Android SharedPreferences Tutorial with Examples
1. What is SharedPreferences?
First to clarify what is SharedPreferences, you can see a situation:
You're playing a game on Android, before playing the game, you choose the parameters of the game such as brightness, volume levels, and difficulty level. After finishing, you can turn off the game and continue to play the next day. SharedPreferences allows you to save all the parameters you have set previously, and allow you to replay the settings that can be used without having to reset.
You're playing a game on Android, before playing the game, you choose the parameters of the game such as brightness, volume levels, and difficulty level. After finishing, you can turn off the game and continue to play the next day. SharedPreferences allows you to save all the parameters you have set previously, and allow you to replay the settings that can be used without having to reset.
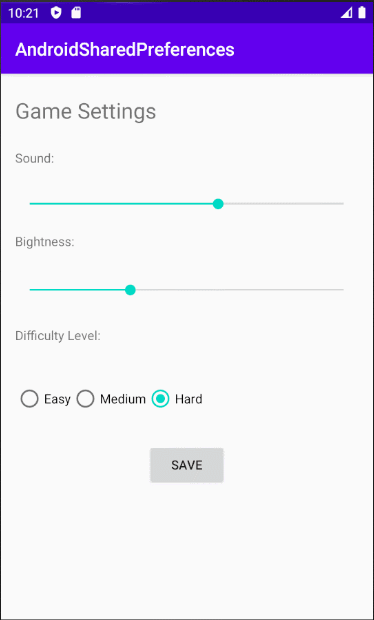
SharedPreferences saves the raw data in the form of pairs of key-value) to the files of the application. You can also choose a private storage mode that other applications can not access these files, so it is safe.
2. SharedPreferences example
Preview images of the application's interface:
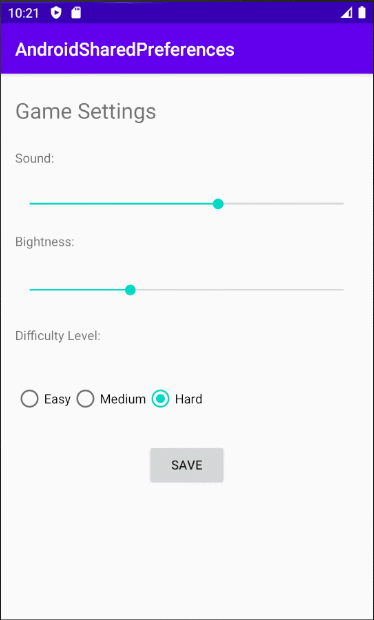
Create a project named AndroidSharedPreferences:
- File > New > New Project > Empty Activity
- Name: AndroidSharedPreferences
- Package name: org.o7planning.androidsharedpreferences
- Language: Java
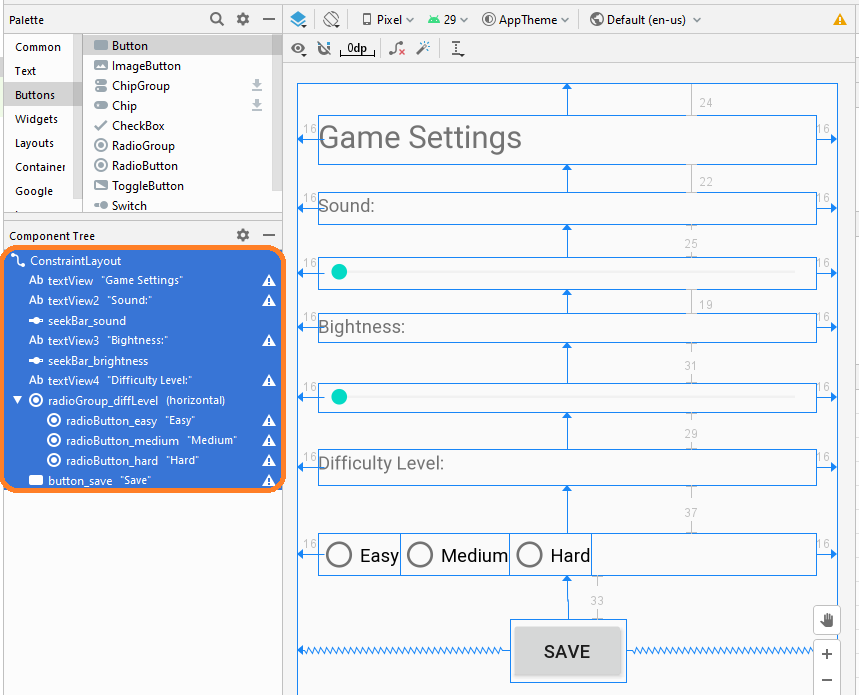
If you are interested in the steps to design this application interface, see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="37dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Game Settings"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="24dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="22dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Sound:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<SeekBar
android:id="@+id/seekBar_sound"
android:layout_width="0dp"
android:layout_height="24dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="25dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<TextView
android:id="@+id/textView3"
android:layout_width="0dp"
android:layout_height="22dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="19dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Bightness:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBar_sound" />
<SeekBar
android:id="@+id/seekBar_brightness"
android:layout_width="0dp"
android:layout_height="22dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="31dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView3" />
<TextView
android:id="@+id/textView4"
android:layout_width="0dp"
android:layout_height="27dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="29dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Difficulty Level:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBar_brightness" />
<RadioGroup
android:id="@+id/radioGroup_diffLevel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="37dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView4">
<RadioButton
android:id="@+id/radioButton_easy"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Easy" />
<RadioButton
android:id="@+id/radioButton_medium"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Medium" />
<RadioButton
android:id="@+id/radioButton_hard"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hard" />
</RadioGroup>
<Button
android:id="@+id/button_save"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="33dp"
android:text="Save"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/radioGroup_diffLevel" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidsharedpreferences;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.SeekBar;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private SeekBar seekBarSound ;
