AngularJS Filters Tutorial with Examples
1. AngularJS Filters
In AngularJS, Filter is used to format the data displayed for the user
Below is a general syntax using Filter:
Filters Usage
{{ expression [| filter_name[:parameter_value] ... ] }}
AngularJS has built in some basis Filters, and you can be willing to use them.
Filter | Description |
lowercase | Format a string to lower case. |
uppercase | Format a string to upper case. |
number | Format a number to a string. |
currency | Format a number to a currency format. |
date | Format a date to a specified format. |
json | Format an object to a JSON string. |
filter | Select a subset of items from an array. |
limitTo | Limits an array/string, into a specified number of elements/characters. |
orderBy | Orders an array by an expression. |
2. uppercase/lowercase
upperlowercase-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.greeting = "Hello World";
});
upperlowercase-example
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="upperlowercase-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filters: uppercase/lowercase</h3>
<p>Origin String: {{ greeting }}</p>
<p>Uppercase: {{ greeting | uppercase }}</p>
<p>Lowercase: {{ greeting | lowercase }}</p>
</div>
</body>
</html>
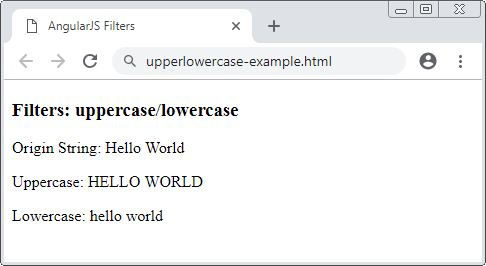
3. number
Number filterhelpsto format a number into a string.
Usage in HTML:
<!-- fractionSize: Optional -->
{{ number_expression | number : fractionSize }}
Usage in Javascript:
// fractionSize: Optional
$filter('number')( aNumber , fractionSize )
Parameters:
aNumber |
number
string | a number to be formated. |
fractionSize (Optional) | number
string | Number of decimal places. If you don't provide values for this parameter, its value will depend on locale. In case of the default locale, its value will be 3. |
number-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="number-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: number</h3>
<p><b>Origin Number:</b> {{ revenueAmount }}</p>
<p><b>Default Number Format:</b> {{ revenueAmount | number }}</p>
<p><b>Format with 2 decimal places:</b> {{ revenueAmount | number : 2 }}</p>
</div>
</body>
</html>
number-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.revenueAmount = 20011.2345;
});
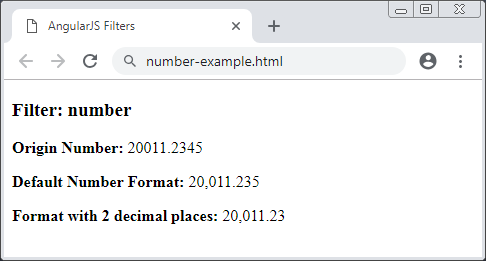
For example, use a number filter in Javascript:
number-example2.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope, $filter) {
var aNumber = 19001.2345;
// Use number filter in Javascript:
$scope.revenueAmountStr = $filter("number")(aNumber, 2);// ==> 19,001.23
});
4. currency
The currency filter is used to format some currency functions (for example, $1,234.56). Basically, you have to provide a currency symbol for formatting; On the contrary, the default currency symbol of locale will be used.
Usage in HTML:
{{ amount | currency : symbol : fractionSize}}
Usage in Javascript:
$filter('currency')(amount, symbol, fractionSize)
Parameters:
amount | number | a number |
symbol (optional) | string | Currency symbol or something equivalent to display. |
fractionSize (optional) | number | Number of decimal places. In case of not being provided, its value will be default based on the current locale of the browser. |
Example:
currency-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="currency-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
Normally, the currency symbol will come in front of amount of money (For example, €123.45), which depends on the locale. You can specify a locale that you want to use, such as locale_de-de, where you will get a result of 123.45€.
Look up the locale libraries of AngularJS:
currency-locale-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-locale-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<!-- Use locale_de-de -->
<!-- https://cdnjs.com/libraries/angular-i18n -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular-i18n/1.7.4/angular-locale_de-de.js"></script>
<script src="currency-locale-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency (LOCALE: de-de)</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
5. date
The date filter is used to format a Date object into a String.
The usage of the date filter in the HTML:
{{ date_expression | aDate : format : timezone}}
Usage of the date filter in the Javascript:
$filter('date')(aDate, format, timezone)
Parameters:
TODO
Example:
date-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myDate = new Date();
});
date-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="date-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: date</h3>
<p>
<!-- $scope.myDate = new Date() -->
<b ng-non-bindable>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</b>:
<span>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'medium'}}</b>:
<span>{{1288323623006 | date:'medium'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</b>:
<span>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'MM/dd/yyyy @ h:mma'}}</b>:
<span>{{'1288323623006' | date:'MM/dd/yyyy @ h:mma'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:"MM/dd/yyyy 'at' h:mma"}}</b>:
<span>{{'1288323623006' | date:"MM/dd/yyyy 'at' h:mma"}}</span>
</p>
</div>
</body>
</html>
6. json
The json filter is used to convert an object into a JSON format string.
Usage in the HTML:
{{ json_expression | json : spacing}}
Cách sử dụng trong Javascript:
$filter('json')(object, spacing)
Parameters:
object | * | Any JavaScript object (including arrays and primitive types) to filter. |
spacing (optional) | number | The number of spaces to use per indentation, defaults to 2. |
Example:
json-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.department = {
id : 1,
name: "Sales",
employees : [
{id: 1, fullName: "Tom", gender: "Male"},
{id: 2, fullName: "Jerry", gender: "Male"}
]
};
});
json-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="json-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: json</h3>
<p>
<b ng-non-bindable>{{ department | json : 5 }}</b>:
<pre>{{ department | json : 5}}</pre>
</p>
<p>
<b ng-non-bindable>{{ department | json }}</b>:
<pre>{{ department | json }}</pre>
</p>
</div>
</body>
</html>
7. limitTo
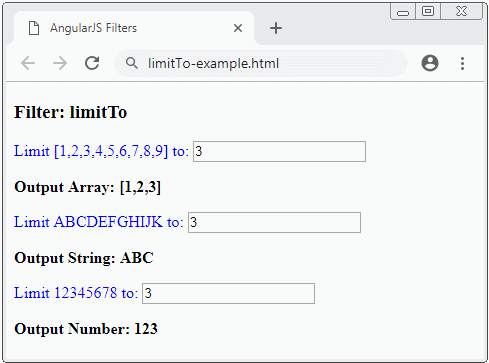
Usage in the HTML:
{{ input | limitTo : limit : begin}}
Usage in the Javascript:
$filter('limitTo')(input, limitLength, startIndex)
Parameters:
Example:
limitTo-example.js
var app = angular.module("myApp", [ ]);
app.controller("myCtrl", function($scope) {
$scope.anArray = [1,2,3,4,5,6,7,8,9];
$scope.aString = "ABCDEFGHIJK";
$scope.aNumber = 12345678;
$scope.arrayLimit = 3;
$scope.stringLimit = 3;
$scope.numberLimit = 3;
});
limitTo-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="limitTo-example.js"></script>
<style>
p {color: blue;}
</style>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: limitTo</h3>
<p>
Limit {{anArray}} to:
<input type="number" step="1" ng-model="arrayLimit">
</p>
<b>Output Array: {{ anArray | limitTo: arrayLimit }}</b>
<p>
Limit {{aString}} to:
<input type="number" step="1" ng-model="stringLimit">
</p>
<b>Output String: {{ aString | limitTo: stringLimit }}</b>
<p>
Limit {{aNumber}} to:
<input type="number" step="1" ng-model="numberLimit">
</p>
<b>Output Number: {{ aNumber | limitTo: numberLimit }}</b>
</div>
</body>
</html>
AngularJS Tutorials
- Introduction to AngularJS and Angular
- Quickstart with AngularJS - Hello AngularJS
- AngularJS Directive Tutorial with Examples
- AngularJS Model Tutorial with Examples
- AngularJS Filters Tutorial with Examples
- AngularJS Events Tutorial with Examples
- AngularJS Validation Tutorial with Examples
Show More