C/C++ strings Tutorial with Examples
1. C-Style String
String
In C++ there are two types of strings, C-style strings, and C++-Style strings.
C-style strings are really arrays, but there are some different functions that are used for strings, like adding to strings, finding the length of strings, and also of checking to see if strings match.
The definition of a string would be anything that contains more than one character strung together. For example, "This" is a string. However, single characters will not be strings, though they can be used as strings.
Strings are arrays of chars. String literals are words surrounded by double quotation marks.
// Declare a C-Style String.
char mystring[] = { 't', 'h', 'i', 's', ' ', 'i', 's' ,' ', 't', 'e', 'x', 't', '\0'};
// This is a string literal.
char mystring[] = "this is text'';
StringLiteralExample.cpp
#include <stdio.h>
int main() {
// Declare a String literal.
char s1[] = "What is this";
// Print out the string
printf("Your string = %s", s1);
fflush(stdout);
return 0;
}
Running the example:
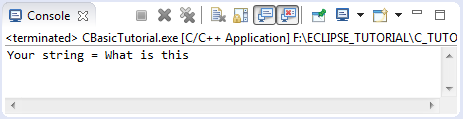
- char mystr[] = "what is this";
Above string has 12 characters, to declare that string in C you need to declare an array of characters with 13 elements, remember that the last element in the array is null character (the code is '\ 0'), it means that the end of the string. The last character does not mean in your string, but it is necessary for C programs, such as pointer point to a location of the string it will stand at the first character, and want to retrieve real content of string, program will browse to the next element until it encounters the null character.
In case you have an array of characters and include null character which is not at the end of the array, or more null characters in the array. But C will only see this array contains a string, including the first character to the first null character in the array.
The following example declares a character array which has 100 elements used to store the text as user input from the keyboard. In this situation the characters of the input string will be assigned to the first elements of the array and then the null character. And the next element can not be assigned

StringFromKeyboardExample.cpp
#include <stdio.h>
int main() {
// Declare an array of characters with 100 elements,
// used to store user input string from the keyboard.
char s1[100];
printf("Enter your string: \n");
fflush(stdout);
// scanf function wait user input from the keyboard.
// (Press enter to end).
// It will scan to get a string and assigned to the variable s1.
// (%s: scan a string does not contain spaces)
scanf("%s", s1);
printf("Your string = %s", s1);
fflush(stdout);
return 0;
}
Running the example:
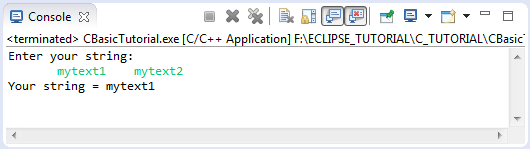
Functions for C-style String
C provides several functions to work with String. It is located in the standard <string.h> library . Below I list some common functions of C relating to string (not all).
Some functions for String:
Function Name | Description |
size_t strlen(const char *str) | Computes the length of the string str up to but not including the terminating null character |
char *strcpy(char *dest, const char *src) | Copies the string pointed to by src to dest.This return a pointer to the destination string dest. |
int strcmp(const char *str1, const char *str2) | Compares the string pointed to by str1 to the string pointed to by str2.
If return value > 0 then str1 > str2, else if returns value = 0 then str1 same as str2, else str1 < str2. |
char *strcat(char *dest, const char *src) | Appends the string pointed to by src to the end of the string pointed to by dest.This function return a pointer to the resulting string dest. |
char *strchr(const char *str, int c) | Searches for the first occurrence of the character c (an unsigned char) in the string pointed to by the argument str. |
StringFunctionsExample.cpp
#include <stdio.h>
// Using string library.
#include <string.h>
int main() {
// Declare a string literal.
char s1[] = "This is ";
// Declare a C-Style string
// (With null character at the end).
char s2[] = { 't', 'e', 'x', 't', '\0' };
// Function: size_t strlen(const char *str)
// strlen funtion return length of the string.
// site_t: is unsigned integer data type.
size_t len1 = strlen(s1);
size_t len2 = strlen(s2);
printf("Length of s1 = %d \n", len1);
printf("Length of s2 = %d \n", len2);
// Declare an array with 100 elements.
char mystr[100];
// Function: char *strcpy(char *dest, const char *src)
// copy s1 to mystr.
strcpy(mystr, s1);
// Function: char *strcat(char *dest, const char *src)
// Using strcat function to concatenate two strings
strcat(mystr, s2);
// Print out content of mystr.
printf("Your string = %s", mystr);
fflush(stdout);
return 0;
}
Running the example:
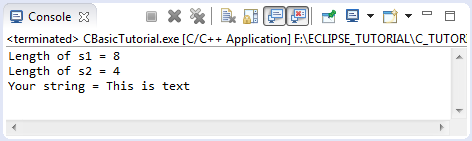
2. C++ Style String
C++ provides string class which helps you work more easily with the string. The methods which string class provide still support for working with the C-Style string.
Declare string library
You need to declare #include <string> preprocessor directives and declare the use of std namespace.
// Declare Preprocessor Directives
#include <string>
// Declare to use the namespace std.
using namespace std;
Declare a string:
// Declare a string object.
string mystring = "Hello World";
// If you do not declare using namespace std.
// You must use the full name:
std::string mystring = "Hello World";
The methods of the String
Below is list methods of String: