Android ImageButton Tutorial with Examples
1. Android ImageButton
In Android, ImageButton is a user interface control, which displays as a button with an image, and allows the user to click to perform an action.
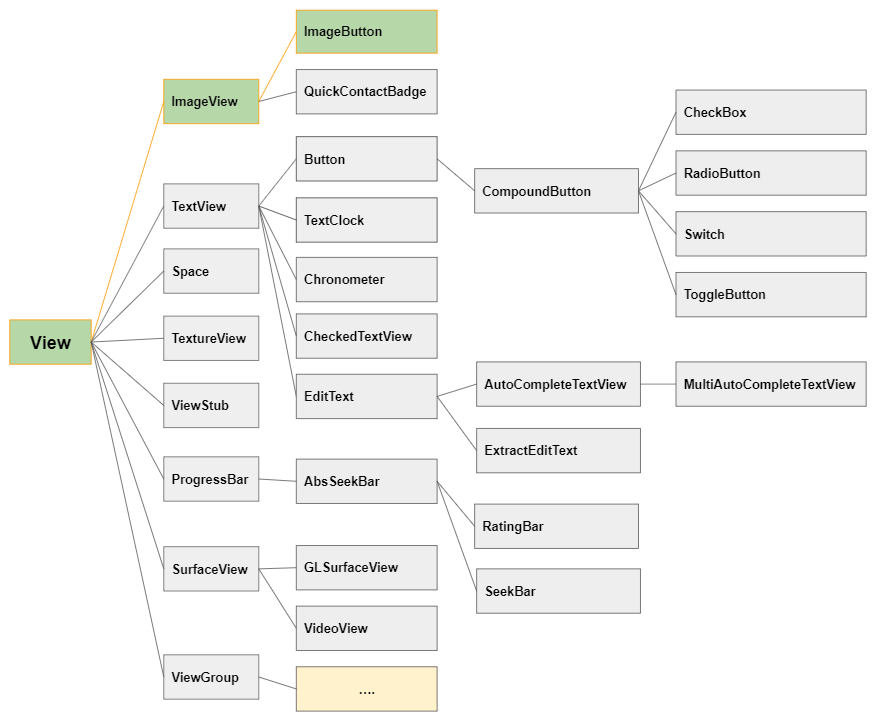
ImageButton is a subclass of ImageView, so you can set an Icon for it by using the app:srcCompat attribute. Note that unlike Button, ImageButton is not possible to display text on it.
<ImageButton
android:id="@+id/imageButton"
app:srcCompat="@drawable/icon_feel_good"
... />
There are other types of buttons that you can take a look in the following articles:
As for Java code, there are several methods that help you set the Icon for ImageButton. All of them are inherited from ImageView.
- setImageBitmap(Bitmap bitmap)
- setImageDrawable(Drawable drawable)
- setImageIcon(Icon icon)
- setImageResource(int resId)
- setImageURI(Uri uri)
2. ImageButton Styles
The style attribute is an option of ImageButton. It permits you to set styles for the ImageButton. There are some styles available in Android library that may be ready to use.
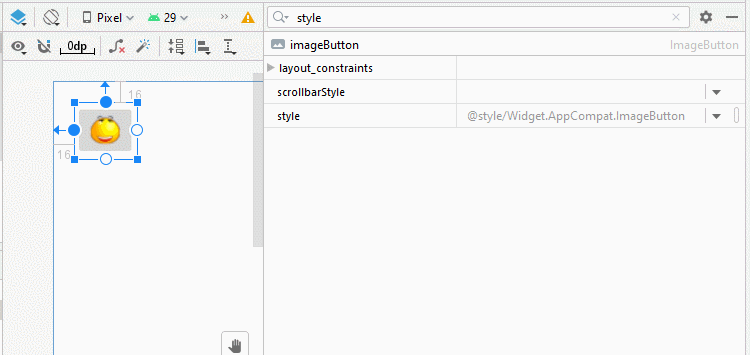
<ImageButton
android:id="@+id/imageButton"
style="@android:style/Widget.Holo.ImageButton"
app:srcCompat="@drawable/icon_feel_good"
... />
- style="@style/Widget.AppCompat.ImageButton"
- style="@android:style/Widget.Holo.ImageButton"
- style="@android:style/Widget.ImageButton"
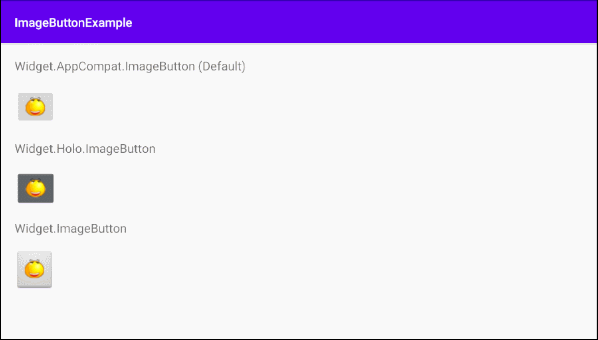
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView11"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.AppCompat.ImageButton (Default)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageButton
android:id="@+id/imageButton11"
style="@style/Widget.AppCompat.ImageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView11"
app:srcCompat="@drawable/icon_feel_good" />
<TextView
android:id="@+id/textView12"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Holo.ImageButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageButton11" />
<ImageButton
android:id="@+id/imageButton12"
style="@android:style/Widget.Holo.ImageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView12"
app:srcCompat="@drawable/icon_feel_good" />
<TextView
android:id="@+id/textView13"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.ImageButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="1.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageButton12" />
<ImageButton
android:id="@+id/imageButton13"
style="@android:style/Widget.ImageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView13"
app:srcCompat="@drawable/icon_feel_good" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. ImageButton Event
Basically, both ImageButton and Button have the same events, which you can read the Button article to learn more about these events.
The events of ImageButton and Button:
- button.setOnTouchListener(View.OnTouchListener)
- button.setOnClickListener(View.OnClickListener)
- button.setOnLongClickListener(View.OnLongClickListener)
- button.setOnContextClickListener(View.OnContextClickListener);
- ...
Android Programming Tutorials
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Configure Android Emulator in Android Studio
- Android Tutorial for Beginners - Hello Android
- Android Tutorial for Beginners - Basic examples
- Using image assets and icon assets of Android Studio
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Setting SD Card for Android Emulator
- How to know the phone number of Android Emulator and change it
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android UI Layouts Tutorial with Examples
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android Button Tutorial with Examples
- Android ToggleButton Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- ChipGroup and Chip Entry Example
- Android QuickContactBadge Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextInputLayout Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Create a simple File Finder Dialog in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android DialogFragment Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android SMS Tutorial with Examples
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
Show More