Quickstart with AngularJS - Hello AngularJS
1. Objectives of lesson
There is a small suggestion for you that you should read my introduction post on AngularJS, which will help you understand the birth history of AngularJS as well as distinguishing it from Angular.
The AngularJS has some a few concepts such as App, Controller, Scope ... For each of these concepts, I have a detailed lesson on it, but in fact, to start with the simplest example: "Hello AngualarJS", You also need to understand a bit about at least the above 3 concepts. Therefore, the purpose of this lesson is to practise a small example with AngularJS and explain briefly some of its basic concepts.
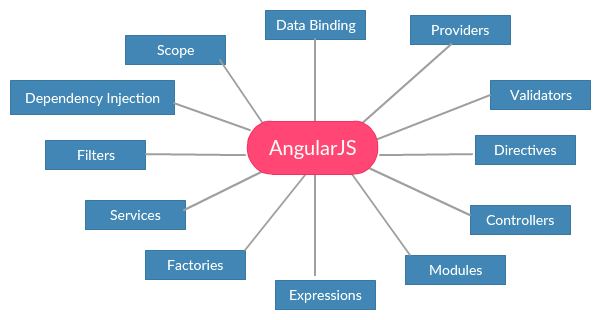
The matters that will be mentioned in this lesson:
- How to download thư the AngularJS library or use it online.
- How to look up the versions of the AngularJS.
- Practize with a "Hello AngularJS" and explain briefly the basic concepts of AngularJS (App, Controller, $scope, Model,.)
2. Download AngularJS
If you want to download the AngularJS, please visit its homepage:
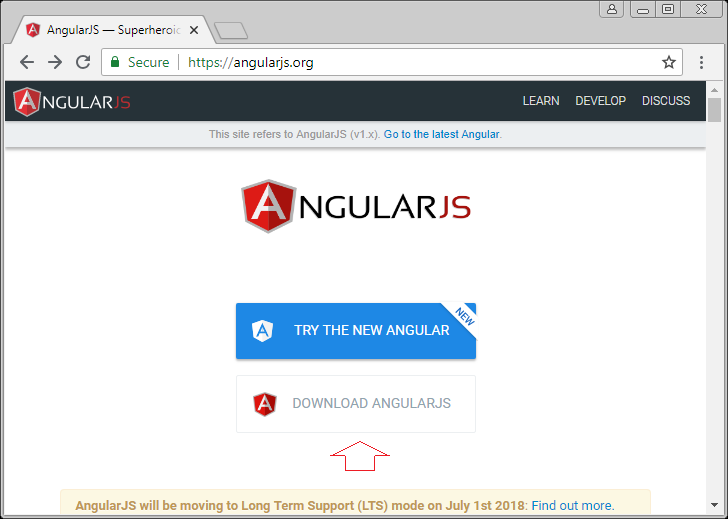
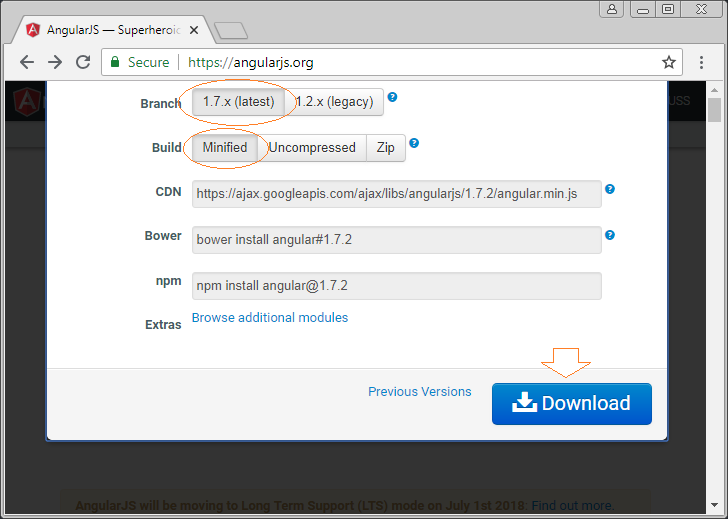
The result of downloading obtained by you is one javascript file.
