Struts2 Tutorial for Beginners (XML Config)
1. Introduction
This document was written based on:
- Eclipse 4.6
- Struts 2 (2.3.20)
You are viewing "Hello World Struts2 - XML Example". You can view the same example using the Annotation at:
2. Create Maven Project
- File/New/Other..
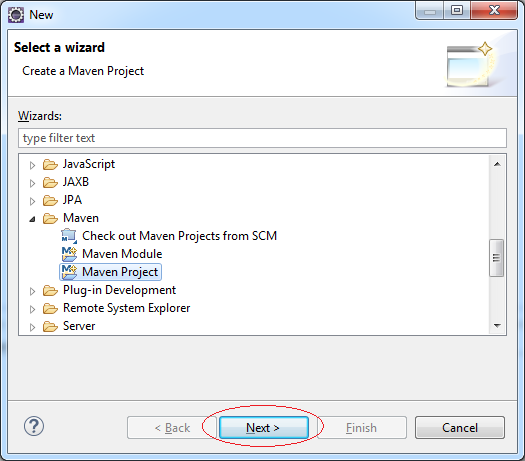
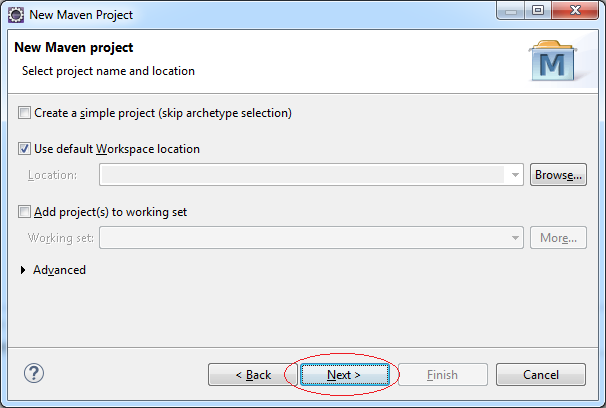
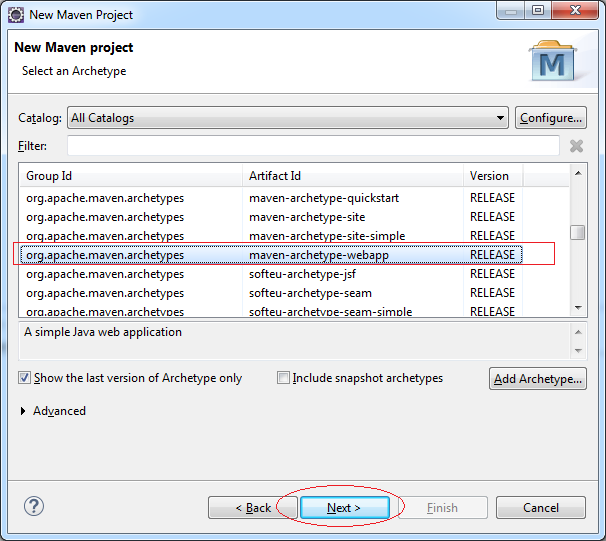
Enter:
- Group ID: org.o7planning
- Artifact ID: Struts2XML
- Package: org.o7planning.tutorial.struts2xml
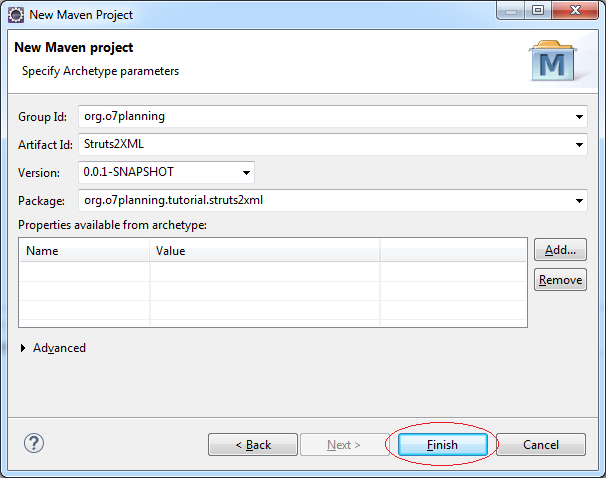
Do not worry about the error message when the Project has been created. The reason is that you do not declare the Servlet library.
