Build a Multiple Module Project with Gradle
1. Introduction
This document is based on:
Eclipse 4.6 (NEON)
You are viewing the advanced Gradle. If you are a beginner Gradle. You should look at the documentation for beginners Gradle (Gradle Hello world) at:
2. Model of example
This is the model example in this document.
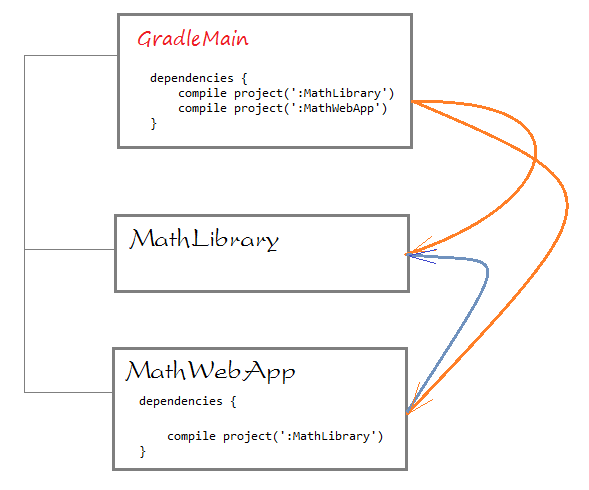
The objective of the guidelines is:
MathWebApp: is a WebApp project
MathLibrary: is a library Project, contains utility classes used by MathWebApp.
GradleMain: will pack two projects above, it is a main module. GradleMain will:
- How a module using other module in Gradle
- Packing multiple Module using Gradle (output: jar, war).
- Run Web App on Gradle Tomcat Plugin.
MathWebApp: is a WebApp project
MathLibrary: is a library Project, contains utility classes used by MathWebApp.
GradleMain: will pack two projects above, it is a main module. GradleMain will:
- Packing MathLibary to a jar file
- Packing MathWebApp to a file war.
3. Create MathLibrary project
- File/New/Other...
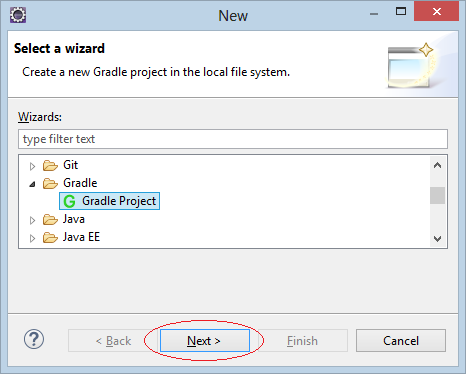
Enter:
- Project Name: MathLibrary
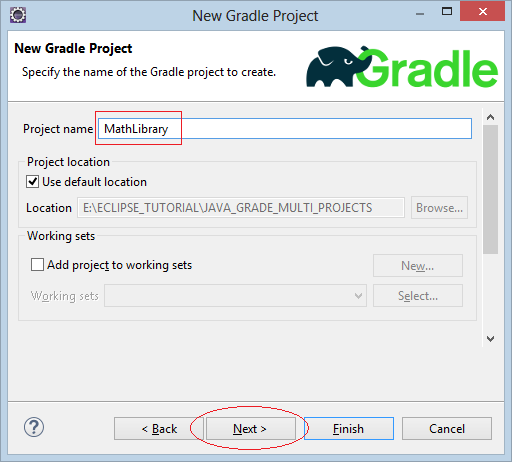
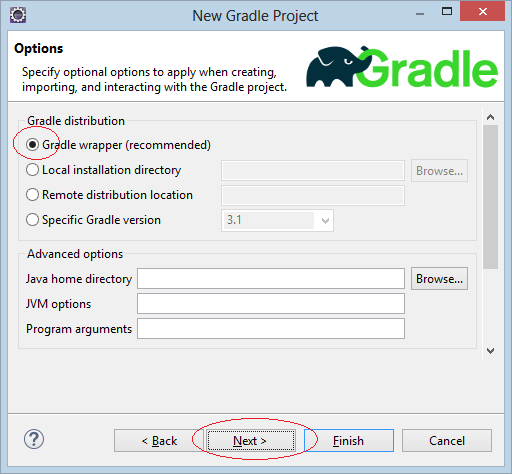
Project is created.
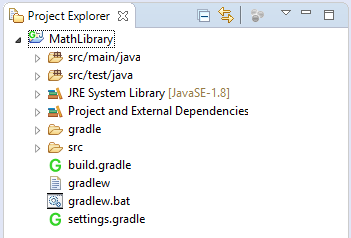
Create class MathUtils:
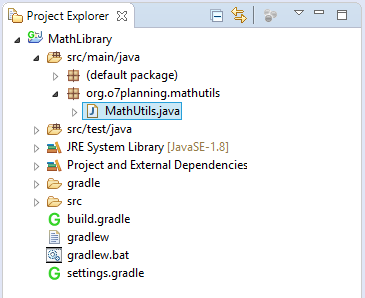
The MathUtils class has a method for caculating the sum of two numbers in the MathLibrary project and will be used in the MathWebApp project.
package org.o7planning.mathutils;
public class MathUtils {
public static int sum(int a, int b) {
return a + b;
}
}
4. Create MathWebApp project
Similarly create MathWebApp project.