private SeekBar seekBarBrightness;
private RadioGroup radioGroupDiffLevel;
private RadioButton radioButtonEasy;
private RadioButton radioButtonMedium;
private RadioButton radioButtonHard;
private Button buttonSave;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.seekBarBrightness= (SeekBar)this.findViewById(R.id.seekBar_brightness);
this.seekBarSound= (SeekBar)this.findViewById(R.id.seekBar_sound);
this.seekBarBrightness.setMax(100);
this.seekBarSound.setMax(100);
this.radioGroupDiffLevel= (RadioGroup) this.findViewById(R.id.radioGroup_diffLevel);
this.radioButtonEasy=(RadioButton) this.findViewById(R.id.radioButton_easy);
this.radioButtonMedium = (RadioButton)this.findViewById(R.id.radioButton_medium);
this.radioButtonHard=(RadioButton) this.findViewById(R.id.radioButton_hard);
this.buttonSave = (Button) this.findViewById(R.id.button_save);
this.buttonSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
MainActivity.this.doSave(view);
}
});
// Load saved game setting.
this.loadGameSetting();
}
private void loadGameSetting() {
SharedPreferences sharedPreferences= this.getSharedPreferences("gameSetting", Context.MODE_PRIVATE);
if(sharedPreferences!= null) {
int brightness = sharedPreferences.getInt("brightness", 90);
int sound = sharedPreferences.getInt("sound",95);
int checkedRadioButtonId = sharedPreferences.getInt("checkedRadioButtonId", R.id.radioButton_medium);
this.seekBarSound.setProgress(sound);
this.seekBarBrightness.setProgress(brightness);
this.radioGroupDiffLevel.check(checkedRadioButtonId);
} else {
this.radioGroupDiffLevel.check(R.id.radioButton_medium);
Toast.makeText(this,"Use the default game setting",Toast.LENGTH_LONG).show();
}
}
// Called when user click to Save button.
public void doSave(View view) {
// The created file can only be accessed by the calling application
// (or all applications sharing the same user ID).
SharedPreferences sharedPreferences= this.getSharedPreferences("gameSetting", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putInt("brightness", this.seekBarBrightness.getProgress());
editor.putInt("sound", this.seekBarSound.getProgress());
// Checked RadioButton ID.
int checkedRadioButtonId = radioGroupDiffLevel.getCheckedRadioButtonId();
editor.putInt("checkedRadioButtonId", checkedRadioButtonId);
// Save.
editor.apply();
Toast.makeText(this,"Game Setting saved!",Toast.LENGTH_LONG).show();
}
}
After saving, you can exit application and re-open it, paramaters that you have saved will be set automatically for the game
Android Programming Tutorials
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Configure Android Emulator in Android Studio
- Android Tutorial for Beginners - Hello Android
- Android Tutorial for Beginners - Basic examples
- Using image assets and icon assets of Android Studio
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Setting SD Card for Android Emulator
- How to know the phone number of Android Emulator and change it
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android UI Layouts Tutorial with Examples
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android Button Tutorial with Examples
- Android ToggleButton Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- ChipGroup and Chip Entry Example
- Android QuickContactBadge Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextInputLayout Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Create a simple File Finder Dialog in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android DialogFragment Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android SMS Tutorial with Examples
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
Show More