Variables in Batch Scripting Language
1. What is a variable?
Variable is a basic concept in computer science. It is a value that can change. Like other languages, Batch also has the concept of variable.
Basically, Batch has 2 kinds of variables:
- Variables are declared in a file through the set command.
- Argument variables are passed from outside when the file is called for execution.
2. Common variables
Batch language does not have a clear concept of data type. Normally, a variable has the value that is a string, including characters after the = sign to the end of the line. (Including whilte spaces if applicable).
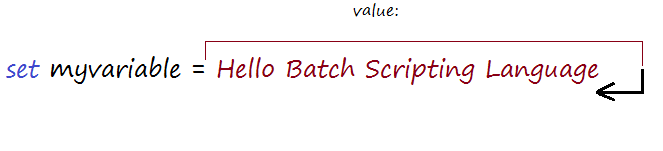
In case you want to declare a variable which is a number, you need to use the set /A statement
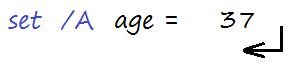
To access value of a variable, you must enclose it in % signs.

Example:
variableExample1.bat
@echo off
set name=Tran
set /A age= 37
echo %name%
echo %age%
set info=My Name is: %name%, Age: %age%
echo %info%
pause
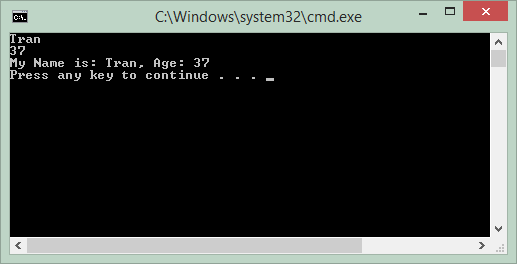
variableExample2.bat
@echo off
set /A a = 100
set /A b = 200
echo a = %a%
echo b = %b%
set /A sum = a + b
echo Sum = %sum%
pause
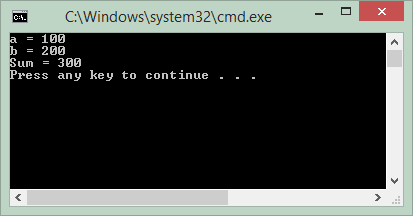
3. Argument variables
When calling to execute a batch file, you can pass values to the file. You can access these values through the variables such as %1, %2, %3,... in the file. These variables are also called argument variables.
argumentVariables.bat
@echo off
@echo First argument is: %1
@echo Second argument is: %2
pause
We test the above example by opening the CMD window and CD to go to the folder containing the argumentVariables.bat file
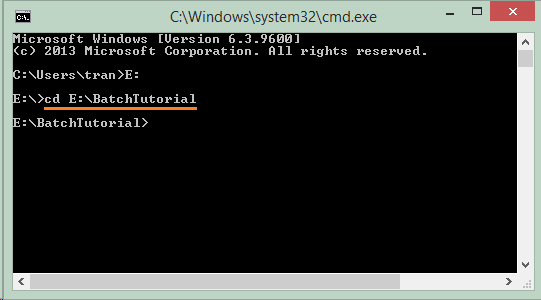
Execute the argumentVariables.bat file with parameters.
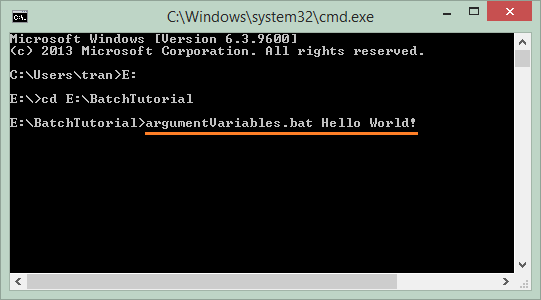
Results:
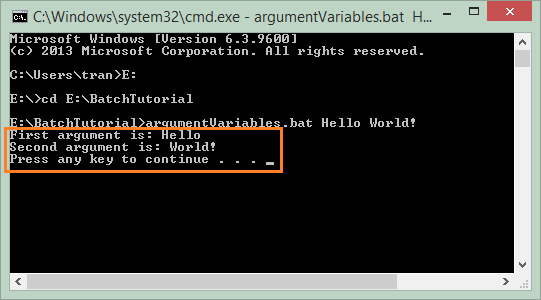
4. Scope of variable
When a Command Window is opened, it will start a session. Such session will finish upon closing the Command Window. During a session you can execute one or more batch files. The variables created in the previous file can be visited in the following file. Such variables are called global variables. However, you can create a local variable. The local variable only exists within the file of defining it or in a section of that file.
