AngularJS Events Tutorial with Examples
1. Overview of AngularJS Events
AngularJS is a Javascript library therefore, all event handling ways of the Javascript can apply in the AngularJS. However, the AngularJS proposes a new approach. When an event occurs, a method of $scope will be called instead of a function of Javascript. To do this, the AngularJS provides a list of built-in Directives:
- ng-blur
- ng-change
- ng-click
- ng-copy
- ng-cut
- ng-dblclick
- ng-focus
- ng-keydown
- ng-keypress
- ng-keyup
- ng-mousedown
- ng-mouseenter
- ng-mouseleave
- ng-mousemove
- ng-mouseover
- ng-mouseup
- ng-paste
Note: An event of AngularJS will not overwrite the event of HTML. Both execute, therefore, you need to consider when writing codes like below:
<!-- Both events are executed -->
<button onclick="someFunction(event)" ng-click="someMethod($event)">
Click me!
</button>
2. Mouse Events
When an user moves the mouse across the surface of an element, events occur in the following order:
- ng-mouseover
- ng-mouseenter
- ng-mousemove
- ng-mouseleave
When an user clicks on elements, the following events will happen in the order:
- ng-mousedown
- ng-mouseup
- ng-click
Example with ng-mouseover, ng-mouseenter, ng-mousemove, ng-mouseleave:
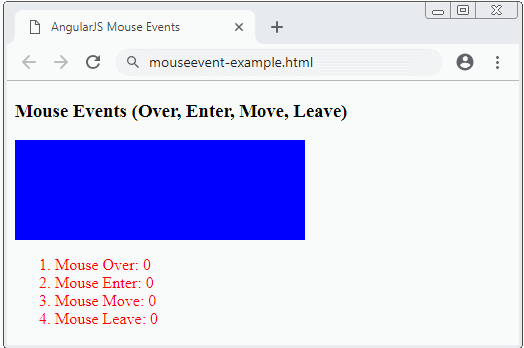
mouseevent-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Mouse Events</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="mouseevent-example.js"></script>
<style>
.my-div {
width: 290px;
height: 100px;
background: blue;
}
</style>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Mouse Events (Over, Enter, Move, Leave)</h3>
<div class="my-div"
ng-mouseover ="mouseoverHandler($event)"
ng-mouseenter ="mouseenterHandler($event)"
ng-mousemove ="mousemoveHandler($event)"
ng-mouseleave ="mouseleaveHandler($event)">
</div>
<ol style="color:red;">
<li>Mouse Over: {{overCount}}</li>
<li>Mouse Enter: {{enterCount}}</li>
<li>Mouse Move: {{moveCount}}</li>
<li>Mouse Leave: {{leaveCount}}</li>
</ol>
</div>
</body>
</html>
mouseevent-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.overCount = 0;
$scope.enterCount = 0;
$scope.moveCount = 0;
$scope.leaveCount = 0;
$scope.mouseoverHandler = function($event) {
$scope.overCount += 1;
}
$scope.mouseenterHandler = function($event) {
$scope.enterCount += 1;
}
$scope.mousemoveHandler = function($event) {
$scope.moveCount += 1;
}
$scope.mouseleaveHandler = function($event) {
$scope.leaveCount += 1;
}
});
Example with ng-mousedown, ng-mouseup, ng-click:
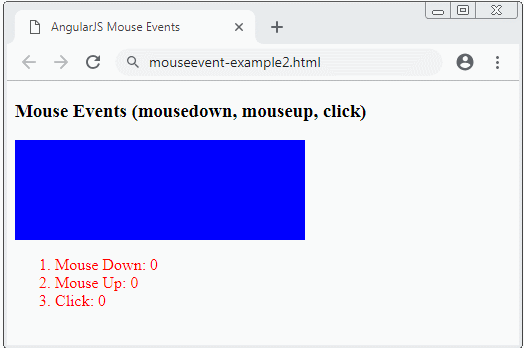
mouseevent-example2.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Mouse Events</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="mouseevent-example2.js"></script>
<style>
.my-div {
width: 290px;
height: 100px;
background: blue;
}
</style>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Mouse Events (mousedown, mouseup, click)</h3>
<div class="my-div"
ng-mousedown ="mousedownHandler($event)"
ng-mouseup ="mouseupHandler($event)"
ng-click ="clickHandler($event)">
</div>
<ol style="color:red;">
<li>Mouse Down: {{mousedownCount}}</li>
<li>Mouse Up: {{mouseupCount}}</li>
<li>Click: {{clickCount}}</li>
</ol>
</div>
</body>
</html>
mouseevent-example2.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.mousedownCount = 0;
$scope.mouseupCount = 0;
$scope.clickCount = 0;
$scope.mousedownHandler = function($event) {
$scope.mousedownCount += 1;
}
$scope.mouseupHandler = function($event) {
$scope.mouseupCount += 1;
}
$scope.clickHandler = function($event) {
$scope.clickCount += 1;
}
});
AngularJS Tutorials
- Introduction to AngularJS and Angular
- AngularJS Directive Tutorial with Examples
- AngularJS Model Tutorial with Examples
- AngularJS Filters Tutorial with Examples
- AngularJS Events Tutorial with Examples
- Quickstart with AngularJS - Hello AngularJS
- AngularJS Validation Tutorial with Examples
Show More