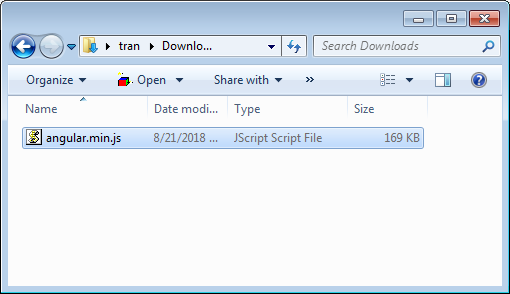
Instead of downloading the AngularJS, you can use sources directly on the Internet.
- https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js
3. Hello AngularJS
In any directory, create hello-angularjs.html file & hello-angularjs.js file. You can use any editing tool, for example, Atom,..
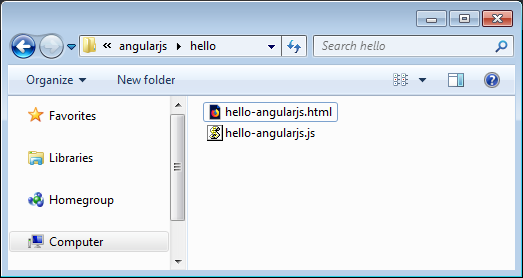
First of all, below is the content of 2 files. The code lines will be explained later.
hello-angularjs.html
<!DOCTYPE html>
<html>
<head>
<title>Hello AngularJS</title>
<!-- Check version: https://code.angularjs.org/ -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="hello-angularjs.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Calculator:</h3>
<p>Variable 1: <input ng-model = "variable1"> </p>
<p>Variable 2: <input ng-model = "variable2"> </p>
<button ng-click = "setOperatorSum()">+</button>
<button ng-click = "setOperatorMinus()">-</button>
<p style="color:blue">{{variable1}} {{ operator }} {{variable2}}</p>
<button ng-click = "calculate()"> = </button>
<p>Result: <b style="color:red">{{ result }}<b></p>
</div>
</body>
</html>
hello-angularjs.js
// Create an Application named "myApp".
var app = angular.module("myApp", []);
// Create a Controller named "myCtrl"
app.controller("myCtrl", function($scope) {
$scope.operator = "+";
$scope.variable1 = 30;
$scope.variable2 = 20;
$scope.result = 0;
$scope.setOperatorSum = function() {
$scope.operator = "+";
}
$scope.setOperatorMinus = function() {
$scope.operator = "-";
}
$scope.calculate = function() {
if ($scope.operator == "+") {
$scope.result = parseFloat($scope.variable1) + parseFloat($scope.variable2);
} else if ($scope.operator == "-") {
$scope.result = parseFloat($scope.variable1) - parseFloat($scope.variable2);
}
}
});
The above example is simply a program for calculation of the sum and difference of two numbers. OK , run the hello-angularjs.html file on the browser and observe how the program works:
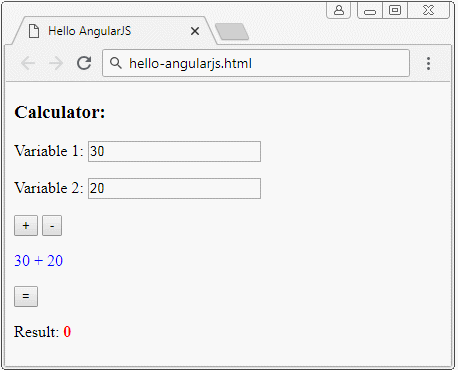
Explain code:
// Create a Application named "myApp".
var app = angular.module("myApp", []);
// Create a Controller named "myCtrl"
app.controller("myCtrl", function($scope) {
// Code
});
- angular is a built-in object in AngularJS. Call the angular.module("myApp",[]) method to create an app named "myApp".
- After there is an app object in the above step, you can create one or more Controller for it, for example, create a Controller with the "myCtrl" name.
