Flutter Center Tutorial with Examples
1. Flutter Center
Center is a widget that places the only child widget in its center.
Center and Align are fairly similar. They only have one child widget, but Align allows you to customize the position of the child widget within it.
Center Constructor
const Center(
{Key key,
double widthFactor,
double heightFactor,
Widget child}
)
If widthFactor is not specified, the width of Center will be as large as possible, otherwise, the width of Center is equal to the width of the child multiplied by the widthFactor. The heightFactor parameter also has the same behavior for the height of the Center. So by default, the size of the Center will be as large as possible.
Center(
child: Icon (
Icons.place,
size: 128,
color: Colors.redAccent
)
)
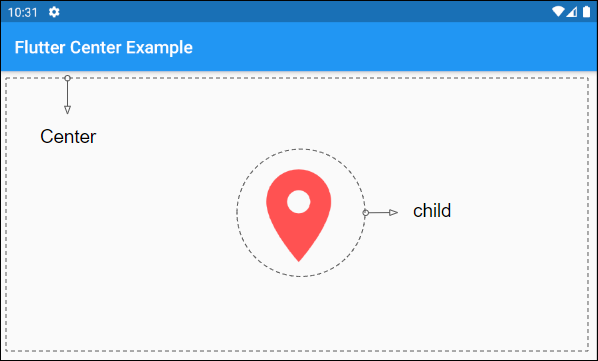
main.dart (ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter Center Example")
),
body: Center(
child: Icon (
Icons.place,
size: 128,
color: Colors.redAccent
)
),
);
}
}
2. child
child is the only child widget of the Center. In some use cases, it can be the Row, Column or Stack object in order to possibly contain many other widgets.
Widget child
For example, if a child is the Row object, it can contain many child widgets on a row.
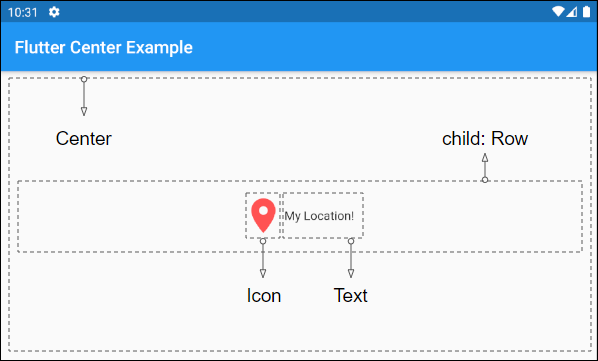
child (ex1)
Center (
child: Row (
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon (
Icons.place,
size: 48,
color: Colors.redAccent
),
Text("My Location!")
],
)
)
For example, if a child is the Column object, it can contain many child widgets on a column.
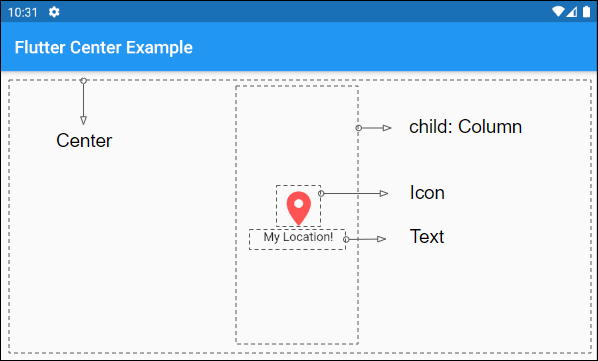
child (ex2)
Center (
child: Column (
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon (
Icons.place,
size: 48,
color: Colors.redAccent
),
Text("My Location!")
],
)
)
3. widthFactor
widthFactor is a factor, which is used to calculate the width of the Center based on the width of the child. If widthFactor is not null, the width of Center is equal to the width of the child multiplied by this factor.
If widthFactor is not specified, the width of the Center will be as large as possible.
double widthFactor
Example:
widthFactor (ex1)
Center(
child: ElevatedButton (
child: Text("Button"),
onPressed: () {}
),
widthFactor: 2.0
)
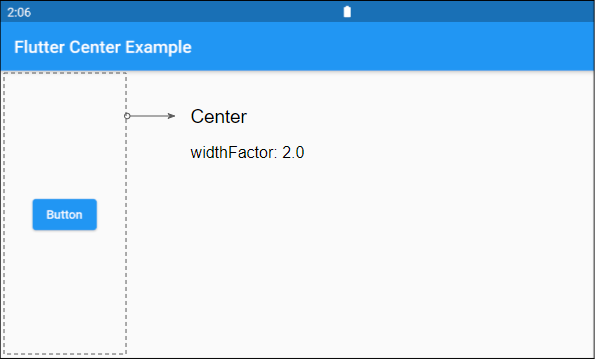
4. heightFactor
heightFactor is a factor, which is used to calculate the height of the Center based on the height of the child. If widthFactor is not null, the height of Center is equal to the height of the child multiplied by this factor.
If heightFactor is not specified the height of the Center will be as large as possible.
double heightFactor
Example:
heightFactor (ex1)
Center(
child: ElevatedButton (
child: Text("Button"),
onPressed: () {}
),
heightFactor: 3.0
)
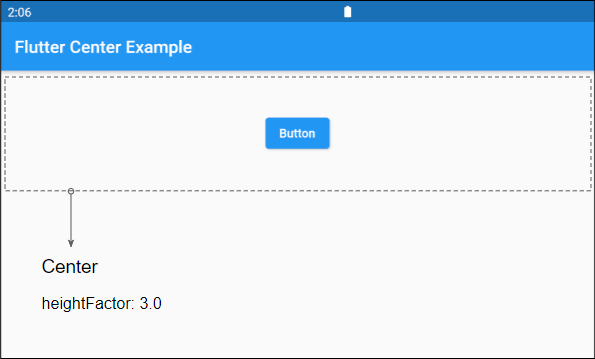
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More