Android Switch Tutorial with Examples
1. Android Switch
In Android, Switch is a user interface control with the two ON/OFF states. Though its features are quite similar to CheckBox and ToggleButton, but different in terms of interface.

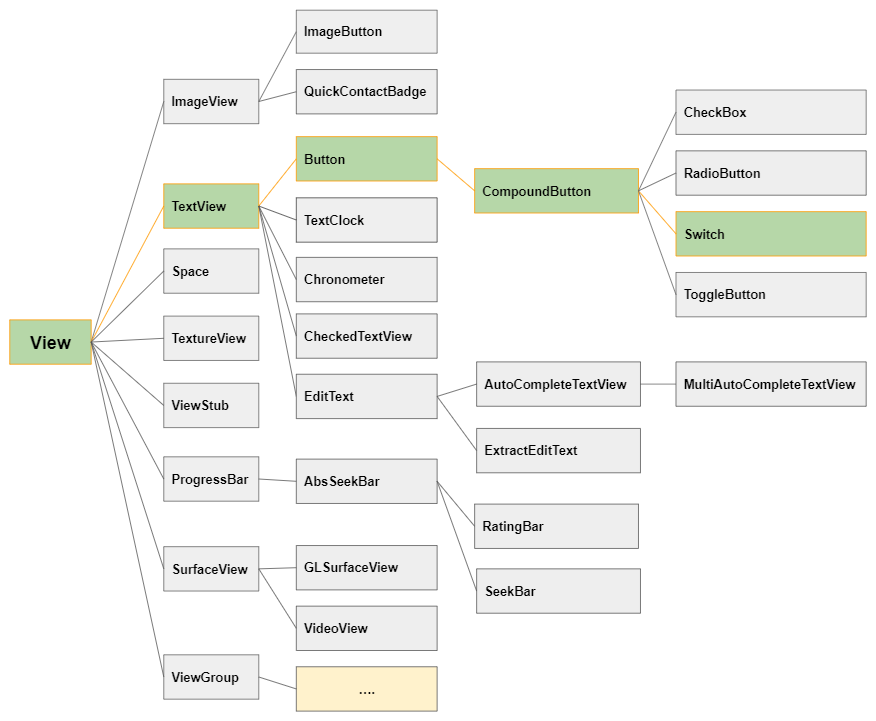
Switch is rather similar to CheckBox and ToggleButton in terms of features and usage. All three classes are subclasses of CompoundButton, and the difference lies in their interface.
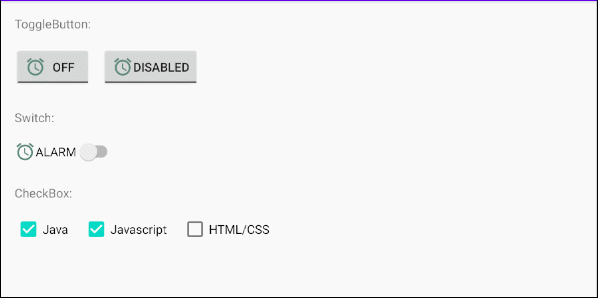
Image (Icon)
Switch is a subclass of Button, so it allows you to display four icons maximum near the four edges by using the attributes of android:drawableLeft, android:drawableTop, android:drawableRight, android:drawableBottom, android:drawableStart, android:drawableEnd.
