AngularJS Directive Tutorial with Examples
1. What is Directive?
What is the Directive in AngularJS? There are many answers to this question that you can find on Google. But it's too lengthy, and I like a shorter answer.
- Directive is an instruction for you to create a "TheNew". "TheNew" herein can be a new tag or create a new attibute for a tag, or a new CSS tag, or a Comment .
- And Directive shows AngularJS how to convert "The New" into HTML code that the browser can understand.
Example, I create a Directive called "helloWorld" to define a new <hello-world> tag, and I use this tag in HTML file. A browser obviously does not understand this tag, but when the file is run in the browser, AngularJS will rely on the above Directive instructions to convert the <hello-world> tag into HTML that the browser can understand.
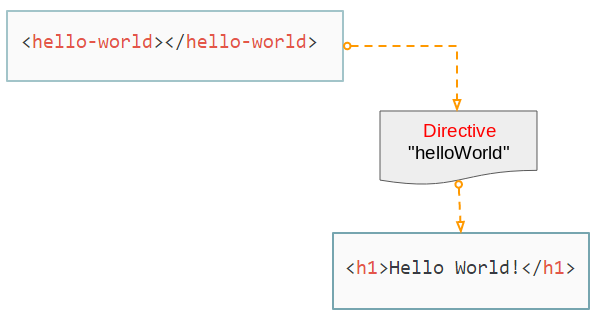
Directive E (Element):
Nothing is better than starting with an example. How can I create a new tag?
On any folder you create 2 directive-e-example.html & directive-e-example.js files:
directive-e-example.js
// Create an Application named "myApp"
var app = angular.module("myApp", []);
// Create a Directvie named "helloWorld"
app.directive("helloWorld", function() {
return {
restrict : "E",
template : "<h1>Hello World!</h1>"
};
});
In the above Javascript , firstly, create a module (app), then create a Directive named helloWorld for this module . The name of a Directive has to be in accordance with the camelCase rules.
- Các cách đặt tên trong các ngôn ngữ lập trình
directive-e-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Directive</title>
<!-- Check version: https://code.angularjs.org/ -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="directive-e-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Directive E (Element):</h3>
<hello-world></hello-world>
</div>
</body>
</html>
Open the directive-e-example.html file on browser, and you have the following result:
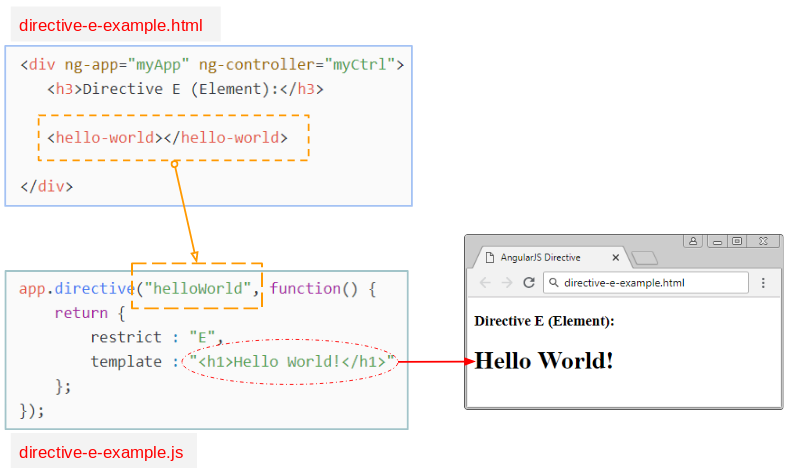
Directive A (Attribute)
In this example, I will create an attribute named good-bye for a tag of HTML:
We will create 2 directive-a-example.html & directive-a-example.js files:
directive-a-example.js