Example of Local Variables
Local variables are declared in a block, starting with setlocal and ending with endlocal.
localVariable.bat
@echo off
set global1=I am a global variable 1
setlocal
set local1=I am a local variable 1
set local2=I am a local variable 2
echo ----- In Local Block! -----
echo local1= %local1%
echo local2= %local2%
endlocal
echo ..
echo ----- Out of Local Block! -----
echo global1= %global1%
echo local1= %local1%
echo local2= %local2%
pause
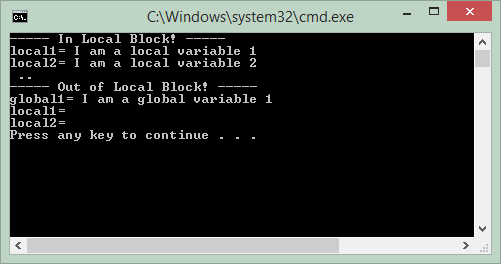
Example of Global Variables:
Variables, declared in the Batch file, and not located in the setlocal .. endlocal block, will be global variables. They can be used in other files in the same session.
In this example, we have two files such as batchFile1.bat and batchFile2.bat. The MY_ENVIRONMENT variable is defined in file 1, and which is used in file 2.
batchFile1.bat
@echo off
set MY_ENVIRONMENT=C:/Programs/Abc;C:/Test/Abc
batchFile2.bat
@echo off
echo In batchFile2.bat
echo MY_ENVIRONMENT=%MY_ENVIRONMENT%
Open CMD, and CD to the folder containing the batchFile1.bat, batchFile2.bat files and run these files respectively.
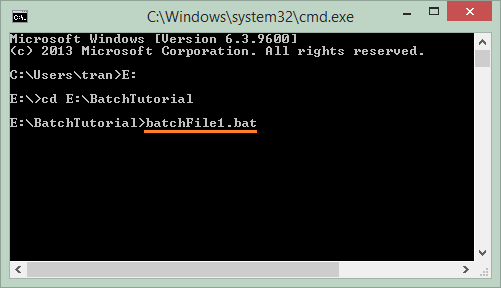
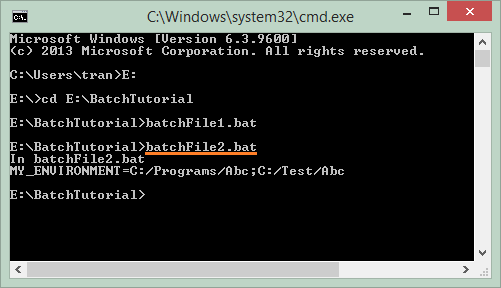
5. The environment variables of Windows
Windows allow you to createenvironment variables. These variables will be global variables which can be used in any batch file.