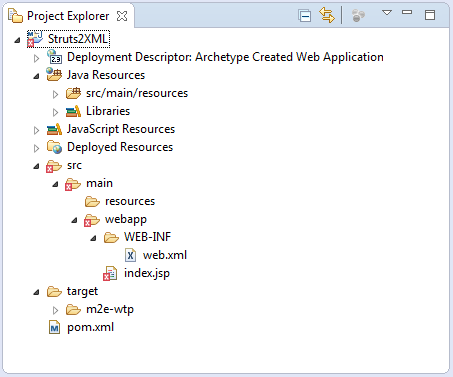
Eclipse create Maven project structure may be wrong. You need to fix
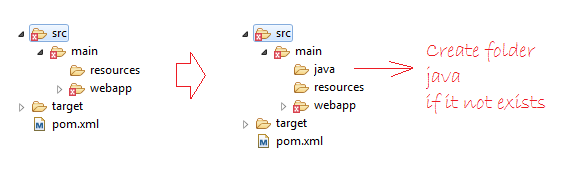
This is the image after Project Completion:
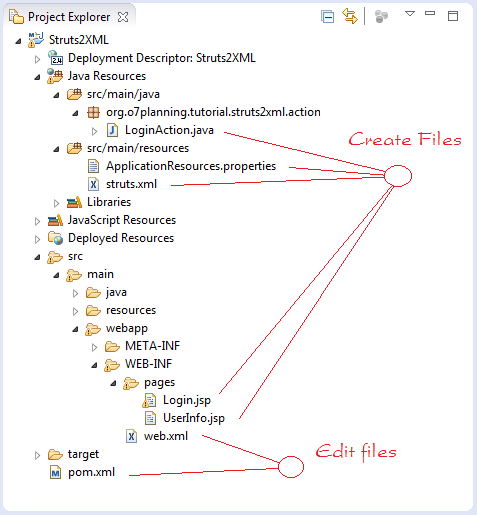
3. Configuring Maven
Configuring Maven to declare the library used. Includes library Servlet, Struts2. Also configure Tomcat Maven Plugin for running web applications directly on Eclipse.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>Struts2XML</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>Struts2XML Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Servlet Library -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- Jstl for jsp page -->
<!-- http://mvnrepository.com/artifact/javax.servlet/jstl -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- JSP API -->
<!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency>
<!-- http://mvnrepository.com/artifact/org.apache.struts/struts2-core -->
<dependency>
<groupId>org.apache.struts</groupId>
<artifactId>struts2-core</artifactId>
<version>2.3.20</version>
</dependency>
</dependencies>
<build>
<finalName>Struts2XML</finalName>
<plugins>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default: /Struts2XML : 8080) -->
<!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build>
</project>
Now your project has no error message.
4. Configuring Struts & web.xml
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>Struts2XML</display-name>
<filter>
<filter-name>struts2</filter-name>
<filter-class>
org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<welcome-file-list>
<welcome-file>/WEB-INF/pages/Login.jsp</welcome-file>
</welcome-file-list>
</web-app>
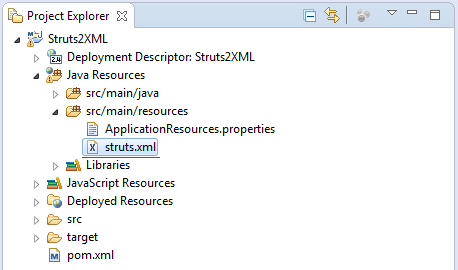
struts.xml is a resource file, it should be placed in src/main/resources, this file is used to configure the struts.
struts.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.enable.DynamicMethodInvocation"
value="false" />
<constant name="struts.devMode" value="true" />
<constant name="struts.custom.i18n.resources"
value="ApplicationResources" />
<package name="default" namespace="/" extends="struts-default">
<action name="login"
class="org.o7planning.tutorial.struts2xml.action.LoginAction">
<result name="success" type="redirect">/userInfo</result>
<result name="error">/WEB-INF/pages/Login.jsp</result>
</action>
<action name="userInfo"
class="org.o7planning.tutorial.struts2xml.action.UserInfoAction">
<result name="userInfoPage">/WEB-INF/pages/UserInfo.jsp</result>
</action>
</package>
</struts>
5. Code Project
Create ApplicationResources.properties file in src:
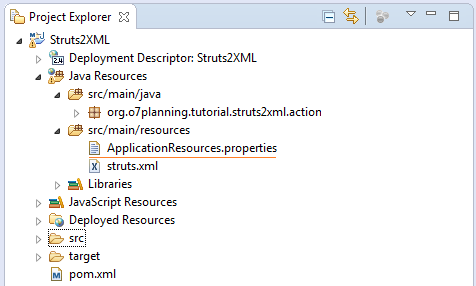
ApplicationResources.properties
label.username= Username
label.password= Password
label.login= Login
error.login= Invalid Username/Password. Please try again.
