Struts2 Namespace Tutorial with Examples
1. @Namespace Annotation
@Namespace Is an annotation, it can use to annotate at package or class level. And it will have the effect for the Action class in the package or the Action class is annotated by @Namespace.
Normally, if your Action class is not annotated by @Namespace, and it is not belong to a package annotated by @Namespace, it is annotated by @Namespace(value = "/") by default.
// Configure an Action:
@Action(value = "hello", //
results = {
// ...
)
public class HelloAction extends ActionSupport
// ==================================
// Same as:
@Namespace(value ="/")
@Action(value = "hello", //
results = {
// ...
)
public class HelloAction extends ActionSupport
The illustration below shows how to access an 'Action' in a namespace.
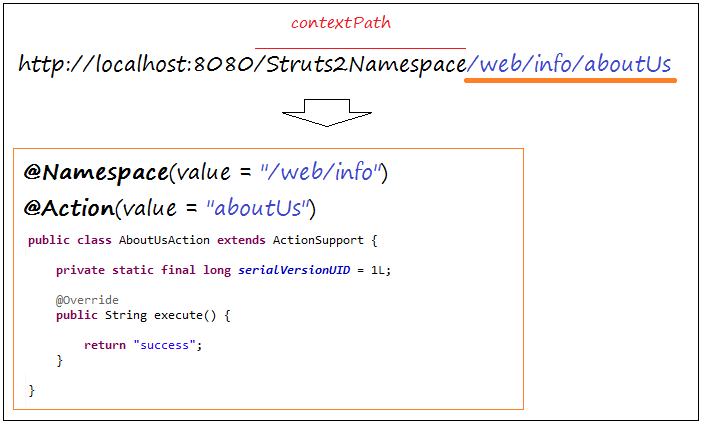
@Namespace annotated on the package:
@Namespace("/path1/path2")
package org.o7planning.struts2namespace.action;
3. Configure Struts2, pom.xml & web.xml
Configure Struts2 in web.xml:
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>Struts2Namespace</display-name>
<filter>
<filter-name>struts2</filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<welcome-file-list>
<welcome-file>/index.jsp</welcome-file>
</welcome-file-list>
</web-app>
Configure maven:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>Struts2Namespace</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>Struts2Namespace Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Servlet Library -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- http://mvnrepository.com/artifact/org.apache.struts/struts2-core -->
<dependency>
<groupId>org.apache.struts</groupId>
<artifactId>struts2-core</artifactId>
<version>2.3.20</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.apache.struts/struts2-convention-plugin -->
<dependency>
<groupId>org.apache.struts</groupId>
<artifactId>struts2-convention-plugin</artifactId>
<version>2.3.20</version>
</dependency>
</dependencies>
<build>
<finalName>Struts2Namespace</finalName>
<plugins>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default: /Struts2Namespace : 8080) -->
<!-- <configuration> <path>/</path> <port>8899</port> </configuration> -->
</plugin>
</plugins>
</build>
</project>
4. Struts2 Action & Jsp
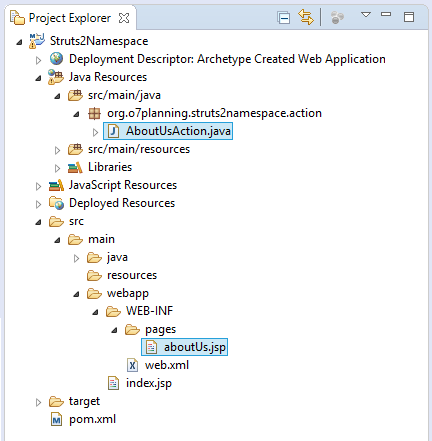
AboutUsAction.java
package org.o7planning.struts2namespace.action;
import org.apache.struts2.convention.annotation.Action;
import org.apache.struts2.convention.annotation.Namespace;
import org.apache.struts2.convention.annotation.Result;
import com.opensymphony.xwork2.ActionSupport;
@Namespace(value = "/web/info")
@Action(value = "aboutUs", //
results = { //
@Result(name = "success", location = "/WEB-INF/pages/aboutUs.jsp") //
} //
)
public class AboutUsAction extends ActionSupport {
private static final long serialVersionUID = 1L;
@Override
public String execute() {
return "success";
}
}
/WEB-INF/pages/aboutUs.jsp
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>About Us</title>
</head>
<body>
<h3>About o7planning</h3>
<p>Address: Vietnam</p>
<p>Contact: ... </p>
</body>
</html>