Loops in Batch Scripting Language
2. For Loop (Default)
For loop (default) of Batch language is used to iterate over a list of files.
Syntax:
@rem set_of_files - Set of Files
@rem Files separated by standard delimiters.
@rem The parameter name 'variable' must be 1 character
FOR %%variable IN ( set_of_files ) DO command
@rem Hoặc:
FOR %%parameter IN ( set_of_files ) DO (
command
)
Example: copy some files into a directory (Note: the files to be copied into the target directory need to be in the same disk drive).
copyFiles.bat
@echo off
@rem Copy to same Disk
FOR %%f IN (E:\test\file1.data E:\test\file2.txt) DO (
echo Copying %%f
copy %%f E:\backup
)
pause
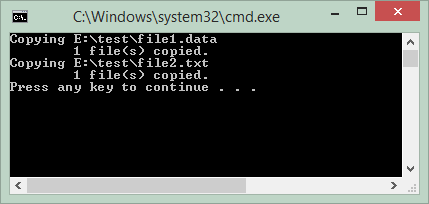
Other example:
@rem The delimiter is a semicolon (;)
FOR %%f IN ("E:\my dir\file1.data" ; E:\test\file2.txt) DO copy %%f E:\backup
@rem The delimiter is a comma ( , )
FOR %%f IN ("E:\my dir\file1.data" , E:\test\file2.txt) DO copy %%f E:\backup
@rem The delimiter is a space.
FOR %%f IN ("E:\my dir\file1.data" E:\test\file2.txt) DO copy %%f E:\backup
3. For /R
The FOR /R loop is used to iterate over the list of files, including files in subdirectories, grandchildren .. It is called Recurse loop.
Syntax:
FOR /R [path] %%variable IN ( set_of_file_filters ) DO command
@rem Or:
FOR /R [path] %%variable IN ( set_of_file_filters ) DO (
command
)
- [path] : This parameter is a root folder. If there is not this parameter "the folder containing executable script file" hoặc "current folder" will be considered as root folder .
- set_of_file_filters : List of file filters, for example *.txt , *.bat, ... or dot ( . ) means all.
- variable: means name of variable and must have one unique characte.
The following example prints the list of all *.txt or *.log files in the C:/Windows/System32 directory (Includes search in subdirectories, grandchildren ..)
forR_example1.bat
@echo off
FOR /R "C:\Windows\System32" %%f IN (*.txt *.log) DO (
echo %%f
)
pause
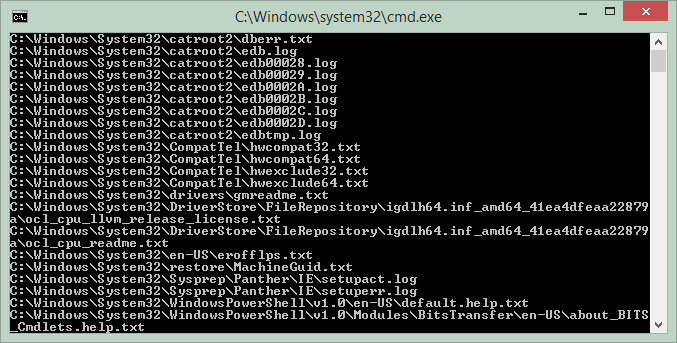
Example of listing all files in the C:/Windows/System32 directory (including files in subdirectories, grandchildren ...)
forR_example2.bat
@echo off
FOR /R "C:\Windows\System32" %%f IN ( . ) DO (
echo %%f
)
pause
4. For /D
The FOR /D loop is used to iterate over the list of directories which are subdirectories of current directory.
Syntax:
FOR /D [/r] %%variable IN ( set_of_directory_filters ) DO command
@rem Or:
FOR /D [/r] %%parameter IN ( set_of_directory_filters ) DO (
command
)
- set_of_directory_filters : List of directory filters for example, en*, fr*,.. separated by a standard delimiter.
- [/r]: This is a recurse parameter and not mandatory. If this parameter is available, subdirectories, grandchildren .. will be envolved in the loop.
- variable: means name of variable and must have a unique character.