// Create an Application named "myApp"
var app = angular.module("myApp", []);
// Create a Directvie named "helloWorld"
app.directive("goodBye", function() {
return {
restrict : "A",
template : "<h1>Good Bye!</h1>"
};
});
directvie-a-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Directive</title>
<!-- Check version: https://code.angularjs.org/ -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="directive-a-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Directive A (Attribute):</h3>
<div good-bye></div>
</div>
</body>
</html>
Open the directive-a-example.html file on browser and receive the result:
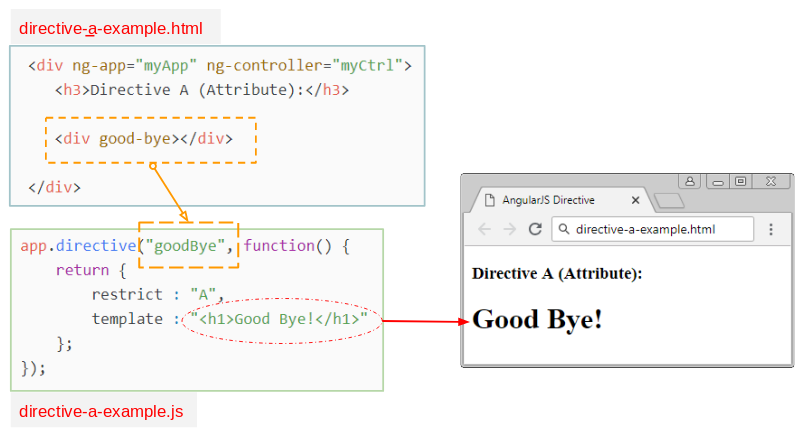
Directive C (Class)
directive-c-example.js
// Create an Application named "myApp"
var app = angular.module("myApp", []);
// Create a Directvie named "errMessage"
app.directive("errMessage", function() {
return {
restrict : "C",
template : "<h1>Something Error!</h1>"
};
});
directive-c-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Directive</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="directive-c-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Directive C (Class):</h3>
<div class="err-message"></div>
</div>
</body>
</html>
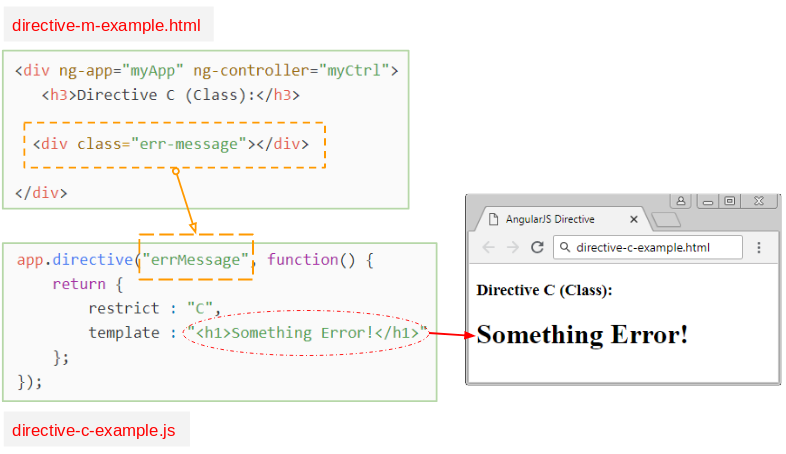
Directive M (Comment)
directive-m-example.js
// Create an Application named "myApp"
var app = angular.module("myApp", []);
// Create a Directvie named "myComment"
app.directive("myComment", function() {
return {
restrict : "M",
replace : true,
template : "<h1>OK Important!</h1>"
};
});
directive-m-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Directive</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="directive-m-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Directive M (Comment):</h3>
<!-- directive: my-comment -->
</div>
</body>
</html>
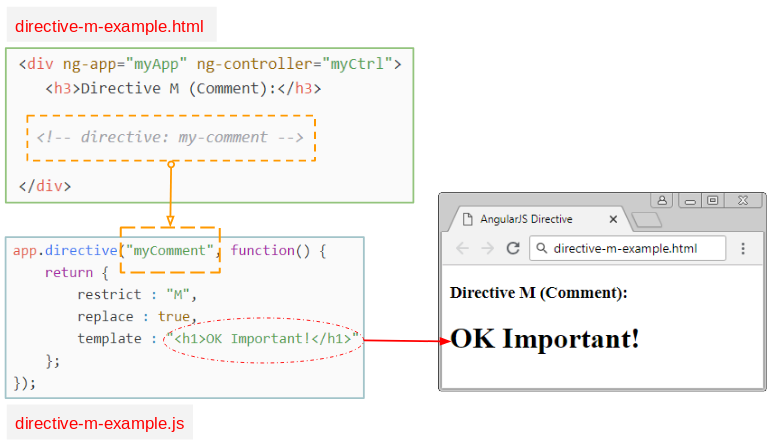
2. Directive with templateUrl
Normally, when you create a Directive you have to provide a HTML content through the "template" property. If the HTML content is too long, it will be a problem. In this case, you should put this HTML content into a file, and then use the "templateUrl" property to point to the position of such file .
