Android Toast Tutorial with Examples
1. Android Toast
Android Toast is a small message that the application sends to the user. It pops up close to the bottom of the screen (by default) and automatically disappears after the timeout.
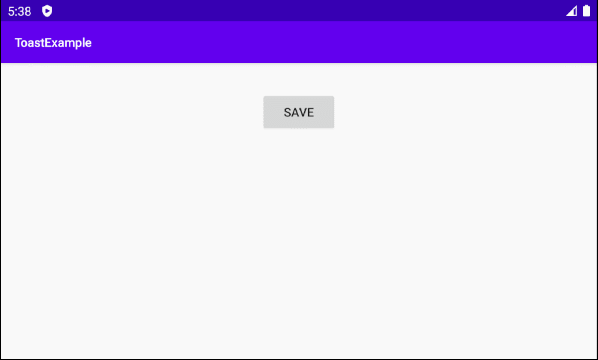
A simple syntax is:
Toast toast = Toast.makeText(context, "message", duration);
toast.show();
context
Application context
message
The message content will be displayed.
duration
It accepts either Toast.LENGTH_LONG ( 1 ) or Toast.LENGTH_SHORT ( 0 ),
- duration = Toast.LENGTH_LONG meaning Toast will be displayed for a long period of time, namely 3.5 seconds.
- duration = Toast.LENGTH_SHORT meaning Toast will be displayed for a short period of time, namely 2 seconds.
For example:
Toast.makeText(MainActivity.this, "Data has been saved successfully!", Toast.LENGTH_LONG).show();
Toast Position
By default Toast will be displayed close to the bottom of the screen and horizontally centered. By using the setGravity() method, you can change its display position.
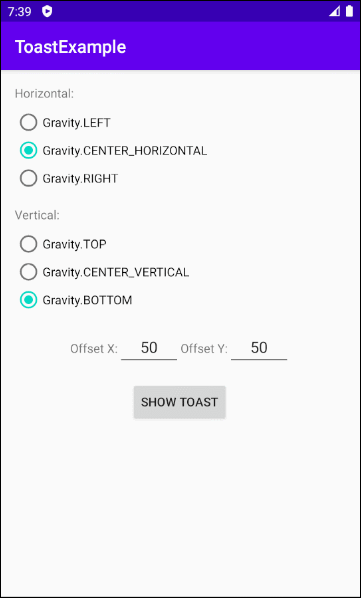
private void showOnLeftTop() {
Toast toast = Toast.makeText(MainActivity.this, "This is a message!", Toast.LENGTH_SHORT);
toast.setGravity(Gravity.LEFT | Gravity.TOP, 20, 30);
toast.show();
}
private void showOnTopRight() {
Toast toast = Toast.makeText(MainActivity.this, "This is a message!", Toast.LENGTH_SHORT);
toast.setGravity(Gravity.TOP | Gravity.RIGHT, 20, 30);
toast.show();
}
private void showOnTopCenter() {
Toast toast = Toast.makeText(MainActivity.this, "This is a message!", Toast.LENGTH_SHORT);
toast.setGravity(Gravity.TOP | Gravity.CENTER, 20, 30);
toast.show();
}
private void showOnCenter() {
Toast toast = Toast.makeText(MainActivity.this, "This is a message!", Toast.LENGTH_SHORT);
// Gravity.CENTER = Gravity.CENTER_VERTICAL | Gravity.CENTER_HORIZONTAL;
toast.setGravity(Gravity.CENTER, 20, 30);
toast.show();
}
2. Custom Toast
Basically, Toast is easy for you to use and good enough to give a notification to the user. However, if you expect a more sophisticated interface, you can customize your Toast by using the setView() method.
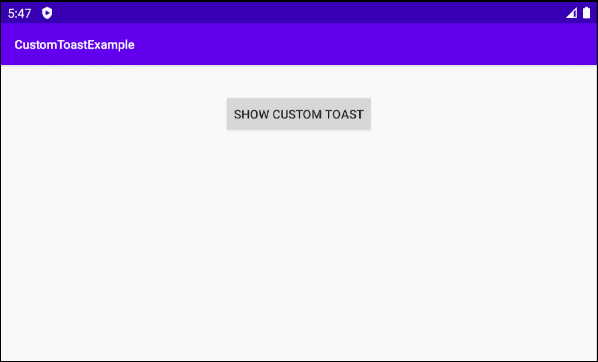
3. Android Snackbar
Snackbar is a small interface component that provides a brief response after the user's action. it appears at the bottom of the screen, and automatically disappears after the timeout or when the user interacts on a different place on the screen. Additionally, Snackbar provides an option button to perform an action. For example, undo an action that has just been performed or retry the action in case it fails.
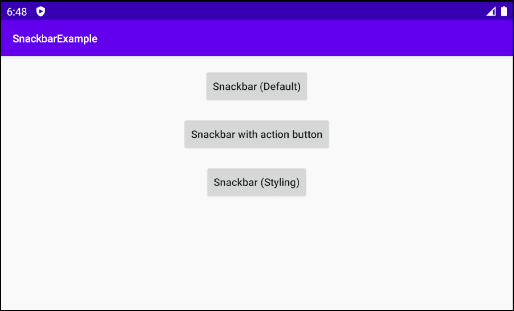
Snackbar is pretty similar to the Toast that you might be interested:
Android Programming Tutorials
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Configure Android Emulator in Android Studio
- Android Tutorial for Beginners - Hello Android
- Android Tutorial for Beginners - Basic examples
- Using image assets and icon assets of Android Studio
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Setting SD Card for Android Emulator
- How to know the phone number of Android Emulator and change it
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android UI Layouts Tutorial with Examples
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android Button Tutorial with Examples
- Android ToggleButton Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- ChipGroup and Chip Entry Example
- Android QuickContactBadge Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextInputLayout Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Create a simple File Finder Dialog in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android DialogFragment Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android SMS Tutorial with Examples
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
Show More