Android ImageSwitcher Tutorial with Examples
2. Android ImageSwitcher example
Create a project named AndroidImageSwitcher:
- File > New Project > Empty Activity
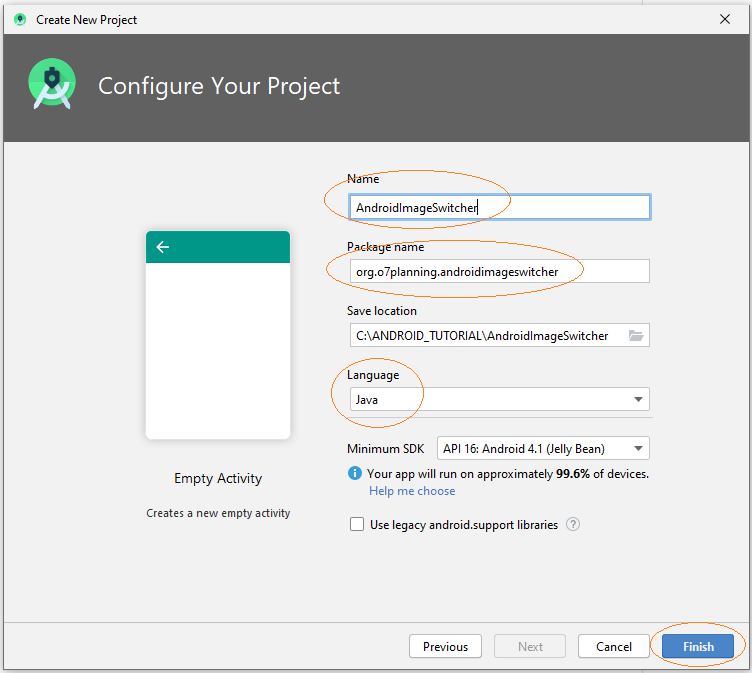
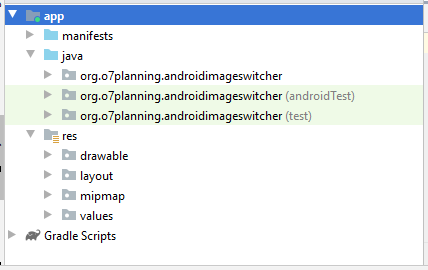
You need some images participate in the examples:
image1.png | |
image2.png | |
image3.png |
Copy the images above into drawable folder.
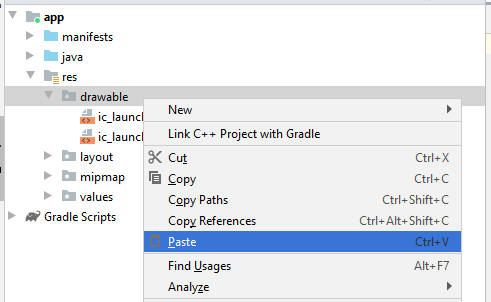
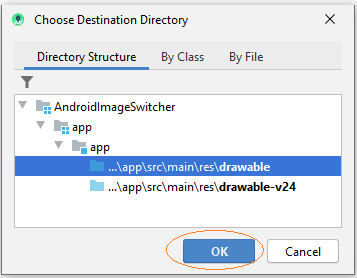
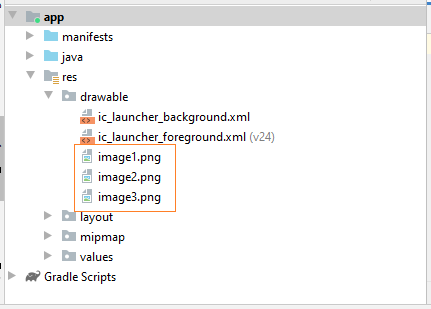
Application interface:
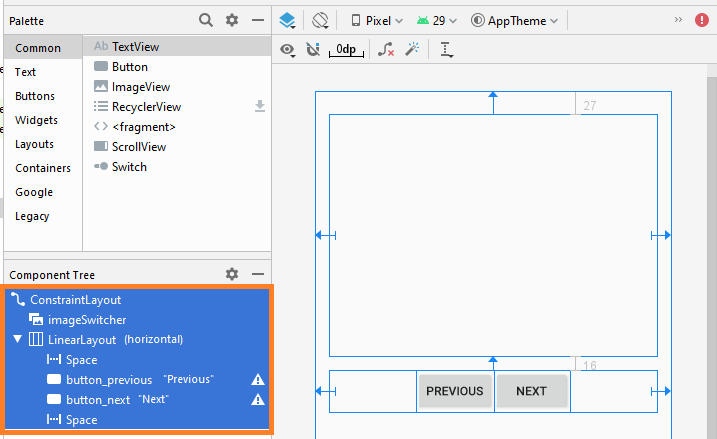
If you are interested in the steps to design the interface of this application, please see the appendix at the end of the article.