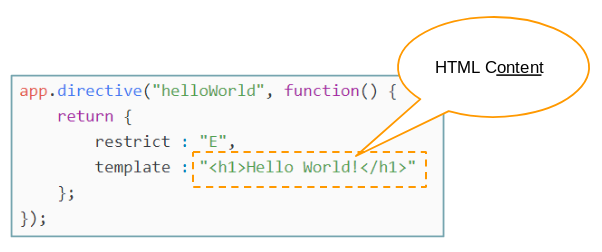
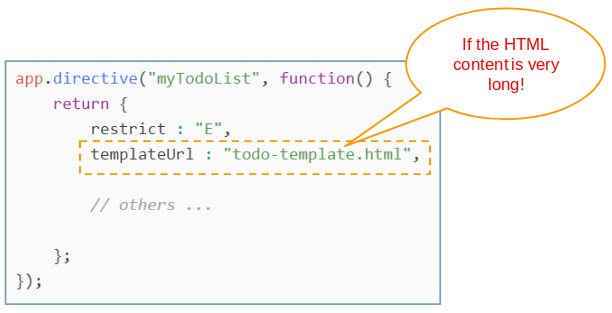
In this example, I have a data which is tasks to be done and I will create a Directive named "myTodoList". The data will be displayed like the following illustration:
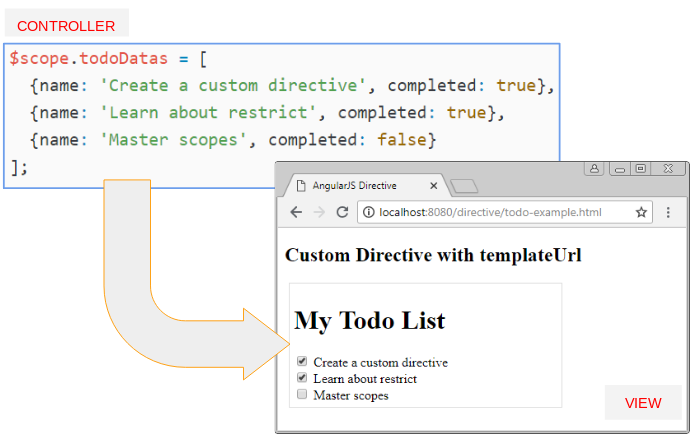
todo-template.html
<div class="my-todo-list">
<h1>{{title}}</h1>
<div ng-repeat="todo in todoList">
<input type="checkbox" ng-model="todo.completed"> {{todo.name}}
</div>
</div>
todo-example.js
var app = angular.module("myApp", []);
var ctrl = app.controller("myCtrl", function($scope) {
$scope.todoDatas = [
{name: 'Create a custom directive', completed: true},
{name: 'Learn about restrict', completed: true},
{name: 'Master scopes', completed: false}
];
});
// Create a Directive named "myTodoList"
// E: Element <my-todo-list todo-list="=" title="@">
app.directive("myTodoList", function() {
return {
restrict : "E",
templateUrl : "todo-template.html",
// '=' : an expression
// '@': a string
scope: {
todoList: '=',
title: '@'
}
};
});
todo-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Directive</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="todo-example.js"></script>
<style>
.my-todo-list {
border: 1px solid #ddd;
padding: 5px;
margin: 5px;
width: 320px;
}
</style>
</head>
<body>
<div ng-app="myApp">
<div ng-controller="myCtrl">
<h2>Custom Directive with templateUrl</h2>
<!-- $scope.todoDatas -->
<my-todo-list todo-list="todoDatas" title="My Todo List"/>
</div>
</div>
</body>
</html>
You need to run the todo-example.html file on a HTTP Server, which is mandatorybecause templateUrl requires that data source must be from http or https. It doesn't accept data source, coming from file:///.
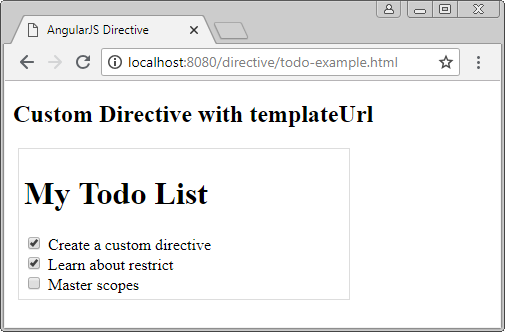
OK, I will explain the code of the above example
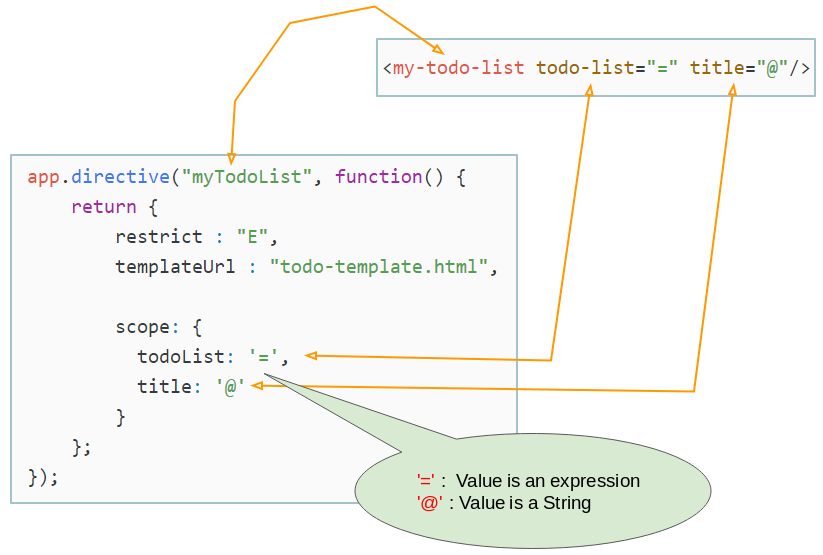
The above illustration shows that we are creating a Directive named "myTodoList". It's like you create a new <my-todo-list> tag with two todo-list & title attributes.