Example: List subdirectories of C:/Windows.
forD_example1.bat
@echo off
C:
cd C:/Windows
FOR /D %%d IN ( * ) DO (
echo %%d
)
pause
Example: List subdirectories, grandchildren ... of the C:/Windows with the name started by "en" or "fr"
forD_example2.bat
@echo off
C:
cd C:/Windows
FOR /D /r %%d IN (en* fr*) DO (
echo %%d
)
pause
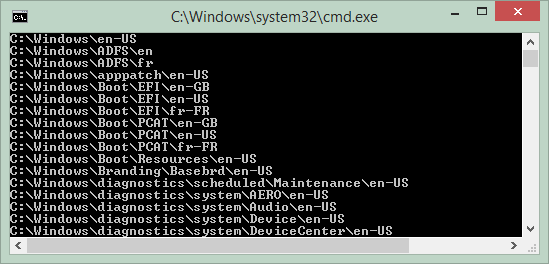
5. For /L
For /L loop is used to iterate over a range of numbers.
Syntax:
FOR /L %%variable IN (start, step, end) DO command
@rem Or:
FOR /L %%variable IN (start, step, end) DO (
command
)
- start: The first value of variable
- step: After each iteration, variable value will be added 'step'.
- end: Last value.
Example:
forL_example1.bat
@echo off
FOR /L %%d IN (1 2 8 ) DO (
echo %%d
)
pause
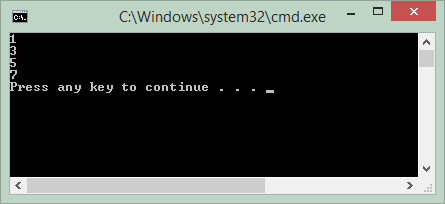
forL_example2.bat
@echo off
FOR /L %%d IN (20 -2 5 ) DO (
echo %%d
)
pause
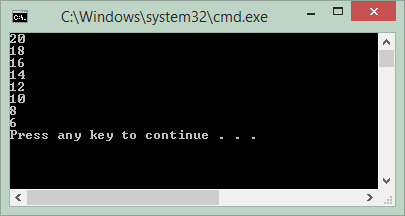
6. For /F
The For /F loop is a complex but powerful loop. It reads a file or a few files and then analyzes the contents of files. The content of a file is a text; it is split into several small pieces of text, each of which is called a Token. The default rule for separating a text is based on white space. However, you can customize the delimiter rule by ["delims = xxx"] parameter.
The For / F loop is also used to analyze the contents of a string, or to execute a set of commands.
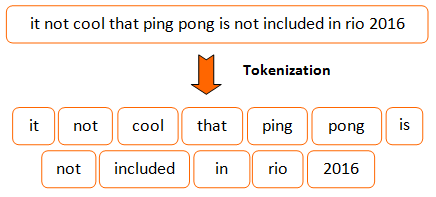
Syntax:
FOR /F ["options"] %%variable IN ( set_of_filenames ) DO command
FOR /F ["options"] %%variable IN ( set_of_filenames ) DO (
command
)
FOR /F ["options"] %%variable IN ("Text string to process") DO command
FOR /F ["options"] %%variable IN ("Text string to process") DO (
command
)
- set_of_filenames: List of a or several files.
- options: Options, for example, "delims=, tokens=1,2,4"
Option | Description |
delims=xxx | Delimiter character(s). by default is a space |
skip=n | The first line number will be ignored in the contents of file, defaulted skip = 0 |
eol=; | eol (End of Line): Specifies a special character, which is put at the beginning of a line to mark this line as comment line. Comment lines will be ignored by the program, defaulted semicolon character (;) |
tokens=n1,n2,n3 | defines the positions cared about (n1, n2, n3, ..), defaulted tokens=1 |
usebackq | (See explanation in examples) |
To be easy to understand, let's analyze the following file:
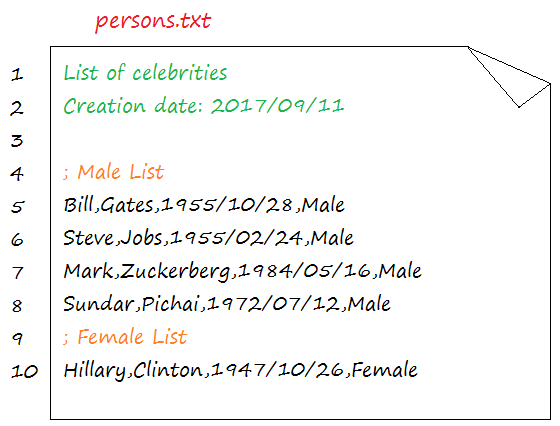
eol (End of Line)
eol: Used to specify a special character, which is by default a semicolon character (;). It is placed at the beginning of a line to mark that such line is a comment. The program will ignore this line.