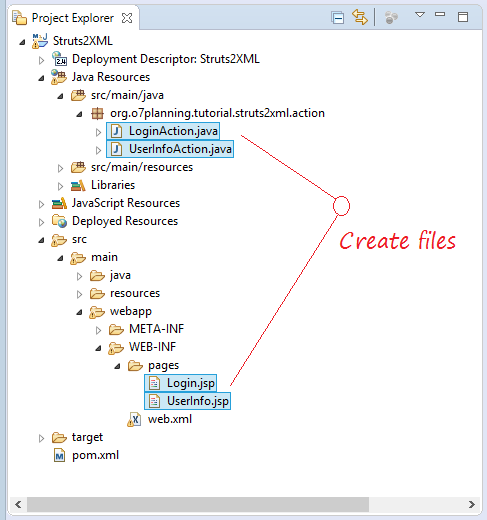
Login.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="s" uri="/struts-tags"%>
<html>
<head>
<title>Struts 2 - Login Application </title>
</head>
<body>
<h2>Struts 2 - Login Application</h2>
<s:actionerror />
<s:form action="/login" method="post">
<s:textfield name="username" key="label.username" size="20" />
<s:password name="password" key="label.password" size="20" />
<s:submit method="execute" key="label.login" align="center" />
</s:form>
<br>
Username: admin, password: admin123
</body>
</html>
UserInfo.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<html>
<head>
<title>User Info</title>
</head>
<body>
<h2>Hello, ${loginedUsername}...!</h2>
</body>
</html>
LoginAction.java
package org.o7planning.tutorial.struts2xml.action;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.apache.struts2.ServletActionContext;
import com.opensymphony.xwork2.ActionSupport;
public class LoginAction extends ActionSupport {
private static final long serialVersionUID = 7299264265184515893L;
private String username;
private String password;
@Override
public String execute() {
HttpServletRequest request = ServletActionContext.getRequest();
if (this.username != null && this.password != null && this.username.equals("admin")
&& this.password.equals("admin123")) {
HttpSession session = request.getSession();
// Store userName in session
session.setAttribute("loginedUsername", this.username);
return "success";
} else {
addActionError(getText("error.login"));
return "error";
}
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
UserInfoAction.java
package org.o7planning.tutorial.struts2xml.action;
import com.opensymphony.xwork2.ActionSupport;
public class UserInfoAction extends ActionSupport {
private static final long serialVersionUID = 7299264265184515893L;
@Override
public String execute() {
return "userInfoPage";
}
}
6. The flow of the program
The illustration below depicts the flow of the user program from accessing the website page to receive response
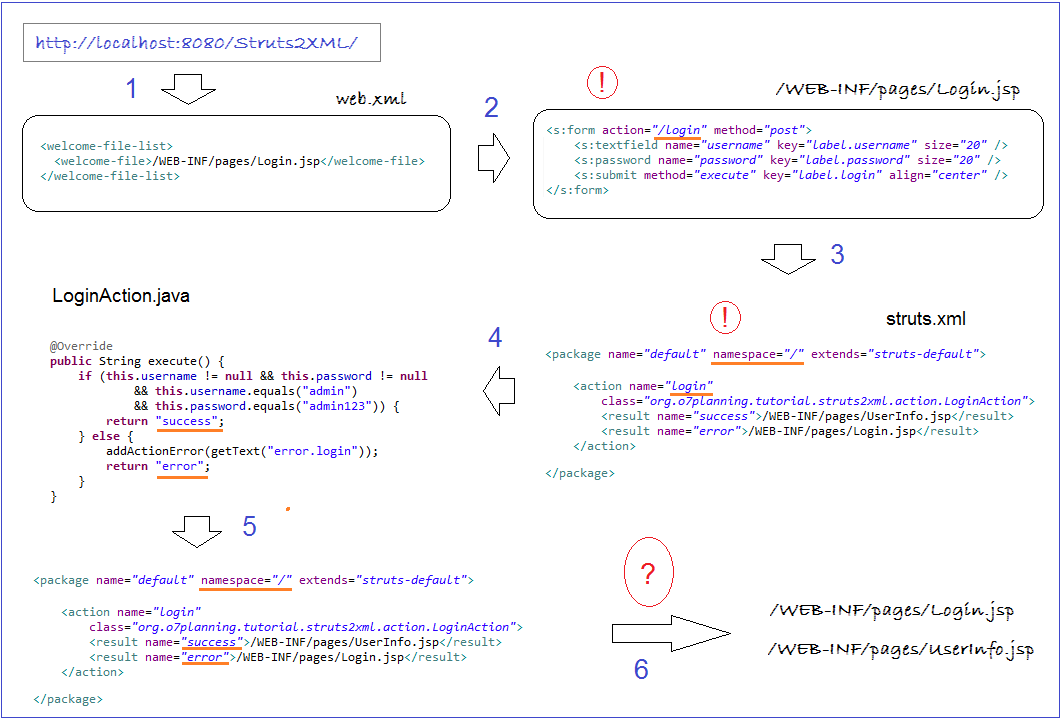
7. Running application
To run the application directly on Eclipse, you need to configure to run Tomcat Maven Plugin.