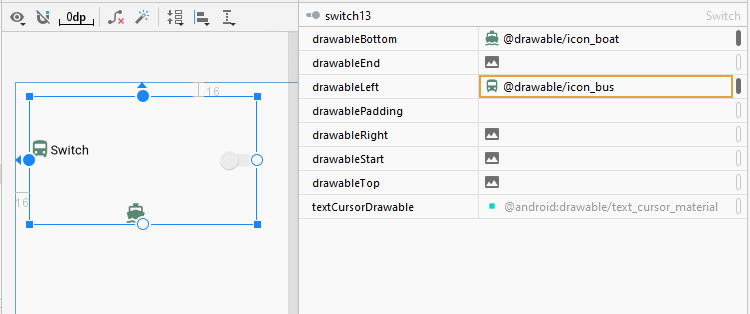
<Switch
android:id="@+id/switch13"
android:drawableLeft="@drawable/icon_bus"
android:drawableTop="@drawable/icon_car"
android:drawableBottom="@drawable/icon_boat"
android:text="Switch"
... />
android:gravity
The android:gravity attribute is used to set the text display position of a Switch. Its value is a combination of the following values:
Constant in Java | Value | Description |
Gravity.LEFT | left | |
Gravity.CENTER_HORIZONTAL | center_horizontal | |
Gravity.RIGHT | right | |
Gravity.TOP | top | |
Gravity.CENTER_VERTICAL | center_vertical | |
Gravity.BOTTOM | bottom | |
Gravity.START | start | |
Gravity.END | end | |
Gravity.CENTER | center | |
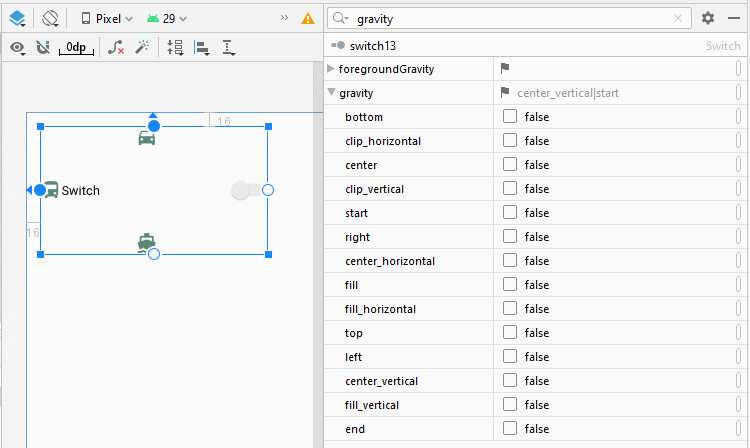
<Switch
android:id="@+id/switch13"
android:text="Switch"
android:gravity="bottom|center"
... />
android:switchPadding
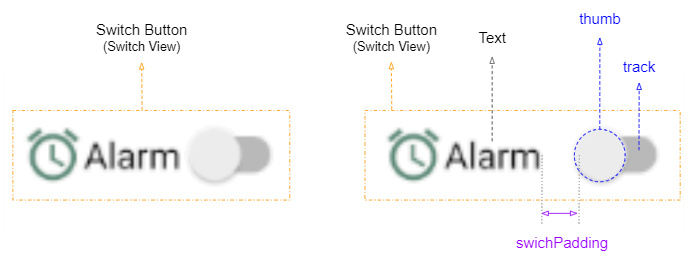
The android:switchPadding allows you to set space between the track and the text of the Switch.
<Switch
android:id="@+id/switch1"
android:drawableLeft="@drawable/icon_alarm"
android:switchPadding="10dp"
android:text="Alarm"
... />
android:layoutDirection = "rtl"
The android:layoutDirection is supported from Android 4.2 (API Level 17), which allows you to set the layout direction of a View. By default the value of this attribute is "ltr" (Left to Right).
In order to use the android:layoutDirection attribute, you need to open the build.gradle (Module: app) file, then modify the value of minSdkVersion and make sure that the new value is equal or greater than 17.
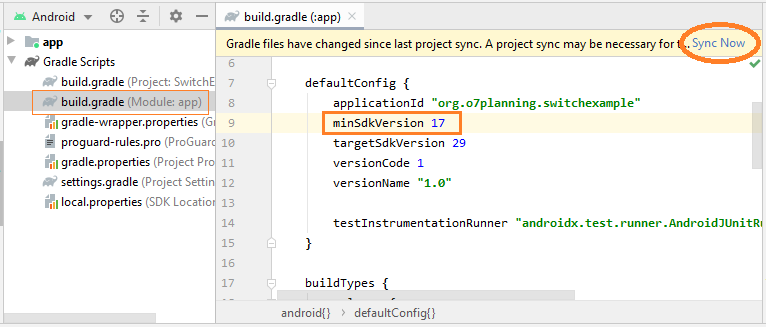
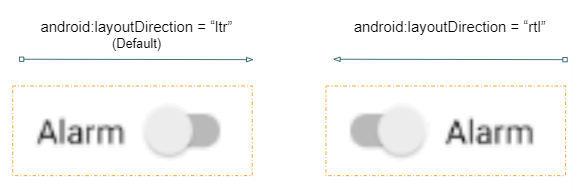
<!-- Layout Direction Default: Left to Right -->
<Switch
android:id="@+id/switch41"
android:switchPadding="5dp"
android:text="Alarm"
... />
<!-- Layout Direction: Right to Left -->
<Switch
android:id="@+id/switch42"
android:layoutDirection="rtl"
android:switchPadding="5dp"
android:text="Alarm"
... />
textOn/textOff
Android 5.0 (API Level 21) allows textOn/textOff to be displayed corresponding to the ON/OFF states of the Switch.