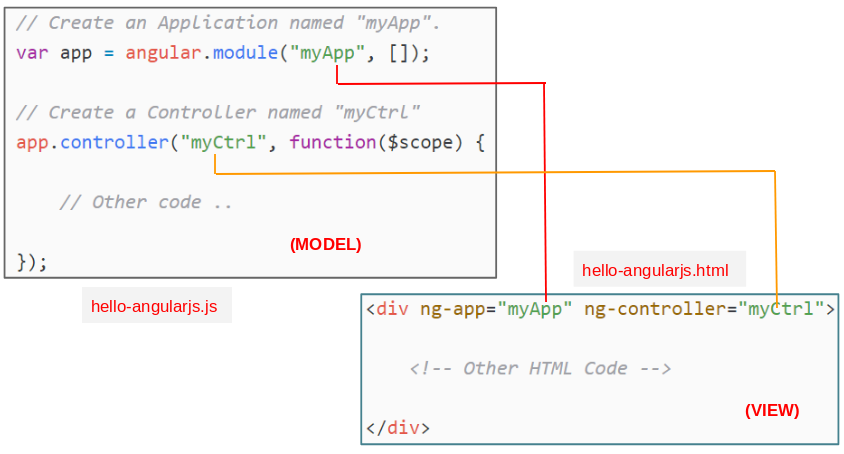
- AngularJS has built in some extended attributes for the tags of HTML, for example, ng-app, ng-controller,..
- The ng-app attribute helps you to establish binding between HTML element and an app object created on Javascript.
- The ng-controller attribute helps you establish a binding between the HTML element and an controller object created on Javascript.
$scope (?)
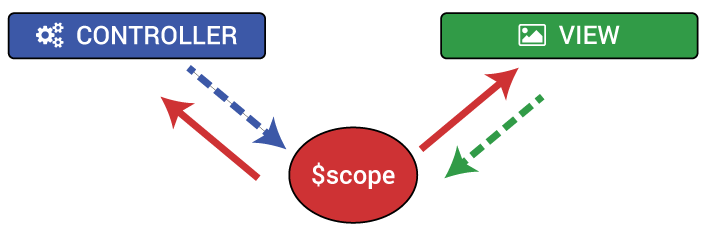
$scope means an object. It is part of binding chain link between HTML (View) and Javascript (Controller). Both View and Controller use this object.
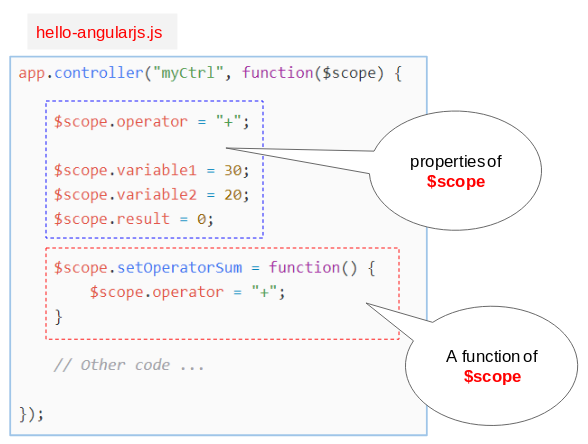
On the Controller, you can define properties for the $scope objects and these properties can be used by View (HTML). You also can create functions for $scope. You can call these functions from View.
{{ expression }}
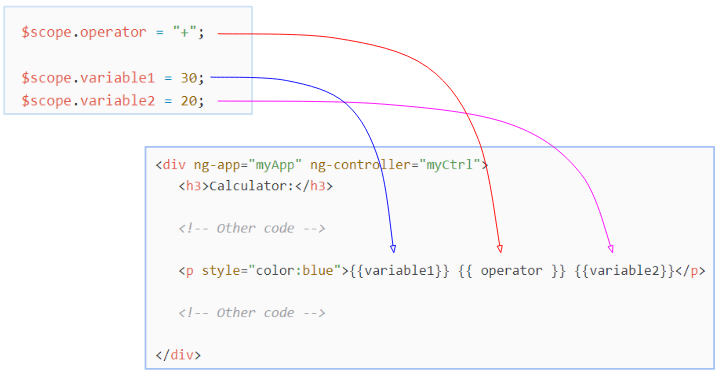
To show the value of $scope.someProperty on View (HTML), you can use {{ someProperty }} expression. On the Controller, if the value of $scope.someProperty changes, it will be updated on View.