- '@' implies that it is a String.
- '=' implies that it is an Expression.
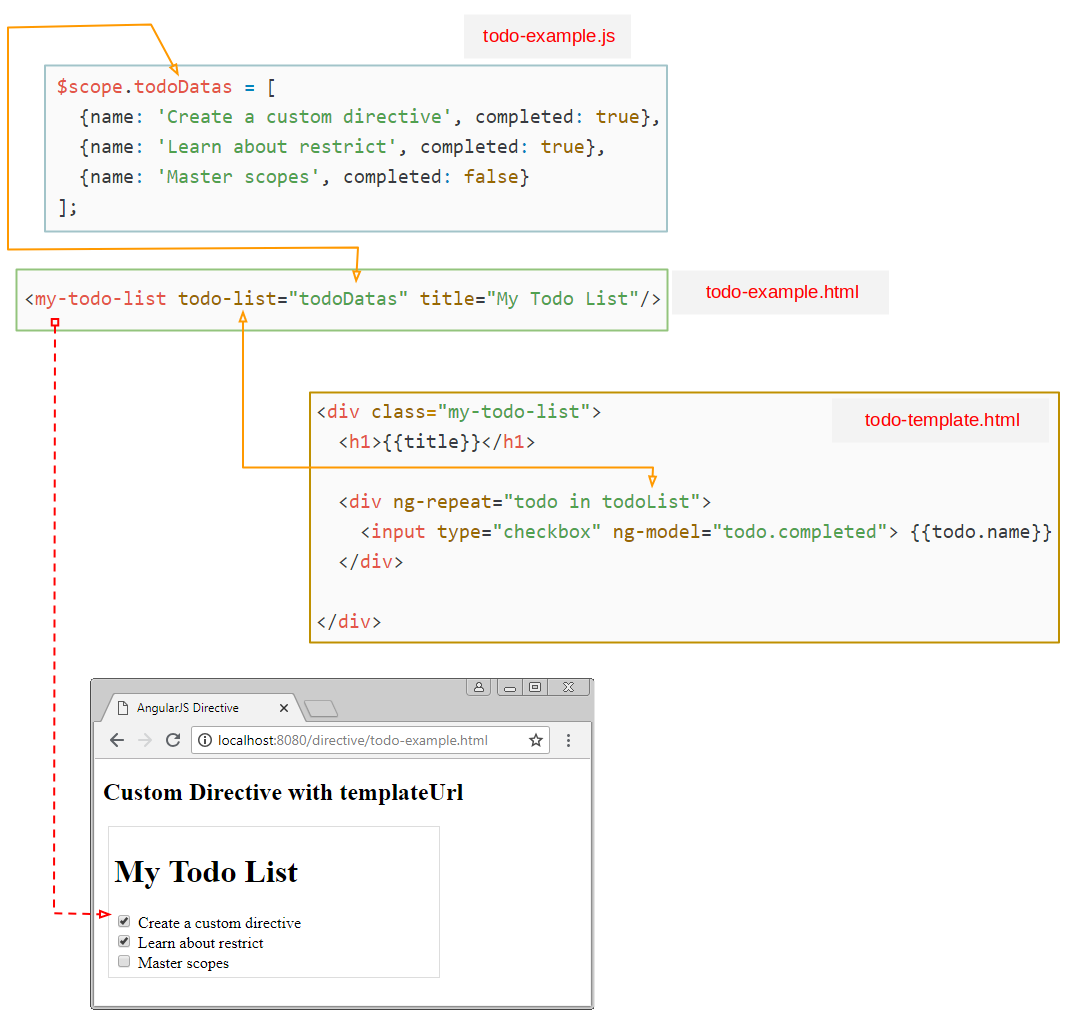
AngularJS Tutorials
- Introduction to AngularJS and Angular
- AngularJS Directive Tutorial with Examples
- AngularJS Model Tutorial with Examples
- AngularJS Filters Tutorial with Examples
- AngularJS Events Tutorial with Examples
- Quickstart with AngularJS - Hello AngularJS
- AngularJS Validation Tutorial with Examples
Show More