Flutter EdgeInsetsGeometry Tutorial with Examples
1. Flutter EdgeInsetsGeometry
EdgeInsetsGeometry is used to create an inner padding or an outer padding for a Widget.
In computer science, the term "margin" is used in place of the "outer padding", and the "padding" is used to in place of the "inner padding".
Let's take a look at this simple illustration, a Container widget before and after being added the "outer padding" and "inner padding":
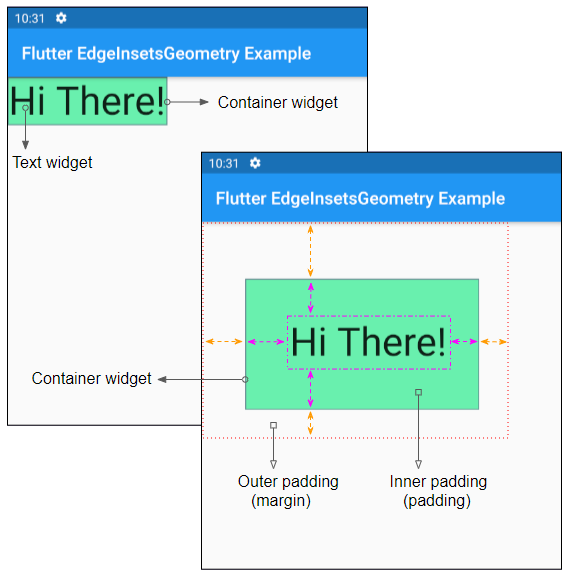
EdgeInsetsGeometry is an abstract class, so it cannot be directly used, depending on the situation you can use its subclass EdgeInsets or EdgeInsetsDirectional.
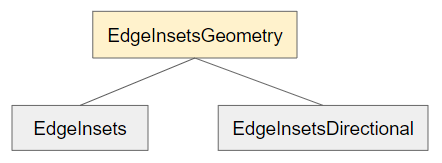
- Flutter EdgeInsets
- Flutter EdgeInsetsDirectional
2. EdgeInsets
EdgeInsets helps create the outer padding (or the inner padding) based on the visual parameters, left, top, right and bottom without depending on the text direction.
For example, you use EdgeInsets to create an "Outer padding" (margin) with the parameters (left, top, right, bottom) = (90, 70, 50, 30):
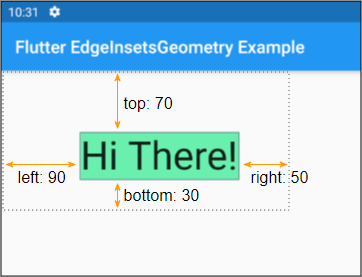
Container(
margin: EdgeInsets.fromLTRB(90, 70, 50, 30),
decoration: BoxDecoration(
color: Colors.greenAccent,
border: Border.all(color: Colors.blueGrey),
),
child: Text(
"Hi There!",
style: TextStyle(fontSize: 45)
)
)
3. EdgeInsetsDirectional
EdgeInsetsDirectional helps create the outer padding (or the inner padding) based on the parameters, start, top, end and bottom. Both start and end parameters depend on the text direction.
Specifically:
- If the text direction is "Left to Right", "start" will correspond to "left" and "end" will correspond to "right".
- Otherwise, if the text direction is "Right to Left", "start" will correspond to "right" and "end" will correspond to "left".
For example, you use EdgeInsetsDirectional to create an "Outer padding" (margin) with the parameters (start, top, end, bottom) = (150, 70, 50, 30). You will get two different results depending on the text direction:
textDirection = TextDirection.rtl (Right to Left):
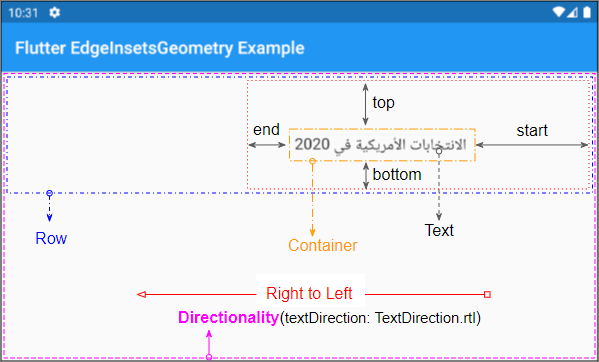
main.dart (ex4)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage()
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter EdgeInsetsGeometry Example")
),
body: Directionality (
textDirection: TextDirection.rtl,
child: Row (
children: [
Container (
margin: EdgeInsetsDirectional.fromSTEB(150, 70, 50, 30),
child:Text(
"الانتخابات الأمريكية في 2020",
style: TextStyle(fontSize: 18)
)
)
],
)
)
);
}
}
- Flutter EdgeInsetsDirectional
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More