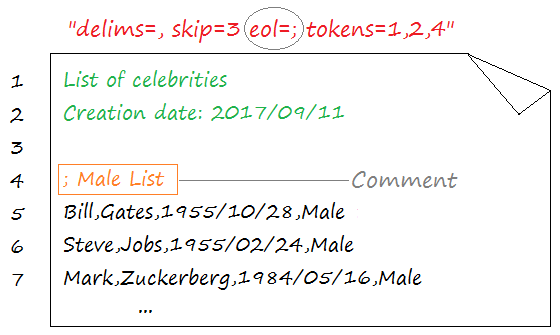
skip=n
skip: Declare the number of first lines of the file will be ignored, the program will not analyze these lines. Default skip = 0
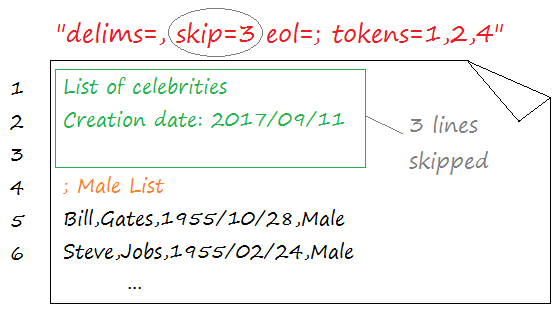
delims (Delimiter character(s))
delims: Defines delimiter characters, helping the program to delimit each line of text into sub-paragraphs, each of which is called a Token. Tokens are marked indices: 1, 2, 3, ...
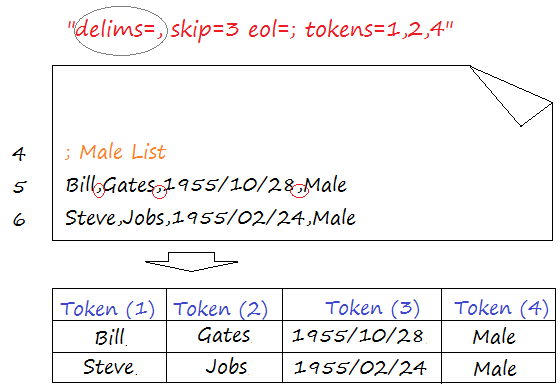
tokens=n1,n2,n3
tokens: Declare a list of indices that are interested in, separated by commas. For example, tokens = 1,2,4, defaulted: tokens = 1
Tokens | Description |
tokens=1,2,4 | The indices such as 1, 2, 4 are interested. |
tokens=2-8 | The indices of 2 to 8 are interested |
tokens=3,* | The indices such as 3, 4,5, ... are interested |
tokens=* | All indices are interested |
For example, "tokens=1,2,4", means that Tokens at the position of 1, 2, 4 are interested and other Tokens will be ignored.
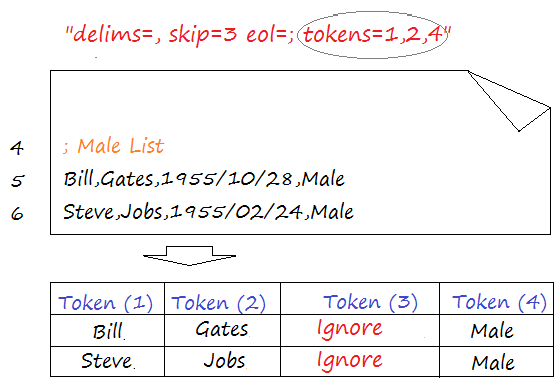
Example of file analysis:
Example of using the FOR /F loop to read persons.txt file:
persons.txt
List of celebrities
Creation date: 2017/09/11
; (Comment) Male List
Bill,Gates,1955/10/28,Male
Steve,Jobs,1955/02/24,Male
Mark,Zuckerberg,1984/05/16,Male
Sundar,Pichai,1972/07/12,Male
; (Comment) Female List
Hillary,Clinton,1947/10/26,Female
forF_example1.bat
@echo off
FOR /F "delims=, skip=3 eol=; tokens=1,2,4" %%i IN ( persons.txt ) DO (
echo Full Name: %%i %%j Gender: %%k
)
pause
Note: Name of variables on the loop has only character .Biến %%i variable is explicitly declared on the loop. %% j, %% k variables are implicitly declared (names of implicit variables are the next characters of explicit variable names).
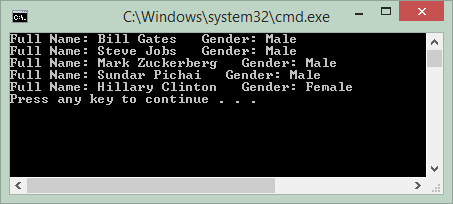
Example of String analysis:
- TODO