Flutter Expanded Tutorial with Examples
1. Expanded
Expanded is a widget that helps to expand the space for a child widget of Row or Column in accordance with the main axis. Notably, the main axis of Row is the horizontal axis, and the main axis of Column is the vertical one.
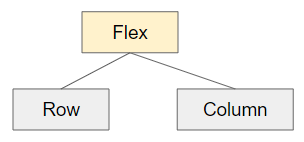
Expanded Constructor
const Expanded(
{Key key,
int flex: 1,
@required Widget child}
)
First of all, please take the illustration below as an example. In this example, a Row object has five child widgets. The question is how to horizontally expand the space for the 2nd and the 4th child widgets.
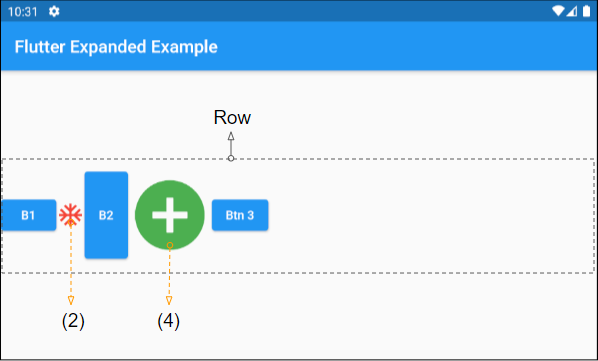
Row (
children: [
ElevatedButton(child: Text("B1"), onPressed:(){}),
Icon(Icons.ac_unit, size: 32, color: Colors.red),
ElevatedButton(
child: Text("B2"),
onPressed:(){},
style: ButtonStyle(
minimumSize: MaterialStateProperty.all(Size(50, 100))
)
),
Icon(Icons.add_circle, size: 96, color: Colors.green),
ElevatedButton(child: Text("Btn 3"), onPressed:(){}),
]
)
Wrapping a Row child widget in an Expanded object helps it expand the space horizontally and take up the rest of the Row space.
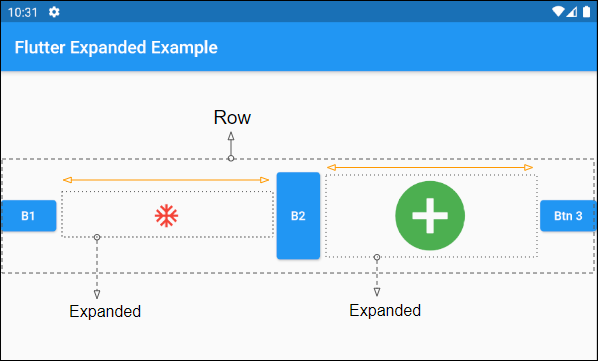
Row (
children: [
ElevatedButton(child: Text("B1"), onPressed:(){}),
Expanded(
child: Icon(Icons.ac_unit, size: 32, color: Colors.red),
),
ElevatedButton(
child: Text("B2"),
onPressed:(){},
style: ButtonStyle(
minimumSize: MaterialStateProperty.all(Size(50, 100))
)
),
Expanded(
child: Icon(Icons.add_circle, size: 96, color: Colors.green),
),
ElevatedButton(child: Text("Btn 3"), onPressed:(){}),
]
)
3. flex
flex propertyis considered the weight of the Expanded. It determines how much space will be allocated to the Expanded. The allocated space is proportional to the flex value. The default value of flex is 1.
int flex: 1
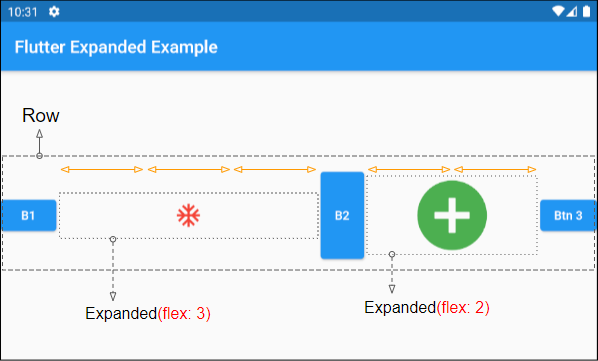
flex (ex1)
Row (
children: [
ElevatedButton(child: Text("B1"), onPressed:(){}),
Expanded(
child: Icon(Icons.ac_unit, size: 32, color: Colors.red),
flex: 3
),
ElevatedButton(
child: Text("B2"),
onPressed:(){},
style: ButtonStyle(
minimumSize: MaterialStateProperty.all(Size(50, 100))
)
),
Expanded(
child: Icon(Icons.add_circle, size: 96, color: Colors.green),
flex: 2
),
ElevatedButton(child: Text("Btn 3"), onPressed:(){}),
]
)
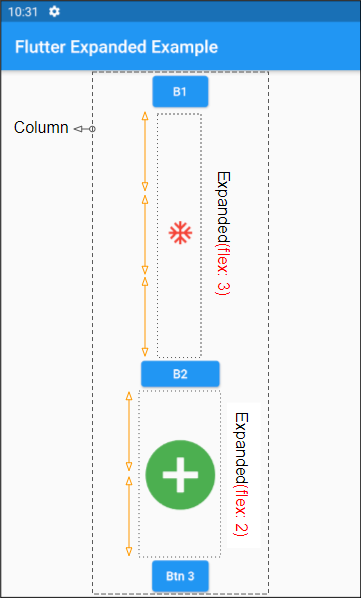
flex (ex2)
Column (
children: [
ElevatedButton(child: Text("B1"), onPressed:(){}),
Expanded(
child: Icon(Icons.ac_unit, size: 32, color: Colors.red),
flex: 3
),
ElevatedButton(
child: Text("B2"),
onPressed:(){},
style: ButtonStyle(
minimumSize: MaterialStateProperty.all(Size(90, 30))
)
),
Expanded(
child: Icon(Icons.add_circle, size: 96, color: Colors.green),
flex: 2
),
ElevatedButton(child: Text("Btn 3"), onPressed:(){}),
]
)
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More