React-Transition-Group CSSTransition Example (NodeJS)
1. The objectives of this lesson
The react-transition-group library provides you with components so that you create animation effects in the React application. The following post introduces in details this library, its components, APIs, etc.
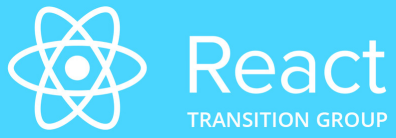
In this lesson, I will guide you to create React applications on the NodeJS environment, using the <CSSTransition> component provided by the react-transition-group library.
Note: The <CSSTransition> component is a private case helping work with CSS Transition more easily. In general case, you should work with the <Transition> component.
2. Create a project and install a library
Create a React project with the csstransition-app name by performing the following commands:
# Install 'create-react-app' tool (If it has never been installed)
npm install -g create-react-app
# Create project with 'create-react-app' tool:
create-react-app csstransition-app
Install the react-transition-group library:
# CD to your project
cd csstransition-app
# Install 'react-transition-group':
npm install react-transition-group --save
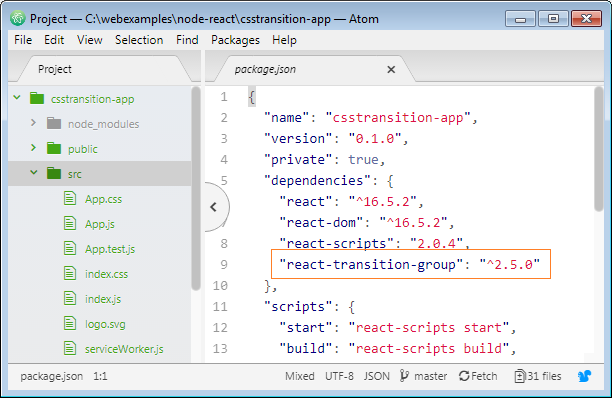
Run your application:
# Run app:
npm start
3. Simple CSSTransition example
OK, below is the preview of this example.
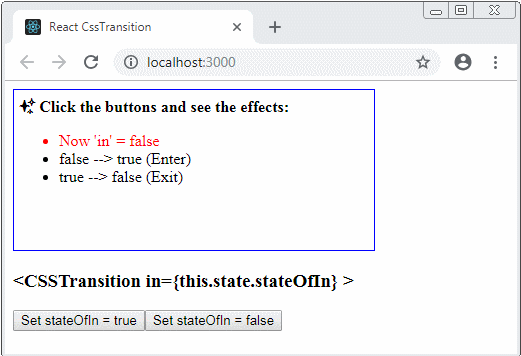
When the value of props: 'in' changes from false to true, <CSSTransition> will change to the 'entering' status and keeps in this status in'timeout' miliseconds, before changing to the 'entered' status. During such process, the CSS classes wil apply to the child element of the <CSSTransition>, like the following illustration:
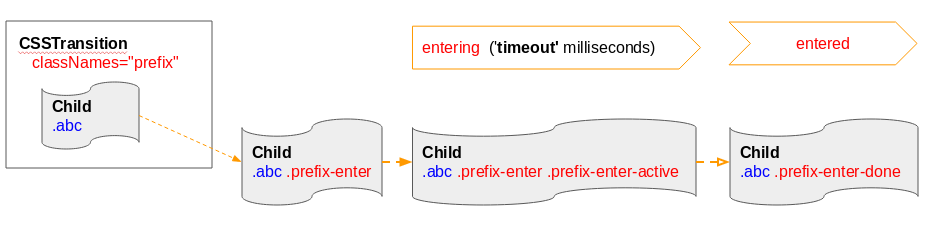
When the value of props: 'in' changes from true to false. The <CSSTransition> will change to the 'exiting'status and keeps in this status in'timeout' miliseconds, before changing to the 'exited' status. During such process, the CSS classes wil apply to the child element of the <CSSTransition>, like the following illustration:
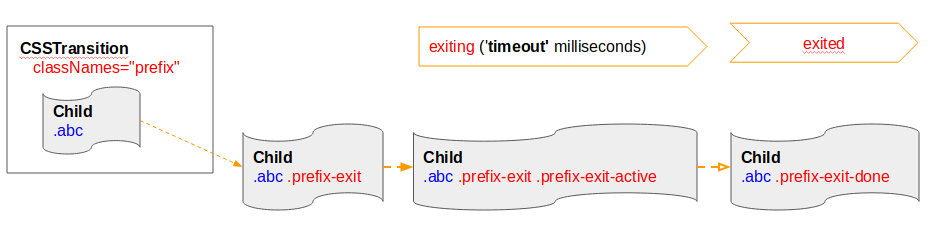
OK, Come back to the above project. Delete all the contents of the App.js & App.css files:
App.css
.example-enter {
opacity: 0.2;
transition: opacity 300ms ease-in;
background: #f9e79f;
}
.example-enter .example-enter-active {
opacity: 1;
background: #eafaf1 ;
}
.example-enter-done {
opacity: 1;
background: #eafaf1 ;
}
.example-exit {
opacity: 1;
transition: opacity 900ms ease-in;
background: #fdebd0 ;
}
.example-exit .example-exit-active {
opacity: 0.5;
background: white ;
}
.example-exit-done {
