ReactJS Lists and Keys Tutorial with Examples
1. React Lists
In Javascript, you want to create a new array from an available array by converting each element of the original array to create the corresponding element of the new array, you can use map() method.
For simplicity, please see the example, you have an array of integer numbers, such as [1, 2, 5], and you want to create another array by multiplying each element of the initial array by 10.
var arr1 = [1, 2, 5];
console.log(arr1); // --> [1, 2, 5]
var arr2 = arr1.map( e => e * 10 );
console.log(arr2); // --> [10, 20, 50]
In JSX, you also may do the same, from an array of objects, create a new array containing tags:
var array1 = [
{ empId: 1, fullName: "Trump", gender: "Male" },
{ empId: 2, fullName: "Ivanka", gender: "Female" },
{ empId: 3, fullName: "Kushner", gender: "Male" }
];
var array2 = array1.map (
e =>
<Emloyee fullName={e.fullName} gender={e.gender} />
);
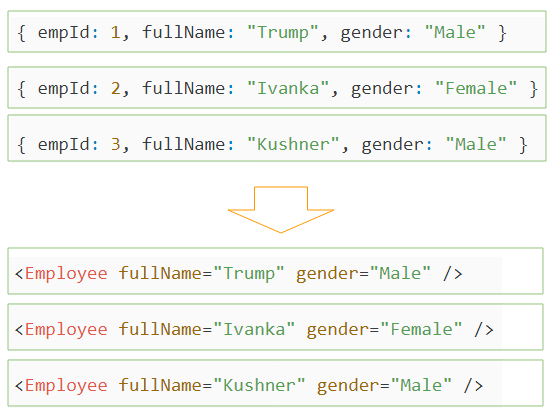
Example:
In this example, I have an array containing employees' information, and I will display this employee's information on the interface, as shown in the following illustration.
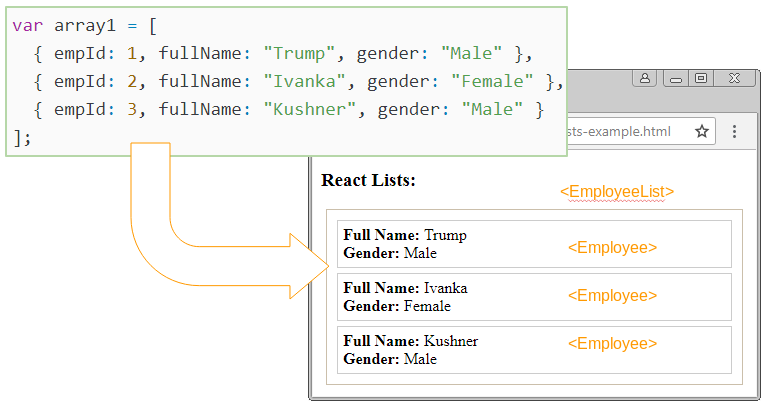
lists-example.jsx
// Employee Component
class Employee extends React.Component {
render() {
return (
<li className="employee">
<div>
<b>Full Name:</b> {this.props.fullName}
</div>
<div>
<b>Gender:</b> {this.props.gender}
</div>
</li>
);
}
}
// EmployeeList Component
class EmployeeList extends React.Component {
constructor(props) {
super(props);
this.state = {
employees: [
{ empId: 1, fullName: "Trump", gender: "Male" },
{ empId: 2, fullName: "Ivanka", gender: "Female" },
{ empId: 3, fullName: "Kushner", gender: "Male" }
]
};
}
render() {
// Array of <Employee>
var listItems = this.state.employees.map(e => (
<Employee fullName={e.fullName} gender={e.gender} />
));
return (
<ul className="employee-list">
{listItems}
</ul>
);
}
}
// Render
ReactDOM.render(<EmployeeList />, document.getElementById("employeelist1"));
lists-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Lists</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.employee-list {
border:1px solid #cbbfab;
list-style-type : none;
padding: 5px;
margin: 5px;
}
.employee {
border: 1px solid #ccc;
margin: 5px;
padding: 5px;
}
</style>
</head>
<body>
<h3>React Lists:</h3>
<div id="employeelist1"></div>
<script src="lists-example.jsx" type="text/babel"></script>
</body>
</html>
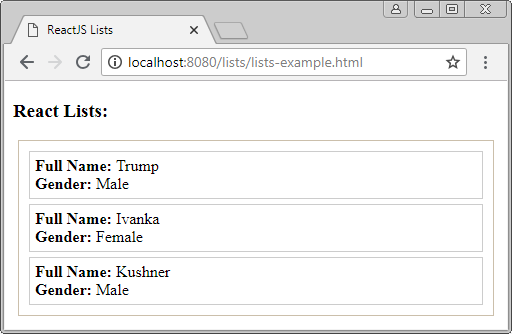
See more about Array's map() method:
2. React Keys
Keys helps React distinguish items in a list. It helps the React manage the changed items, new items added, or items removed from the list.
From an array of objects, you create a new array containing tags, which should have the key attribute, and their values are not allowed to be the same.
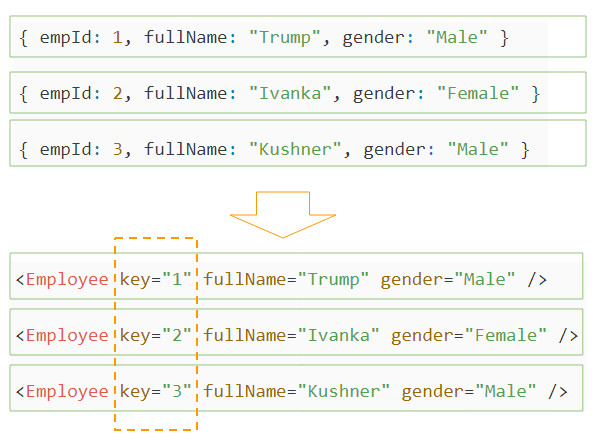
keys-example.jsx
// Employee Component
class Employee extends React.Component {
render() {
return (
<li className="employee">
<div>
<b>Full Name:</b> {this.props.fullName}
</div>
<div>
<b>Gender:</b> {this.props.gender}
</div>
</li>
);
}
}
// EmployeeList Component
class EmployeeList extends React.Component {
constructor(props) {
super(props);
this.state = {
employees: [
{ empId: 1, fullName: "Trump", gender: "Male" },
{ empId: 2, fullName: "Ivanka", gender: "Female" },
{ empId: 3, fullName: "Kushner", gender: "Male" }
]
};
}
render() {
// Array of <Employee>
var listItems = this.state.employees.map(e => (
<Employee key={e.empId} fullName={e.fullName} gender={e.gender} />
));
return (
<ul className="employee-list">
{listItems}
</ul>
);
}
}
// Render
ReactDOM.render(<EmployeeList />, document.getElementById("employeelist1"));
key-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Lists</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.employee-list {
border:1px solid #cbbfab;
list-style-type : none;
padding: 5px;
margin: 5px;
}
.employee {
border: 1px solid #ccc;
margin: 5px;
padding: 5px;
}
</style>
</head>
<body>
<h3>React Lists:</h3>
<div id="employeelist1"></div>
<script src="keys-example.jsx" type="text/babel"></script>
</body>
</html>
ReactJS Tutorials
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS
Show More