Access Modifier in C#
1. Modifier in Java
The access modifiers in CSharp specifies accessibility (scope) of a data member, method, constructor or class.
There are 5 types of access modifiers in CSharp:
- private
- protected
- internal
- protected internal
- public
2. Override of access modifiers
Modifier | Description |
private | Access is limited to within the class definition. This is the default access modifier type if none is formally specified |
protected | Access is limited to within the class definition and any class that inherits from the class |
internal | Access is limited exclusively to classes defined within the current project assembly |
protected internal | Access is limited to the current assembly and types derived from the containing class. All members in current project and all members in derived class can access the variables. |
public | There are no restrictions on accessing public members. |
The table below illustrates give you an overview of how to use the access modifiers.
Same Assembly | Different Assembly | ||||
Access Modifier | Access within class | Access within package | Access outside package by subclass only | Access outside package and not in subclass. | |
private | Y | ||||
protected | Y | Y | Y | ||
internal | Y | Y | Y | ||
protected internal | Y | Y | Y | ||
public | Y | Y | Y | Y | Y |
You can see more details follow the example below:
3. private access modifier
The private access modifier is accessible only within class.
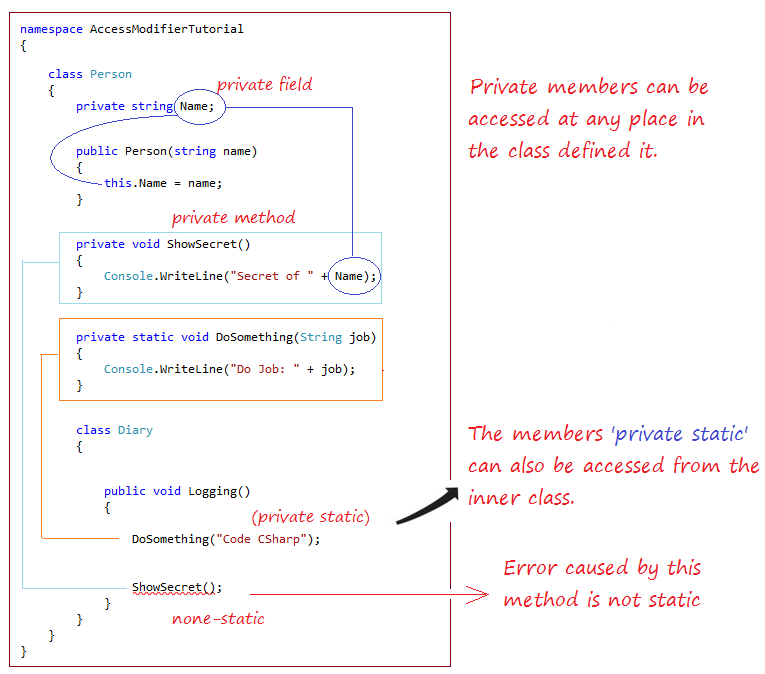
You cannot access to private member outside the class that defines that member. CSharp will notify error at the compile time of the class.
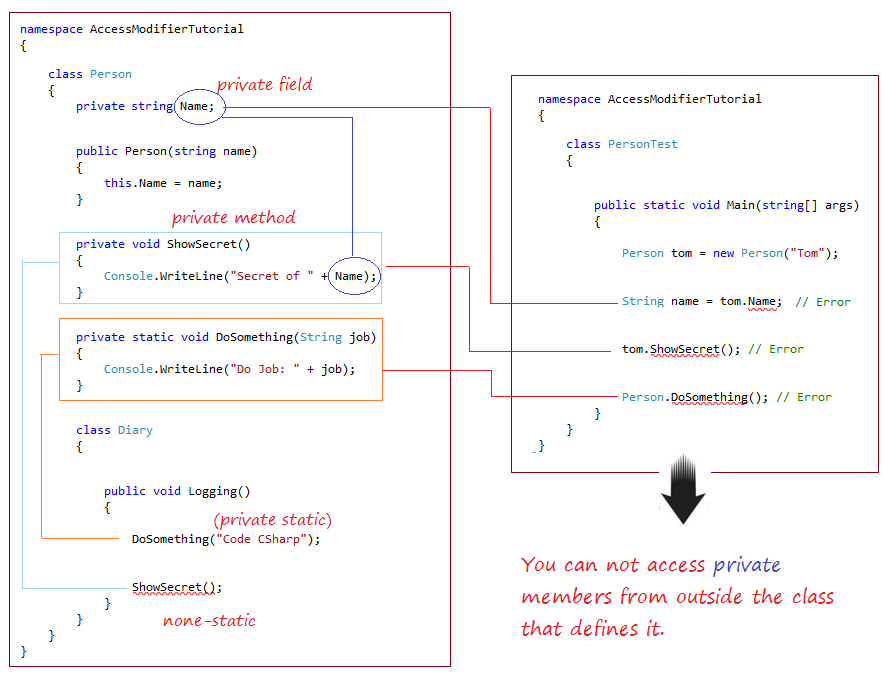
4. private constructor
Constructor, method, field are known as members of the class.