ng-model
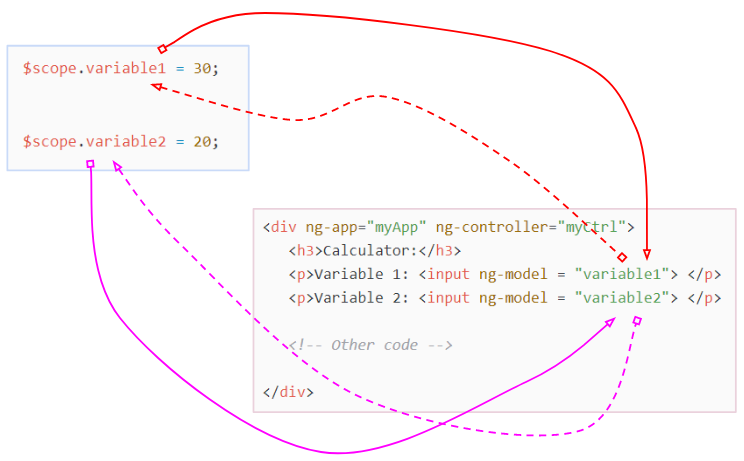
ng-model means an extended attribute of AngularJS. It can be used for the <input>, <select>, <textarea> elements helping with two-way binding between the value of this element and a property of the $scope object (For example, $scope.someProperty).
This means if the value of $scope.someProperty changes, such value will be updated for elements and vice versa, if the value of the element changes, it will be updated for $scope.someProperty.
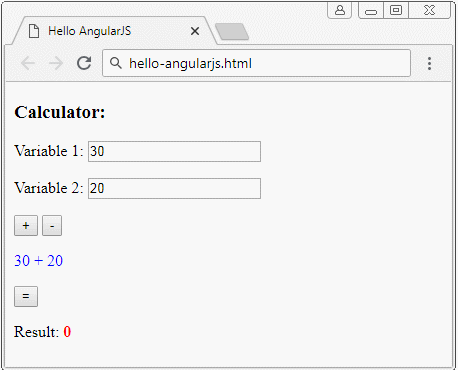
ng-click
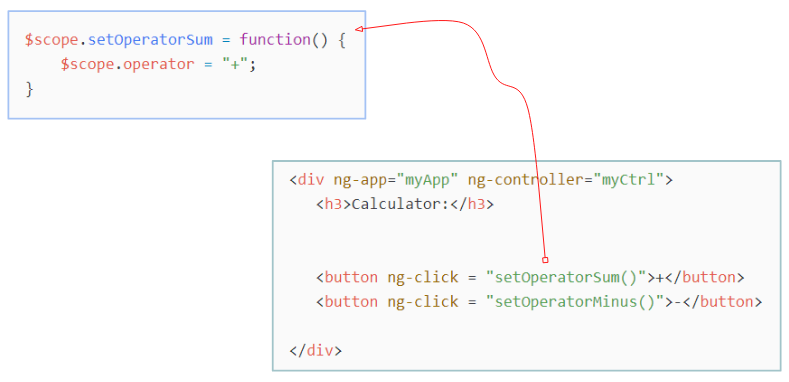
ng-click means an extended attribute of AngularJS. It is the same as the onclick property of HTML. The difference herein is that the onlick calls to a normal Javascript function while the ng-click calls to a function of $scope object.
AngularJS Tutorials
- Introduction to AngularJS and Angular
- Quickstart with AngularJS - Hello AngularJS
- AngularJS Directive Tutorial with Examples
- AngularJS Model Tutorial with Examples
- AngularJS Filters Tutorial with Examples
- AngularJS Events Tutorial with Examples
- AngularJS Validation Tutorial with Examples
Show More