<!-- textOn/textOff = ON/OFF (Default) -->
<Switch
android:id="@+id/switch51"
android:text="Alarm"
android:showText="true"
... />
<!-- textOn/textOff = Enabled/Disabled -->
<Switch
android:id="@+id/switch52"
android:text="Alarm"
android:showText="true"
android:textOff="Disabled"
android:textOn="Enabled"
... />
toggle()
All 4 classes of ToggleButton, CheckBox, RadioButton, Switch are subclasses of CompoundButton, so they inherit the toggle() method, which is the commonly used method to change their state from ON (Checked) to OFF (Unchecked), and vice versa.
CompoundButton button = (Switch) findViewById(R.id.switch);
button.toggle();
2. Switch Styles
The style attribute is an option of Switch, which allows you to set the style for the Switch. There are several styles available in Android library that may be ready to use.
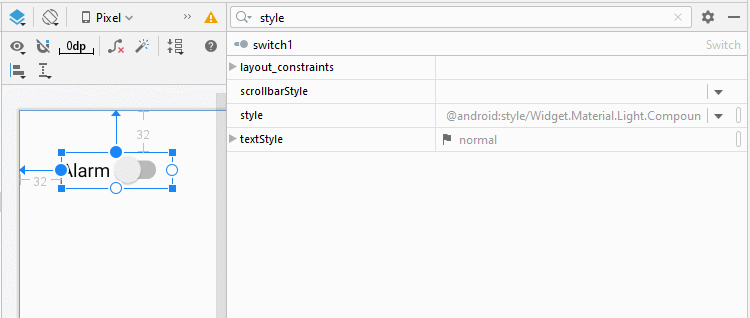
Note: Currently, there are not many available style(s) in the library, and they are not really different from the default styles. This is such a disappointing thing.
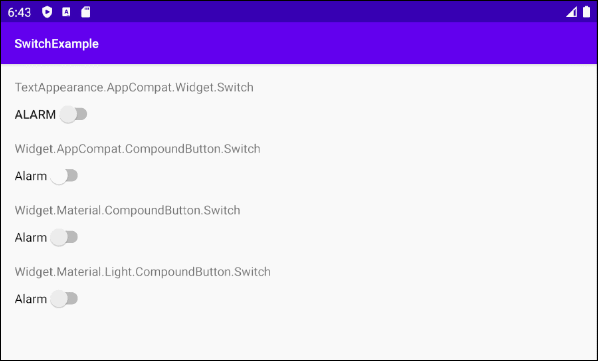
Switch Styles Example
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView61"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:text="TextAppearance.AppCompat.Widget.Switch"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Switch
android:id="@+id/switch61"
style="@style/TextAppearance.AppCompat.Widget.Switch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:showText="false"
android:text="Alarm"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView61" />
<TextView
android:id="@+id/textView62"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:text="Widget.AppCompat.CompoundButton.Switch"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/switch61" />
<Switch
android:id="@+id/switch62"
style="@style/Widget.AppCompat.CompoundButton.Switch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:showText="false"
android:text="Alarm"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView62" />
<TextView
android:id="@+id/textView63"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:text="Widget.Material.CompoundButton.Switch"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/switch62" />
<Switch
android:id="@+id/switch63"
style="@android:style/Widget.Material.CompoundButton.Switch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:showText="false"
android:text="Alarm"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView63" />
<TextView
android:id="@+id/textView64"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:text="Widget.Material.Light.CompoundButton.Switch"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/switch63" />
<Switch
android:id="@+id/switch64"
style="@android:style/Widget.Material.Light.CompoundButton.Switch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:showText="false"
android:text="Alarm"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView64" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. Switch Events
There are quite a few events related to a Switch; however, the following two events are most frequently used:
- setOnClickListener(View.OnClickListener)
- setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
On Click Event:
The event happens when the user clicks the Switch. It is the same as the action of the user clicking a Button.
Switch switch1 = (Switch) findViewById(R.id.switch1);
switch1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
boolean checked = ((Switch) v).isChecked();
if (checked){
// Your code
}
else{
// Your code
}
}
});
On Checked Change Event:
The event happens when the Switch changes its state due to the user's action or the effect of calling the setChecked(newState) method, etc.
Switch switch1 = (ToggleButton) findViewById(R.id.switch1);
switch1.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked) {
// Your code
} else {
// Your code
}
}
});
Android Programming Tutorials
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Configure Android Emulator in Android Studio
- Android Tutorial for Beginners - Hello Android
- Android Tutorial for Beginners - Basic examples
- Using image assets and icon assets of Android Studio
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Setting SD Card for Android Emulator
- How to know the phone number of Android Emulator and change it
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android UI Layouts Tutorial with Examples
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android Button Tutorial with Examples
- Android ToggleButton Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- ChipGroup and Chip Entry Example
- Android QuickContactBadge Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextInputLayout Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Create a simple File Finder Dialog in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android DialogFragment Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android SMS Tutorial with Examples
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
Show More