opacity: 1;
background: white;
}
.my-div {
border: 1px solid blue;
width: 350px;
height: 150px;
padding: 5px;
}
.my-message {
font-style: italic;
color: blue;
}
.my-highlight {
color: red;
}
App.js
import React, { Component } from 'react';
import logo from './logo.svg';
import './App.css';
import { TransitionGroup, CSSTransition } from "react-transition-group";
class App extends React.Component {
render() {
return (
<div>
<MyDiv></MyDiv>
</div>
);
}
}
class MyDiv extends React.Component {
constructor(props) {
super(props);
this.state = {
stateOfIn: false,
message : ""
};
}
// Begin Enter: Do anything!
onEnterHandler() {
this.setState({message: 'Begin Enter...'});
}
onEnteredHandler () {
this.setState({message: 'OK Entered!'});
}
onEnteringHandler() {
this.setState({message: 'Entering... (Wait timeout!)'});
}
// Begin Exit: Do anything!
onExitHandler() {
this.setState({message: 'Begin Exit...'});
}
onExitingHandler() {
this.setState({message: 'Exiting... (Wait timeout!)'});
}
onExitedHandler() {
this.setState({message: 'OK Exited!'});
}
render() {
return (
<div>
<CSSTransition
classNames="example"
in={this.state.stateOfIn}
timeout={1500}
onEnter = {() => this.onEnterHandler()}
onEntering = {() => this.onEnteringHandler()}
onEntered={() => this.onEnteredHandler()}
onExit={() => this.onExitHandler()}
onExiting={() => this.onExitingHandler()}
onExited={() => this.onExitedHandler()}
>
<div className ="my-div">
<b>{"\u2728"} Click the buttons and see the effects:</b>
<ul>
<li className ="my-highlight">Now 'in' = {String(this.state.stateOfIn)}</li>
<li> false --> true (Enter)</li>
<li> true --> false (Exit)</li>
</ul>
<div className="my-message">{this.state.message}</div>
</div>
</CSSTransition>
<h3><CSSTransition in={'{this.state.stateOfIn}'} ></h3>
<button onClick={() => {this.setState({ stateOfIn: true });}}>Set stateOfIn = true</button>
<button onClick={() => {this.setState({ stateOfIn: false });}}>Set stateOfIn = false</button>
</div>
);
}
}
export default App;
It is not necessary to change the contents of the index.js & index.html files:
index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(<App />, document.getElementById('root'));
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: http://bit.ly/CRA-PWA
serviceWorker.unregister();
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<link rel="manifest" href="%PUBLIC_URL%/manifest.json">
<title>React CssTransition</title>
</head>
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root"></div>
</body>
</html>
ReactJS Tutorials
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
Show More