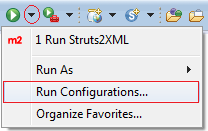
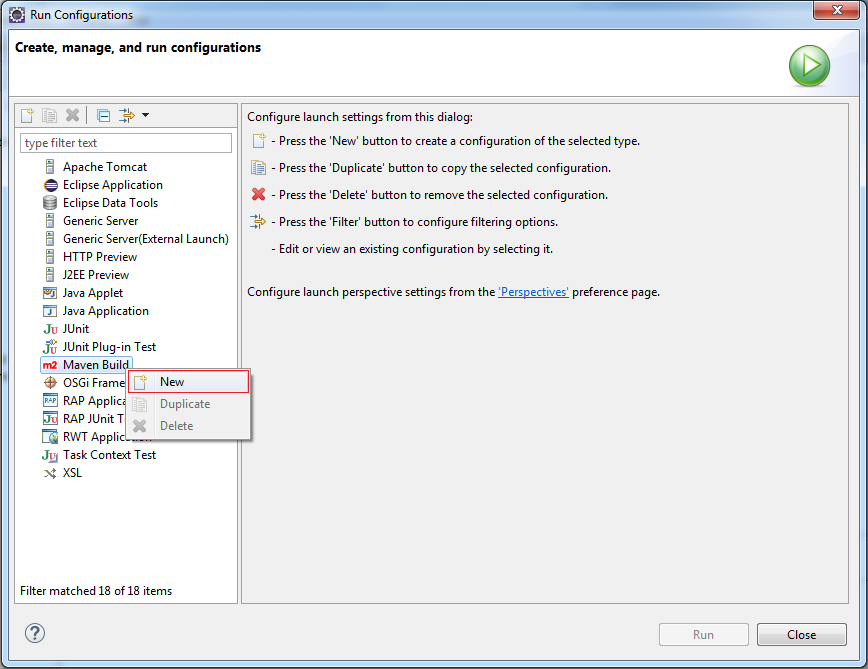
Enter:
- Name: Run Struts2XML
- Base Directory: ${workspace_loc:/Struts2XML}
- Goals: tomcat7:run
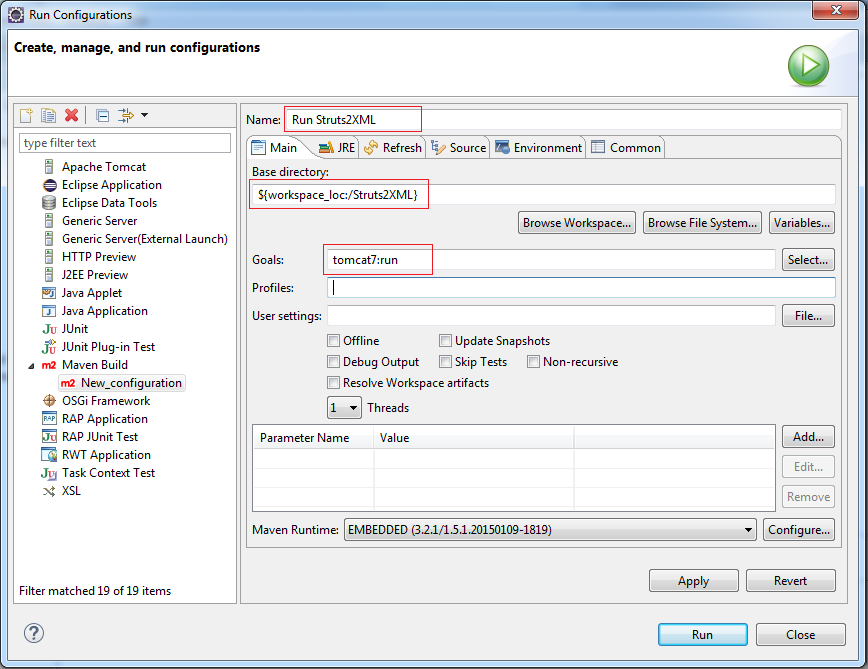
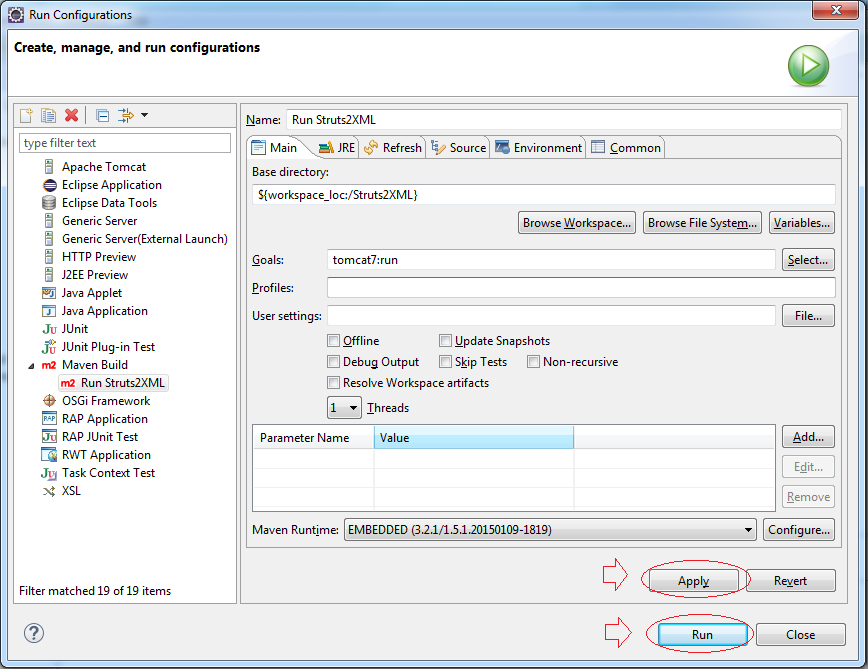
Tomcat Maven Plugin is running