If you create a class and have a private constructor, you cannot create a object of this class from that private constructor, outside this class. Let's see an illustrative example:
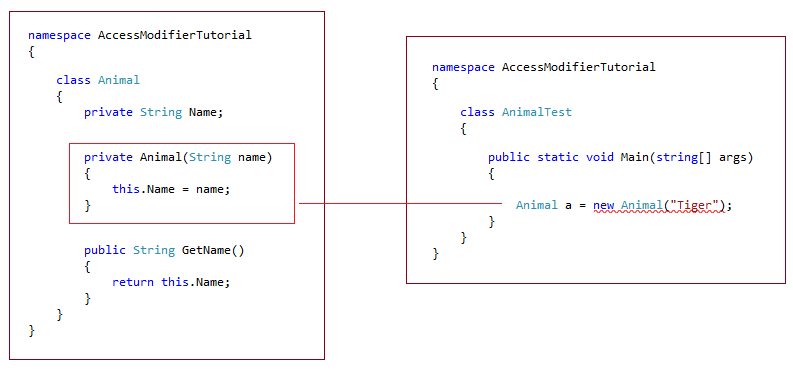
5. protected access modifier
protected access modifier: Access is limited to within the class definition and any class that inherits from the class.
The protected access modifier can be applied on the data member, method and constructor. It can't be applied on the class, interface...
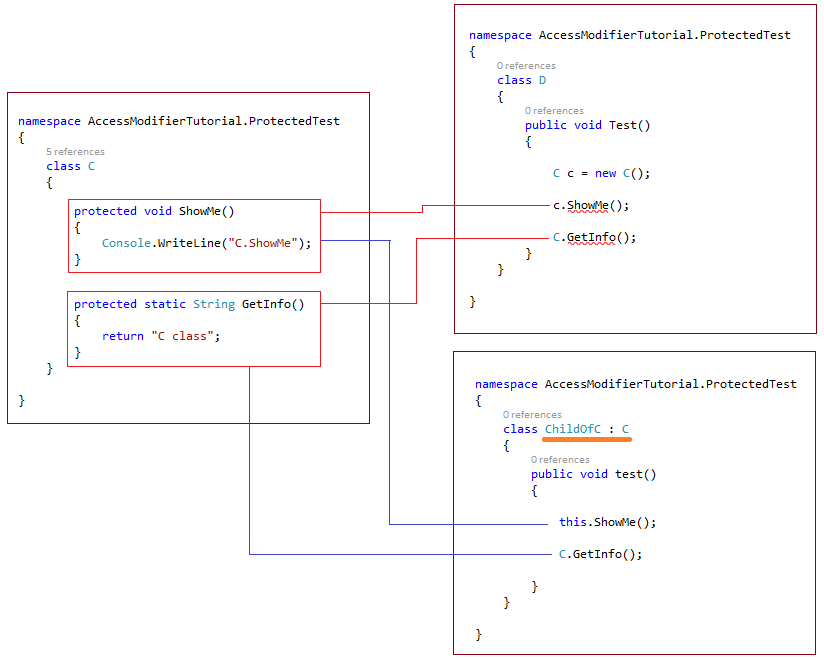
6. internal access modifier
Access is limited exclusively to classes defined within the current project assembly
An assembly is the compiled output of your code, typically a DLL, but your EXE is also an assembly. It's the smallest unit of deployment for any .NET project.
The assembly typically contains .NET code in MSIL (Microsoft Intermediate language) that will be compiled to native code ("JITted" - compiled by the Just-In-Time compiler) the first time it is executed on a given machine. That compiled code will also be stored in the assembly and reused on subsequent calls.
7. protected internal access modifier
protected internal access modifier is a combination of protected and internal access modifier, the member of the class with this access modifier, you can only access to this member in the class that defines it or subclasses and included in the same Assembly.
8. public access modifier
The public access modifier is accessible everywhere. It has the widest scope among all other modifiers.