main_actvity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageSwitcher
android:id="@+id/imageSwitcher"
android:layout_width="0dp"
android:layout_height="279dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="27dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageSwitcher">
<Space
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
<Button
android:id="@+id/button_previous "
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Previous" />
<Button
android:id="@+id/button_next"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Next" />
<Space
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidimageswitcher;
import android.os.Bundle;
import android.app.ActionBar.LayoutParams;
import android.graphics.Color;
import android.util.Log;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
import android.widget.Toast;
import android.widget.ViewSwitcher.ViewFactory;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private ImageSwitcher imageSwitcher;
private Button buttonPrevious;
private Button buttonNext;
private final String[] imageNames={"image1", "image2", "image3"};
private int currentIndex;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
buttonPrevious = (Button) findViewById(R.id.button_previous);
buttonNext = (Button) findViewById(R.id.button_next);
imageSwitcher = (ImageSwitcher) findViewById(R.id.imageSwitcher);
// Animation when switching to another image.
Animation out= AnimationUtils.loadAnimation(this, android.R.anim.fade_out);
Animation in= AnimationUtils.loadAnimation(this, android.R.anim.fade_in);
// Set animation when switching images.
imageSwitcher.setInAnimation(in);
imageSwitcher.setOutAnimation(out);
//
imageSwitcher.setFactory(new ViewFactory() {
// Returns the view to show Image
// (Usually should use ImageView)
@Override
public View makeView() {
ImageView imageView = new ImageView(getApplicationContext());
imageView.setBackgroundColor(Color.LTGRAY);
imageView.setScaleType(ImageView.ScaleType.CENTER);
ImageSwitcher.LayoutParams params= new ImageSwitcher.LayoutParams(
LayoutParams.MATCH_PARENT, LayoutParams.MATCH_PARENT);
imageView.setLayoutParams(params);
return imageView;
}
});
this.currentIndex=0;
this.showImage(this.currentIndex);
buttonPrevious.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
previousImage();
}
});
buttonNext.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
nextImage();
}
});
}
private void previousImage() {
if(currentIndex > 0) {
currentIndex--;
}else {
Toast.makeText(getApplicationContext(), "No Previous Image", Toast.LENGTH_SHORT).show();
return;
}
this.showImage(currentIndex);
}
private void nextImage() {
if(currentIndex < this.imageNames.length-1) {
currentIndex++;
}else {
Toast.makeText(getApplicationContext(), "No Next Image", Toast.LENGTH_SHORT).show();
return;
}
this.showImage(currentIndex);
}
private void showImage(int imgIndex) {
String imageName= this.imageNames[imgIndex];
int resId= getDrawableResIdByName(imageName);
if(resId!= 0) {
this.imageSwitcher.setImageResource(resId);
}
}
// Find Image ID corresponding to the name of the image (in the drawable folder).
public int getDrawableResIdByName(String resName) {
String pkgName = this.getPackageName();
// Return 0 if not found.
int resID = this.getResources().getIdentifier(resName , "drawable", pkgName);
Log.i("MyLog", "Res Name: " + resName + "==> Res ID = " + resID);
return resID;
}
}
Running the apps:
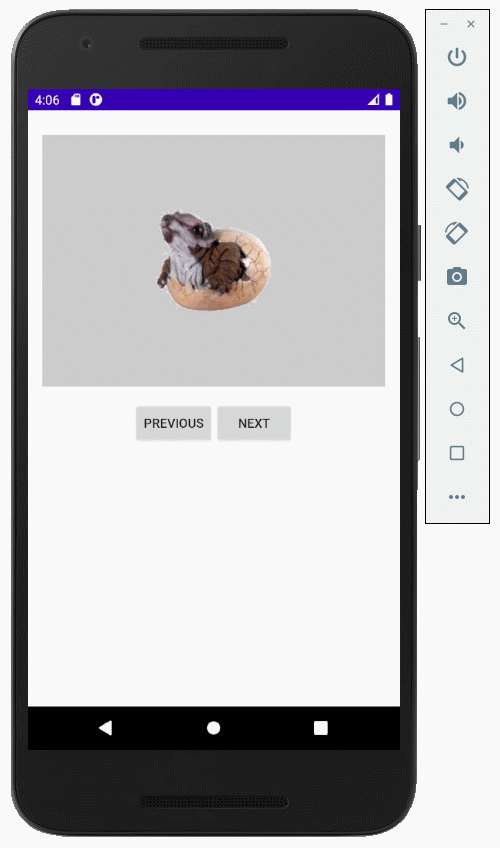
3. Appendix: Interface design
I don't know why ImageSwitcher is not available on design window of Android Studio 3.6.x, so you can't drag it into the interface. The best way is to add the following XML code to the activity_main.xml file and you will get ImageSwitcher on the interface.
