Java BufferedWriter Tutorial with Examples
1. BufferedWriter
BufferedWriter is a subclass of Writer, which is used to simplify writing text to a character output stream, and improve program performance.
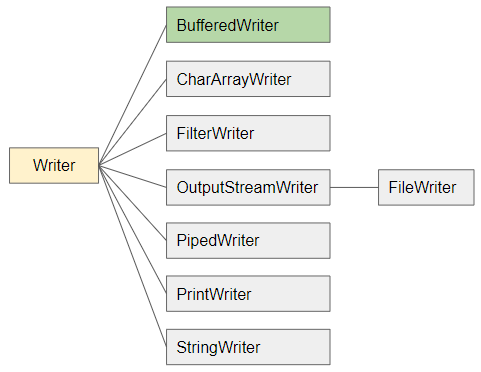
Operating principle of BufferedWriter looks like the following illustration:
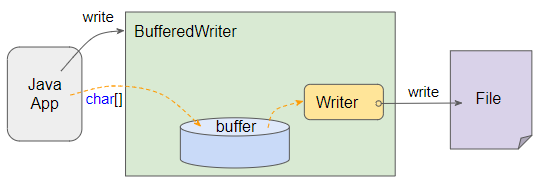
BufferedWriter wraps inside it a Writer object, which is responsible for writing data to the target (such as file).
BufferdWrite overrides methods inherited from its parent class, such as write(), write(char[]),... to ensure that data will be written to buffer and not to target (eg file). But when buffer is full, all of the data in buffer will be pushed into Writer and free buffer. You can also call BufferedWriter.flush() method to actively push all the data on buffer to Writer and clear buffer. Data is also pushed from buffer to Writer when calling BufferedWriter.close() method.
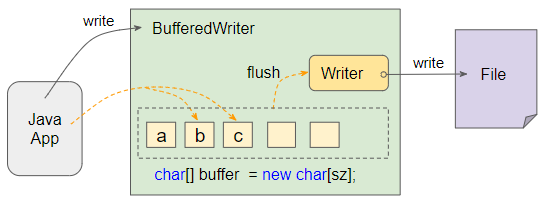
The so-called "buffer" mentioned above is actually just a character array. You can specify the size of this array when initializing BufferedWriter object.
Data that is temporarily written to buffer minimizes continuous writing to target (eg files on a hard drive), thus it enhances the performance of program.
BufferedWriter constructors
BufferedWriter(Writer out)
BufferedWriter(Writer out, int sz)
- BufferedWriter(Write,int) constructor creates a BufferedWriter object with buffer array of specified size.
- BufferedWriter(Writer) constructor creates a BufferedWriter object with buffer array of default size (sz = 8192).
2. Examples
Example: Create a BufferedWriter with buffer array size of 16384. Note: Java's char data type is 2 bytes. That means this buffer is 32786 bytes (32 KB) in size.
File outFile = new File("/Volumes/Data/test/outfile.txt");
outFile.getParentFile().mkdirs(); // Create parent folder.
// Create Writer to write a file.
Writer writer = new FileWriter(outFile, StandardCharsets.UTF_8);
// Create a BufferedWriter with buffer array size of 16384 (32786 bytes = 32 KB).
BufferedWriter br = new BufferedWriter(writer, 16384);
Creates a BufferedWriter with default buffer array size (8192), which is equivalent to 16384 bytes (16 KB).
File outFile = new File("/Volumes/Data/test/outfile.txt");
outFile.getParentFile().mkdirs(); // Create parent folder.
// Create Writer to write a file.
Writer writer = new FileWriter(outFile, StandardCharsets.UTF_8);
// Create a BufferedWriter with default buffer array size of 8192 (16384 bytes = 16 KB).
BufferedWriter br = new BufferedWriter(writer);
Full code of example:
BufferedWriterEx1.java
package org.o7planning.bufferedwriter.ex;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
public class BufferedWriterEx1 {
public static void main(String[] args) throws IOException {
File outFile = new File("/Volumes/Data/test/outfile.txt");
outFile.getParentFile().mkdirs(); // Create parent folder.
// Create Writer to write a file.
Writer writer = new FileWriter(outFile, StandardCharsets.UTF_8);
// Create a BufferedWriter with buffer array size of 16384 (32786 bytes = 32 KB).
BufferedWriter br = new BufferedWriter(writer, 16384);
br.write("Line 1");
br.newLine();
br.write("Line 2");
br.flush();
br.newLine();
br.write("Line 3");
br.close();
}
}
Output:
outfile.txt
Line 1
Line 2
Line 3
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More