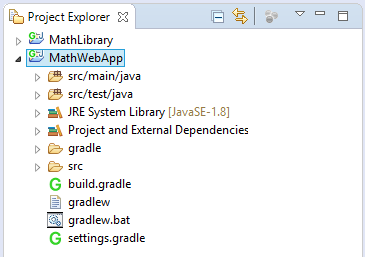
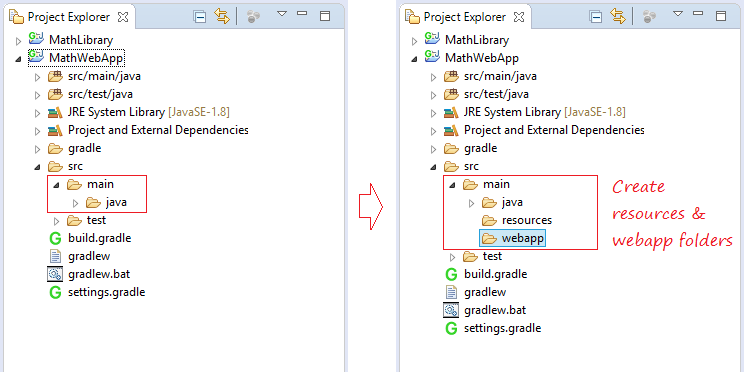
- Open file pom.xml
MathWebApp is a web application that uses the MathLibrary project as a library. Therefore you need to declare MathWebApp dependent on MathLibrary.
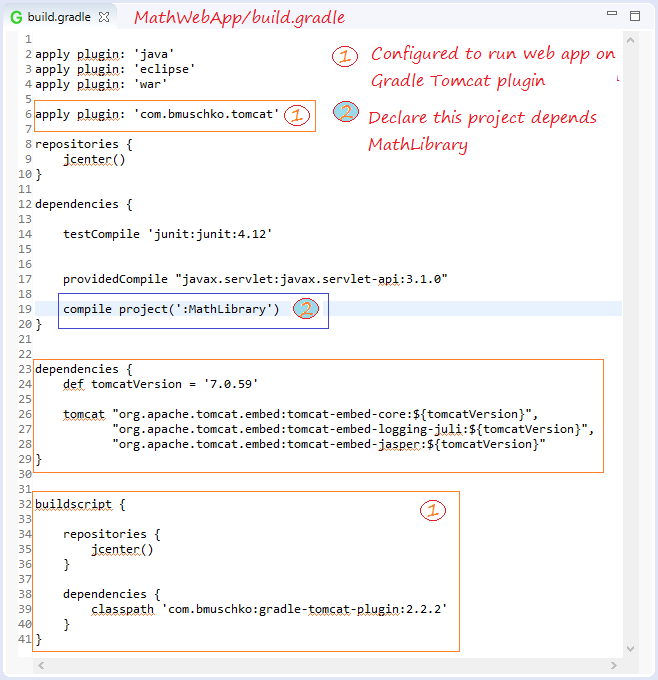
MathWebApp/build.gradle
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'war'
apply plugin: 'com.bmuschko.tomcat'
repositories {
jcenter()
}
dependencies {
testCompile 'junit:junit:4.12'
providedCompile "javax.servlet:javax.servlet-api:3.1.0"
compile project(':MathLibrary')
}
dependencies {
def tomcatVersion = '7.0.59'
tomcat "org.apache.tomcat.embed:tomcat-embed-core:${tomcatVersion}",
"org.apache.tomcat.embed:tomcat-embed-logging-juli:${tomcatVersion}",
"org.apache.tomcat.embed:tomcat-embed-jasper:${tomcatVersion}"
}
buildscript {
repositories {
jcenter()
}
dependencies {
classpath 'com.bmuschko:gradle-tomcat-plugin:2.2.2'
}
}
Each time you change build.gradle you need to use the Gradle tool to refresh the project.

Create index.jsp file using MathUtils, class in project MathLibrary.
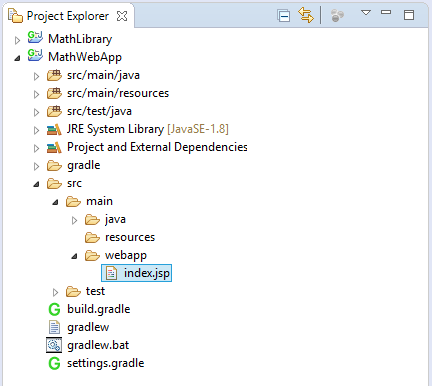
index.jsp
<html>
<body>
<h2>Hello World!</h2>
<%
int a = 100;
int b = 200;
int c = org.o7planning.mathutils.MathUtils.sum(a,b);
out.println("<h2>"+ c+"</h2>");
%>
</body>
</html>
5. Create GradleMain project
Similarly create GradleMain project.
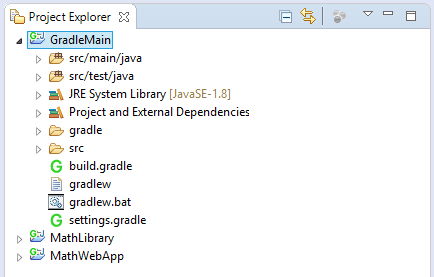
GradleMain is a project that builds 2 projects MathLibrary and MathWebApp. You need to define these two projects in the settings.gradle.
GradleMain/settings.gradle
rootProject.name = 'GradleMain'
include ':MathLibrary', ':MathWebApp'
project(':MathLibrary').projectDir = new File(settingsDir, '../MathLibrary')
project(':MathWebApp').projectDir = new File(settingsDir, '../MathWebApp')
And declare GradleMain depends on Math Library & MathWebApp.
GradleMain/build.gradle
apply plugin: 'java'
apply plugin: 'eclipse'
repositories {
jcenter()
}
dependencies {
testCompile 'junit:junit:4.12'
}
dependencies {
compile project(':MathLibrary')
compile project(':MathWebApp')
}
Each time you change build.gradle you need to use the Gradle tool to refresh the project.
