").get());
DBObject query = queryBuilder.get();
return query;
}
public static void main(String args[]) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
DBCollection dept = db.getCollection("Department");
DBObject query = getWhereClase_1();
DBCursor cursor = dept.find(query);
int i = 1;
while (cursor.hasNext()) {
System.out.println("Document: " + i);
System.out.println(cursor.next());
i++;
}
System.out.println("Done!");
}
}
Results of running the example:
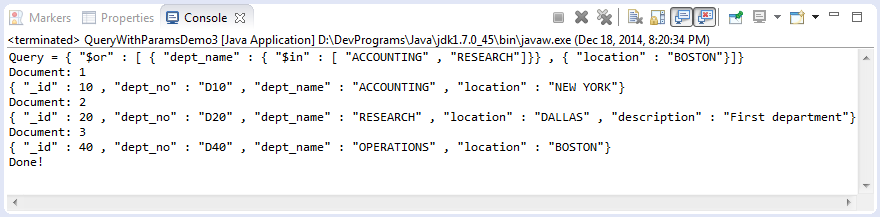
9. Update Document
First of all, we need to create some data to test. You need to run the following CreateDataForUpdate class to get data.
CreateDataForUpdate.java
package org.o7planning.tutorial.mongodb.update;
import java.net.UnknownHostException;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBObject;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.MongoClient;
public class CreateDataForUpdate {
public static void main(String[] args) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
// Retrieving Collection named City.
// This Collection not necessarily have to exist in the DB.
DBCollection city = db.getCollection("City");
String[] cityNos = new String[] { "CHI", "NYO", "WAS" };
String[] cityNames = new String[] { "Chicago", "New York", "Washington" };
int[] populations = new int[] { 3000000, 8000000, 1000000 };
String[] descriptions = new String[] { "Pop 2013", null, "Pop 2013"};
// Insert some Document into Collection.
for (int i = 0; i < 3; i++) {
BasicDBObject doc = new BasicDBObject();
doc.append("_id", i);
doc.append("city_no", cityNos[i]);
doc.append("city_name", cityNames[i]);
doc.append("population", populations[i]);
if (descriptions[i] != null) {
doc.append("description", descriptions[i]);
}
city.insert(doc);
}
System.out.println("Done!");
}
}
Results of running example,collection City was created with 3 document (See on visualization tools RoboMongo).
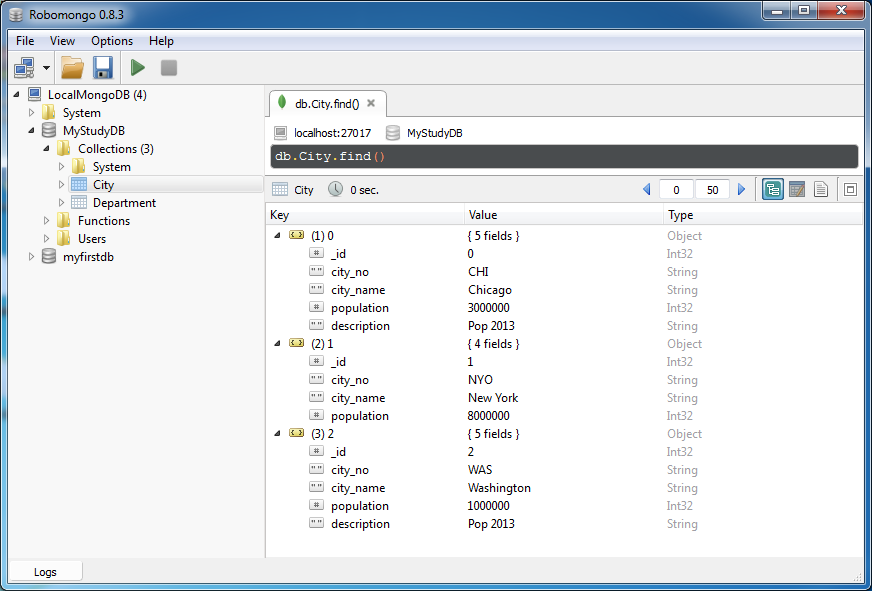
Update Example
UpdateDemo.java
package org.o7planning.tutorial.mongodb.update;
import java.net.UnknownHostException;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBObject;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
import com.mongodb.WriteResult;
public class UpdateDemo {
public static void main(String[] args) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
// Lấy ra Collection với tên City.
DBCollection city = db.getCollection("City");
// Find City has city_no ='WAS'
DBObject whereClause = new BasicDBObject("city_no", "WAS");
DBObject values = new BasicDBObject();
values.put("population", 1200000);
values.put("description", "Pop 2014");
values.put("note", "Document replaced!");
// Execute update.
