Android DialogFragment Tutorial with Examples
1. Android DialogFragment
Android DialogFragment is a fragment containing a Dialog. It controls the operation of the Dialog, such as determines when to hide, display, or dismiss this Dialog. You should interact with the Dialog through the methods of DialogFragment instead of interacting directly.
You might ask the question: "Why do they need a DialogFragment when we already have a Dialog?". The answer is DialogFragment was created to overcome some of Dialog's weaknesses.
TODO
See more at:
It is easy for you to get a DialogFragment, just take 2 steps as follows:
Starting with writing a class of YourDialogFragment extended from the DialogFragment class. And then override the onCreateDialog(Bundle) method which returns a Dialog (YourDialog). As a result, YourDialogFragment now plays a role of a container of YourDialog.
Android DialogFragment Javadocs:
2. Example of DialogFragment
In this example, we are going to create a YesNoDialogFragment class extended from the DialogFragment class. This class contains an AlertDialog with 2 buttons, YES/NO.
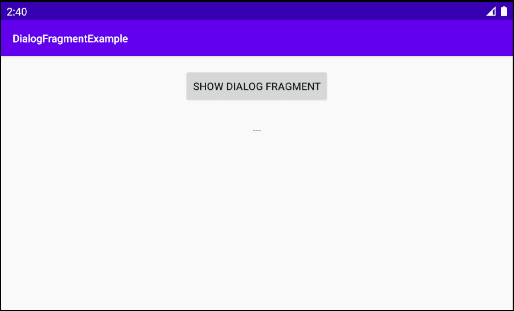
This example will showcase how to create and display YesNoDialogFragment from an Activity. You can also create and display YesNoDialogFragment from one another fragment with a little change in the code.
Alright, now on Android Studio create a project:
- File > New > New Project > Empty Activity
- Name: DialogFragmentExample
- Package name: org.o7planning.dialogfragmentexample
- Language: Java
The application interface looks like this:
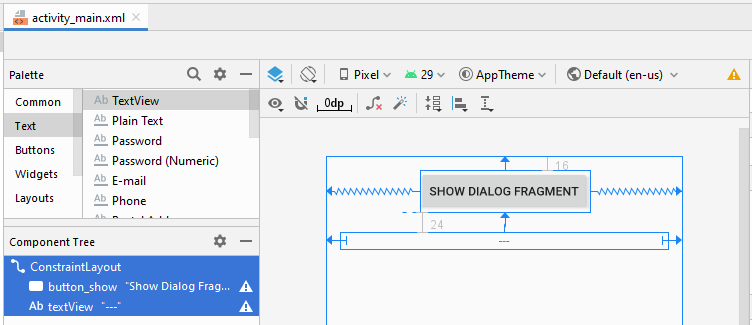
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_show"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Show Dialog Fragment"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:gravity="center"
android:text="---"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_show" />
</androidx.constraintlayout.widget.ConstraintLayout>
YesNoDialogFragment.java
package org.o7planning.dialogfragmentexample;
import android.app.Activity;
import android.app.Dialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AlertDialog;
import androidx.fragment.app.DialogFragment;
public class YesNoDialogFragment extends DialogFragment {
public static final String ARG_TITLE = "YesNoDialog.Title";
public static final String ARG_MESSAGE = "YesNoDialog.Message";
private YesNoDialogFragmentListener listener;
public interface YesNoDialogFragmentListener {
/**
*
* @param resultCode - Activity.RESULT_OK, Activity.RESULT_CANCEL
* @param data
*/
public void onYesNoResultDialog(int resultCode, @Nullable Intent data);
}
public YesNoDialogFragment() {
}
public void setOnYesNoDialogFragmentListener(YesNoDialogFragmentListener listener) {
this.listener = listener;
}
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Bundle args = getArguments();
String title = args.getString(ARG_TITLE);
String message = args.getString(ARG_MESSAGE);
return new AlertDialog.Builder(getActivity())
.setTitle(title)
.setMessage(message)
.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
buttonOkClick();
}
})
.setNegativeButton("No", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
buttonNoClick();
}
})
.create();
}
private void buttonOkClick() {
int resultCode = Activity.RESULT_OK;
Bundle bundle = new Bundle();
bundle.putString("key1", "Value 1");
bundle.putString("key2", "Value 2");
Intent data = new Intent().putExtras(bundle);
// Open this DialogFragment from an Activity.
if(this.listener != null) {
this.listener.onYesNoResultDialog(Activity.RESULT_OK, data);
}
// Open this DialogFragment from another Fragment.
else {
// Send result to your TargetFragment.