On the Windows, select:
- Start Menu/Control Panel/System
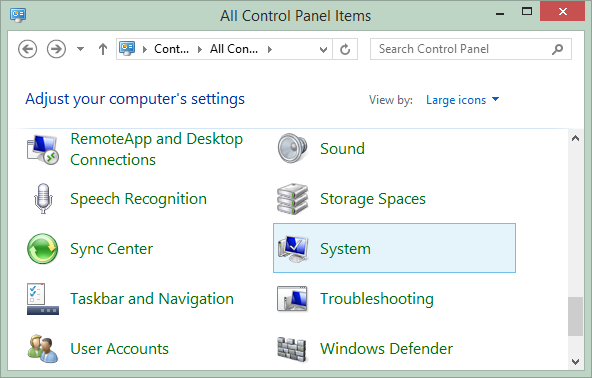
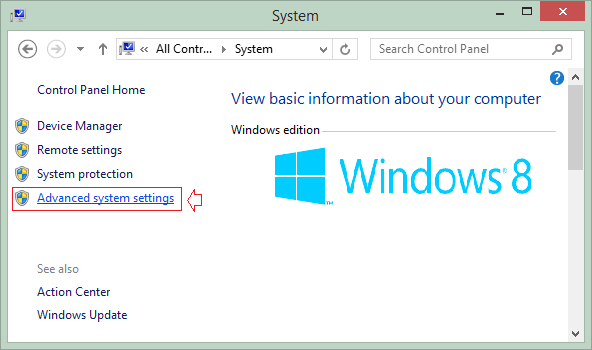
- Advanced (Tab) > Environment Variables..
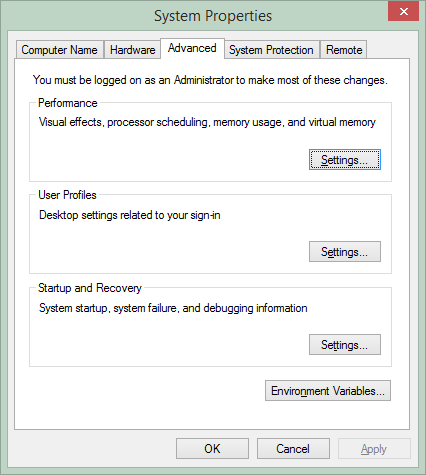
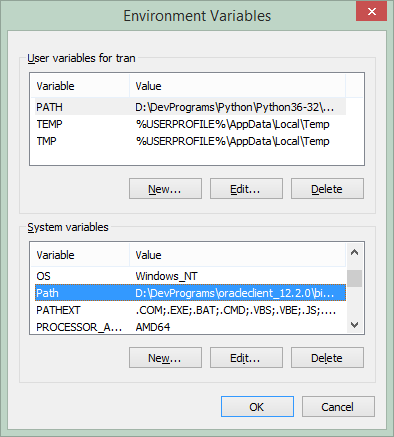
Here, you can create an environment variable for current user, or a system environment variable that can be used by any user.
For example, I create a environment variable with the name of MY_ENVIRONMENT_VARIABLE and its value of "My Environment Variable Value!".
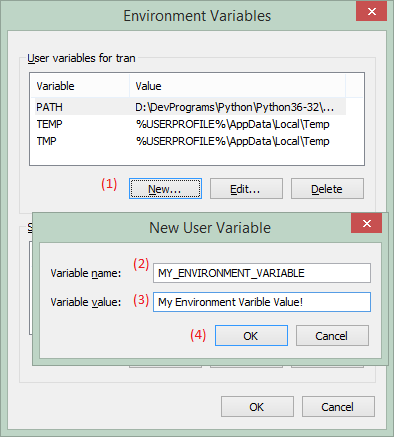
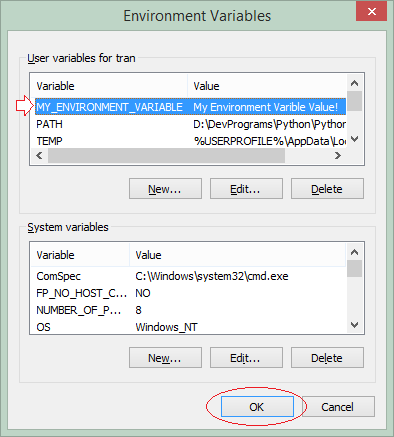
And you can use the environment variable created in a batch file.
testEnvVariable.bat
@echo off
echo %MY_ENVIRONMENT_VARIABLE%
pause
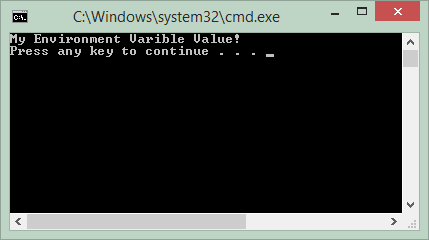