WriteResult result = city.update(whereClause, values);
int effectedCount = result.getN();
System.out.println("Effected Count: " + effectedCount);
System.out.println("Done!");
}
}
Results of running examples and compare before and after the update document:
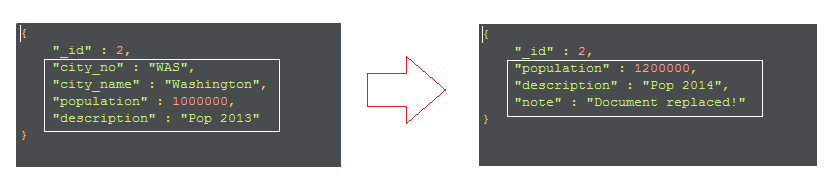
Look at the above result, you realize it is not the way of update as you think. In other words, it is Replacement.
You thought it should be:
You thought it should be:
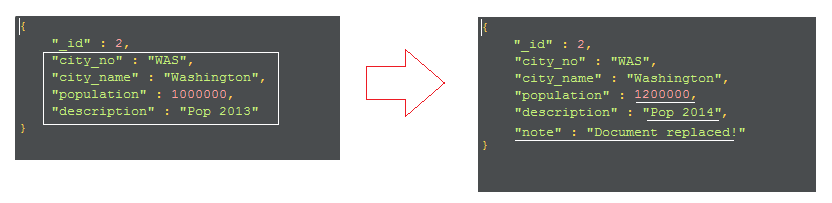
You need to add $set in update. Let's see the next example.
Update example with $set
UpdateWithSetDemo.java
package org.o7planning.tutorial.mongodb.update;
import java.net.UnknownHostException;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBObject;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
import com.mongodb.WriteResult;
public class UpdateWithSetDemo {
public static void main(String[] args) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
// Get Collection with name 'City'
DBCollection city = db.getCollection("City");
// Get City has city_no = 'CHI'
DBObject whereClause = new BasicDBObject("city_no", "CHI");
DBObject values = new BasicDBObject();
values.put("population", 3400000);
values.put("description", "Pop 2014");
values.put("note", "Document update with $set");
DBObject valuesWithSet = new BasicDBObject();
valuesWithSet.put("$set", values);
// Execute update.
WriteResult result = city.update(whereClause, valuesWithSet);
int effectedCount = result.getN();
System.out.println("Effected Count: " + effectedCount);
System.out.println("Done!");
}
}
Results of update:
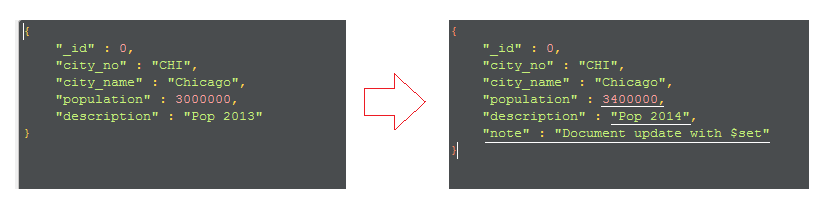
Update example with $inc
Use $inc if you want to increase the value of some column. For example, find City and update column population increased by 10000.
UpdateWithIncDemo.java
package org.o7planning.tutorial.mongodb.update;
import java.net.UnknownHostException;
import org.o7planning.tutorial.mongodb.MongoUtils;
import org.o7planning.tutorial.mongodb.MyConstants;
import com.mongodb.BasicDBObject;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
import com.mongodb.WriteResult;
public class UpdateWithIncDemo {
public static void main(String[] args) throws UnknownHostException {
// To connect to mongodb server
MongoClient mongoClient = MongoUtils.getMongoClient();
// Now connect to your databases
DB db = mongoClient.getDB(MyConstants.DB_NAME);
// Lấy ra Collection với tên City.
DBCollection city = db.getCollection("City");
// Find City has city_no = 'NYO'
DBObject whereClause = new BasicDBObject("city_no", "NYO");
DBObject values = new BasicDBObject();
values.put("population", 10000);
DBObject valuesWithInc = new BasicDBObject();
valuesWithInc.put("$inc", values);
// Execute update.
WriteResult result = city.update(whereClause, valuesWithInc);
int effectedCount = result.getN();
System.out.println("Effected Count: " + effectedCount);
System.out.println("Done!");
}
}
Results of update:

Update example with $set and $inc
Example:
DBObject values1 = new BasicDBObject();
values1.put("population", 10000);
DBObject values2 = new BasicDBObject();
values2.put("description", "Pop 2014");
DBObject valuesSI = new BasicDBObject();
valuesSI.put("$inc", values1);
valuesSI.put("$set", values2);
WriteResult result = city.update(whereClause, valuesSI);
Update multiple documents
Using method:
// DBCollection class:
public WriteResult updateMulti( DBObject query , DBObject value )