// See (Your) TargetFragment.onActivityResult()
getTargetFragment().onActivityResult(getTargetRequestCode(), resultCode, data);
}
}
private void buttonNoClick() {
int resultCode = Activity.RESULT_CANCELED;
Intent data = null;
// Open this DialogFragment from an Activity.
if(this.listener != null) {
this.listener.onYesNoResultDialog(resultCode, data);
}
// Open this DialogFragment from another Fragment.
else {
// Send result to your TargetFragment.
// See (Your) TargetFragment.onActivityResult()
getTargetFragment().onActivityResult(getTargetRequestCode(), resultCode, data);
}
}
}
MainActivity.java
package org.o7planning.dialogfragmentexample;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import androidx.fragment.app.DialogFragment;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity
implements YesNoDialogFragment.YesNoDialogFragmentListener {
private Button buttonShow;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.buttonShow = (Button) this.findViewById(R.id.button_show);
this.textView = (TextView) this.findViewById(R.id.textView);
this.buttonShow.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
buttonShowClicked();
}
});
}
// User click on "Show Dialog" button.
private void buttonShowClicked() {
this.textView.setText("---");
// Create YesNoDialogFragment
DialogFragment dialogFragment = new YesNoDialogFragment();
// Arguments:
Bundle args = new Bundle();
args.putString(YesNoDialogFragment.ARG_TITLE, "Confirmation");
args.putString(YesNoDialogFragment.ARG_MESSAGE, "Do you like this example?");
dialogFragment.setArguments(args);
FragmentManager fragmentManager = this.getSupportFragmentManager();
// Show:
dialogFragment.show(fragmentManager, "Dialog");
}
@Override
public void onAttachFragment(Fragment fragment) {
if (fragment instanceof YesNoDialogFragment) {
YesNoDialogFragment yesNoDialogFragment = (YesNoDialogFragment) fragment;
yesNoDialogFragment.setOnYesNoDialogFragmentListener(this);
}
}
// Implement method of YesNoDialogFragment.YesNoDialogFragmentListener
@Override
public void onYesNoResultDialog(int resultCode, @Nullable Intent data) {
if(resultCode == Activity.RESULT_OK) {
String value1 = data.getStringExtra("key1"); // ...
this.textView.setText("You select YES");
} else if(resultCode == Activity.RESULT_CANCELED) {
this.textView.setText("You select NO");
} else {
this.textView.setText("You don't select");
}
}
}
Below is the code to create and display YesNoDialogFragment from another Fragment.
ExampleFragment.java
package org.o7planning.dialogfragmentexample;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import androidx.annotation.Nullable;
import androidx.fragment.app.DialogFragment;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Toast;
public class ExampleFragment extends Fragment {
private static final int REQUEST_CODE_YESNO_DIALOG_FRAGMENT = 1000;
public ExampleFragment() {
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_example, container, false);
}
// Call this method to show YesNoDialogFragment
private void showYesNoDialogFragment() {
// Create YesNoDialogFragment
DialogFragment dialogFragment = new YesNoDialogFragment();
// Arguments:
Bundle args = new Bundle();
args.putString(YesNoDialogFragment.ARG_TITLE, "Confirmation");
args.putString(YesNoDialogFragment.ARG_MESSAGE, "Do you like this example?");
dialogFragment.setArguments(args);
// YesNoDialogFragment will return results for targetFragment.
// So, Need to Override onActivityResult() method, to get results.
Fragment targetFragment = this;
// IMPORTANT!!
dialogFragment.setTargetFragment(targetFragment, REQUEST_CODE_YESNO_DIALOG_FRAGMENT);
FragmentManager fragmentManager = this.getFragmentManager();
// Show:
dialogFragment.show(fragmentManager, "Dialog");
}
// Results are returned by YesNoDialogFragment.
@Override
public void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(requestCode == REQUEST_CODE_YESNO_DIALOG_FRAGMENT) {
if(resultCode == Activity.RESULT_OK) {
Toast.makeText(this.getContext(), "You select YES", Toast.LENGTH_LONG).show();
} else if(resultCode == Activity.RESULT_CANCELED) {
Toast.makeText(this.getContext(), "You select NO", Toast.LENGTH_LONG).show();
}
}
}
}
Android Programming Tutorials
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Configure Android Emulator in Android Studio
- Android Tutorial for Beginners - Hello Android
- Android Tutorial for Beginners - Basic examples
- Using image assets and icon assets of Android Studio
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Setting SD Card for Android Emulator
- How to know the phone number of Android Emulator and change it
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android UI Layouts Tutorial with Examples
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android Button Tutorial with Examples
- Android ToggleButton Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- ChipGroup and Chip Entry Example
- Android QuickContactBadge Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextInputLayout Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Create a simple File Finder Dialog in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android DialogFragment Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android SMS Tutorial with Examples
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
Show More