Arrays in Batch Scripting Language
1. Array in Batch language
An array is a row of contiguous elements which are marked indices: 0, 1, 2, ....
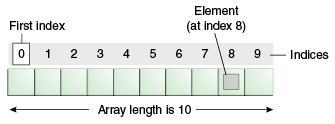
For other languages an array has fixed size. But in the Batch language, the array has a dynamic size, and there is no attribute describing array length (the number of array elements). And no function directly helps you get the number of array elements.
All array elements need to be assigned values through a set command. If not, such element doesn't exist .
@echo off
set myarray[0] = Abc
set /A myarray[1] = 234
set myarray[2]=Def
set myarray[0]=A new value
Example:
arrayExample1.bat
@echo off
set names[0]=Tom
set names[1]=Jerry
set names[2]=Donald
set names[3]=Aladin
echo names[0]= %names[0]%
echo names[3]= %names[3]%
@rem names[10] does not exists!
echo names[10]= %names[10]%
pause
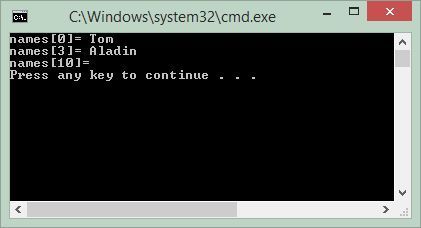
You can assign new values to array elements. Below is an example:
arrayExample2.bat
@echo off
set names[0]=Tom
set names[1]=Jerry
echo names[0]= %names[0]%
@rem: Assign new value
set names[0]=Donald
echo After assign new value to names[0]:
echo names[0]= %names[0]%
pause
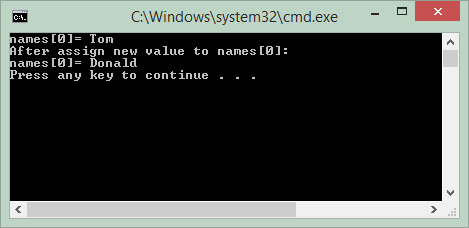
2. Test the existance of an element
Using defined command helps you to check whether an element in an array exists or not?
arrayDefinedExample.bat
@echo off
set Arr[0]=1000
set Arr[1]=5000
set Arr[2]=3000
if not defined Arr[5] (
echo Element at 5 does not exists!
)
if defined Arr[1] (
echo Element at 1 exists!
)
pause
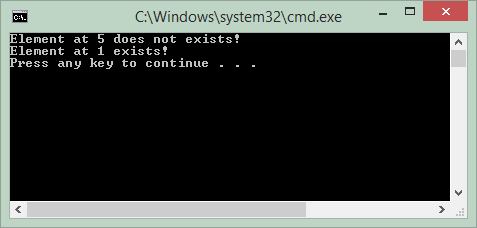
3. Iterate through the elements of the array
See more:
For /F loop can be approved on a range of numbers, therefore, it can be approved on a range of array indices
fetchArrayExample1.bat
@echo off
set fruits[0]=Apple
set fruits[1]=Apricot
set fruits[2]=Asparagus
set fruits[3]=Aubergine
set fruits[4]=Banana
FOR /L %%i IN (0 1 4) DO (
call echo Element At %%i = %%fruits[%%i]%%
)
pause
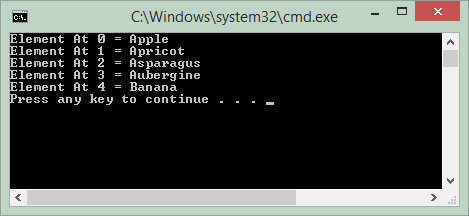
If you don't know the number of array elements in advance, you can iterate over its elements by using goto command.
fetchArrayExample2.bat
@echo off
set fruits[0]=Apple
set fruits[1]=Apricot
set fruits[2]=Asparagus
set fruits[3]=Aubergine
set fruits[4]=Banana
set /A i = 0
:my_loop
if defined fruits[%i%] (
call echo Element At %i% = %%fruits[%i%]%%
set /a i = %i% + 1
goto :my_loop
)
echo Done!
pause
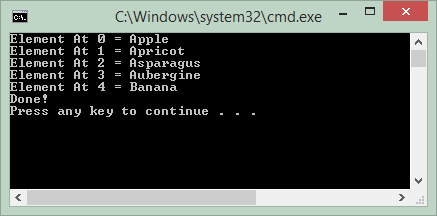
4. An element has stucture
In the Batch language, an array element can have structure. A structure is an object with many attributes, for example an object represents for a person with 2 attributes such as firstName, lastName.
structureArrayExample.bat
@echo off
set persons[0].firstName=Bill
set persons[0].lastName=Gates
set persons[1].firstName=Steve
set persons[1].lastName=Jobs
set persons[2].firstName=Mark
set persons[2].lastName=Zuckerberg
set persons[3].firstName=Sundar
set persons[3].lastName=Pichai
FOR /L %%i IN (0 1 3) DO (
call echo Person At %%i = %%persons[%%i].firstName%% %%persons[%%i].lastName%%
)
pause
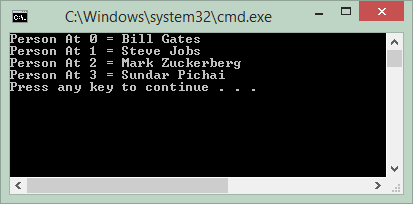