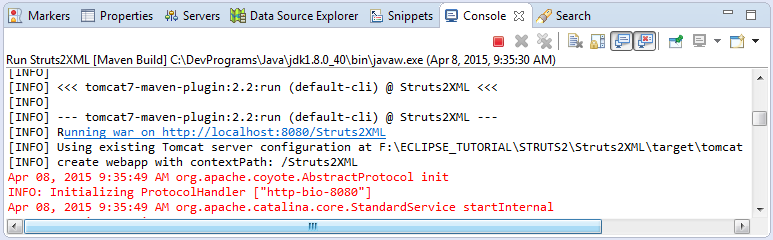
In the browser, enter the path:
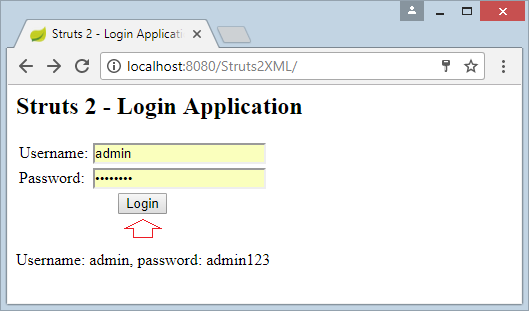
If you entered an incorrect username/password, page will be displayed as shown below:
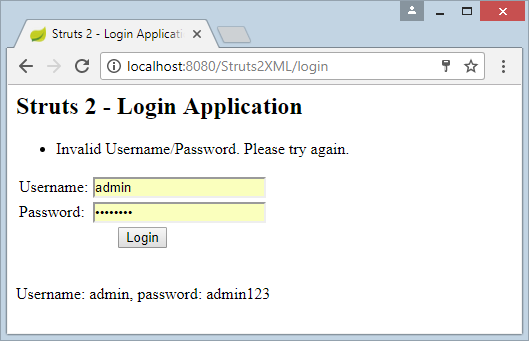
Else:
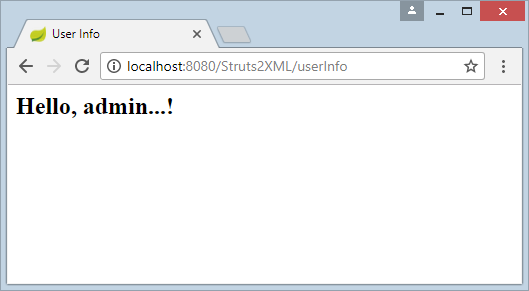