<ImageSwitcher
android:layout_width="100dp"
android:layout_height="100dp" />
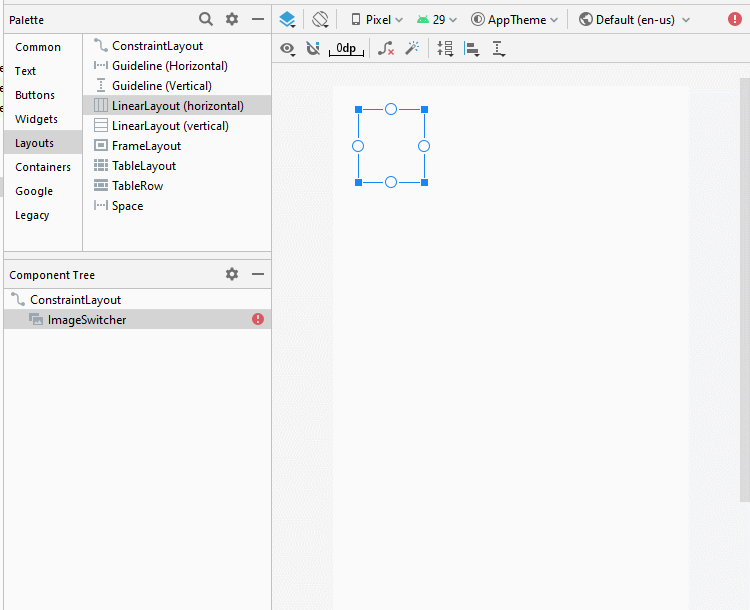
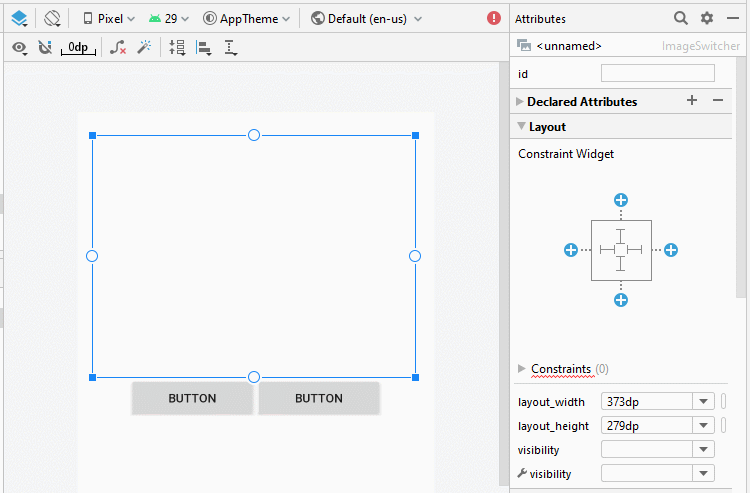
Set ID, Text for components on the interface.
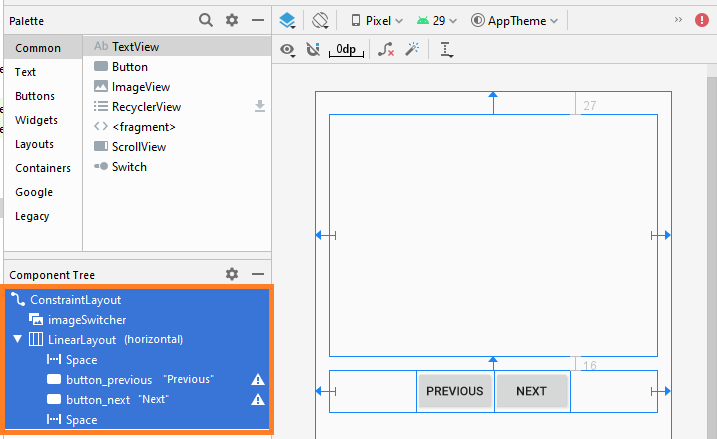
Android Programming Tutorials
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Configure Android Emulator in Android Studio
- Android Tutorial for Beginners - Hello Android
- Android Tutorial for Beginners - Basic examples
- Using image assets and icon assets of Android Studio
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Setting SD Card for Android Emulator
- How to know the phone number of Android Emulator and change it
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android UI Layouts Tutorial with Examples
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android Button Tutorial with Examples
- Android ToggleButton Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- ChipGroup and Chip Entry Example
- Android QuickContactBadge Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextInputLayout Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Create a simple File Finder Dialog in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android DialogFragment Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android SMS Tutorial with Examples
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
Show More