AngularJS Model Tutorial with Examples
1. ngModel Directive
ngModel is a built-in Directive of AngularJS. It defines a new property such as ng-model, which can apply to the HTML Controls (input, textarea, select). This Directive helps two-way binding between the values of HTML Control and application data.
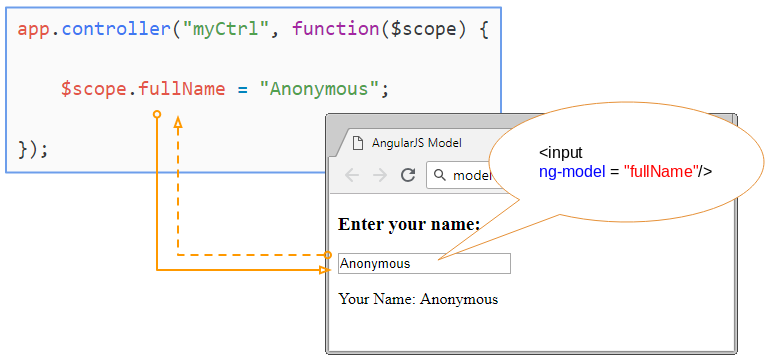
OK, a simple example. In this example, I will perform the two-way binding between the value of a <input> and $scope.fullName. This means if the value of <input> changes, it will be updated into the $scope.fullName, and vice versa if the $ scope.fullName value changes it will be updated to <input>.
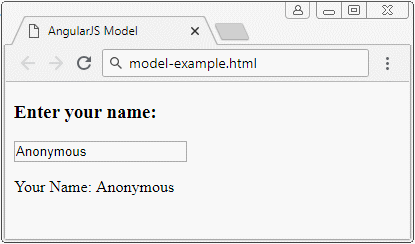
model-example.js
// Create an Application named "myApp".
var app = angular.module("myApp", []);
// Create a Controller named "myCtrl"
app.controller("myCtrl", function($scope) {
$scope.fullName = "Anonymous";
});
model-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Model</title>
<!-- Check version: https://code.angularjs.org/ -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="model-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Enter your name:</h3>
<p><input ng-model = "fullName"/></p>
<p>Your Name: {{fullName}}</p>
</div>
</body>
</html>
AngularJS Tutorials
- Introduction to AngularJS and Angular
- AngularJS Directive Tutorial with Examples
- AngularJS Model Tutorial with Examples
- AngularJS Filters Tutorial with Examples
- AngularJS Events Tutorial with Examples
- Quickstart with AngularJS - Hello AngularJS
- AngularJS Validation Tutorial with Examples
Show More