Flutter Positioned Tutorial with Examples
1. Positioned
Positioned widget is used to position a child widget of a Stack.
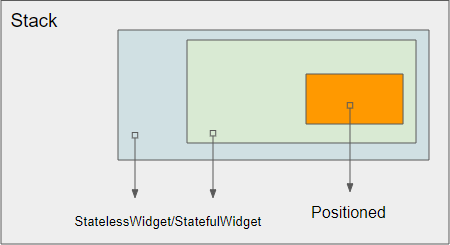
Positioned is only used as a direct (or descendant) child widget of Stack. On the path from Positioned to Stack, it includes only StatelessWidget or StatefulWidget widgets, other widgets are not allowed (eg RenderObjectWidget).
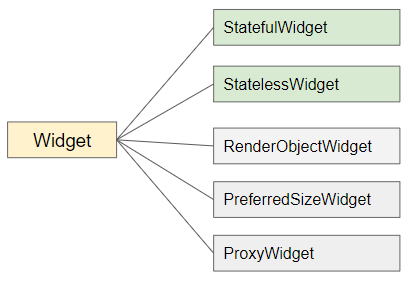
Positioned constructor
const Positioned(
{Key key,
double left,
double top,
double right,
double bottom,
double width,
double height,
@required Widget child}
)
Example:
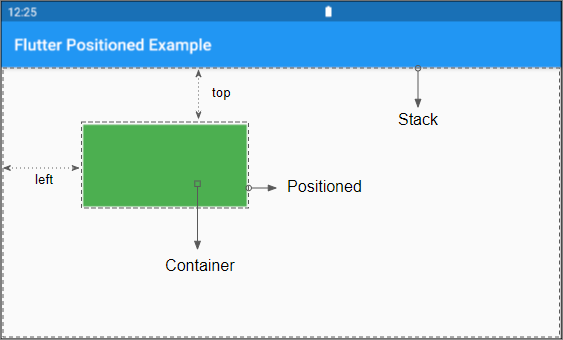
(ex1)
SizedBox (
width: double.infinity,
height: double.infinity,
child: Stack(
alignment: Alignment.centerLeft,
children: <Widget>[
Positioned (
left: 100,
top: 70,
child: Container(
width: 200,
height: 100,
color: Colors.green,
),
)
],
)
)
The size of the Positioned and its child are always the same.
Let's look at an example: A Positioned with a non-nulltop and bottom forces the height of the child widget to change to accommodate that constraint.
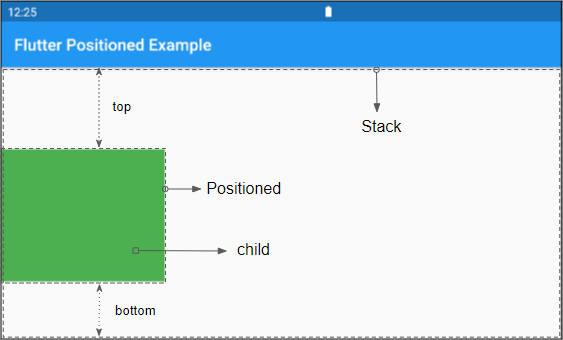
(ex2)
SizedBox (
width: double.infinity,
height: double.infinity,
child: Stack(
alignment: Alignment.centerLeft,
children: <Widget>[
Positioned (
top: 100,
bottom: 70,
child: Container (
width: 200,
height: 30, // !!
color: Colors.green,
),
)
],
)
)
If all three parameters left, right, width are null then Stack.alignment property will be used to position the child widget horizontally. Likewise, if all three parameters top, bottom and height are null then Stack.alignment property will be used to position the child widget vertically.
2. Positioned.directional constructor
Positioned.directional is used to create a Positioned based on text direction.
Positioned.directional constructor
Positioned.directional(
{Key key,
@required TextDirection textDirection,
double start,
double top,
double end,
double bottom,
double width,
double height,
@required Widget child}
)
textDirection parameter is required and not null. It accepts value TextDirection.ltr (Left to Right) or TextDirection.rtl (Right to Left).
If textDirection is TextDirection.ltr, parameters (start, end) will correspond to (left, right). Otherwise (start, end) will correspond to (right, left).
3. Positioned.fill constructor
Positioned.fill is a constructor with parameters left, right, top and bottom have default values 0.
Positioned.fill constructor
const Positioned.fill(
{Key key,
double left: 0.0,
double top: 0.0,
double right: 0.0,
double bottom: 0.0,
@required Widget child}
)
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More