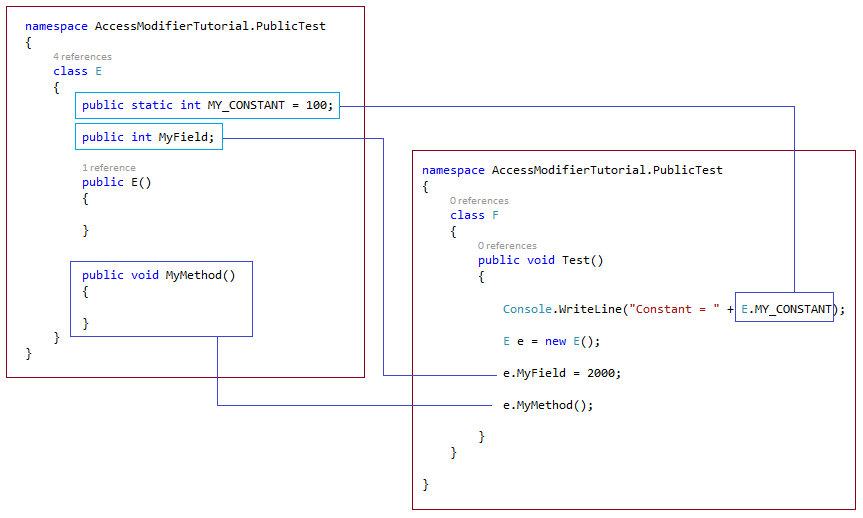
9. Access modifier and inheritance
In CSharp, you can override amethod of superclass with a method of the same name and parameters, and return type in the subclass, but you are not allowed to change its access level.
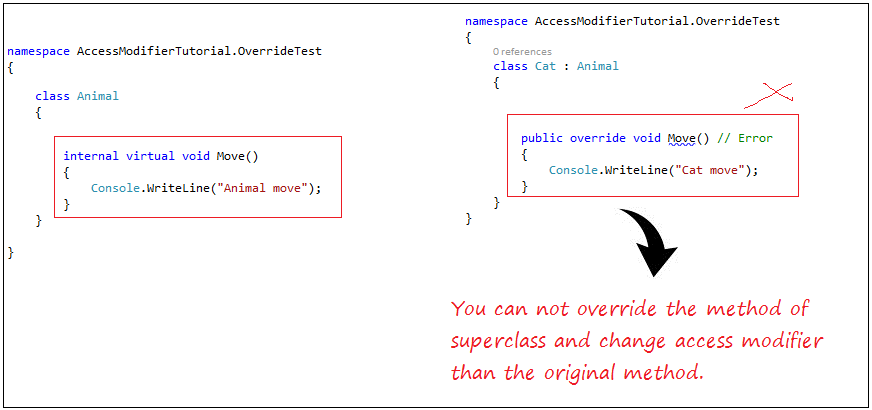
However, you can create a method with the same name, parameters, return type, but different access levels if using the new keyword, the fact that this is a different method unrelated to the method of the parent class.
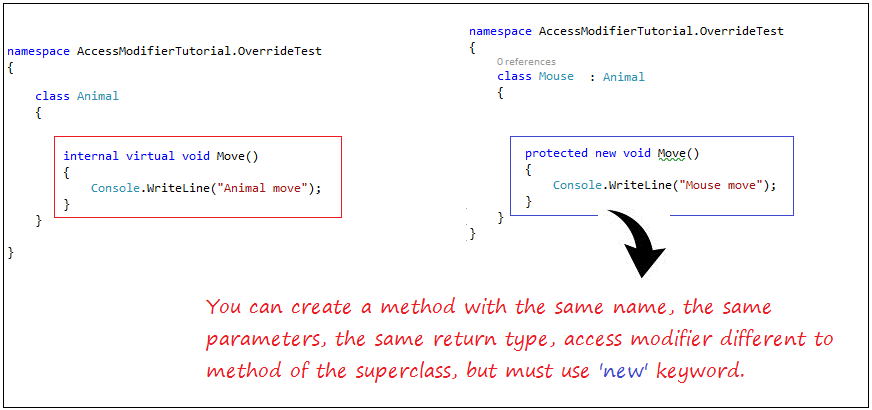
The difference between the overrideandnew has been explained in the document "Inheritance and polymorphism in CSharp" you can look at:
C# Programming Tutorials
- Inheritance and polymorphism in C#
- What is needed to get started with C#?
- Quick learning C# for Beginners
- Install Visual Studio 2013 on Windows
- Abstract class and Interface in C#
- Install Visual Studio 2015 on Windows
- Compression and decompression in C#
- C# Multithreading Programming Tutorial with Examples
- C# Delegates and Events Tutorial with Examples
- Install AnkhSVN on Windows
- C# Programming for Team using Visual Studio and SVN
- Install .Net Framework
- Access Modifier in C#
- C# String and StringBuilder Tutorial with Examples
- C# Properties Tutorial with Examples
- C# Enums Tutorial with Examples
- C# Structures Tutorial with Examples
- C# Generics Tutorial with Examples
- C# Exception Handling Tutorial with Examples
- C# Date Time Tutorial with Examples
- Manipulating files and directories in C#
- C# Streams tutorial - binary streams in C#
- C# Regular Expressions Tutorial with Examples
- Connect to SQL Server Database in C#
- Work with SQL Server database in C#
- Connect to MySQL database in C#
- Work with MySQL database in C#
- Connect to Oracle Database in C# without Oracle Client
- Work with Oracle database in C#
Show More